Editing features is the process of adding, deleting, or updating features in a feature layer or table. To do so, you can use ArcGIS Maps SDKs, open source libraries, scripting APIs, or the REST APIs. The editing capabilities of a feature service are enabled in the Editing settings of the hosted feature layer (item). You use the settings to control the editing functionality you need to support your applications and workflows. Learn more in Editing and synchronization.
Types of editing workflows
The are two types of editing workflows: simple edits and edits with tracking.
Simple editing
You use simple editing when you want to add, delete, or update features.
- Add: Submits new geometry and/or attribute values to the feature service.
- Delete: Removes existing features from the feature service.
- Update: Updates existing geometry and/or attribute value in a feature service.
Edits with tracking
You use edits with tracking when you want to track the changes, users, and offline usage of features.
-
Tracking of changes to the data: Allows custom applications to identify which features have been added, updated, or deleted.
-
Tracking of the users making edits
Allows you to track:
- Which user created a feature.
- The date and time a feature was created.
- Which user last edited a feature.
- The date and time the feature was last edited.
-
Offline sync: Allow other users to take the hosted feature layer offline and work with it while disconnected from the network.
How to edit features
The general steps to edit features in a feature layer are outlined below.
- Find the URL for the feature layer and feature service you want to edit.
- Configure the service to enable editing.
- Determine the feature layer edit operation (
add
,Feature update
,Features delete
, orFeatures apply
) that is appropriate for your workflow.Edits - Send a POST request containing your edits in a
Feature object
JSON structure to the feature service.
Add features
https://{host}/{orgainizationId}/ArcGIS/rest/services/{serviceName}>/FeatureServer/{id}/addFeatures?{addParameters}
Required parameters
Name | Description | Examples |
---|---|---|
f | The format of the data returned. | f=json f=html |
token | An API key or OAuth 2.0 access token. Learn how to get an access token in Security and authentication. | token=< |
Key parameters
Name | Description | Examples |
---|---|---|
features | The array of features to be added. The structure of each feature in the array is the same as the structure of the json feature object returned by the ArcGIS REST API. Features to be added to a feature layer should include the geometry. Records to be added to a table should not include the geometry. | features=[<feature1>, <feature2>] |
Delete features
https://{host}/{orgainizationId}/ArcGIS/rest/services/{serviceName}>/FeatureServer/{id}/deleteFeatures?{deleteParameters}
Required parameters
Name | Description | Examples |
---|---|---|
f | The format of the data returned. | f=json f=html |
token | An API key or OAuth 2.0 access token. Learn how to get an access token in Security and authentication. | token=< |
Key parameters
Name | Description | Examples |
---|---|---|
object | The object IDs of the layer or table to be deleted. | object |
Update features
https://{host}/{orgainizationId}/ArcGIS/rest/services/{serviceName}>/FeatureServer/{id}/updateFeatures?{updateParameters}
Required parameters
Name | Description | Examples |
---|---|---|
f | The format of the data returned. | f=json f=html |
token | An API key or OAuth 2.0 access token. Learn how to get an access token in Security and authentication. | token=< |
Key parameters
Name | Description | Examples |
---|---|---|
features | The array of features to be updated. The structure of each feature in the array is the same as the structure of the json feature object returned by the ArcGIS REST API. Features to be updated to a feature layer should include the geometry. Records to be added to a table should not include the geometry.The attributes property of the feature should include the object ID (and the global ID, if available) of the feature along with the other attributes: | features=[<feature1>, <feature2>] |
Code examples
Add a feature to a feature layer
To add features to a feature layer, create a geometry (for example, point, line, or polygon), create attributes for the new feature(s), and then call add
.
Request
POST https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/my_points/FeatureServer/0/AddFeatures HTTP/1.1
f=json
&token={ACCESS_TOKEN}
&features=[
{
"geometry": {
"spatialReference": { "latestWkid": 3857, "wkid": 102100 },
"x": -13225414.91018997,
"y": 4028962.1014019456
},
"attributes": {
"name": "Point Dume natural preserve",
"rating": "3"
"SOURCEID": "111111"
}
}
]
Response
{
"addResults": [
{
"objectId": 4,
"uniqueId": 4,
"globalId": null,
"success": true
}
]
}
Update the attributes of a feature
To update existing features in a feature layer, inlcude the ObjectID(s) and/or GlobalId(s) of the features to be updated, create attributes for the new feature(s), and then call add
.
