An item, also known as a content item, is a reference to a specific type of content in the portal service. An item is created when content is added to the portal. It is used to manage and access the resource. Items can reference many different types of content, such as maps, layers, styles, tools, applications, files, data services, or notebooks. The structure of the content is typically Text/JSON or a reference to a file.
You can use an item to:
- Securely store different types of content in the portal.
- Manage all properties and capabilities of the content.
- Share content with different users and groups.
- Access data and services.
How to work with items
To create and access items, you can use interactive tools, client APIs, or the REST API.
The typical workflow is:
- Create and store an item with tools, such as ArcGIS Online and the developer dashboard.
- Manage your items with tools.
- Access items in the portal service with a client API or the REST API.
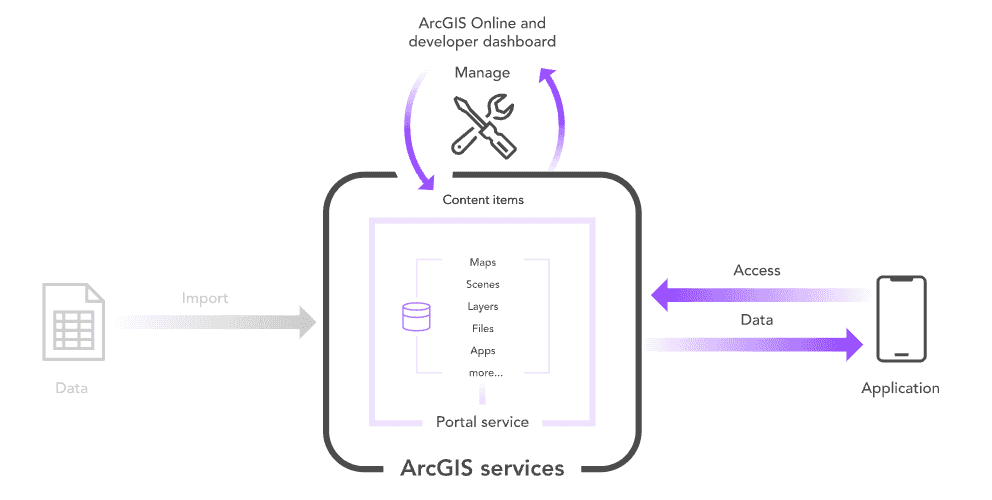
Item types
Many different types of items can be stored in the portal, including maps, layers, styles, tools, applications, files, data sources, or notebooks. Each item has a set of properties that describe and reference the content. For items that reference content that can be stored as text, such as a web map, the JSON data is stored in a child data source. For items that reference services, properties such as the URL
are stored, and depending upon the type of service, child data may also be stored with the item such as a renderer or pop-up configuration.
Below is a list of some of the more common item types, the label used in ArcGIS Online, and the data type.
Type | Label (in ArcGIS Online) | Data Type | Description |
---|---|---|---|
Web Map | Web map | Text/JSON | A map with its configuration stored as a JSON structure. |
Web Scene | Web scene | Text/JSON | A scene with its configuration stored as a JSON structure. |
Feature Service | Feature Layer (hosted) | Text/JSON | A hosted layer that references a feature layer in a feature service. |
Vector Tile Service | Tile Layer (hosted) | Text/JSON | A hosted layer that references tile data and styles for a vector tile service. |
Map Service | Tile Layer (hosted) | Text/JSON | A hosted layer that references static tile data in a Map service. |
CSV | CSV | File | A reference to a file that contains comma separated values. |
Shapefile | Shapefile | File | A reference to a zipped file that contains a shapefile. |
Item properties
All items contain a common set of properties such as the owner
, title
, type
, tags
, thumbnail
, and description
. These properties are common to all types of items and are set when the item is created. The properties are also used to search for the item.
When an item is created, it is assigned a unique identifier, also known as an item ID. You can use an item ID to find and directly access an item and its content in the portal.
Items that reference services can be used to manage the properties and capabilities of that service. For example, you can use a hosted feature layer item to store the pop-up and styling information for a hosted feature layer. It can also be used to set the editing capabilities for the feature layer in the feature service.
An item's sharing permissions can also be set with its access
property, so that it can be accessed by only its owner (private
), the members of an organization (org
), or everyone (public
).
These properties are typically set using an item page. See Manage an item for more details.
Manage an item
The easiest way to access and manage an item is to use an item page. An item page is a web page where you can access item properties through tools such as ArcGIS Online and the developer dashboard.
Item pages
https://www.arcgis.com/home/item.html?id=<ITEM_ID>
Examples:
https://developers.arcgis.com/layers/<ITEM_ID>
Example:
- Public/Private: https://developers.arcgis.com/layers/<ITEM_ID>
Access an item
To access and work with items in the portal, you can use client APIs or the REST API.
Get an item
To access an item and its properties, you can access it by item ID
. The response contains the item properties.
The public example shows how to access a public web map item by its ID. The private example shows how to access any private item that you own by ID.
APIs
const item = new PortalItem({
id: "5a5147abe878444cbac68dea9f2f64e7",
portal: "https://www.arcgis.com/sharing/rest"
});
item.load().then((response)=>{
console.log(response);
});
const portal = new Portal({
url: "https://www.arcgis.com/",
authMode = "immediate"
});
// OR
esriConfig.apiKey = "YOUR_API_KEY" // Scoped to access item below
const portalItem = new PortalItem({
portal: portal,
id: "ITEM_ID"
});
portalItem.load().then((response)=>{
console.log(response);
});
REST API
## Public
curl https://www.arcgis.com/sharing/rest/content/items/5a5147abe878444cbac68dea9f2f64e7 \
-d 'f=pjson'
## Private
curl https://www.arcgis.com/sharing/rest/content/items/<ITEM_ID> \
-d 'f=pjson' \
-d 'token=<ACCESS_TOKEN>'
Response
// Public
{
"id": "5a5147abe878444cbac68dea9f2f64e7",
"owner": "esri_devlabs",
"orgId": "GVgbJbqm8hXASVYi",
"created": 1569537110000,
"modified": 1569537111000,
"guid": null,
"name": null,
"title": "Seven Natural Wonders of the World Map",
"type": "Web Map"
}
// Private
{
"id": "ITEM_ID",
"owner": "OWNER",
"orgId": "ORG_ID",
"created": "DATE_CREATED",
"modified": "DATE_MODIFIED",
"guid": null,
"name": null,
"title": "ITEM_TITLE",
"type": "ITEM_TYPE"
}
Get item data
Items can contain child data. The data is typically stored as text (JSON) or as a reference to services, files, or other resources.
The public example gets the child data for a public web map item. The private example gets the child data for an item that you own. The data returned is the JSON. To create your own private item, see Create a web map.
