You can use the portal service to search for items. Item search is the process of querying the portal service to find users, groups, or different types of content such as maps, scenes, layers, files, services, or apps from templates and builders.
You can use content search to:
- Search for public or private items.
- Find different types of items.
- Sort search results.
- Return pages of search results.
How to search the portal
To search for items, you make an HTTPS request to the portal service and use the search
operation. The search
operation supports a query string with fields, and operators, such as AND, OR, and NOT. You can perform an open search for text using the default fields or you can search for text in specific fields such as the title
, description
, or tags
. You can also specify a location to search near, or a bounding box to search in by defining the bbox
parameter.
The general steps to search for items are:
- Define the URL to the portal service:
https:
.//www.arcgis.com/sharing/rest/search - Provide the text for your search. For example, "Streets", or "Elevation level".
- Define the item type for your search. For example,
Web Map
orFeature Service
. - Set the sort order for your return results.
- Any additional fields, such as tags, date created, or last modified.
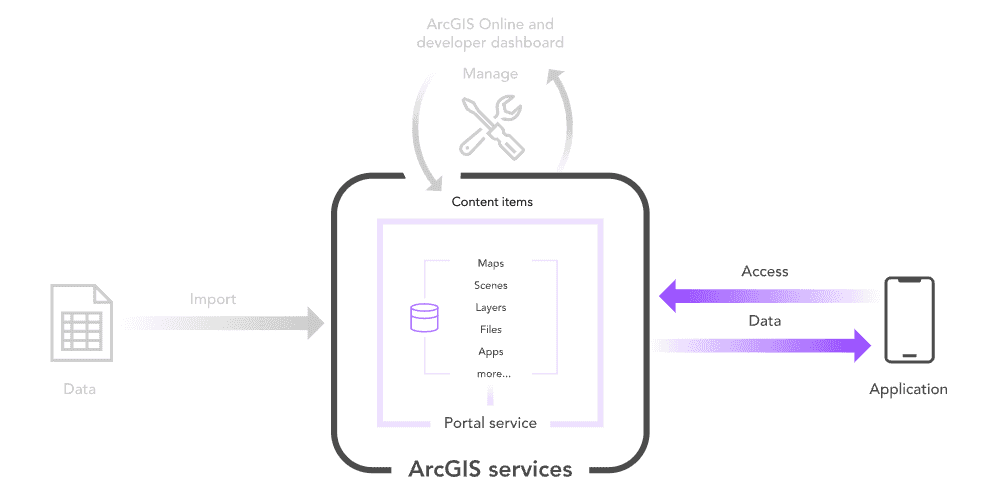
URL request
https://www.arcgis.com/sharing/rest/search?<parameters>
Key parameters
Name | Description | Examples |
---|---|---|
q | The text or formatted query with text. | q="streets", q="streets AND highways", q=(title:"streets" OR description:"streets") |
sort | The primary sort field. | sortField=numviews, sortField=created, sortField=modified |
sort | Return results in ascending or descending order. | sortOrder=asc, sortOrder=desc |
Additional parameters: To refine the search further, you can use parameters such as bbox
, num
, start
, and categories
.
Query structure
Here are a some of the general rules for formatting queries:
- For an open search, specify the text at the beginning. e.g.
q="streets"
- To search specific fields, use a colon
:
after the field name. - Use double quotes
"text"
around all search text. - Capitalize all AND, OR, and NOT operators.
- Use
()
to group operators. - Use the
type:
field to define the type of items to return. e.g.type:
Web Map
For example:
https://www.arcgis.com/sharing/rest/search?f=json&q=title:"London Tube" AND type:"Web Map" AND tags:Transportation
Default fields
When searching for text in items or groups, if you do not provide fields, the following default fields are searched.
Items | Groups |
---|---|
title | title |
tags | tags |
description | description |
snippet | snippet |
tagKeywords | owner |
For example:
https://www.arcgis.com/sharing/rest/search?f=json&q="Seven Natural Wonders of the World"
Code examples
The following examples illustrate how to format queries to search for different types of content in the portal service. Most APIs set the default portal URL to https://www.arcgis.com/sharing/rest.
Search for text
Find any type of item with the keywords title or description.
APIs
portal = new Portal(); // Default is "https://www.arcgis.com/sharing/rest"
portal.load().then(()=>{
const query = {
query: ["title:\"Seven Natural Wonders of the World\" OR description:\"Seven Natural Wonders of the World\""]
};
portal.queryItems(query).then((response)=>{
console.log(response);
});
});
REST API
curl https://www.arcgis.com/sharing/rest/search \
-d 'q=title:"Seven Natural Wonders of the World" OR description:"Seven Natural Wonders of the World"' \
-d 'f=pjson'
{
"query": "title:\"Seven Natural Wonders of the World\" OR description:\"Seven Natural Wonders of the World\"",
"total": 15,
"start": 1,
"num": 10,
"nextStart": 11,
"results": [
{
"id": "1772830e6d4d42c6a9fb12c333d41e1d",
"owner": "olaoluwaatere",
Search for tags and an owner
Find items by searching for tags and an owner name.
APIs
portal = new Portal(); // Default is "https://www.arcgis.com/sharing/rest"
portal.load().then(()=>{
const query = {
query: "tags:\"USA Demographics\" AND owner:\"esri\""
};
portal.queryItems(query).then((response)=>{
console.log(response);
});
});
REST API
curl https://www.arcgis.com/sharing/rest/search \
-d 'q=tags:"USA Demographics" AND owner:"esri"' \
-d 'f=pjson'
{
"query": "tags:\"USA Demographics\" AND owner:\"esri\"",
"total": 36,
"start": 1,
"num": 10,
"nextStart": 11,
"results": [
{
"id": "d77560f788c04f1ea186cbdab654b053",
"owner": "esri",
Search for a type of item
Find items by searching for a type of item. Learn more about the types of items in items.
APIs
portal = new Portal(); // Default is "https://www.arcgis.com/sharing/rest"
portal.load().then(()=>{
const query = {
query: ["title:\"London Map\" AND type:\"Web Map\""]
};
portal.queryItems(query).then((response)=>{
console.log(response);
});
});
REST API
curl https://www.arcgis.com/sharing/rest/search \
-d 'q=title:"London Map" AND type:"Web Map"' \
-d 'f=pjson'
{
"query": "title:\"London Map\" AND type:\"Web Map\"",
"total": 57,
"start": 1,
"num": 10,
"nextStart": 11,
"results": [
{
"id": "1c0ae668422341f298b72d08c1a6ddfe",
"owner": "alexhhorton",
Search for a group
Find groups by searching for a text in the title.