Request
POST https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/my_points/FeatureServer/0/updateFeatures HTTP/1.1
f=json
&token={ACCESS_TOKEN}
&features=[
{
"attributes": {
"objectid": 6
"name": "Point Dume natural preserve (updated)",
"rating": "3"
"SOURCEID": "222"
}
}
]
Response
{
"updateResults": [
{
"objectId": 6,
"uniqueId": 6,
"globalId": null,
"success": true
}
]
}
Delete features from a feature layer
To display a hosted feature layer, you reference the layer by its URL or ID, and then add it to a map or scene. The API communicates with the feature service to retrieve data for the current visible extent. ArcGIS Maps SDKs optimize data access by utilizing feature service functionality such as spatial indexes and caching. APIs also need to specify which data attributes to return.
POST https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/my_points/FeatureServer/0/deleteFeatures HTTP/1.1
f=json
&token={ACCESS_TOKEN}
&objectids=[6]
Response
{
"deleteResults": [
{
"objectId": 6,
"uniqueId": 6,
"globalId": null,
"success": true
}
]
}
Tutorials
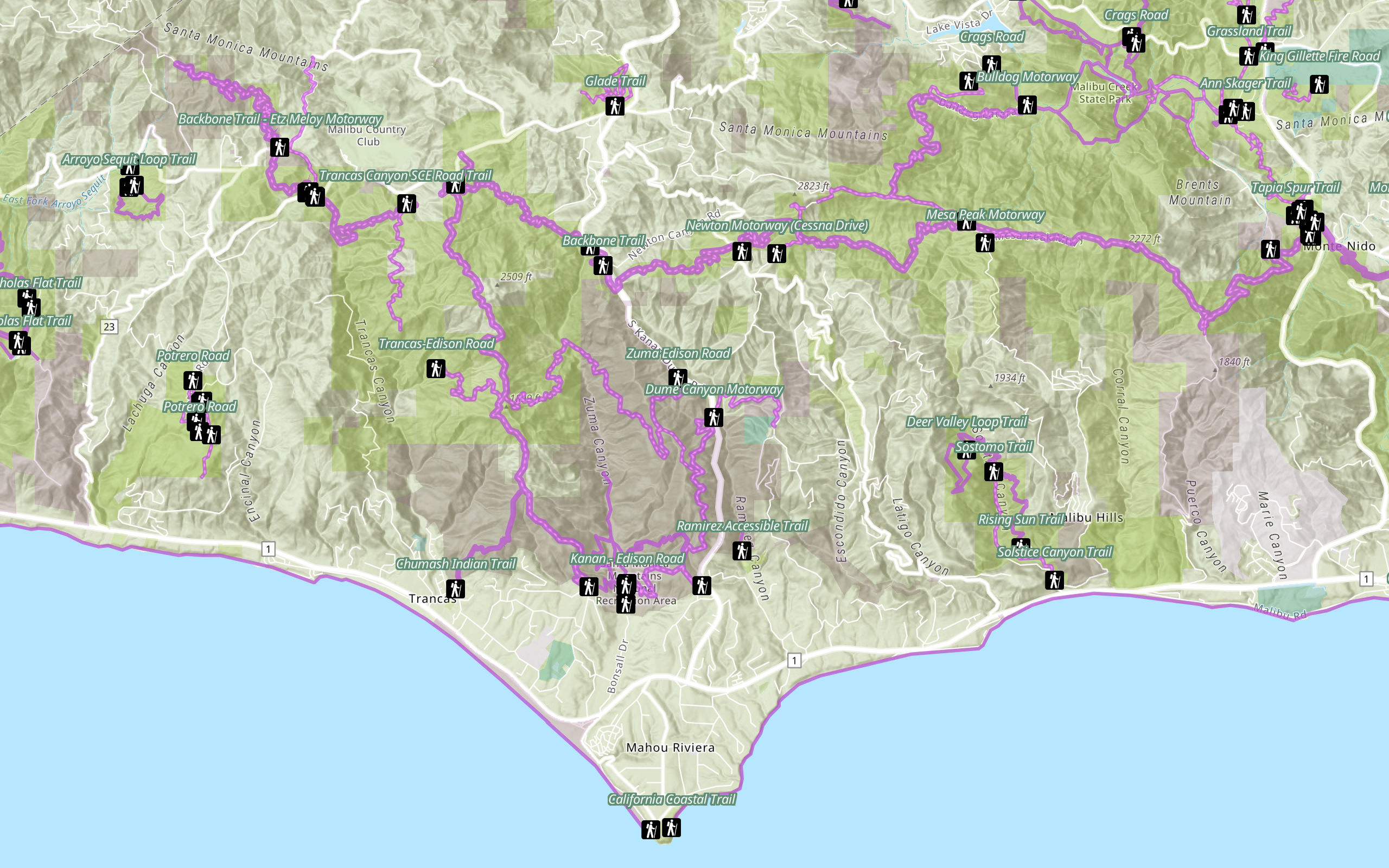
Add a feature layer
Access and display point, line, and polygon features from a feature service.