APIs
const item = new PortalItem({
portal: "https://www.arcgis.com/sharing/rest",
id: "5a5147abe878444cbac68dea9f2f64e7"
});
item.load().then(()=>{
item.fetchData().then((response)=>{
console.log(response);
});
});
const portal = new Portal({
url: "https://www.arcgis.com/",
authMode: "immediate"
});
// OR
esriConfig.apiKey = "YOUR_API_KEY"
const portalItem = new PortalItem({
portal: portal,
id: "ITEM_ID" // private
});
portalItem.load().then(()=>{
portalItem.fetchData().then((response)=>{
console.log(response);
});
});
REST API
## Public
curl https://www.arcgis.com/sharing/rest/content/items/5a5147abe878444cbac68dea9f2f64e7/data \
-d 'f=pjson'
## Private
curl https://www.arcgis.com/sharing/rest/content/items/<ITEM_ID>/data \
-d 'f=pjson' \
-d 'token=<ACCESS_TOKEN>'
// Public
{
"operationalLayers":[
{
"id":"Seven_Natural_Wonders_of_the_World_6051",
"layerType":"ArcGISFeatureLayer",
"url":"https://services.arcgis.com/uCXeTVveQzP4IIcx/arcgis/rest/services/Seven_Natual_Wonders_of_the_World/FeatureServer/0",
"visibility":true,
"opacity":1,
"title":"Seven Natural Wonders of the World",
"itemId":"da7cda8d38224b368bc74cc7d6f05d0f"
}
],
"baseMap":{
"baseMapLayer":[
{
"id":"defaultBasemap",
"layerType":"ArcGISTiledMapServiceLayer",
"url":"https://services.arcgisonline.com/ArcGIS/rest/services/World_Topo_Map/MapServer",
"visibility":true,
"opacity":1,
"itle":"Topographic"
}
],
"title":"Topographic"
},
"spatialReference":{
"wkid":102100,
"latestWkid":3857
},
"authoringApp":"WebMapViewer",
"authoringAppVersion":"5.4",
"version":"2.11"
}
// Private
{
"operationalLayers":[
{
"id":"",
"layerType":"",
"url": "ITEM_URL"
"visibility":true,
"opacity":1,
"title":"YOUR_ITEM_TITLE",
"itemId":"ITEM_ID"
}
],
"baseMap":{
"baseMapLayer":[
{
"id":"defaultBasemap",
"layerType":"ArcGISTiledMapServiceLayer",
"url":"https://services.arcgisonline.com/ArcGIS/rest/services/World_Topo_Map/MapServer",
"visibility":true,
"opacity":1,
"itle":"TILE"
}
],
"title":"BASEMAP_TITLE"
},
"spatialReference":{
"wkid":102100,
"latestWkid":3857
},
"authoringApp":"WebMapViewer",
"authoringAppVersion":"5.4",
"version":"2.11"
}
Search for an item
Use a query to search for items in the portal. If many results are returned, use paging.
The public example searches for all items that contain the string, type, and owner. The private example searches for your private items that are web maps.
APIs
const portal = new Portal({
url: "https://www.arcgis.com/"
});
portal.load().then(()=>{
const query = {
query: "title: Seven Natural Wonders, type: web map, owner: esri_devlabs"
};
portal.queryItems(query).then((response)=>{
console.log(response);
});
});
const portal = new Portal({
url: "https://www.arcgis.com/",
authMode = "immediate"
});
portal.load().then(()=>{
const query = {
query: `type: web map, owner: ${portal.user.username}`
};
portal.queryItems(query).then((response)=>{
console.log(response);
});
});
REST API
## Public
curl https://www.arcgis.com/sharing/rest/search \
-d 'q=title:"Seven Natural Wonders of the World" AND type:"web map" AND owner:"esri_devlabs"' \
-d 'f=pjson'
## Private
curl https://www.arcgis.com/sharing/rest/search \
-d 'q=title:"Seven Natural Wonders of the World" AND type:"web map" AND owner:"<YOUR_USER_ID>"' \
-d 'f=pjson'
-d 'token=<ACCESS_TOKEN>'
{
"query": "title:\"Seven Natural Wonders of the World\" AND type:\"web map\" AND owner:\"esri_devlabs\"",
"total": 1,
"start": 1,
"num": 10,
"nextStart": -1,
"results": [{
"id": "5a5147abe878444cbac68dea9f2f64e7",
"owner": "esri_devlabs",
"created": 1569537110000,
Create an item
You can create and store new items in the portal by specifying the item type and other required parameters. This request requires an access token.
This example creates a web map item.
NOTE: Child data should also be provided to define the JSON for the web map itself. See Create a web map to learn how to create a web map and get the JSON.
APIs
const portal = new Portal();
portal.url = "https://www.arcgis.com/"
portal.authMode = "immediate";
// Prompts for username and password
portal.load()
.then(()=> {
const item = new PortalItem({
title: "World geography",
description: "This is a map of the world",
tags: ["map", "world", "geography"],
type: "Web Map"
});
const params = {
item: item
};
portal.user.addItem(params).then((response)=>{
console.log(response); // Item information
});
});
REST API
curl https://www.arcgis.com/sharing/rest/content/users/<USER_NAME>/addItem \
-d 'f=pjson' \
-d 'title=World geography' \
-d 'description=This is the map of the world' \
-d 'type=Web Map'\
-d 'tags=map, world, geography' \
-d 'token=<ACCESS_TOKEN>'
{
"success": true,
"id": "ITEM_ID"
}
Share an item
To share an item with different users, set the access
property. The scope can be public (everyone), organization (your organization members only), group (private or public group), or private (only you).
You must be the item's owner or an administrator for the organization to change this property.
APIs
from arcgis.gis import GIS
gis = GIS(username="USERNAME", password="PASSWORD")
item = gis.content.get("ITEM_ID")
results = item.share(everyone=True)
print(results)
REST API
curl https://www.arcgis.com/sharing/rest/content/users/<YOUR_ORG>/items/<ITEM_ID>/share \
-d 'everyone=true' \
-d 'org=true' \
-d 'groups=<GROUP_IDs>' \
-d 'f=pjson' \
-d 'token=<ACCESS_TOKEN>'
{"results": [{"itemId": "ITEM_ID",
"success": true,
"notSharedWith": []}]} // Groups item not shared with
Tutorials
Use tools to create different types of content and build content-driven applications.
