Learn how to use data management tools to create a new hosted feature layer.
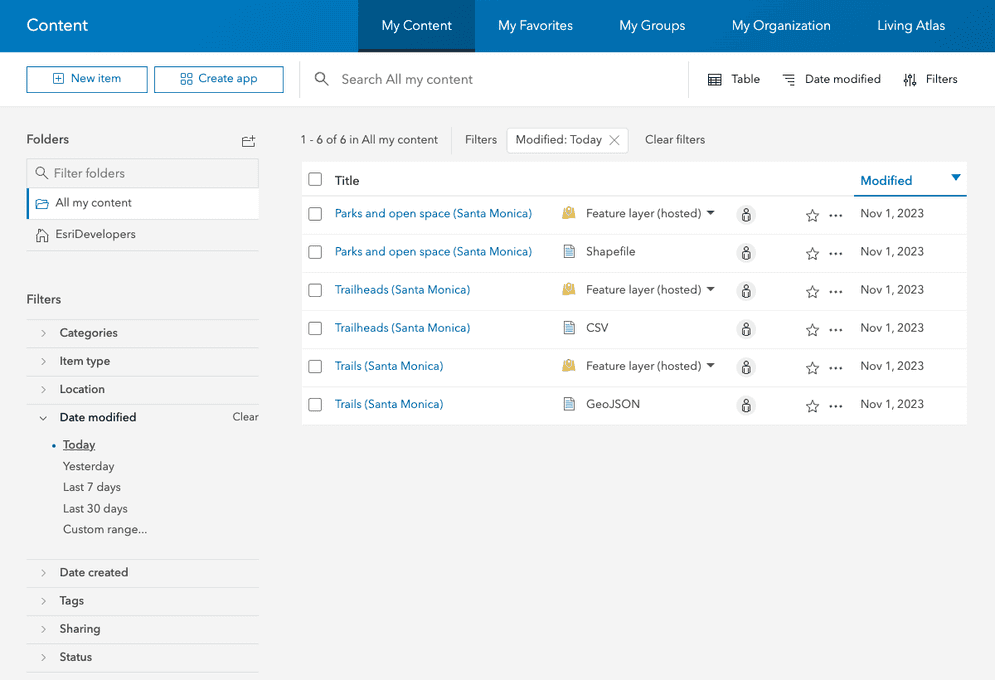
To create a new feature layer in a feature service, you can define a new dataset. To do so, you use data management tools in your portal to define the fields and schema for a new empty feature layer in a feature service. A feature layer is used to store point, line, or polygon geometries with attributes. After a feature layer is created, applications can access it by ID or URL and then query, edit, and display features.
In this tutorial, you use data management tools and scripting APIs to create and define a new feature layer that can store points with attributes. You also use Map Viewer or scripting APIs to add new features and set field values.
Prerequisites
You need an account for ArcGIS Platform, ArcGIS Online, or ArcGIS Enterprise to create hosted data services. If you need an account, go to Get started.
Steps
Create a point feature layer
Use data management tools or scripting APIs to create a point feature layer that is powered by a feature service. The feature service will contain point geometries and attributes.
Follow the steps below to use Data management tools or Scripting APIs:
In your web browser, go to ArcGIS.com and sign in with your ArcGIS Developer account.
-
In the top navigation bar, click Content.
-
Click New item > Feature Layer > Define your own layer.
-
Set the following properties of the feature layer:
- Name:
m
y_ points - Type: Point layer.
- Name:
-
Set the following properties of the ArcGIS Online item:
- Title:
My Points
- Tags:
Beach access
Malibu
- Summary:
Place points along the California coast line.
- Title:
-
Click Next to create the new My Points feature layer and feature service.
-
In the item page of the My Points layer, click the Data tab > Fields > Add.
-
Create the following fields in the Fields window:
- Field 1
- Field Name:
id
- Display Name:
id
- Type:
Integer
- Click: Add New Field
- Field Name:
- Field 2
- Click Add.
- Field Name:
name
- Display Name:
name
- Type:
String
- Length:
256
- Click: Add New Field
- Field 3
- Click Add
- Field Name:
rating
- Display Name:
rating
- Type:
String
- Length:
256
- Click: Add New Field
- Field 1
To access a hosted layer from an application, you need to be able to identify its ID and URL. If a layer is public, you use the URL or item ID to access it directly with your web browser or any application. If the layer is private, you need to provide an access token.
-
Scroll down to find the service URL. For example:
https:
//services3.arcgis.com/GVgb Jbqm8h XASVYi/arcgis/rest/services/my_ points/Feature Server -
Click View to access the metadata for the feature layer. Find the Service ItemId. For example:
70264c2e37784a819e235d3a0129832f
.
In your web browser, go to ArcGIS.com and sign in with your ArcGIS Online account.
-
In the top navigation bar, click Content.
-
Click New item > Feature Layer > Define your own layer.
-
Set the following properties of the feature layer:
- Name:
m
y_ points - Type: Point layer.
- Name:
-
Set the following properties of the ArcGIS Online item:
- Title:
My Points
- Tags:
Beach access
Malibu
- Summary:
Place points along the California coast line.
- Title:
-
Click Next to create the new My Points feature layer and feature service.
-
In the item page of the My Points layer, click the Data tab > Fields > Add.
-
Create the following fields in the Fields window:
- Field 1
- Field Name:
id
- Display Name:
id
- Type:
Integer
- Click: Add New Field
- Field Name:
- Field 2
- Click Add.