APIs
portal = new Portal(); // Default is "https://www.arcgis.com/sharing/rest"
portal.load().then(()=>{
const query = {
query: "title: Hikers"
};
portal.queryGroups(query).then((response)=>{
console.log(response);
});
});
REST API
curl https://www.arcgis.com/sharing/rest/community/groups \
-d 'q=title:"hikers"' \
-d 'f=pjson'
{
"query": "title:\"hikers\"",
"total": 7,
"start": 1,
"num": 10,
"nextStart": -1,
"results": [
{
"id": "a015574916894dfd8dc2ca2e48272e35",
"title": "Citizen Problem Reporter -Hikers",
Search near a location
Find items near point or a bounding box (extent).
APIs
portal = new Portal(); // Default is "https://www.arcgis.com/sharing/rest"
portal.load().then(()=>{
const query = {
query: "snippet: food cart, type: Web Map",
extent: {
xmax: "-122.6750",
xmin: "-122.6750",
ymax: "45.5051",
ymin: "45.5051"
}
};
portal.queryItems(query).then((response)=>{
console.log(response);
});
});
REST API
curl https://www.arcgis.com/sharing/rest/search \
-d 'bbox=-122.6750,45.5051, -122.6750,45.5051' \
-d 'q="food cart" AND type:"web map"' \
-d 'f=pjson'
{
"query": "\"food cart\" AND type: \"web map\"",
"total": 9,
"start": 1,
"num": 10,
"nextStart": -1,
"results": [
{
"id": "e3fc8d6a8bd44c61868d11ff7ee88978",
"owner": "ross34_pdxedu",
Tutorials
Use tools to create different types of content and build content-driven applications.
Web maps
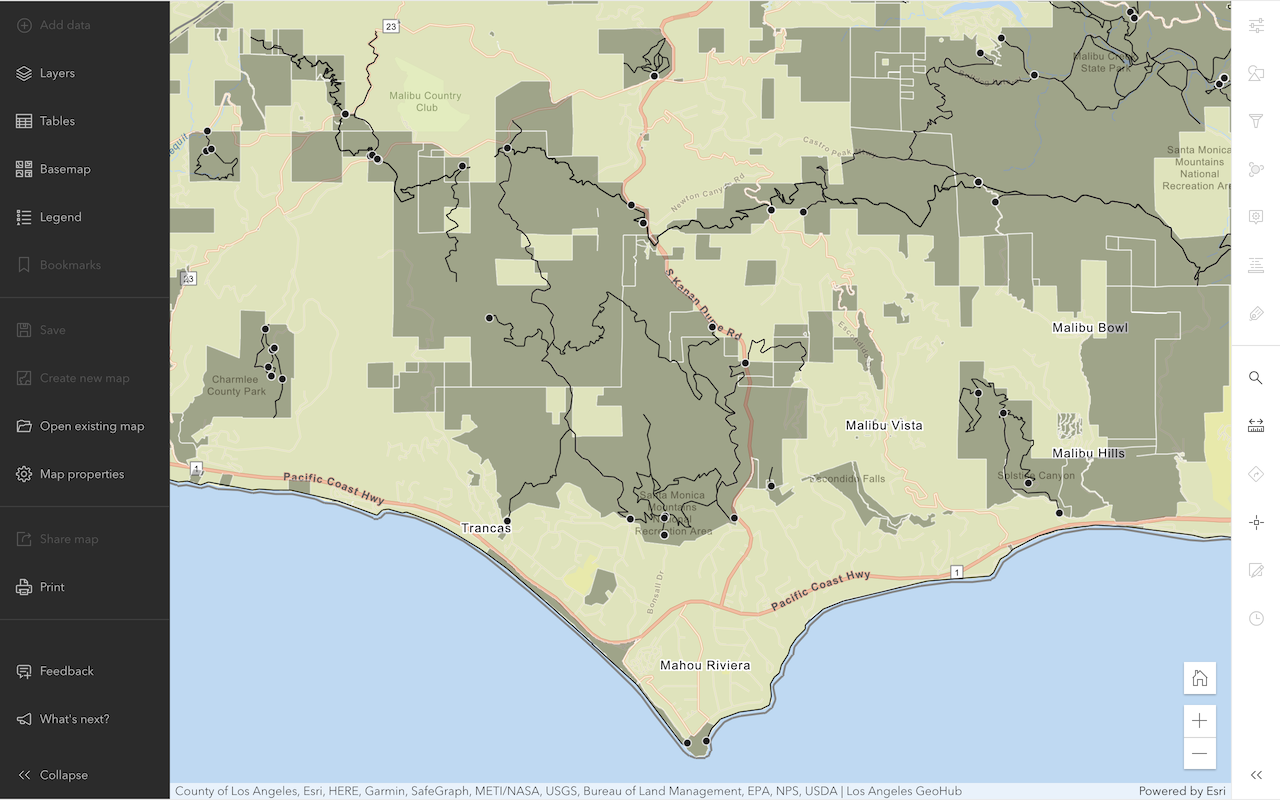
Create a web map
Use Map Viewer to create a web map for your application.
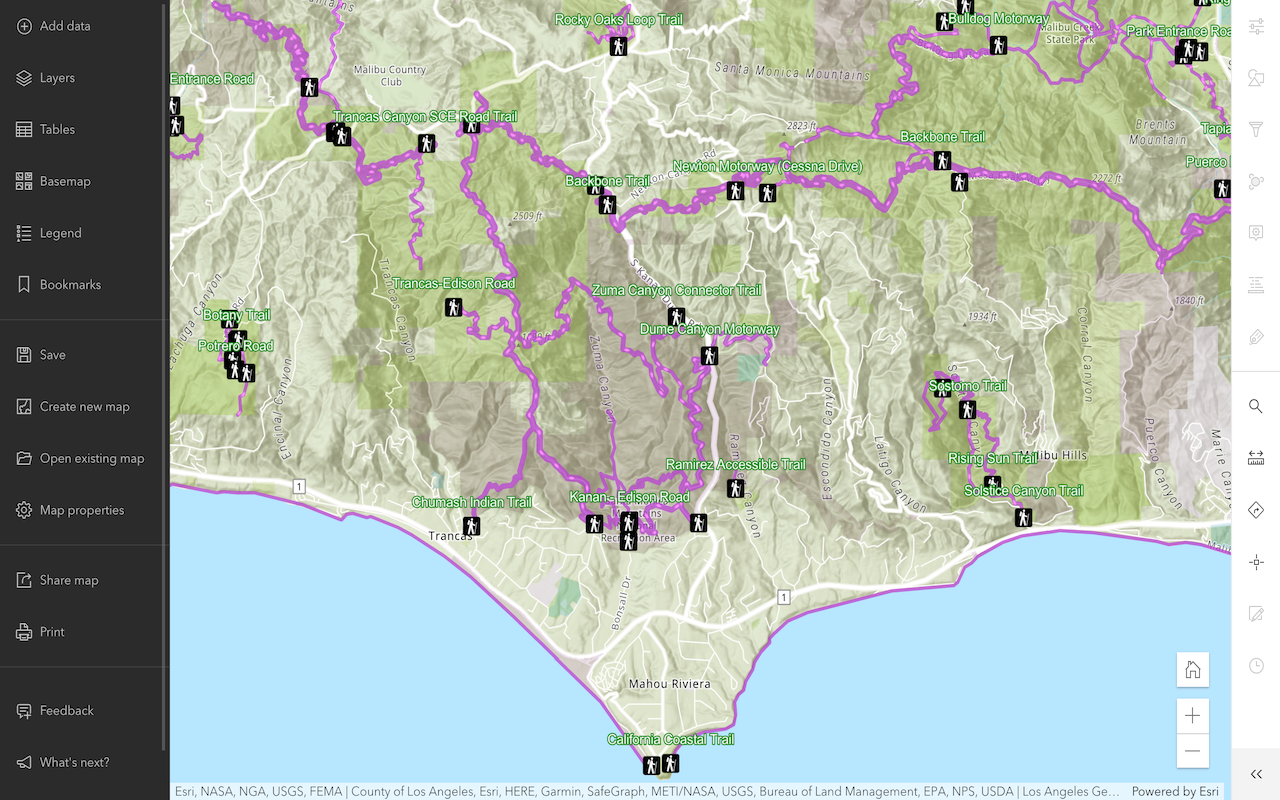
Style layers in a web map
Use Map Viewer to style layers in a web map.