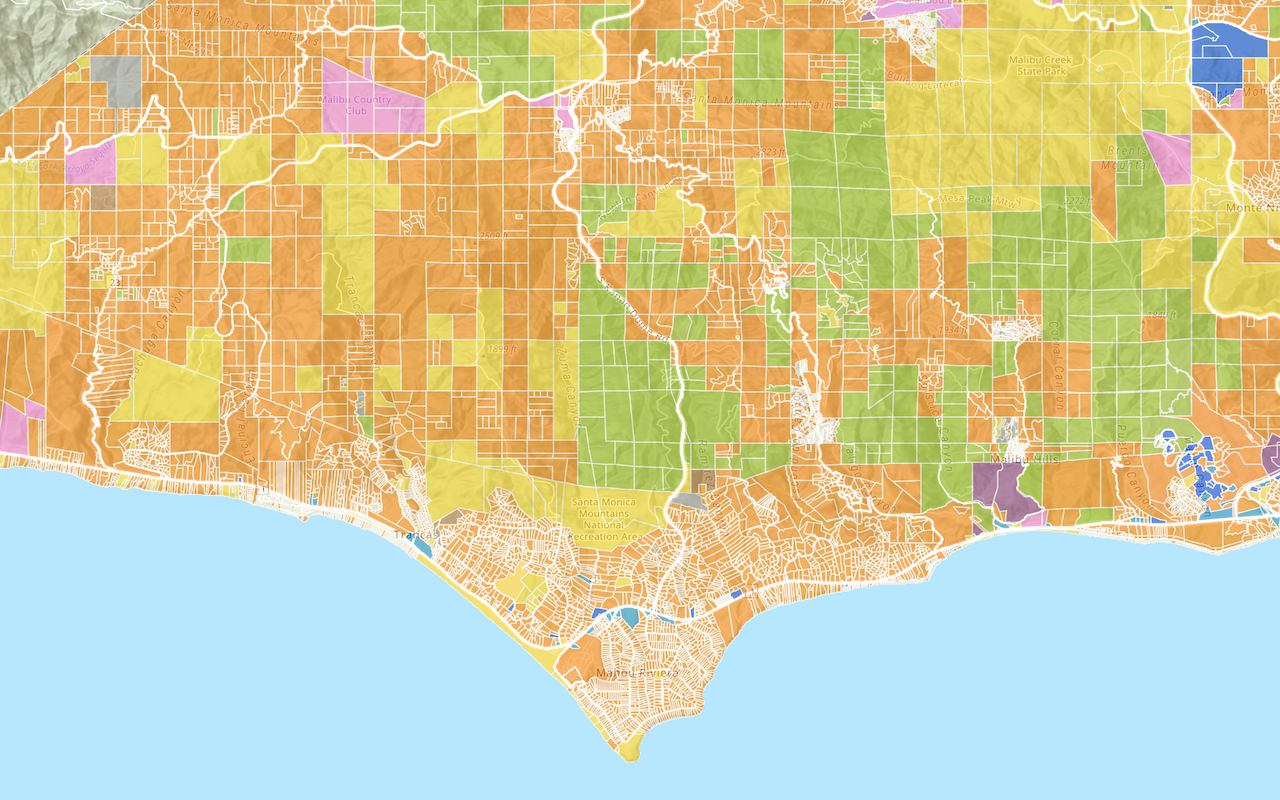
Add a vector tile layer
Access and display a vector tile layer in a map.