- Field Name:
name
- Display Name:
name
- Type:
String
- Length:
256
- Click: Add New Field
- Field 3
- Click Add
- Field Name:
rating
- Display Name:
rating
- Type:
String
- Length:
256
- Click: Add New Field
- Field 1
To access a hosted layer from an application, you need to be able to identify its ID and URL. If a layer is public, you use the URL or item ID to access it directly with your web browser or any application. If the layer is private, you need to provide an access token.
-
Scroll down to find the service URL. For example:
https:
//services3.arcgis.com/GVgb Jbqm8h XASVYi/arcgis/rest/services/my_ points/Feature Server -
Click View to access the metadata for the feature layer. Find the Service ItemId. For example:
70264c2e37784a819e235d3a0129832f
.
In your web browser, go to your ArcGIS Enterprise portal website and sign in with your ArcGIS Enterprise account.
-
In the top navigation bar, click Content.
-
Click New item > Feature Layer > Define your own layer.
-
Set the following properties of the feature layer:
- Name:
m
y_ points - Type: Point layer.
- Name:
-
Set the following properties of the ArcGIS Online item:
- Title:
My Points
- Tags:
Beach access
Malibu
- Summary:
Place points along the California coast line.
- Title:
-
Click Next to create the new My Points feature layer and feature service.
-
In the item page of the My Points layer, click the Data tab > Fields > Add.
-
Create the following fields in the Fields window:
- Field 1
- Field Name:
id
- Display Name:
id
- Type:
Integer
- Click: Add New Field
- Field Name:
- Field 2
- Click Add.
- Field Name:
name
- Display Name:
name
- Type:
String
- Length:
256
- Click: Add New Field
- Field 3
- Click Add
- Field Name:
rating
- Display Name:
rating
- Type:
String
- Length:
256
- Click: Add New Field
- Field 1
To access a hosted layer from an application, you need to be able to identify its ID and URL. If a layer is public, you use the URL or item ID to access it directly with your web browser or any application. If the layer is private, you need to provide an access token.
-
Scroll down to find the service URL. For example:
https:
//your.server.com/ArcGIS/rest/services/my_ points/Feature Server -
Click View to access the metadata for the feature layer. Find the Service ItemId. For example:
70264c2e37784a819e235d3a0129832f
.
Create a stand-alone feature class
-
Launch ArcGIS Pro and create a new map project.
-
In the Catalog pane, right-click the geodatabase in which you want to create a feature class.
-
Click New > Feature Class to launch the Create Feature Class tool.
-
Set the following information in the Define page of the tool:
- Name:
m
y_ points - Alias:
My Points
- Feature Class Type:
Point
- Check the Add output dataset to the current map check box to add the feature class to the active map.
- Name:
-
Click Next.
Define the fields
You will define three fields in the Fields page: id
, name
, and rating
.
-
Click Click here to add a new field.
-
Set the following information:
- Field Name:
id
- Data Type:
integer
- Set the following in the Field Properties section at the bottom of the pane:
- Alias:
ID
- Alias:
- Field Name:
-
Set the following information:
- Field Name:
name
- Data Type:
string
- Set the following in the Field Properties section at the bottom of the pane:
- Alias:
Name
- Alias:
- Field Name:
-
Click Click here to add a new field.
-
Set the following information:
- Field Name:
rating
- Data Type:
string
- Set the following in the Field Properties section at the bottom of the pane:
- Alias:
Rating
- Alias:
- Field Name:
-
Click Next to complete defining fields.
-
On the Spatial Reference page, click Next to accept the default spatial reference.
-
On the Tolerance page, click Next to accept the default value.
-
On the Resolution page, click Next to accept the default settings.
-
On the Storage Configuration page, click Next to accept the default settings.
-
Click Finish to create the point feature class.
Publish as web layer
Before you can share the feature layer as a hosted feature layer, you will need to enable Allow assignment of unique numeric IDs for sharing web layers option. By default, this option is not enabled. Enabling this option will allow you to assign static IDs to feature layers in your project.
- In the Contents pane, right-click the Map > Properties
- In the General section, enable Allow assignment of unique numeric IDs for sharing web layers.
With that option enabled, you can now publish the My Points point feature layer as a hosted feature layer.
- Right-click on feature layer and click Sharing > Share as Web Layer.
- The Share As Web Layer pane will be displayed.
- Set the following required information in the General tab:
- Name:
My Points
- Tags:
Beach access
,Malibu
- Summary:
Place points along the California coast line
- Layer Type:
Feature
- Set a location in your organization in Location
- Name:
- Click Analyze
- Any errors or warnings during the analyze process will be displayed in the Messages tab.
- Click Publish
A success message will be displayed with Manage the web layer link allowing you to visit the item page details of the hosted feature layer.
- Import the required libraries.
- Provide an access token.
- Reference the feature service.
- Execute the
update
operation.Definition - Handle the results.
# create the service
new_service = portal.content.create_service(
name="My Points",
create_params=create_params,
tags="Beach Access,Malibu",
)
# Add layer definition and schema
new_feature_layer = FeatureLayerCollection.fromitem(new_service)
new_feature_layer.manager.add_to_definition(layer_schema)
print(
f"New hosted featurelayer created: \n\tid: {new_feature_layer.id}\n\t"
"url: {new_feature_layer.url}"
)
Enable editing
To add, update, and delete features in your feature layer, you need to enable editing.
Follow the steps below to use Data management tools or Scripting APIs:
In ArcGIS.com, click Content > My points.
-
In the item page, click Share to ensure that sharing permissions are set to Owner > Save.
-
Click the Settings tab.