Web maps
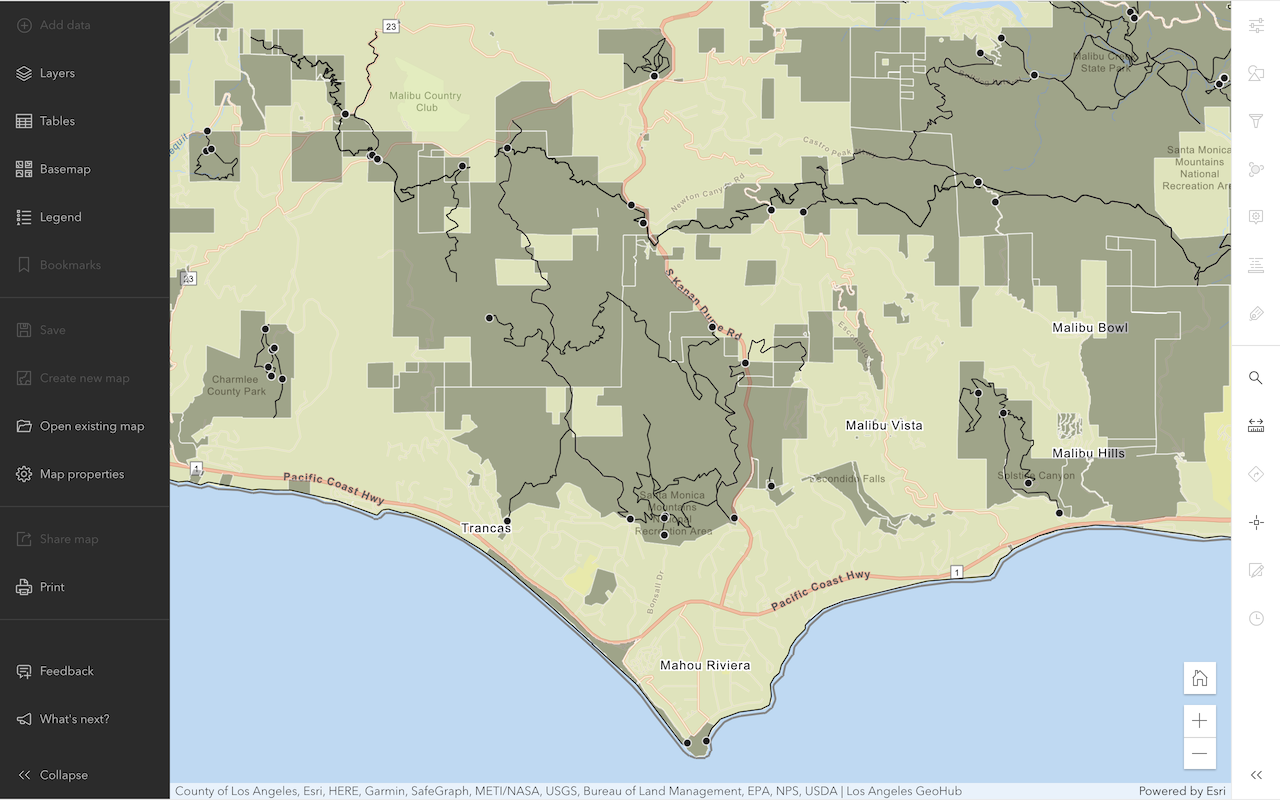
Create a web map
Use Map Viewer to create a web map for your application.
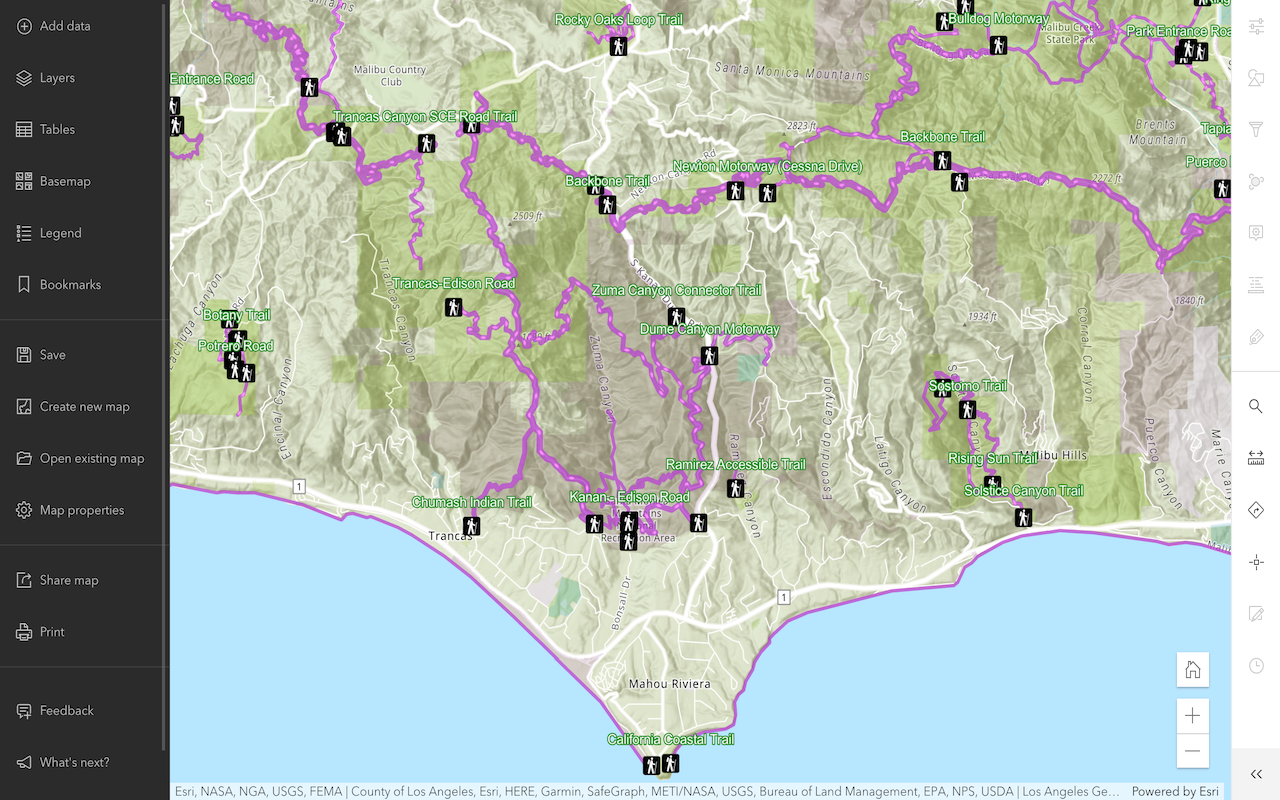
Style layers in a web map
Use Map Viewer to style layers in a web map.
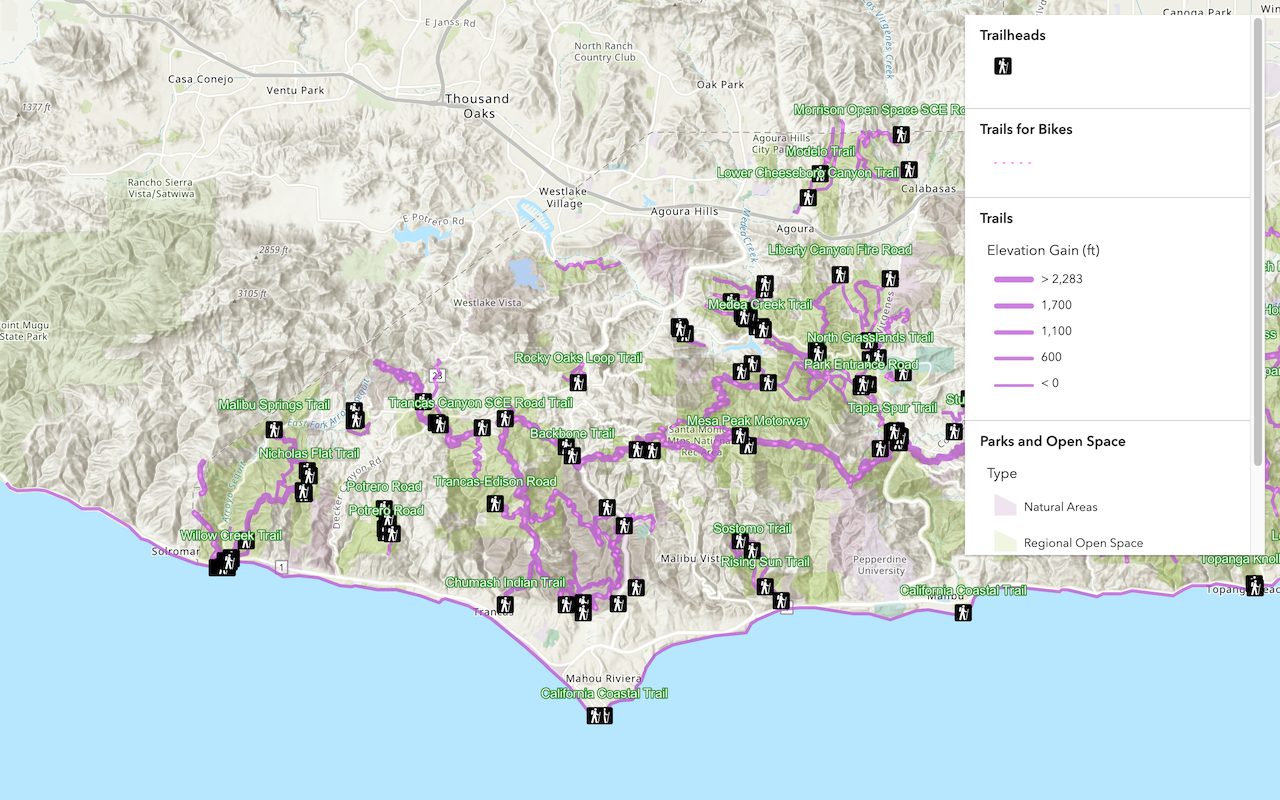
Display a web map
Create and display a map from a web map.