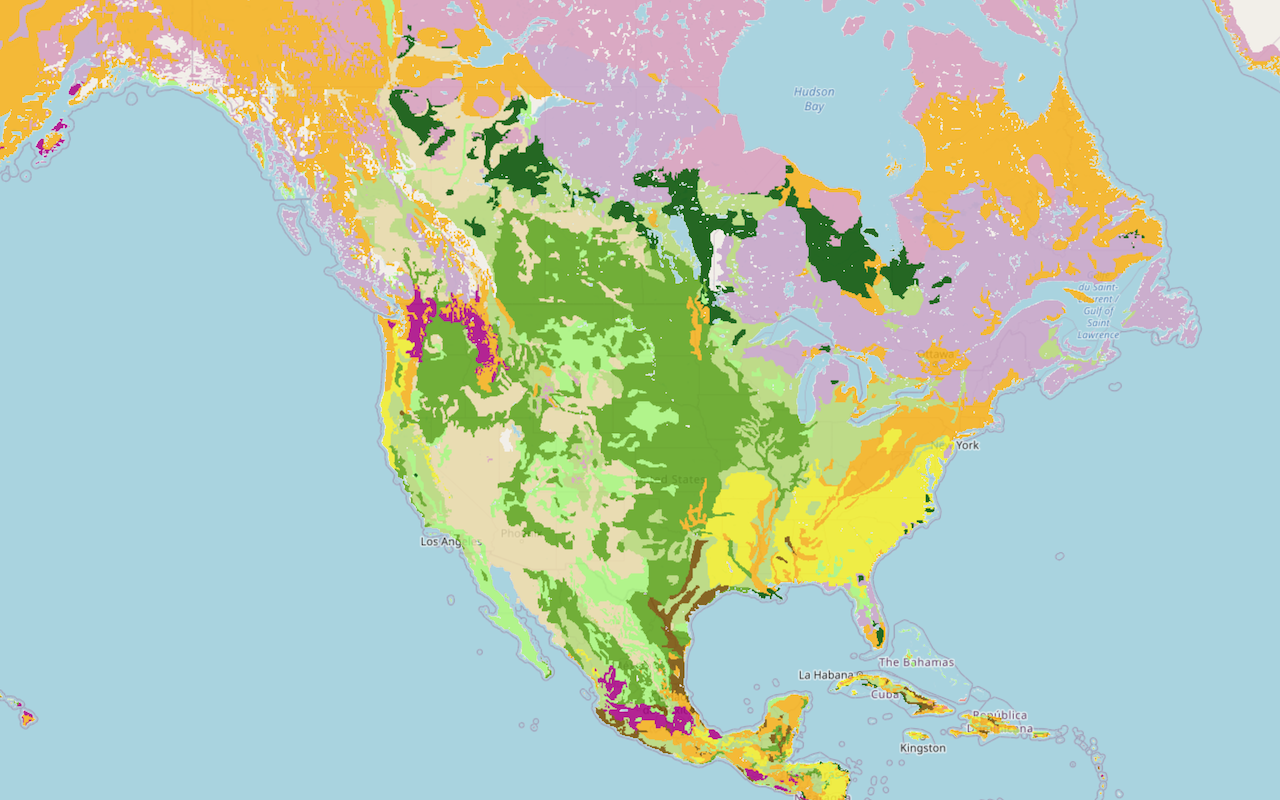
Add a map tile layer
Access and display a map tile layer in a map.
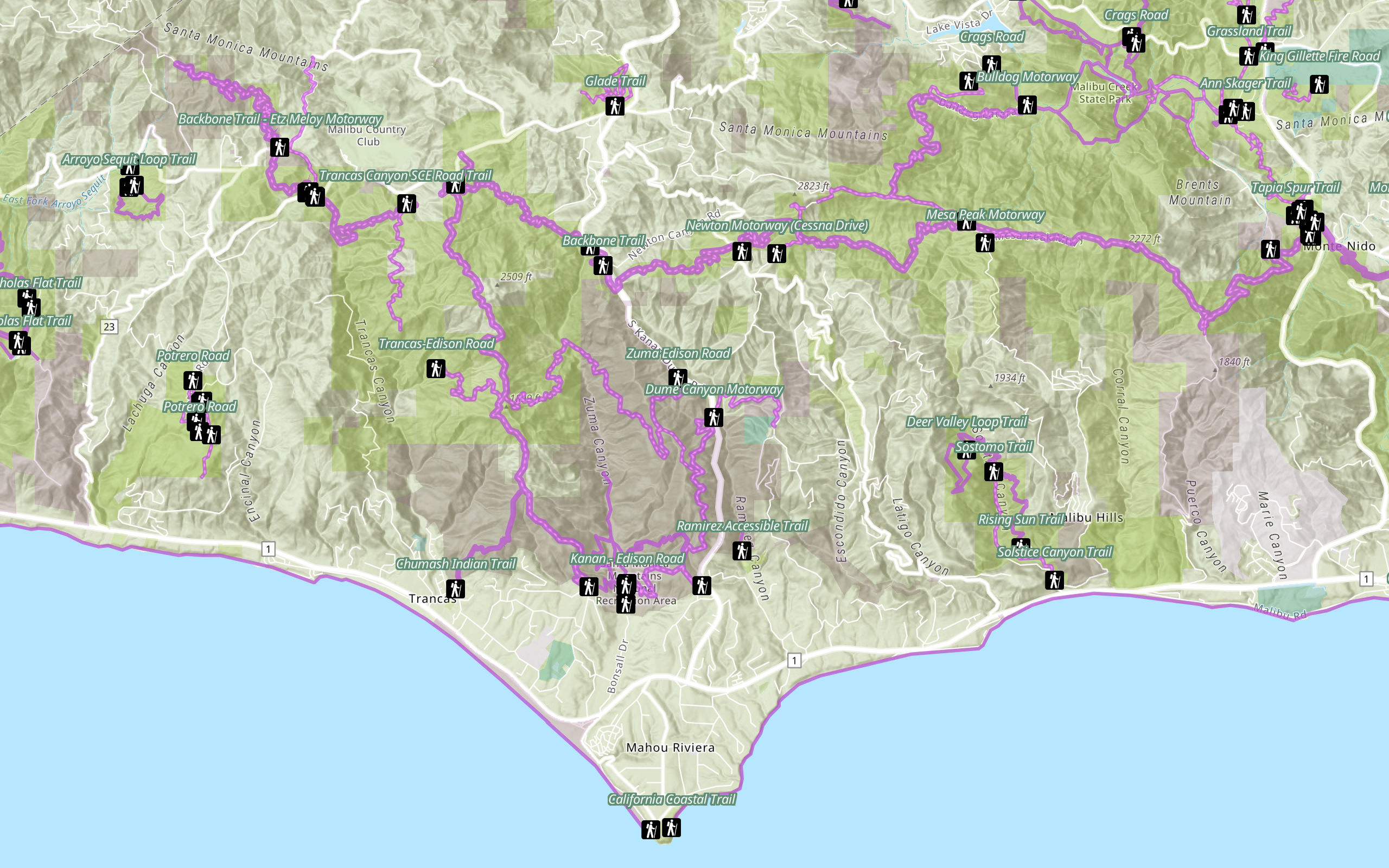
Style a feature layer