-
Under Feature layer (hosted), click Enable editing.
-
Click Save.
In ArcGIS.com, click Content > My points.
-
In the item page, click Share to ensure that sharing permissions are set to Owner > Save.
-
Click the Settings tab.
-
Under Feature layer (hosted), click Enable editing.
-
Click Save.
In your ArcGIS Enterprise portal, click Content > My points.
-
In the item page, click Share to ensure that sharing permissions are set to Owner > Save.
-
Click the Settings tab.
-
Under Feature layer (hosted), click Enable editing.
-
Click Save.
- Import the required libraries.
- Provide an access token.
- Reference the feature service.
- Execute the
update
operation.Definition - Handle the results.
# get feature service as a FeatureLayerCollection
feature_layer = FeatureLayerCollection.fromitem(feature_service)
# update capabilities to enable editing
results = feature_layer.manager.update_definition(
{"capabilities": "Query, Extract, Editing, Create, Delete, Update"}
)
print(results)
Add feature data
You can use Map Viewer, ArcGIS Pro, or a scripting API to add, update, or delete features.
Map Viewer can be used to add and edit feature layer data. Use it to position the map to the Santa Monica mountains and then add new point features interactively.
-
Open Map Viewer.
-
Sign in to your account.
-
In the left panel, click Layers > Add > My Points > Add to Map.
-
On the right panel, click Map tools > Search. Type in
34.01757,-118.82549
and zoom to Zuma Beach. -
On the bottom of the right panel, click Edit to open the editor.
-
In the Editor, click New Feature and click on the map to create a new point. Set the following attribute values:
- id:
1
- name:
Zuma Beach
- rating:
Good
- Click Create
- id:
-
Add another point to the map at Westward Beach:
- Search for Westward Beach at the following coordinates:
34.00637,-118.812791
- Click Edit > New Feature and create a new point at the coordinates with the following attribute values:
- id:
2
- name:
Westward Beach
- rating:
Excellent
- Click Create
- id:
- Search for Westward Beach at the following coordinates:
-
Add another point to the map at Point Dume County Beach:
- Search for Point Dume County Beach at the following coordinates:
34.00339,-118.80485
- Click Edit > New Feature and create a new point at the coordinates with the following attribute values:
- id:
3
- name:
Point Dume County Beach
- rating:
Poor
- Click Create
- id:
- Search for Point Dume County Beach at the following coordinates:
-
Add any additional points you would like to include on your map using the method described above.
-
In the left panel, click Layers > My Points > ... > Show table to view the attribute data of your hosted feature layer. This opens the table view that you can use to inspect and edit data values.
ArcGIS Pro can be used to add and edit feature layer data. Use it to position the map to the Santa Monica mountains and then add new point features interactively.
-
Launch ArcGIS Pro
-
Create a new map project.
-
In the Catalog pane, click Portal and add the My Points feature service to the map.
- The feature service can be added by clicking and dragging onto the map or
- Right-click on the feature services and click Add To Current Map.
-
In the Map ribbon, click Go To XY tool and type in
34.01757,-118.82549
and zoom to Zuma Beach. -
In the Edit ribbon, click Create to launch the Create Features pane.
-
In the Templates section, click New Feature and click on the map to create a new point. Set the following attribute values:
- id:
1
- name:
Zuma Beach
- rating:
Good
- id:
-
Add another point to the map at Westward Beach:
- Using the Go To XY tool, enter the following coordinates:
34.00637,-118.812791
- Create a new point on the map with the following attribute values:
- id:
2
- name:
Westward Beach
- rating:
Excellent
- id:
- Using the Go To XY tool, enter the following coordinates:
-
Add another point to the map at Point Dume County Beach:
- Using the Go To XY tool, enter the following coordinates:
34.00339,-118.80485
- Create a new point at the coordinates with the following attribute values:
- id:
3
- name:
Point Dume County Beach
- rating:
Poor
- id:
- Using the Go To XY tool, enter the following coordinates:
-
Add any additional points you would like to include on your map using the method described above.
-
In the Contents pane, right-click on My Points and click Attribute Table to view the attribute data. This opens a table view that you can use to inspect and edit data values.
- Import the relevant libraries.
- Provide an access token.
- Reference the feature service.
- Create new features.
- Execute the edit operation.
- Handle results.
// execute method
const results = await addFeatures({
authentication: auth,
url: '<YOUR_FEATURELAYER_URL>',
features: newFeatures,
});
// handle results
results.addResults.forEach(res=>{
console.log(res)
})
You now have a hosted feature layer and feature service. You can access the hosted layer (item) with its URL or layer ID in your applications. To manage your hosted layer (item) properties and capabilities, visit the Manage a feature layer tutorial.
What's next?