Web scenes
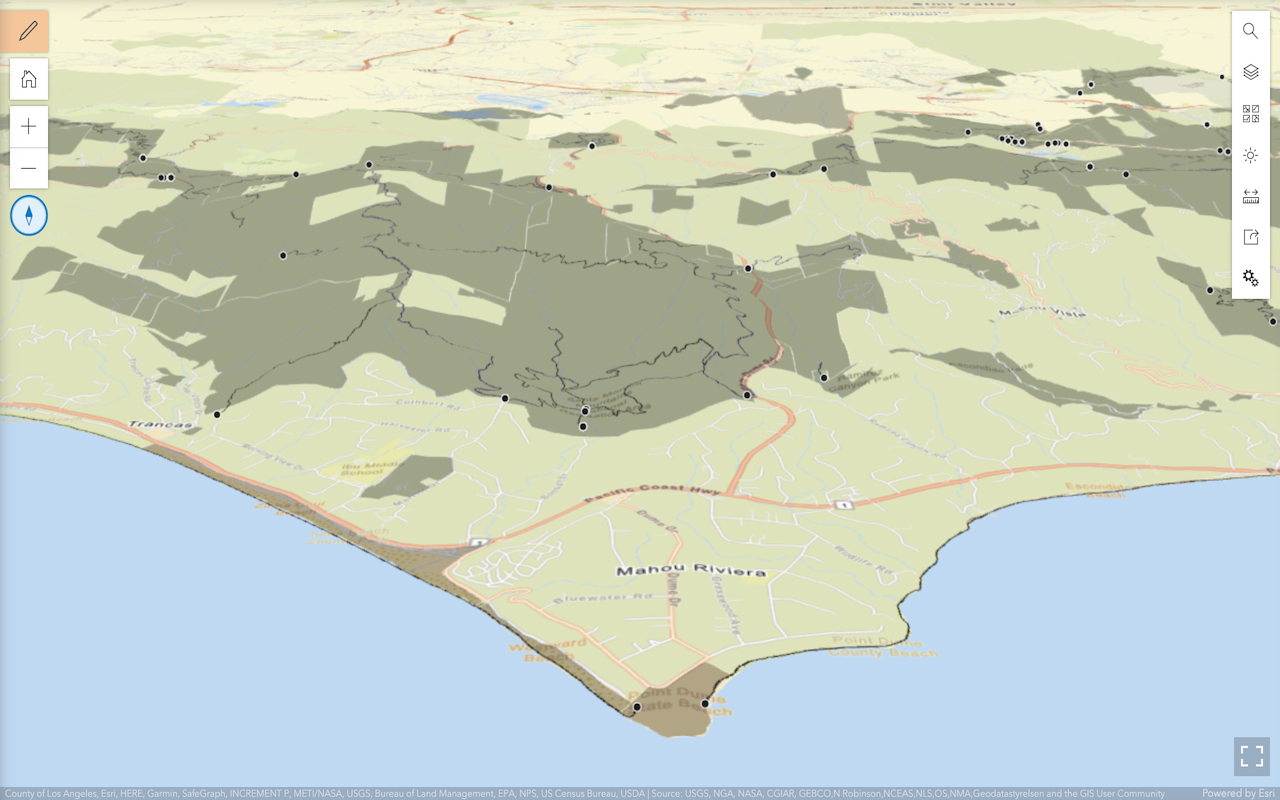
Create a web scene
Use Scene Viewer to create a web scene for your application.
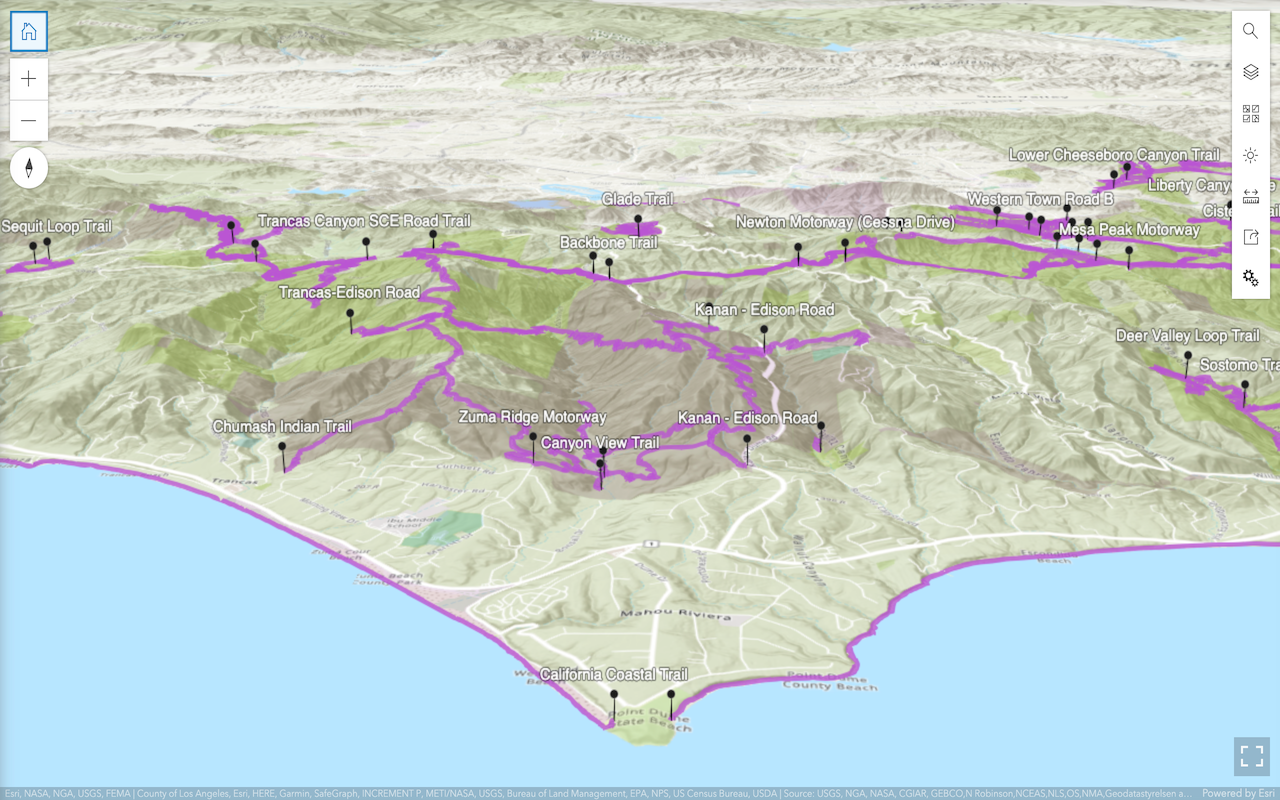
Style layers in a web scene
Use Scene Viewer to style layers in a web scene.