Use symbols and renderers to style feature layers.
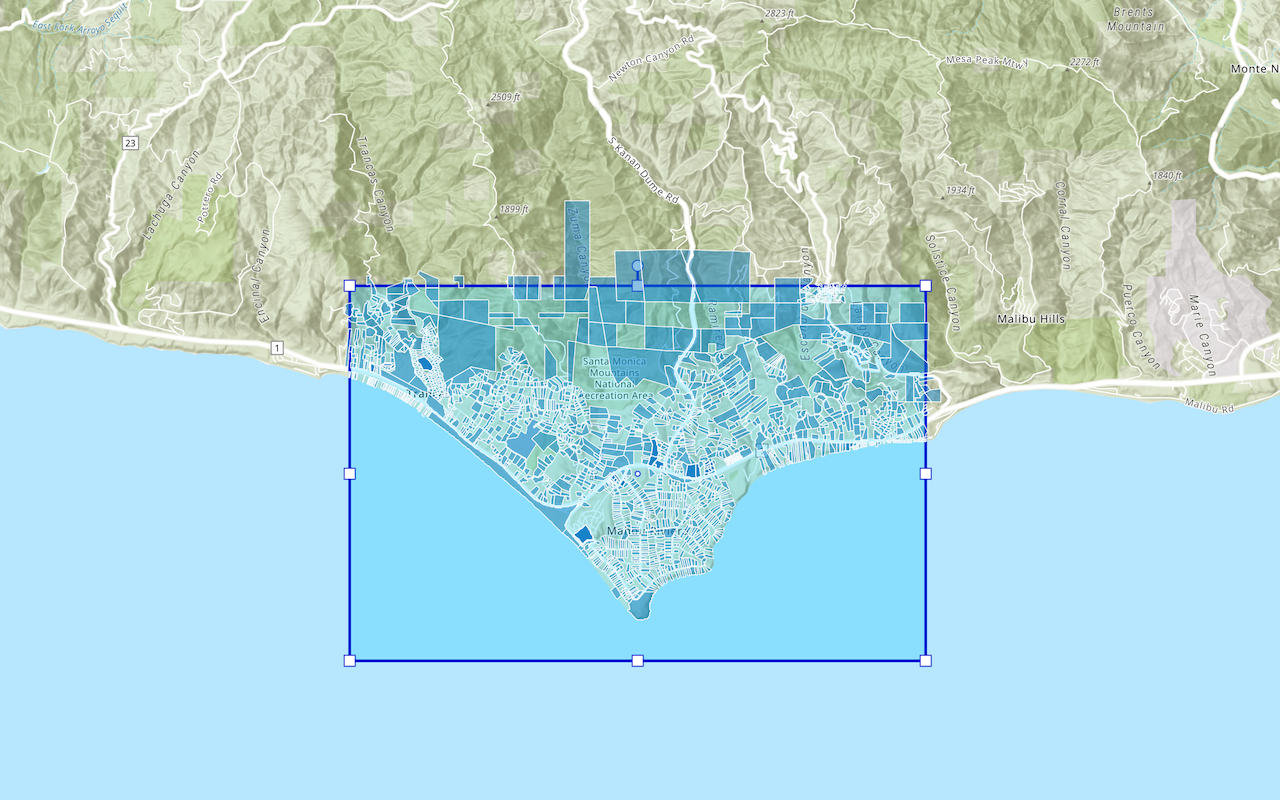
Query a feature layer (spatial)
Execute a spatial query to get features from a feature layer.