Learn how to use additional tools, APIs, and location services in these tutorials:
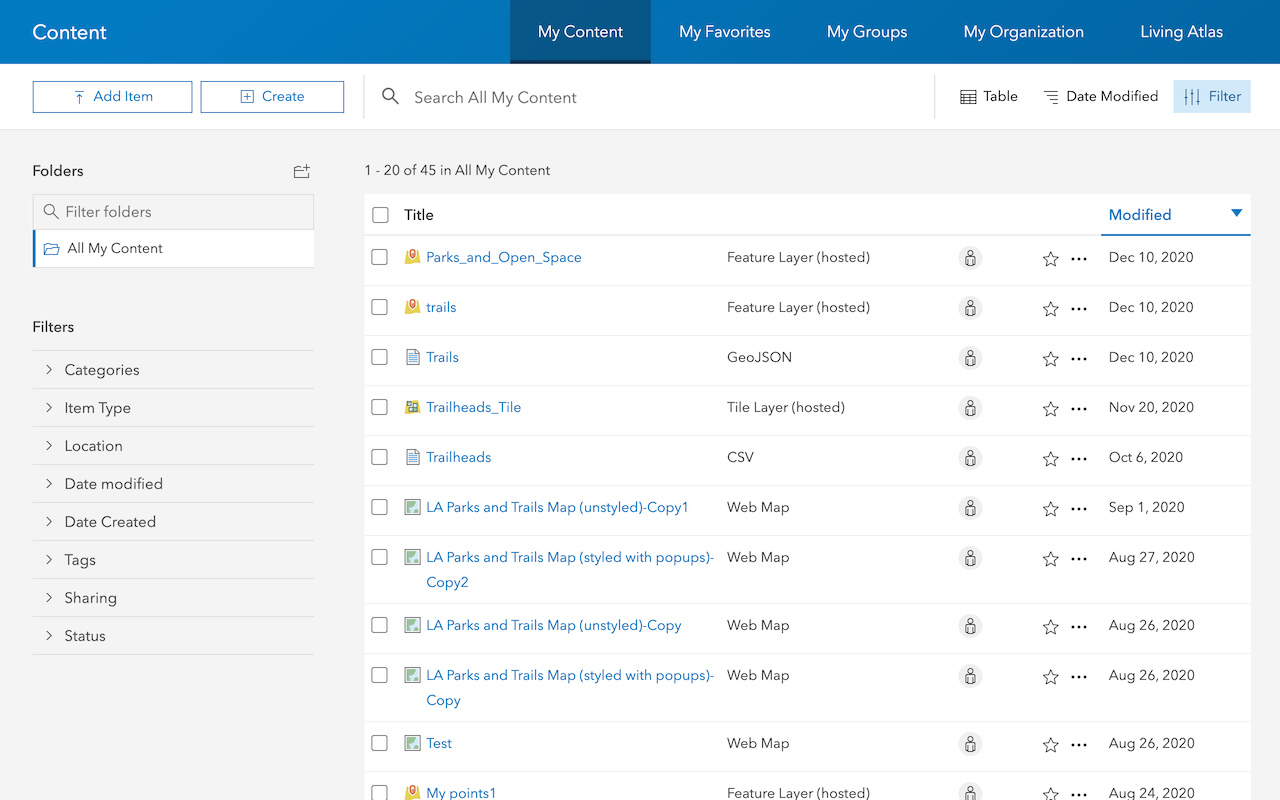
Import data to create a feature layer
Use data management tools to import files and create a feature layer in a feature service.
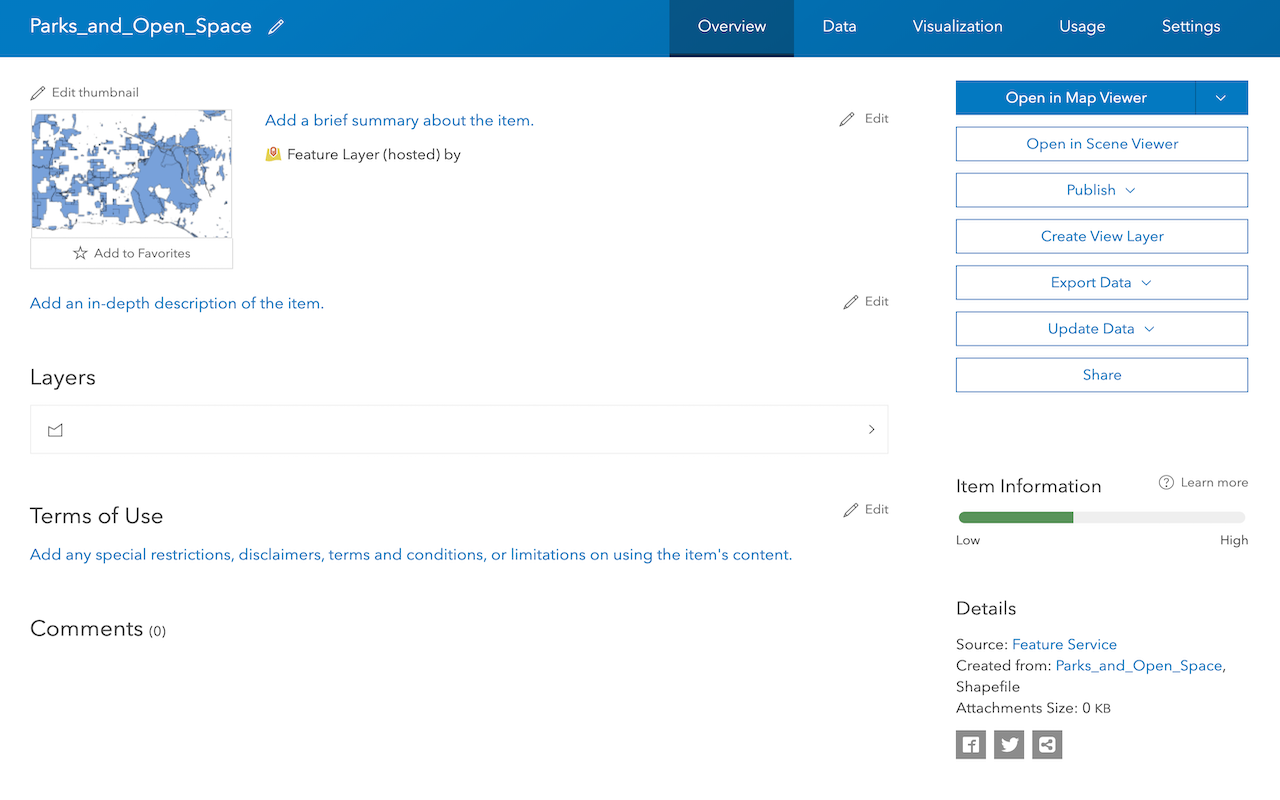
Manage a feature layer
Use a hosted feature layer item to set the properties and settings of a feature layer in a feature service.