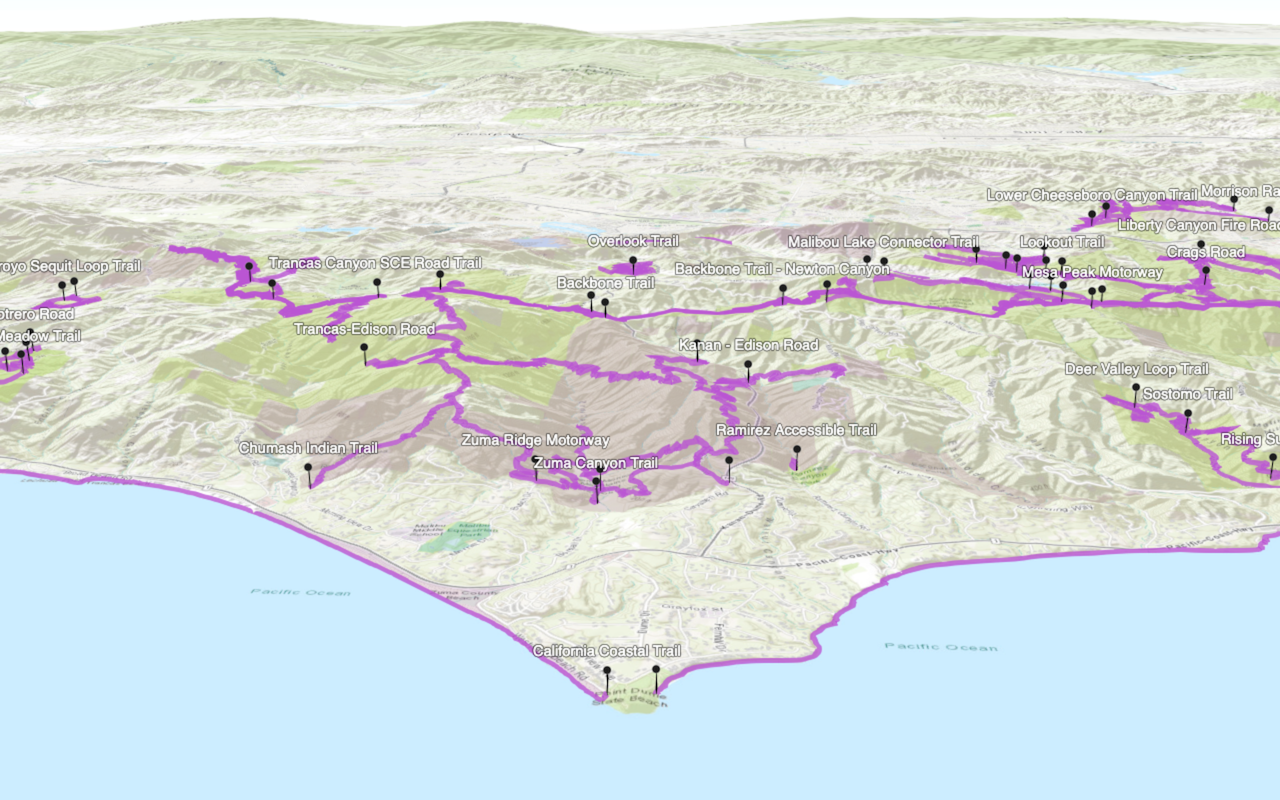
Display a web scene
Create and display a scene from a web scene.
Feature layers
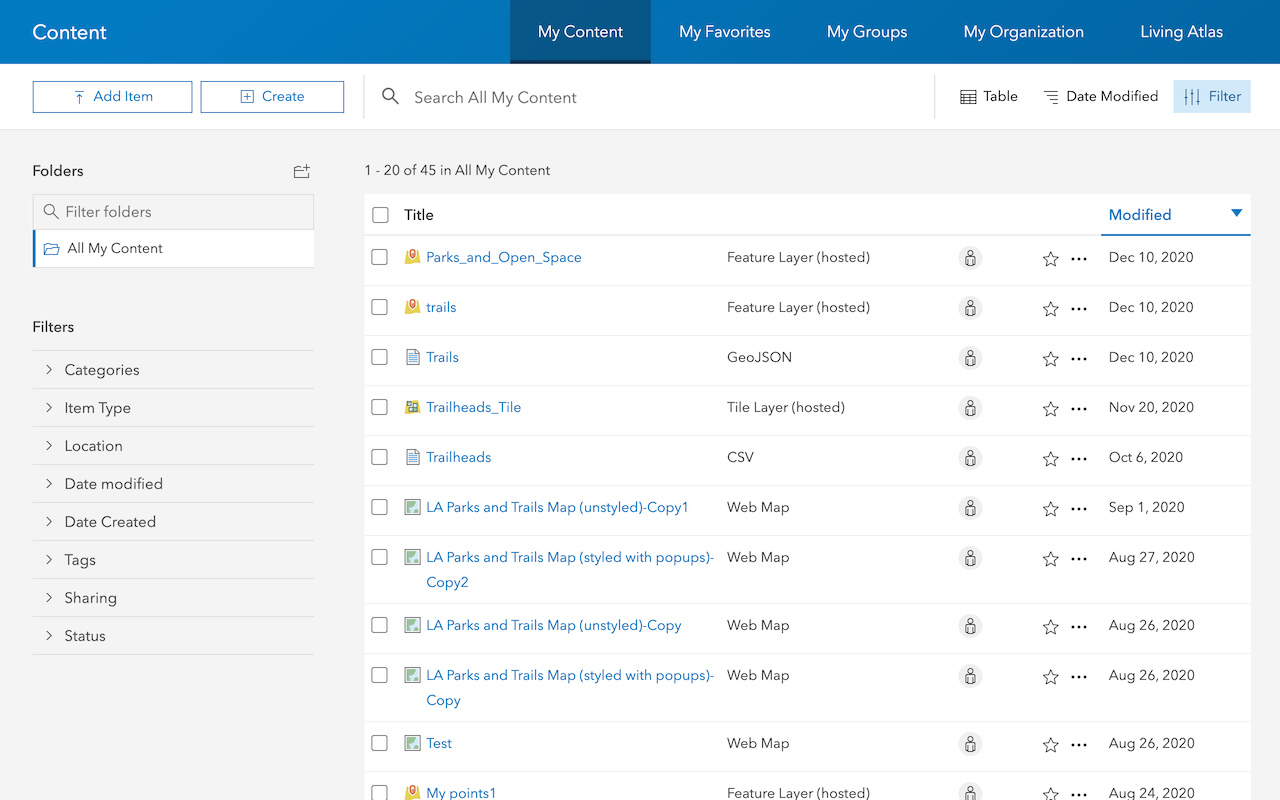
Import data to create a feature layer
Use data management tools to import files and create a feature layer in a feature service.