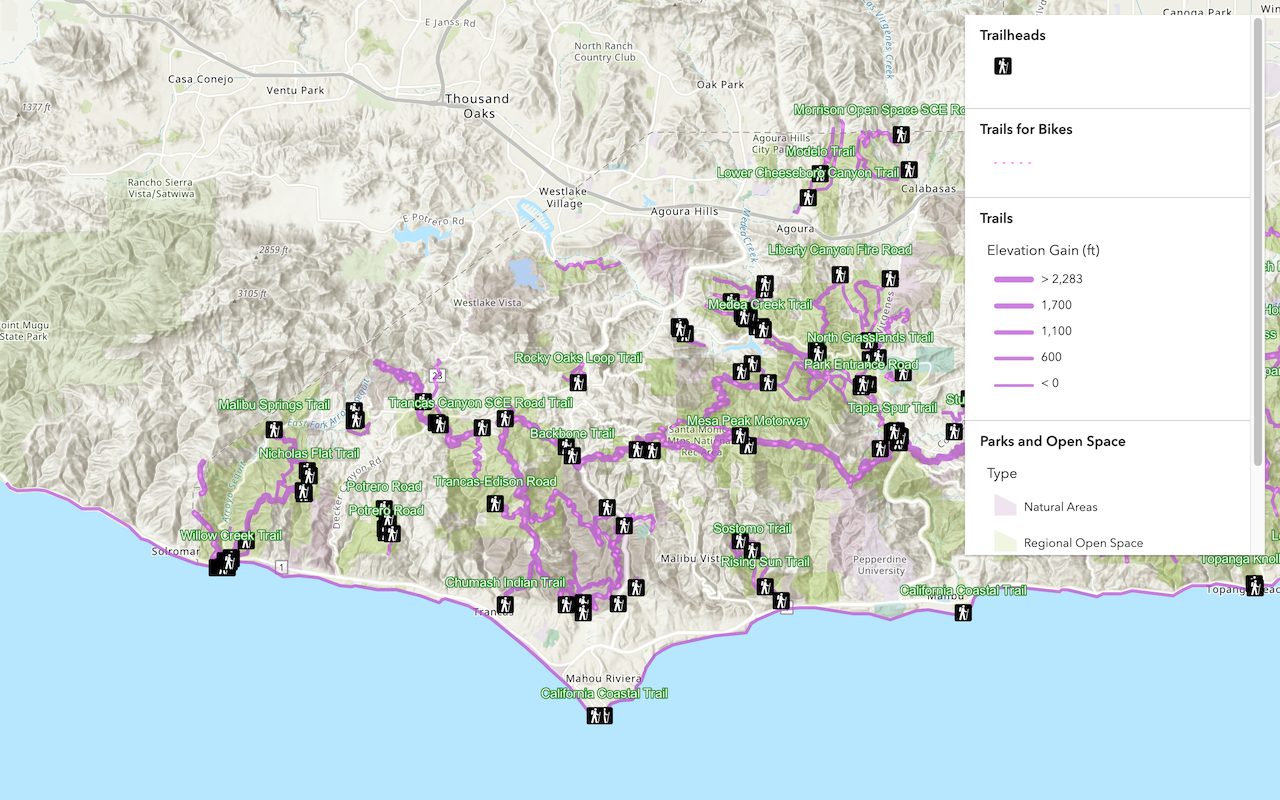
Display a web map
Create and display a map from a web map.
Web scenes
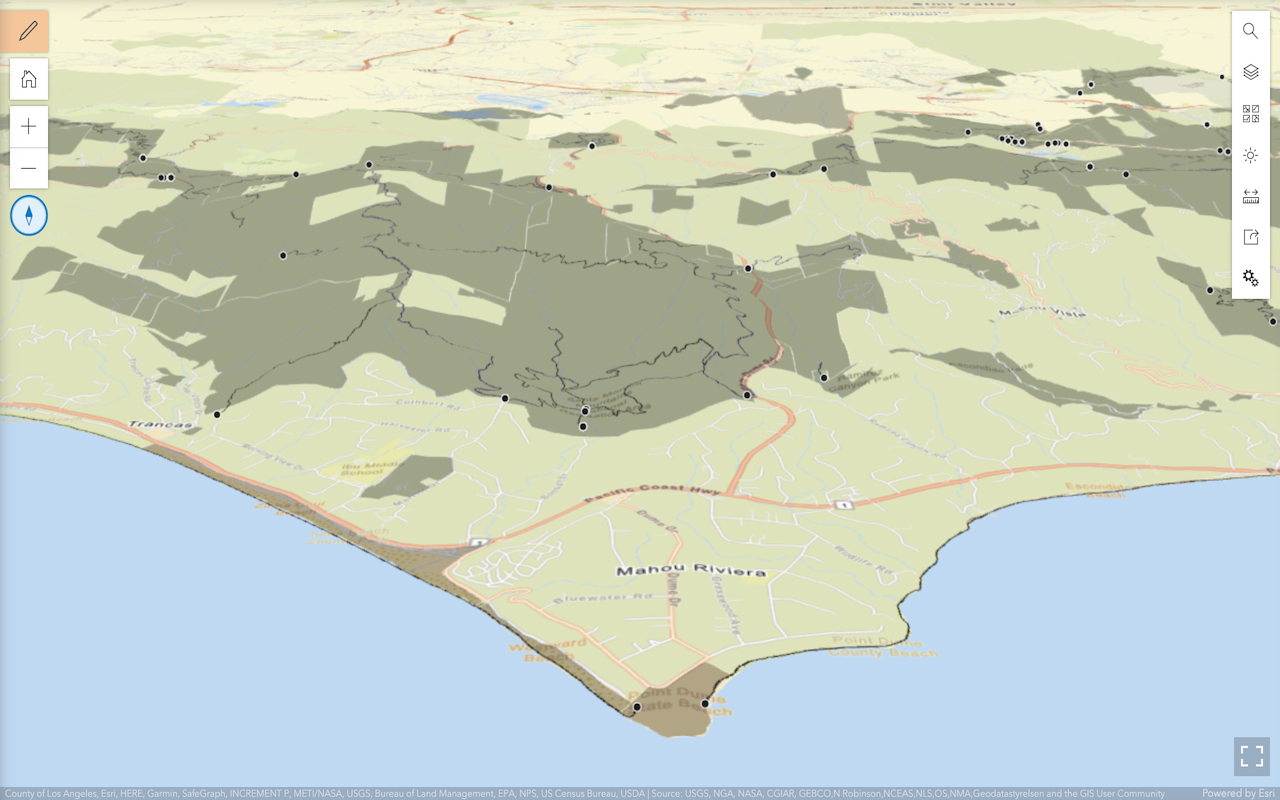
Create a web scene
Use Scene Viewer to create a web scene for your application.