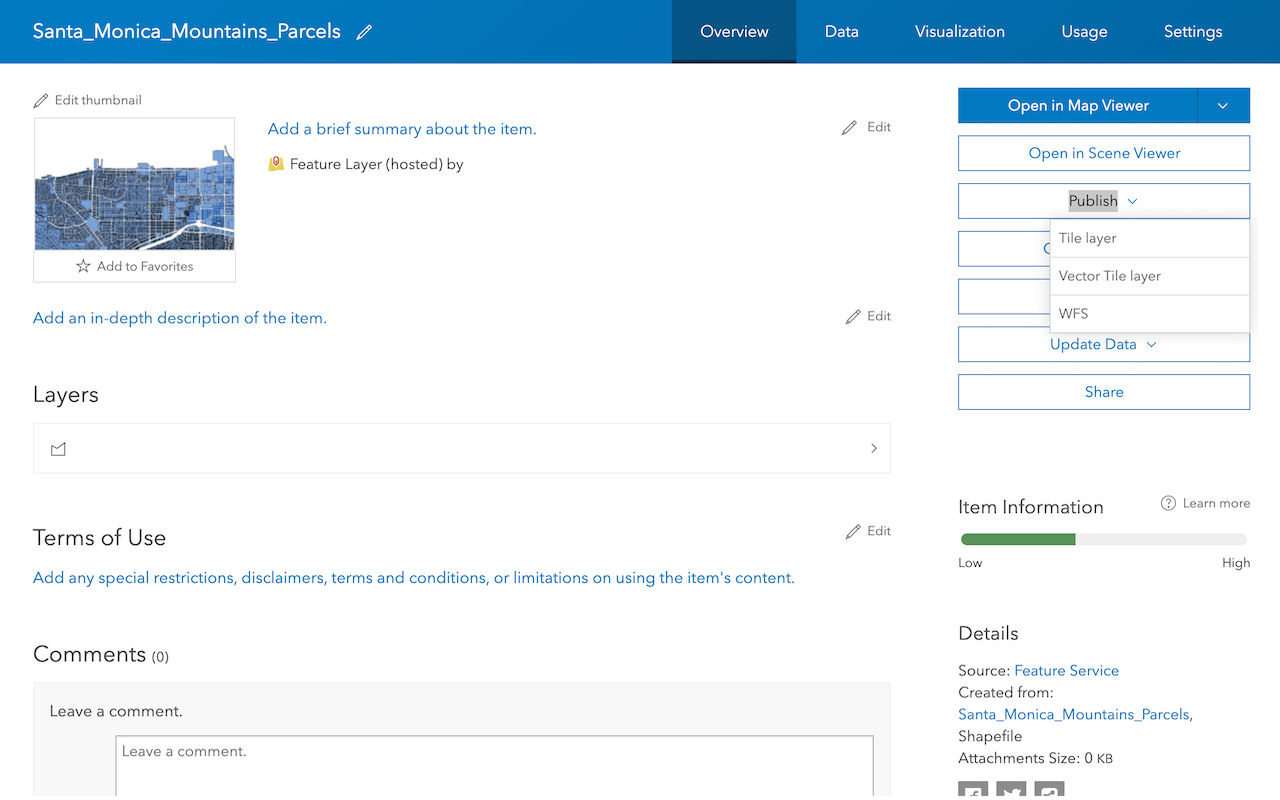
Create a vector tile service
Use data management tools to create a new vector tile service from a feature service.