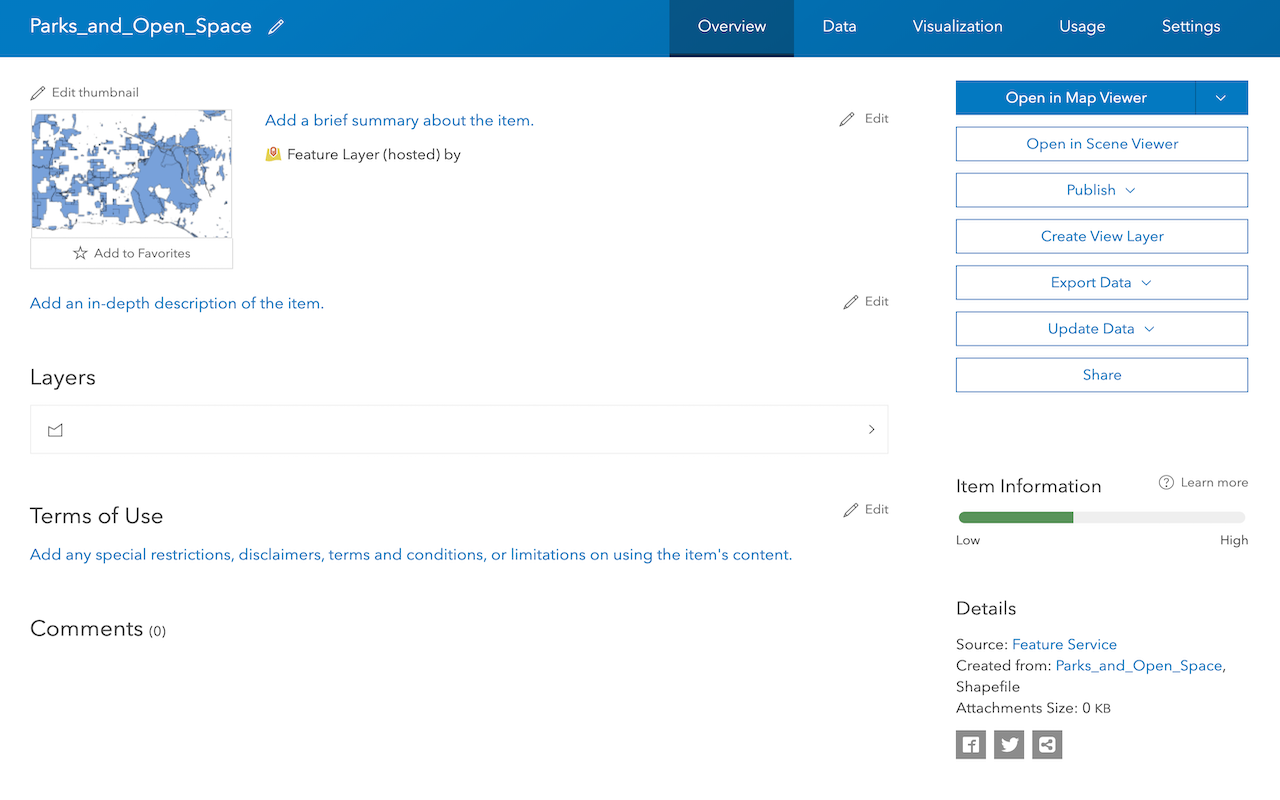
Manage a feature layer
Use a hosted feature layer item to set the properties and settings of a feature layer in a feature service.
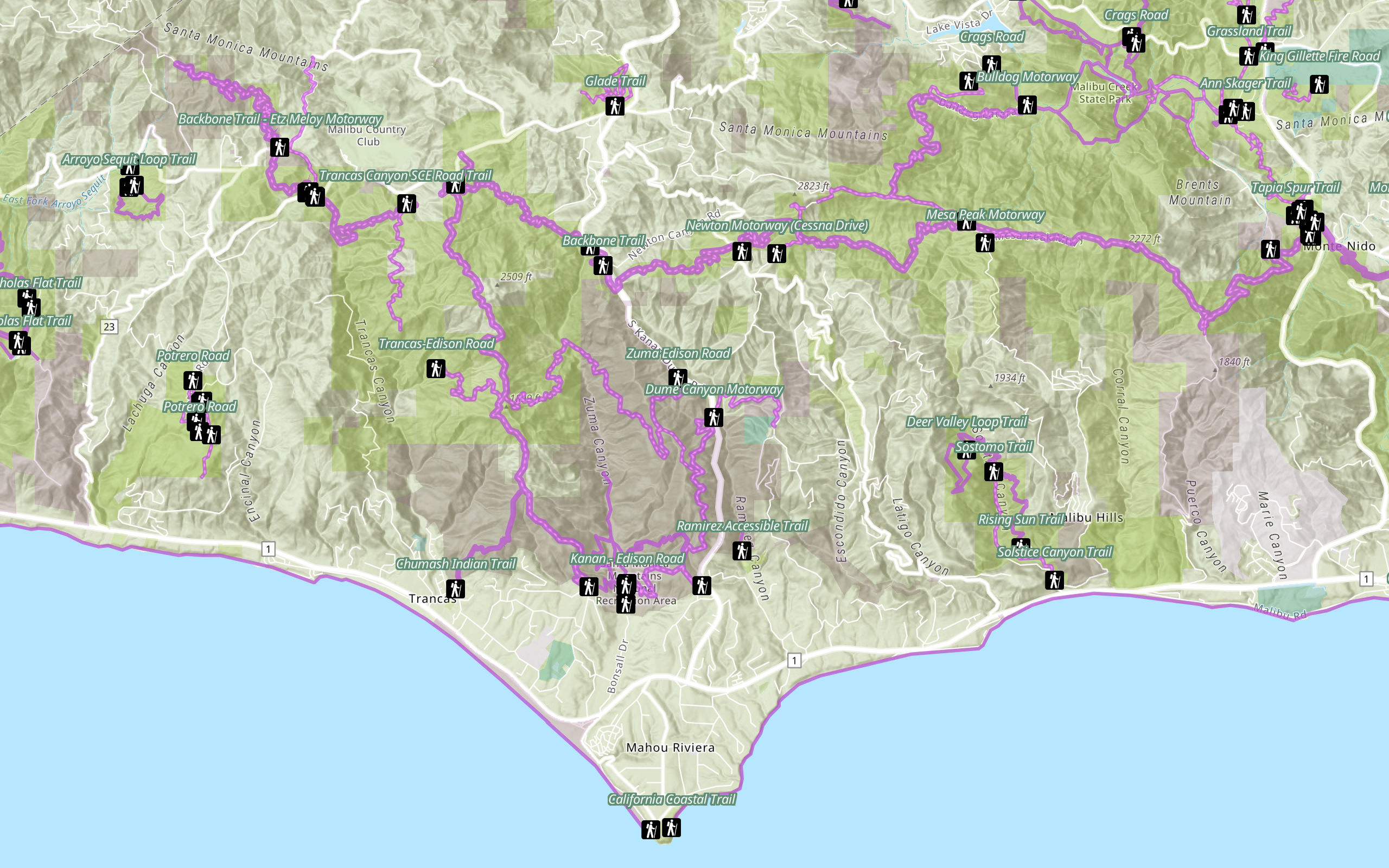
Add a feature layer
Access and display point, line, and polygon features from a feature service.