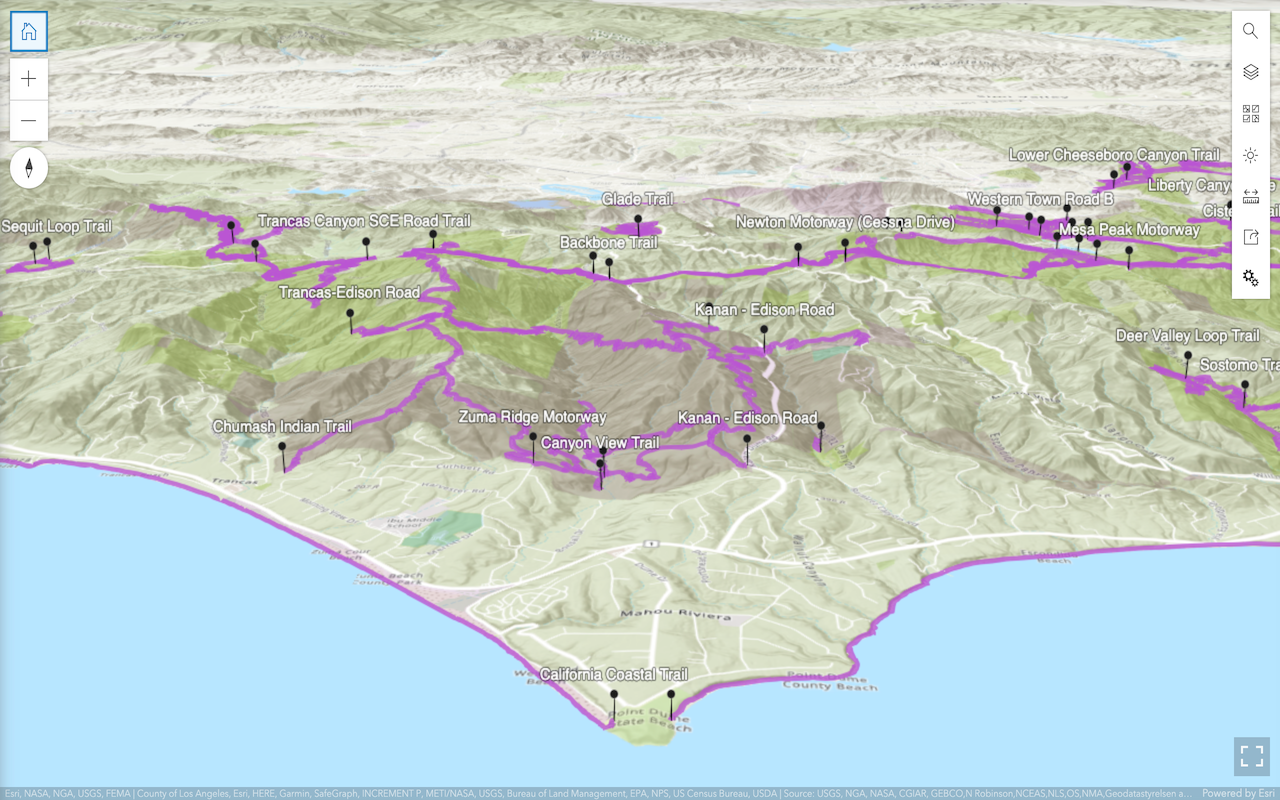
Style layers in a web scene
Use Scene Viewer to style layers in a web scene.