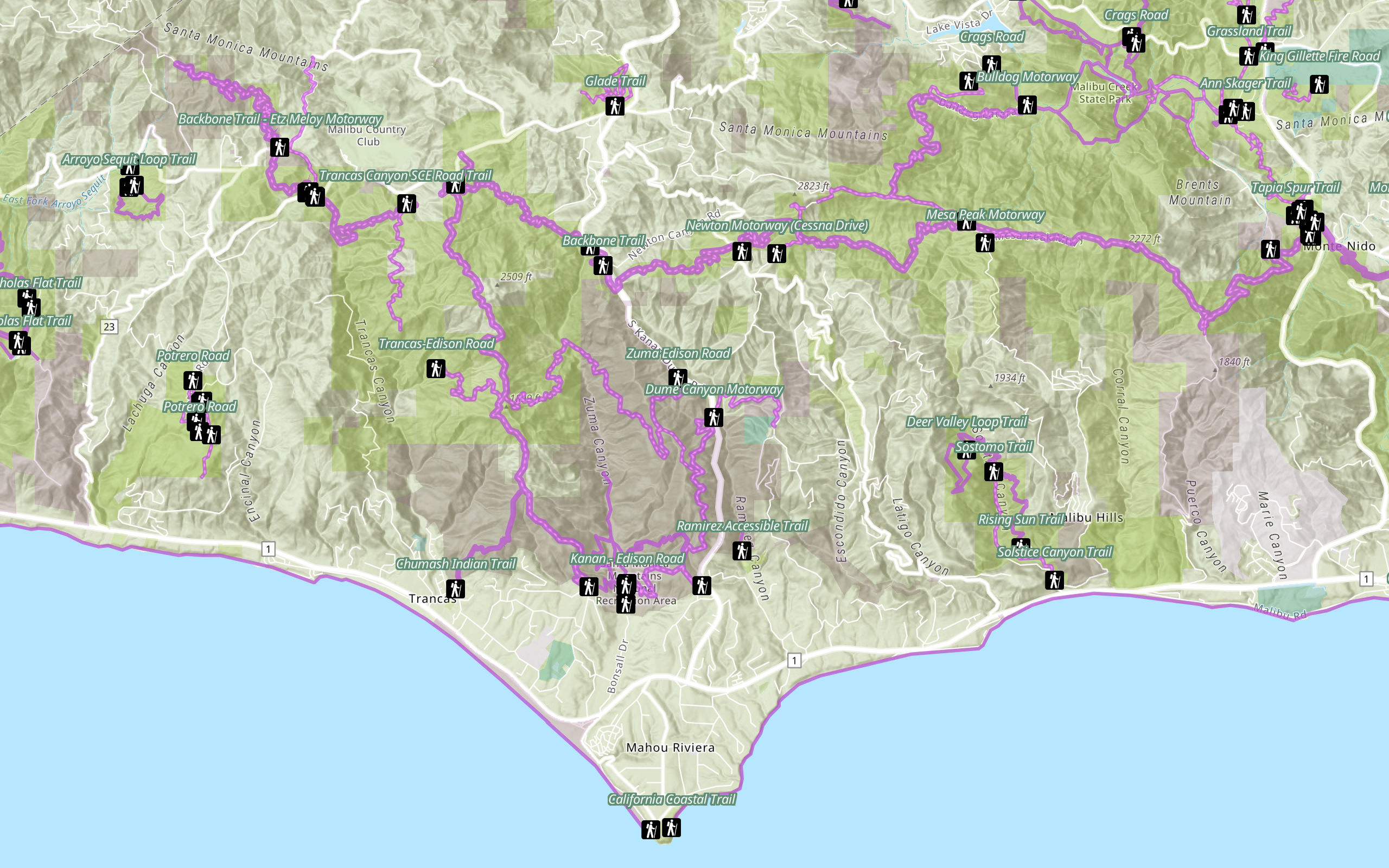
Add a feature layer
Access and display point, line, and polygon features from a feature service.
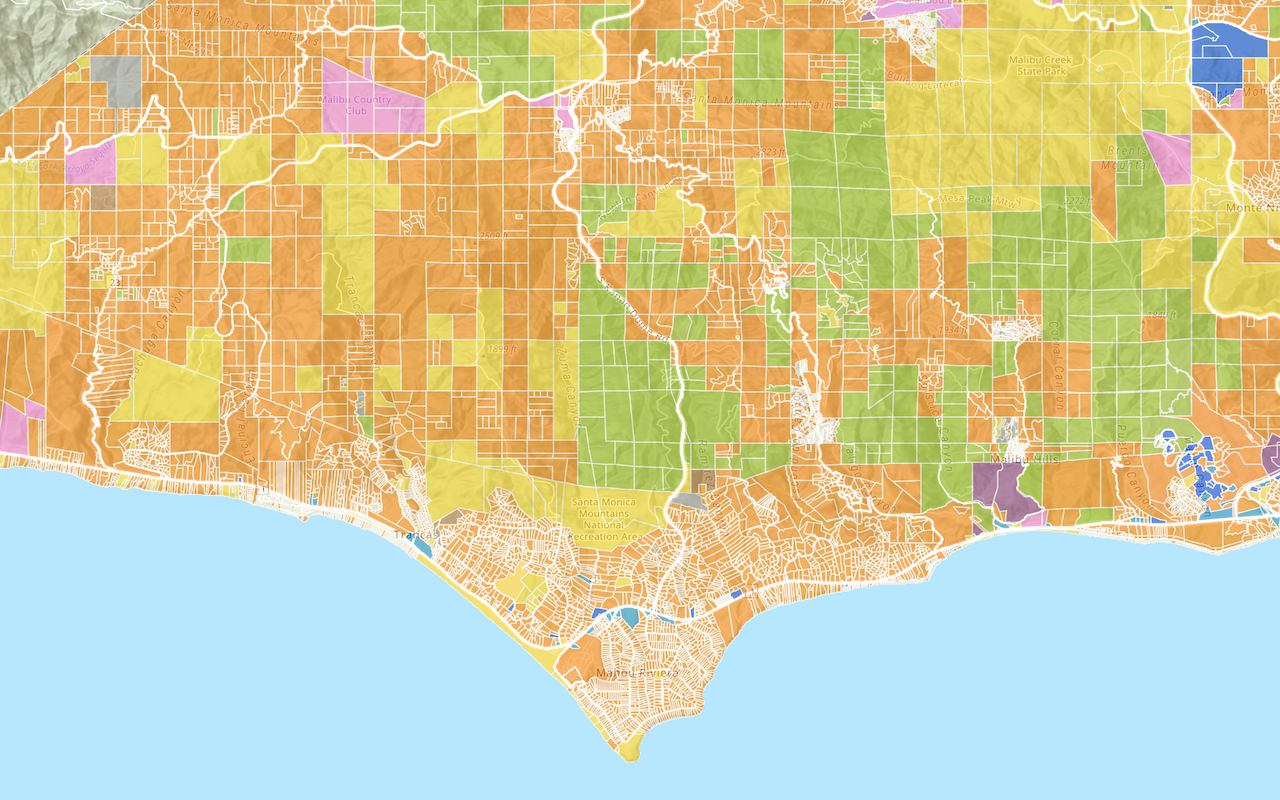
Add a vector tile layer
Access and display a vector tile layer in a map.