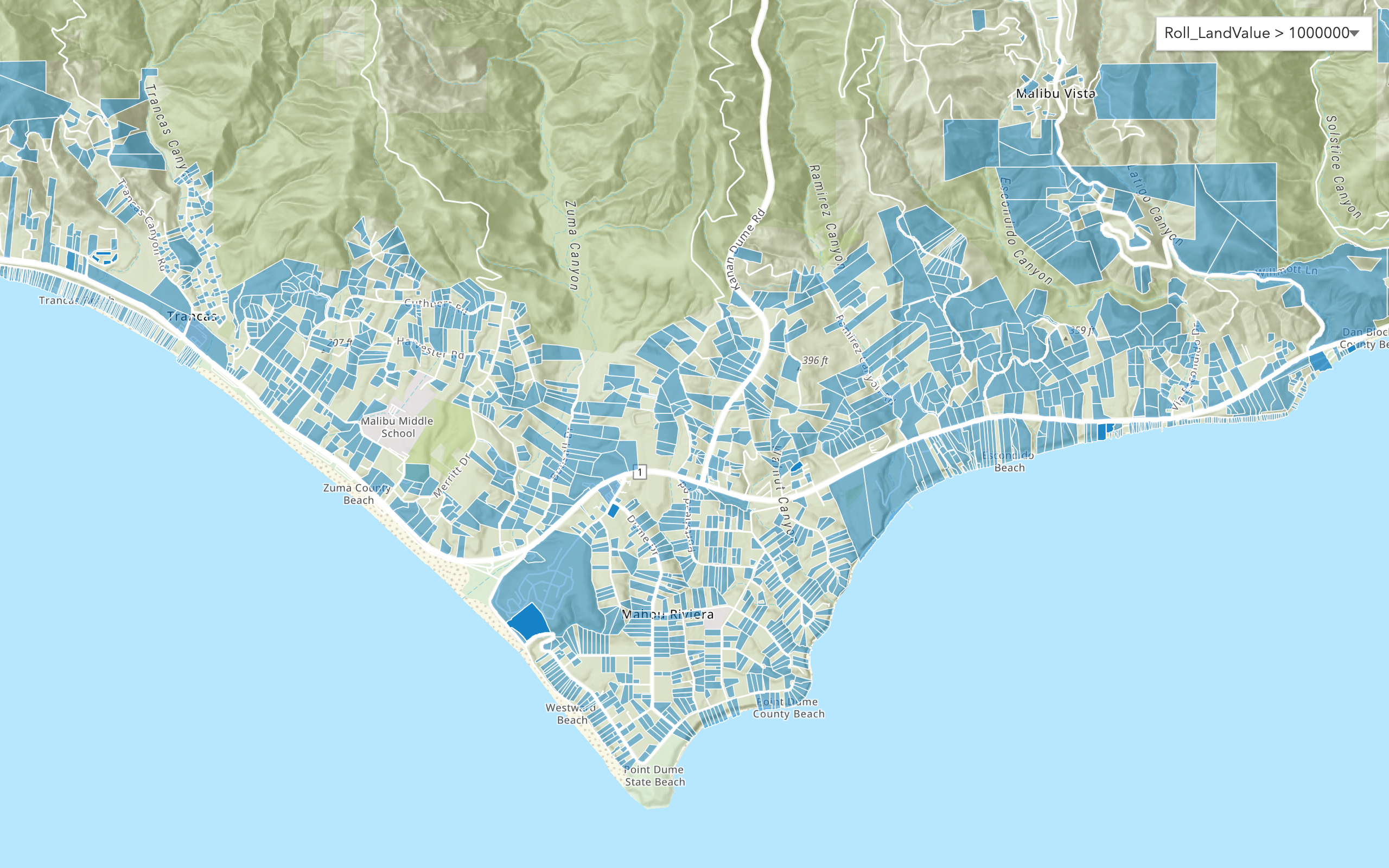
Query a feature layer (SQL)
Execute a SQL query to access polygon features from a feature layer.