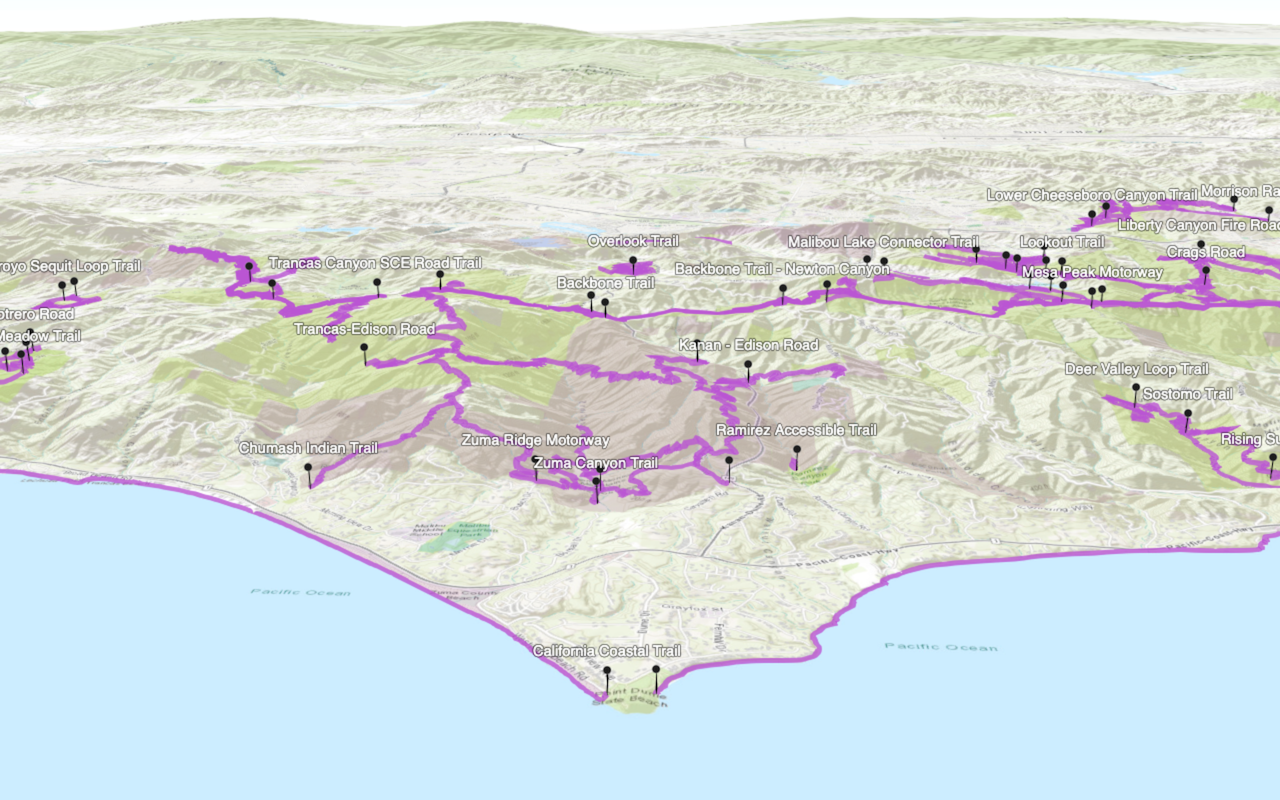
Display a web scene
Create and display a scene from a web scene.
Feature layers
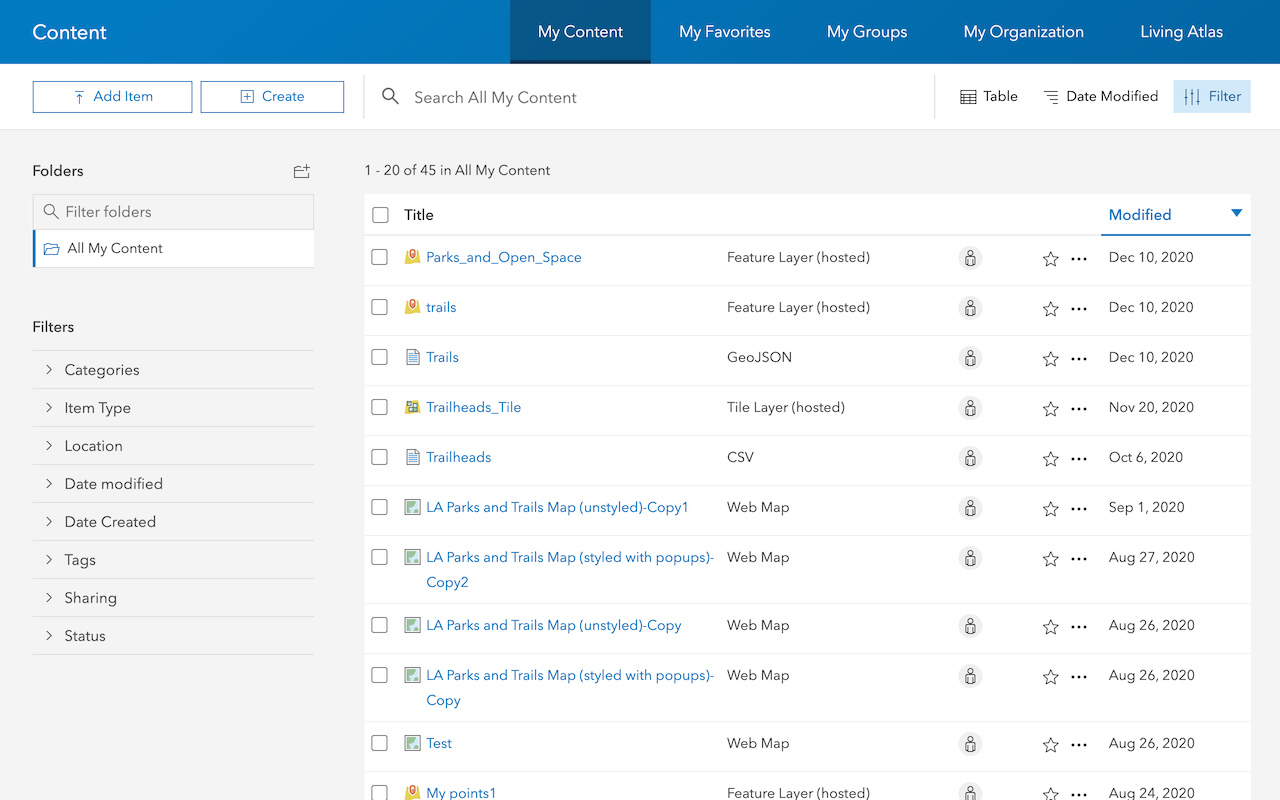
Import data to create a feature layer
Use data management tools to import files and create a feature layer in a feature service.