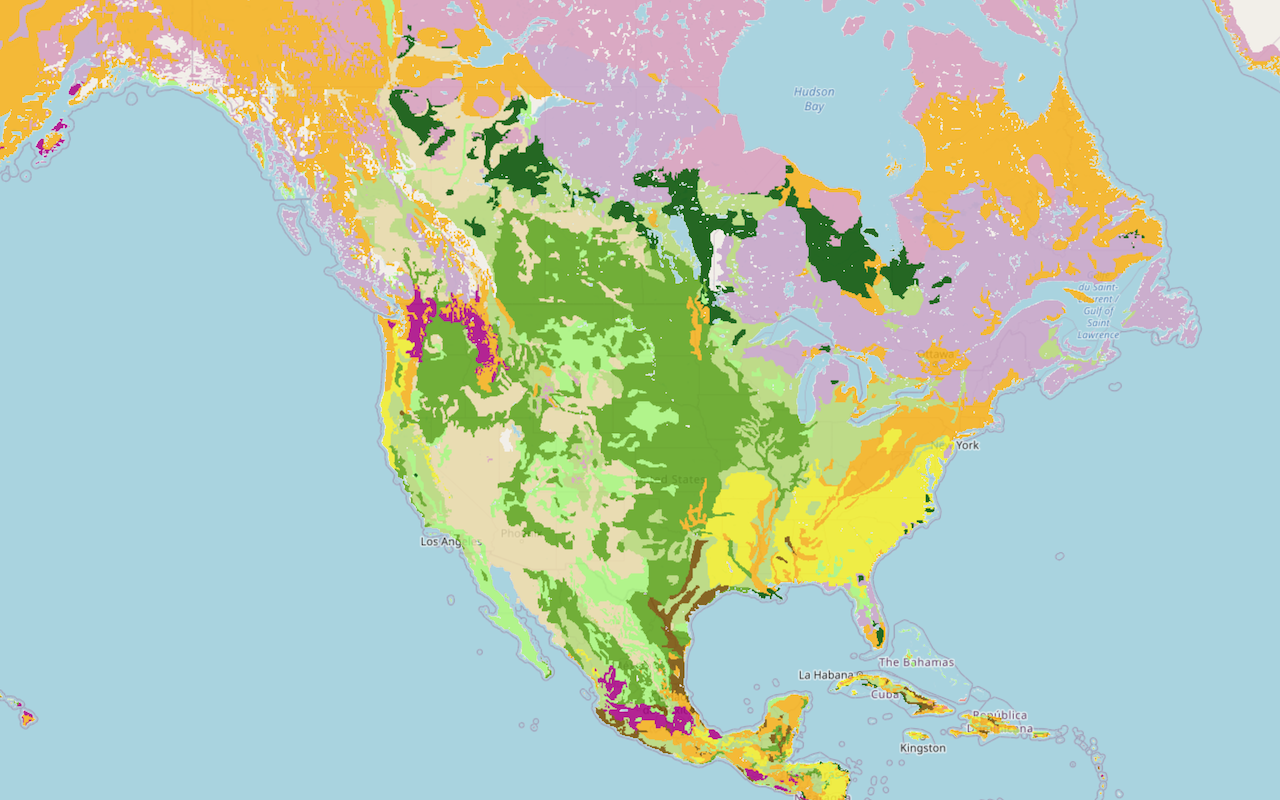
Add a map tile layer
Access and display a map tile layer in a map.
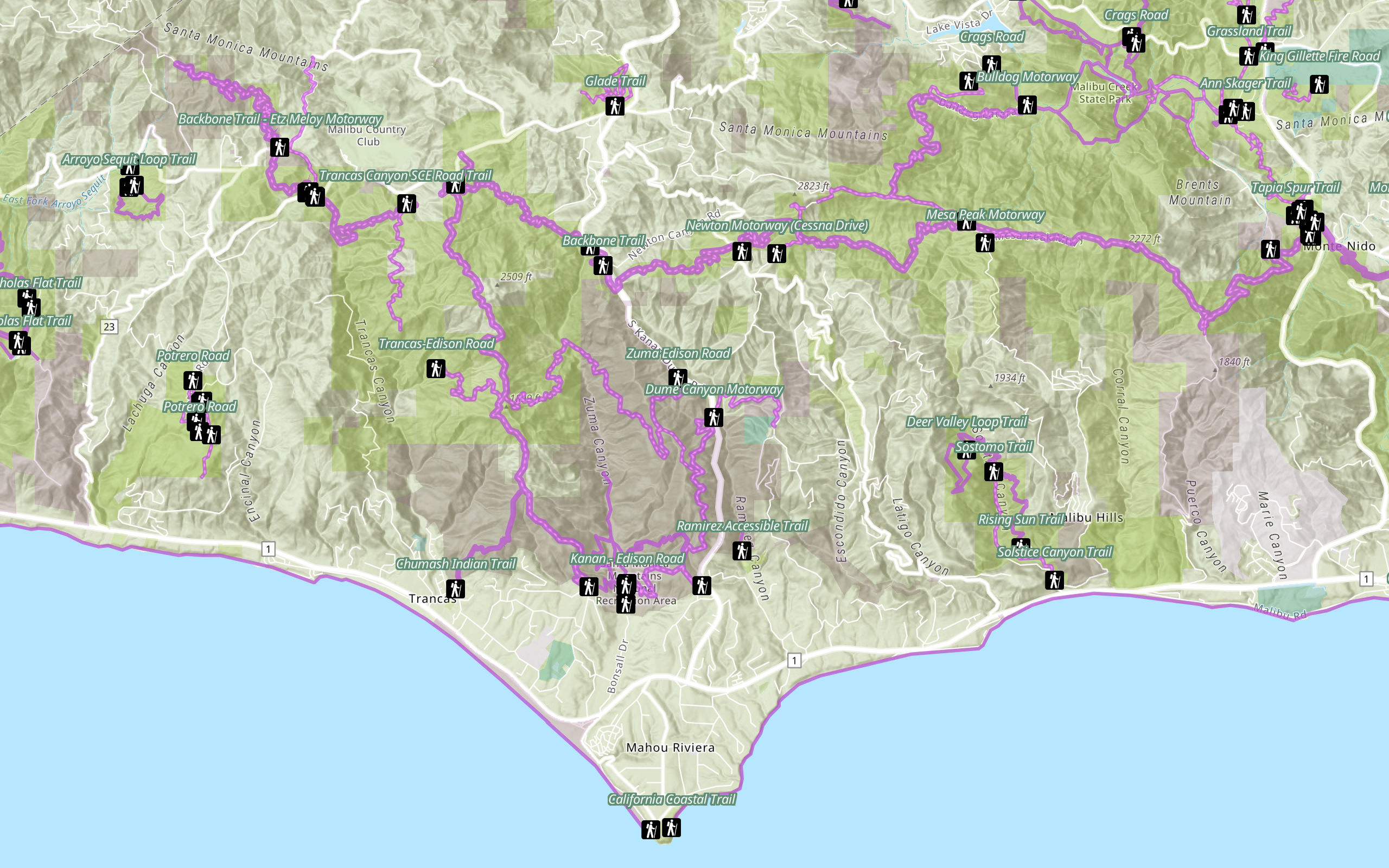
Style a feature layer