Vector tile layers
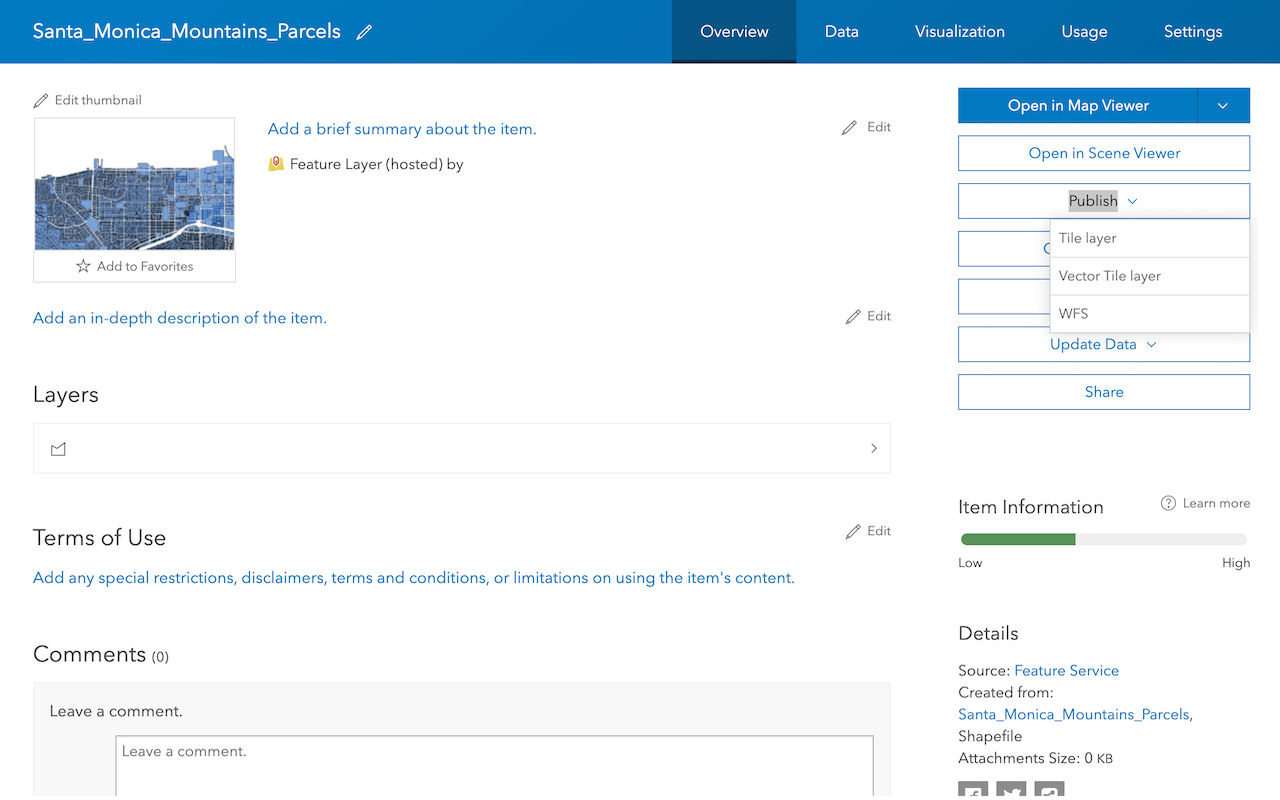
Create a vector tile service
Use data management tools to create a new vector tile service from a feature service.
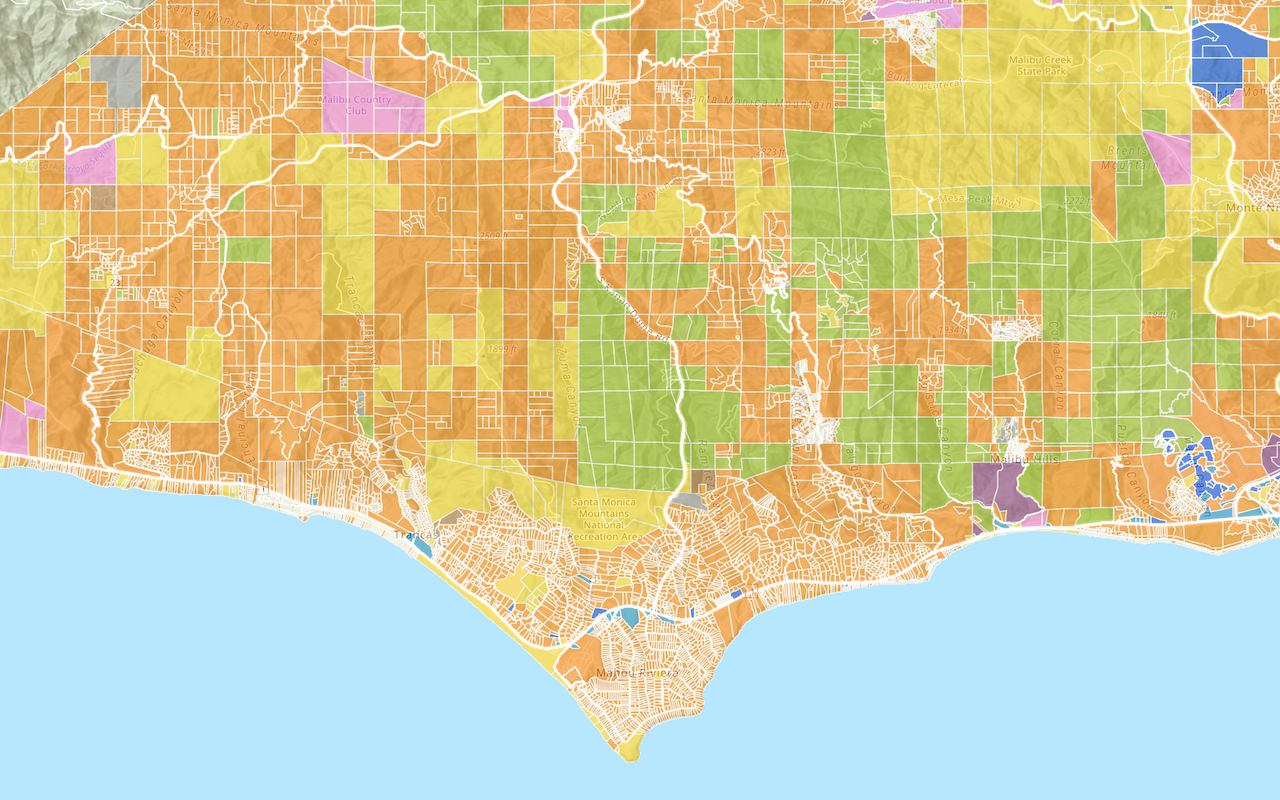
Add a vector tile layer
Access and display a vector tile layer in a map.