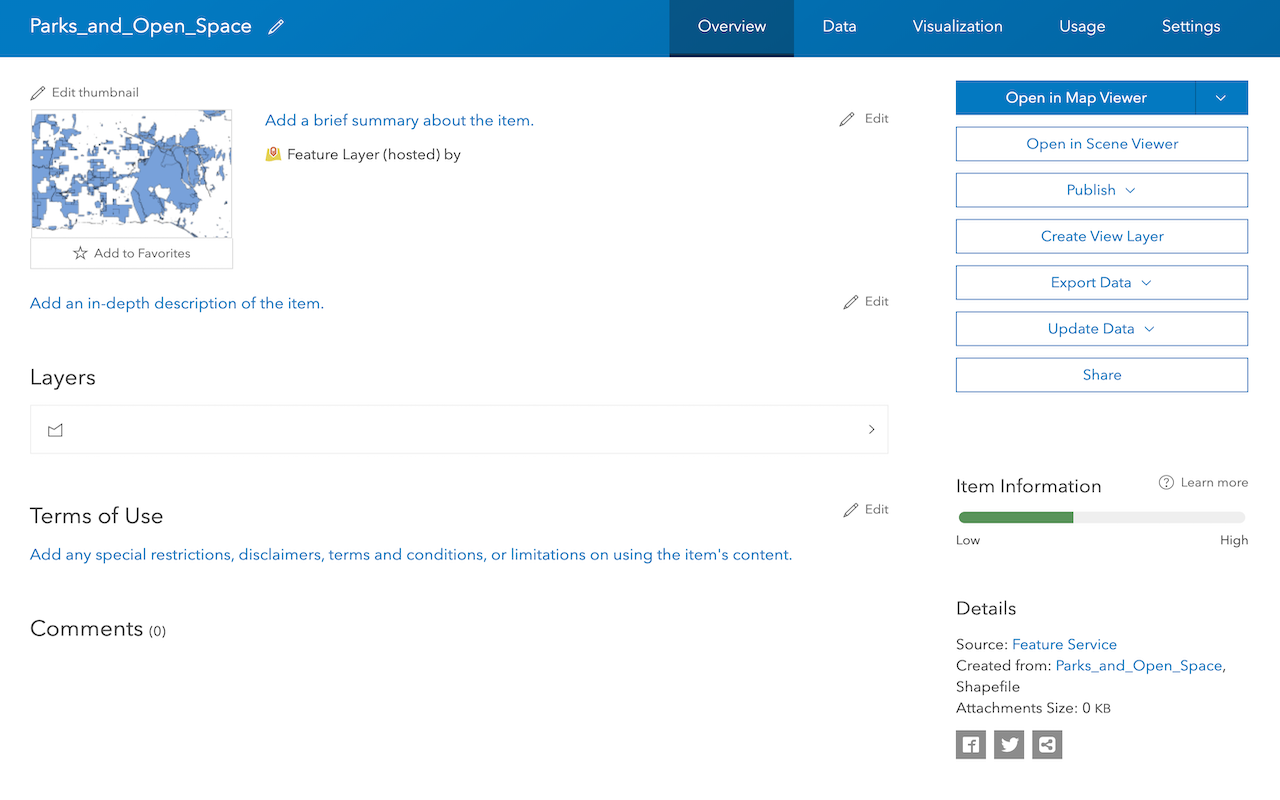
Manage a feature layer
Use a hosted feature layer item to set the properties and settings of a feature layer in a feature service.
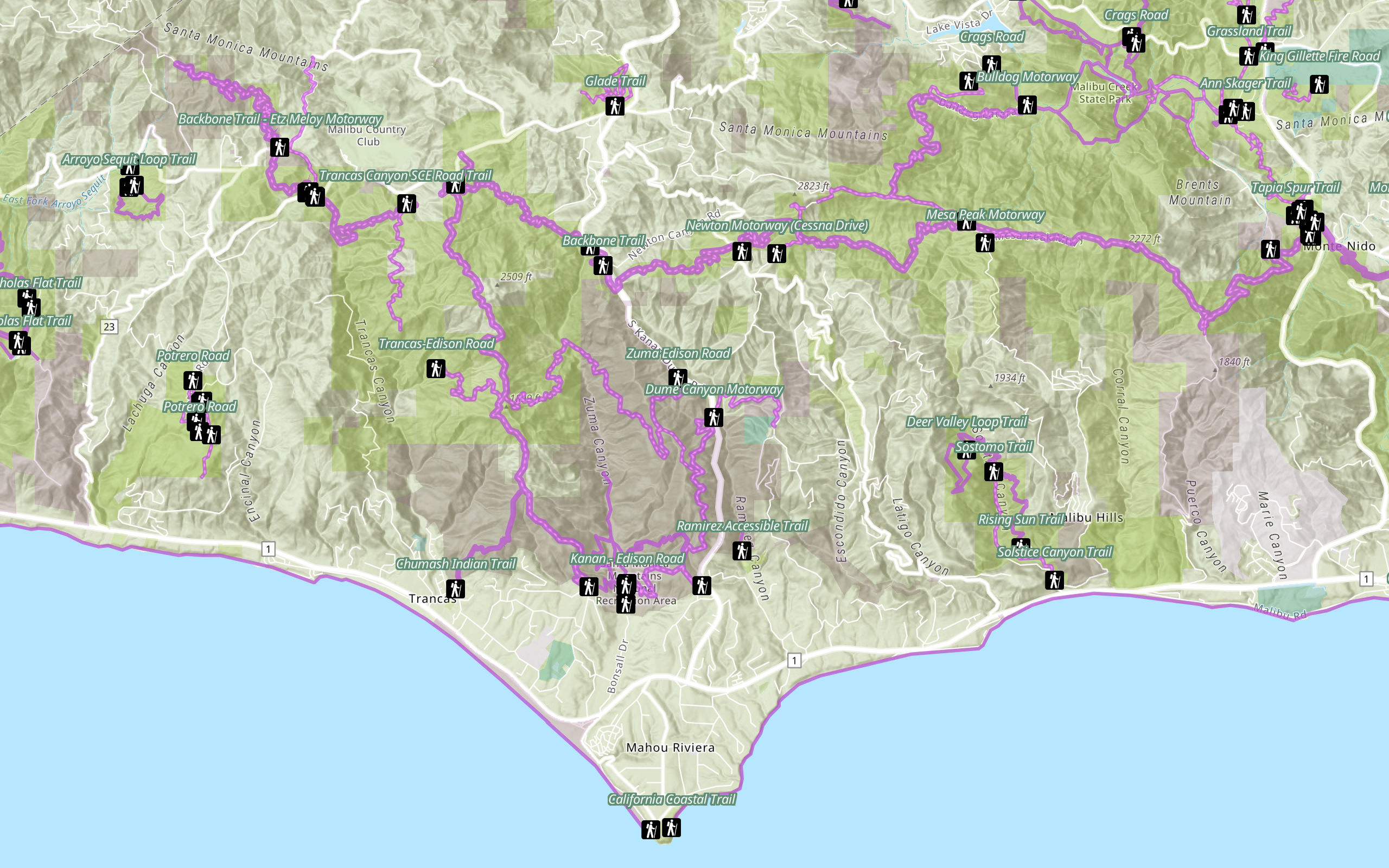
Add a feature layer
Access and display point, line, and polygon features from a feature service.