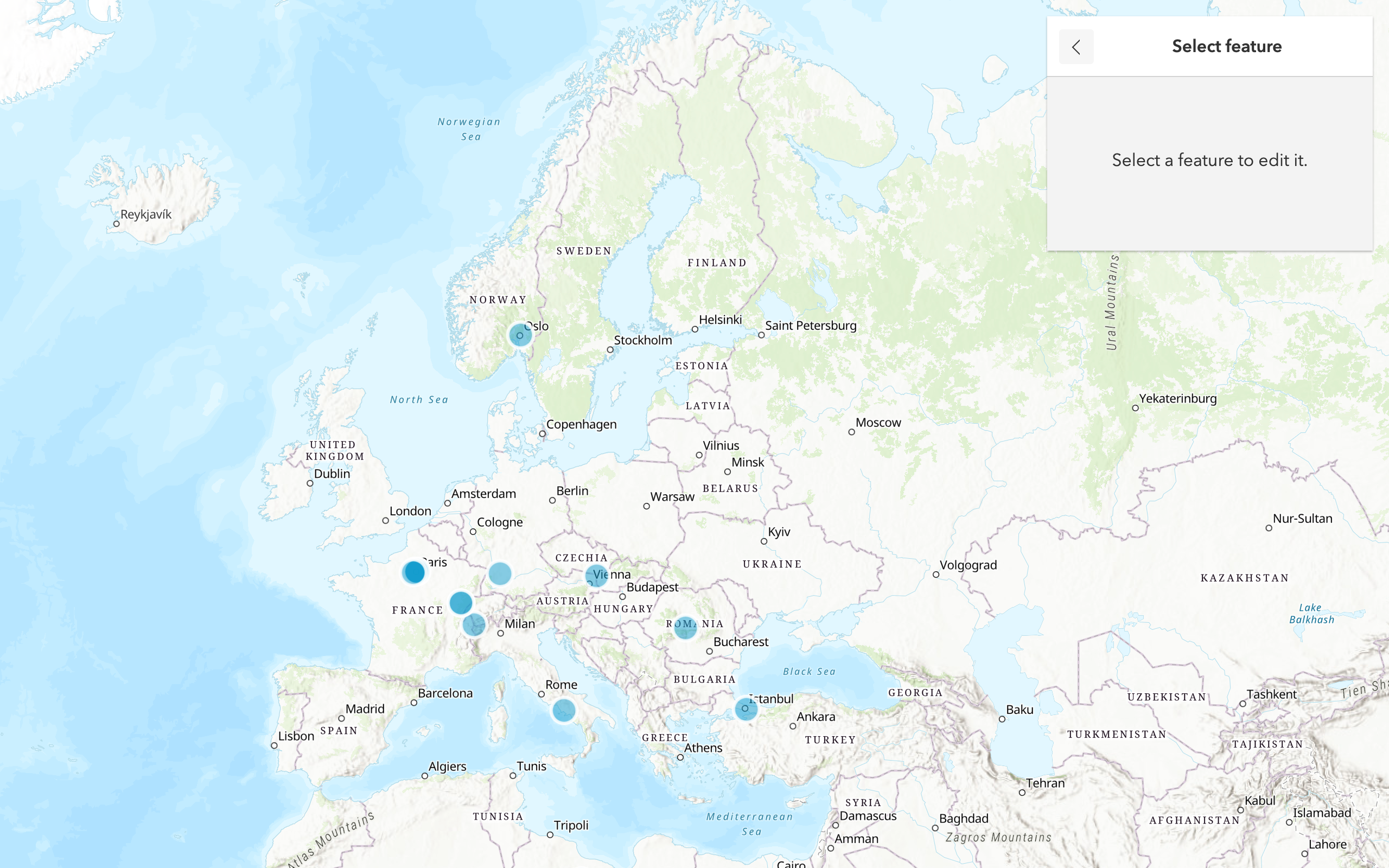
Edit feature data
Add, update, and delete features in a feature service.