Basemap layers
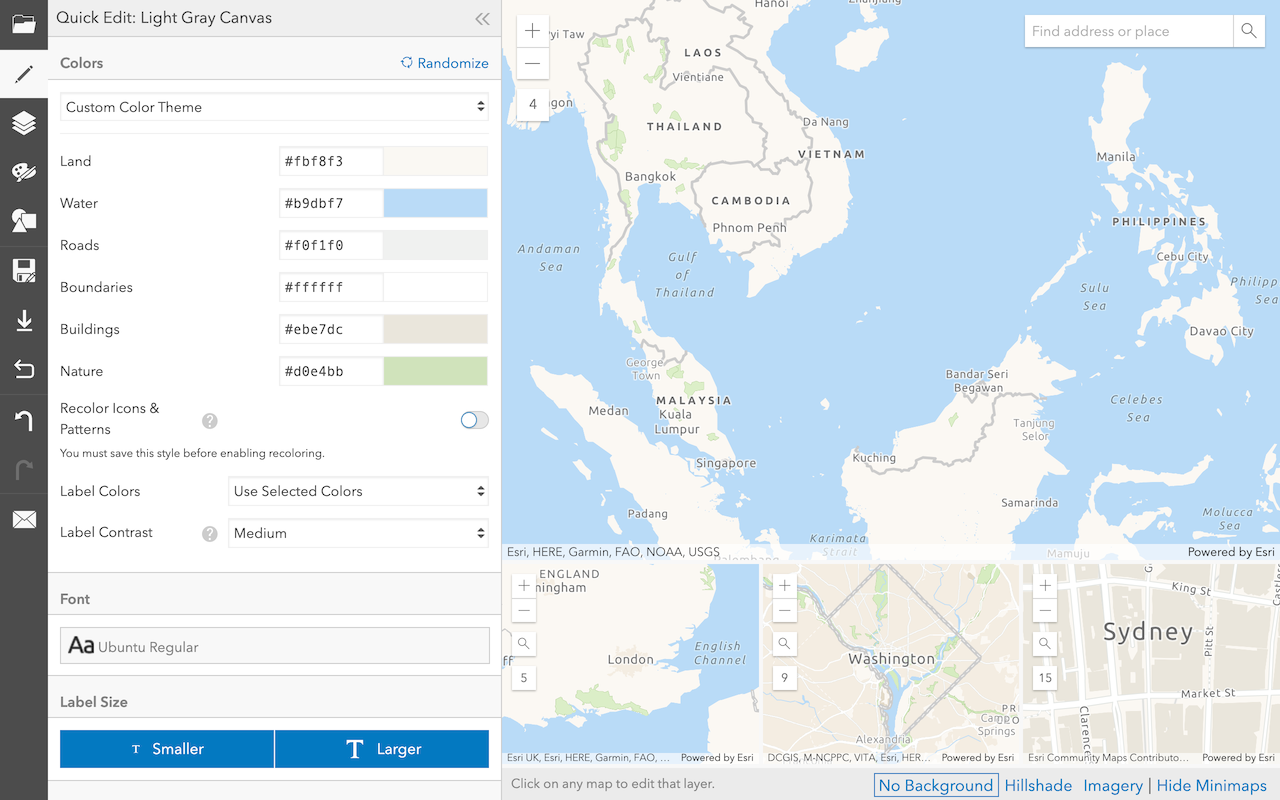
Create a custom basemap style
Use the Vector tile style editor to style a vector tile basemap layer.
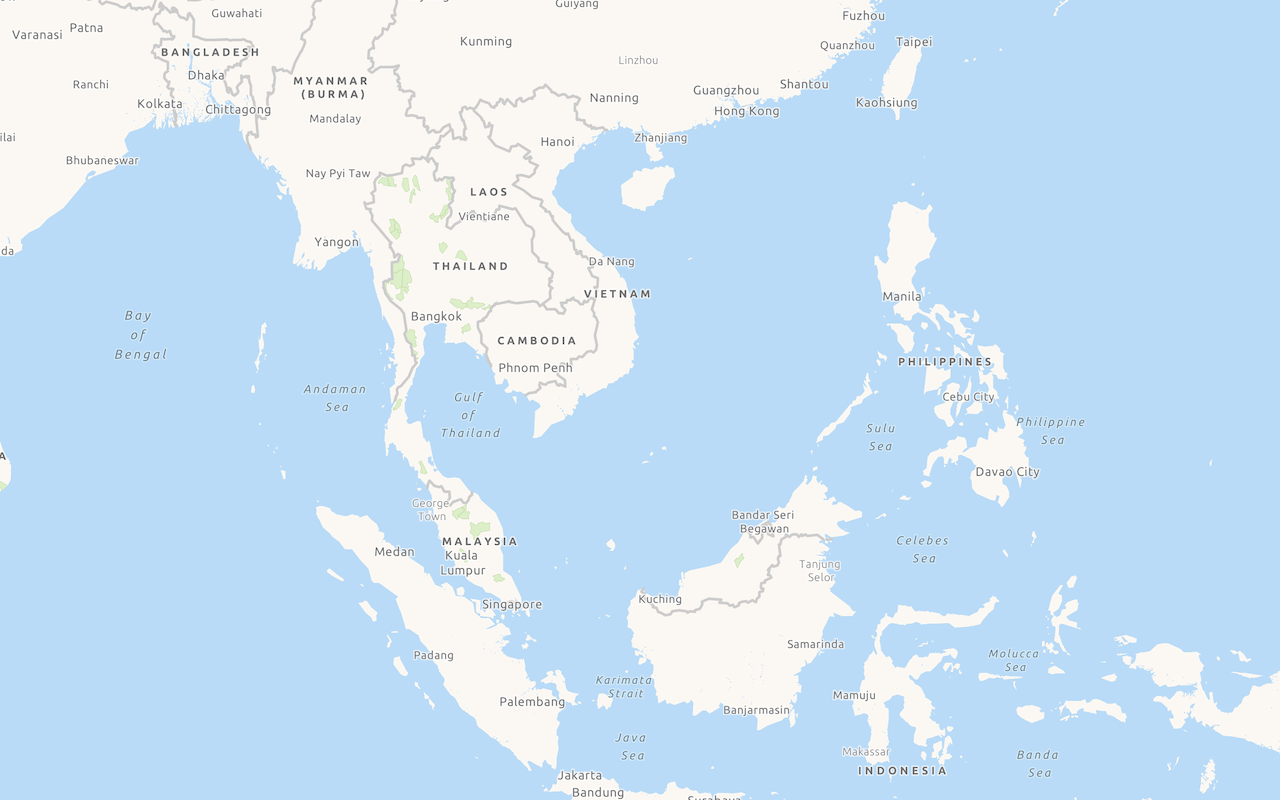
Display a custom basemap style
Add and display a styled vector tile basemap layer.
Services
Portal service
Store, manage, and access private and public content.
API support
- 1. Limited operations, use HTTP requests.
- 2. Access via ArcGIS REST JS.
Tools
Use tools to access the portal and create and manage content for applications.
Developer dashboard
Manage API keys, service usage, and data with the ArcGIS Developers website.
ArcGIS.com
Create, manage, and share content and data with GIS tools.
ArcGIS Enterprise
Create, manage, analyze, and share data, maps, and applications in your organization.
Map Viewer
Create, explore, and share web maps for 2D applications.
Scene Viewer
Create, explore, and share web scenes for 3D applications.
Vector tile style editor
Style vector tile basemap layers for applications.
ArcGIS Pro
Explore, visualize, and analyze both 2D and 3D data with desktop GIS tools.