Vector tile layers
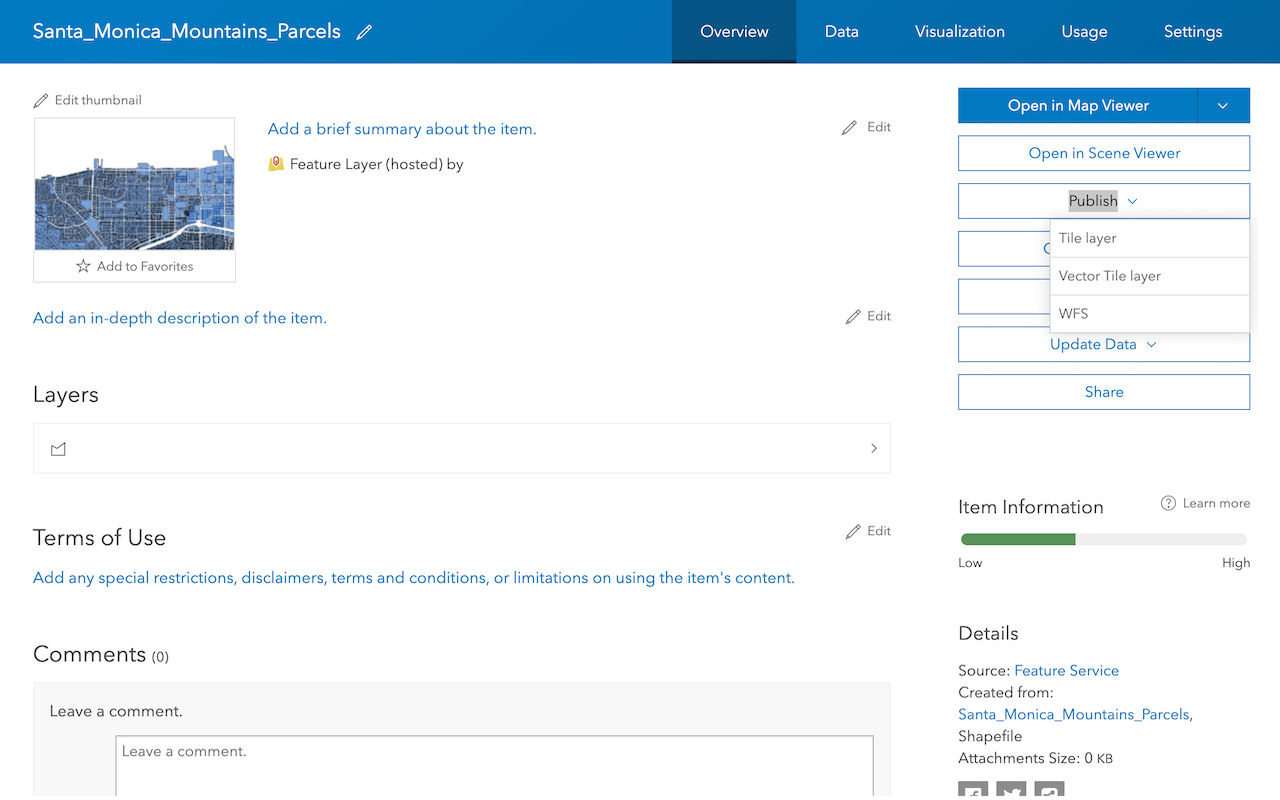
Create a vector tile service
Use data management tools to create a new vector tile service from a feature service.
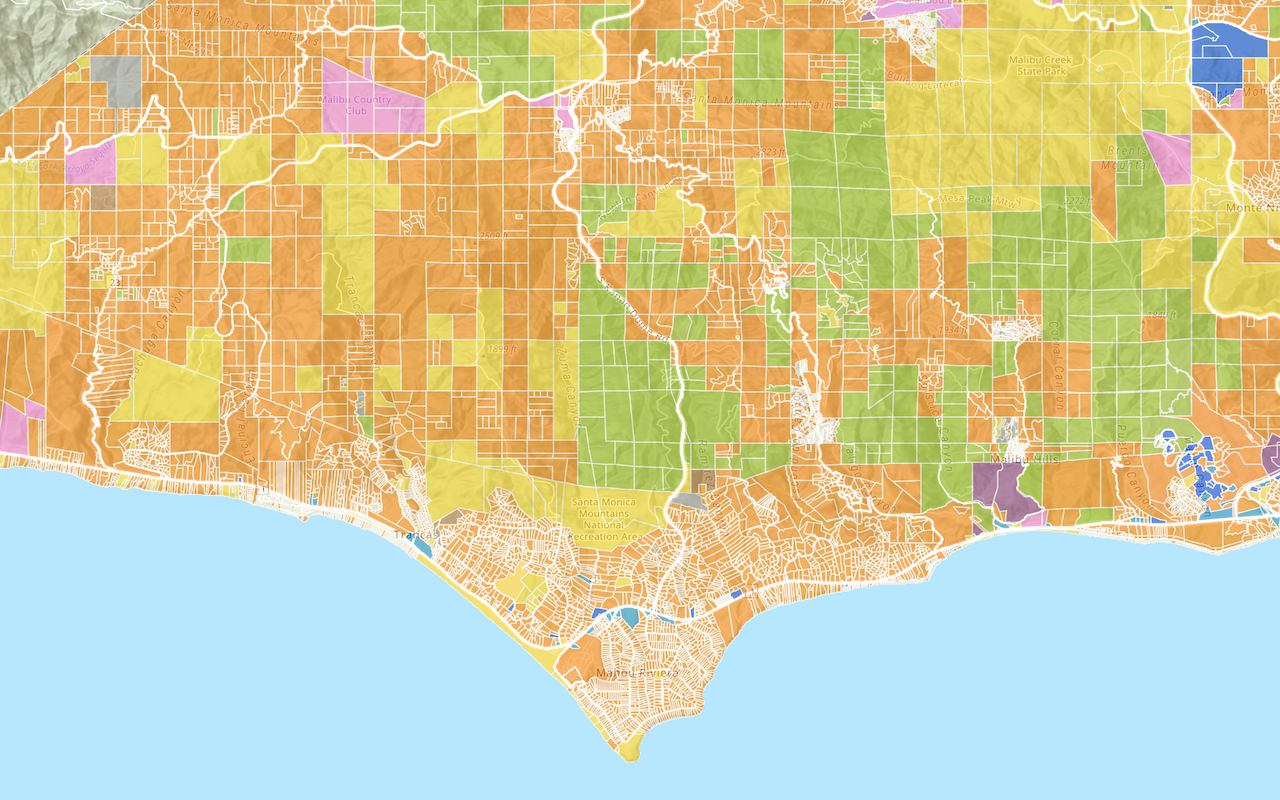
Add a vector tile layer
Access and display a vector tile layer in a map.