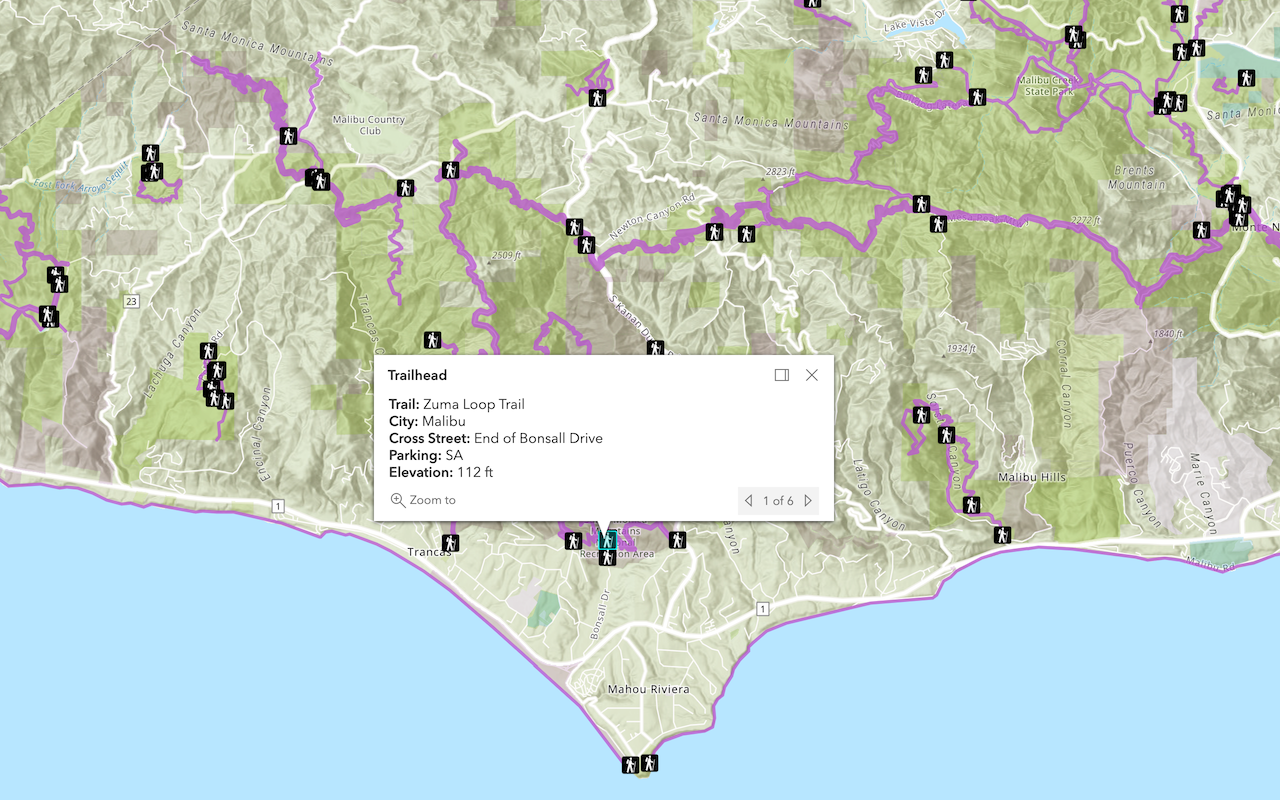
Display a popup
Format a popup to show attributes in a feature layer.
Workflows
Create a feature service for an app
Learn how to import parcel data, create and style a feature layer, and then access the features in an app.

Create a feature layer view for an editor app
Learn how to import parcel data, create and style a feature layer view, and then access the features in an editing app.

Create a vector tile service for an app
Learn how to import parcel data, style a feature layer, and then create a vector tile service for an app.

Create a map tile service for an app
Learn how to import contour data, style a feature layer, and create a map tile service for an app.

Services
Feature service
Add, update, delete, and query feature data.
Vector tile service
Store and access vector tile data.
Map tile service
Store and access map tile data.
API support
Use Client APIs to create, manage, and access data services. The table below outlines the level of support for each API.
- 1. Use portal class and direct REST API requests
- 2. Access via ArcGIS REST JS
- 3. Requires manually setting styles for renderers