Basemap layers
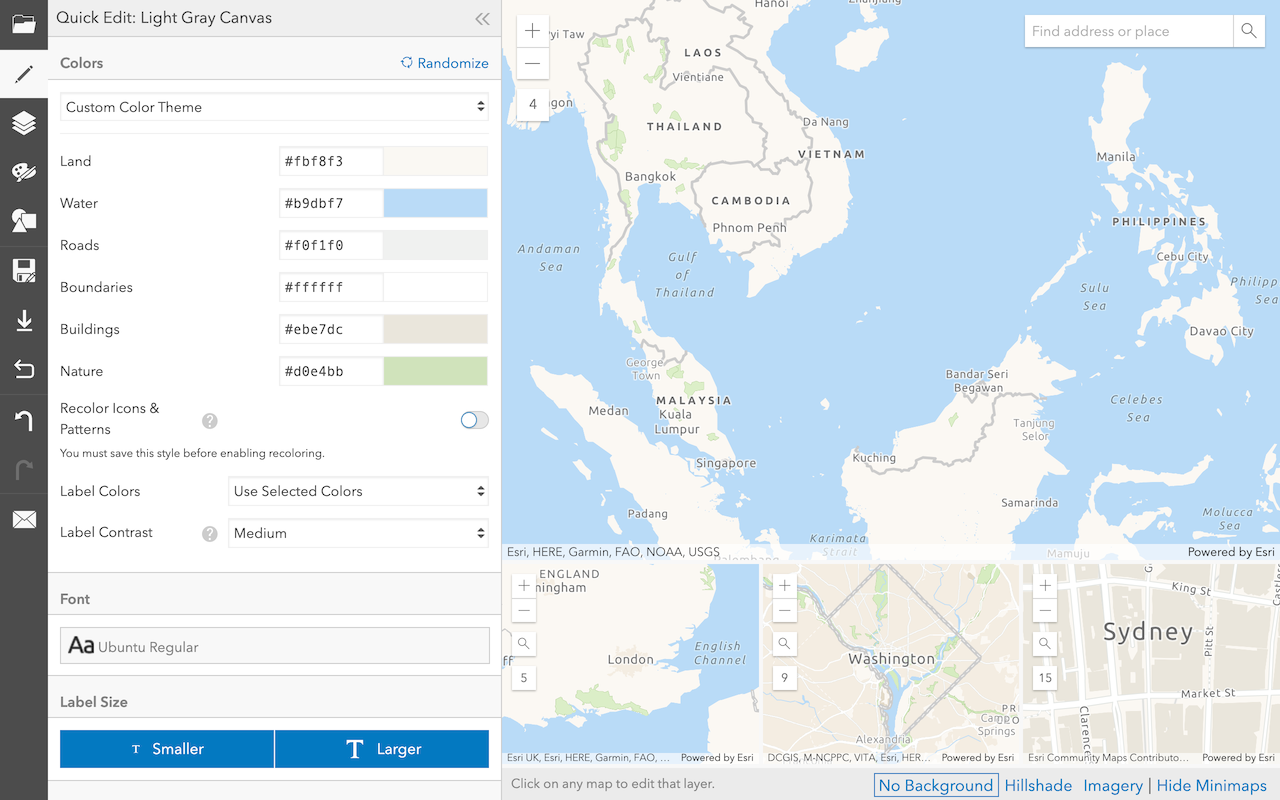
Create a custom basemap style
Use the Vector tile style editor to style a vector tile basemap layer.
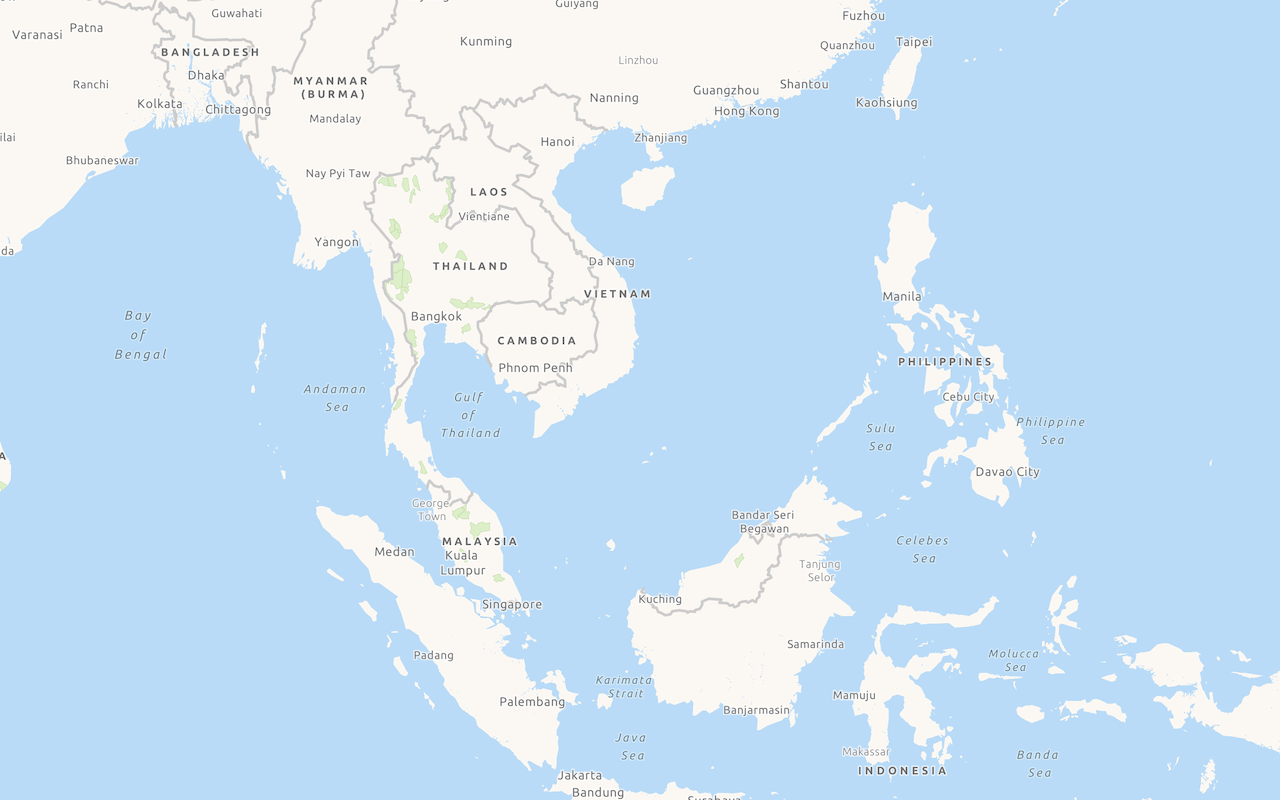
Display a custom basemap style
Add and display a styled vector tile basemap layer.
Services
Portal service
Store, manage, and access private and public content.
API support
- 1. Limited operations, use HTTP requests.
- 2. Access via ArcGIS REST JS.
Tools
Developer dashboard
Manage API keys, service usage, and data with the ArcGIS Developers website.
ArcGIS.com
Create, manage, and share content and data with GIS tools.
ArcGIS Enterprise
Create, manage, analyze, and share data, maps, and applications in your organization.
Map Viewer
Create, explore, and share web maps for 2D applications.
Scene Viewer
Create, explore, and share web scenes for 3D applications.
Vector tile style editor
Style vector tile basemap layers for applications.
ArcGIS Pro
Explore, visualize, and analyze both 2D and 3D data with desktop GIS tools.