Use symbols and renderers to style feature layers.
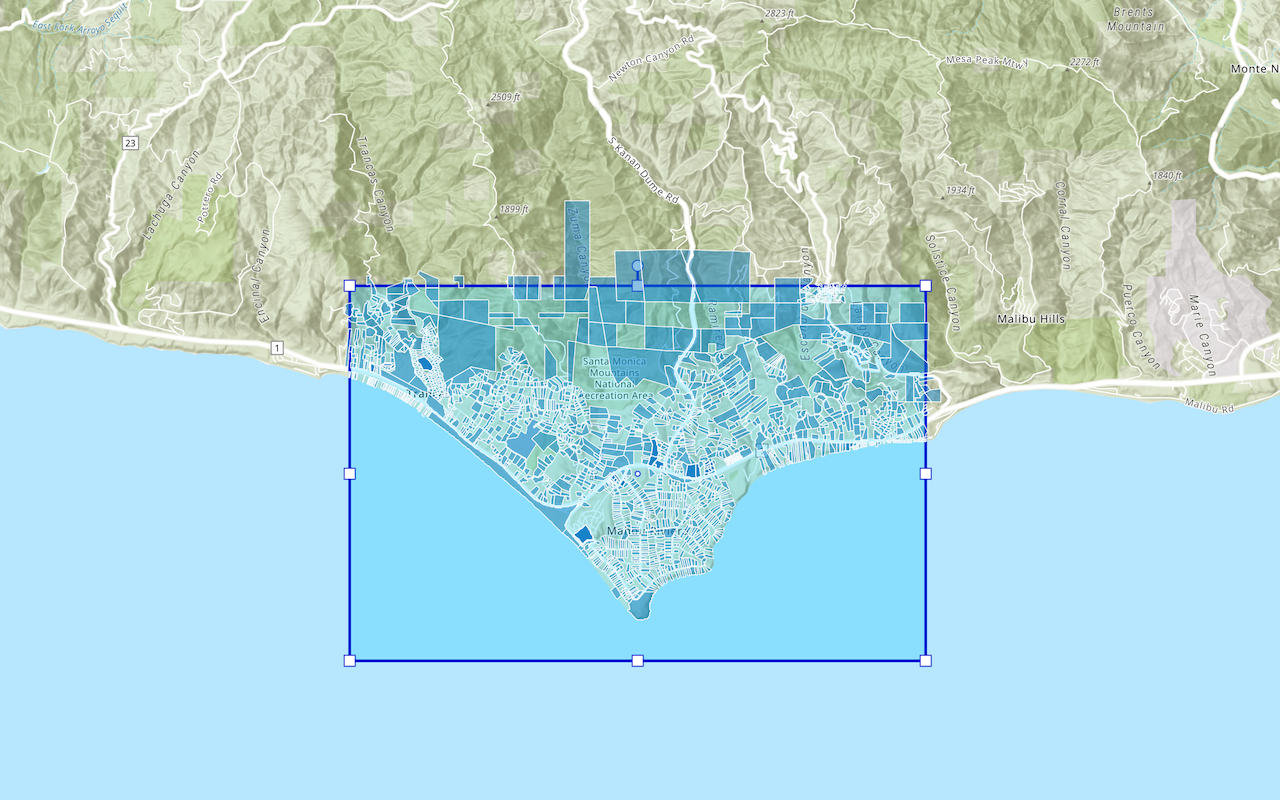
Query a feature layer (spatial)
Execute a spatial query to get features from a feature layer.
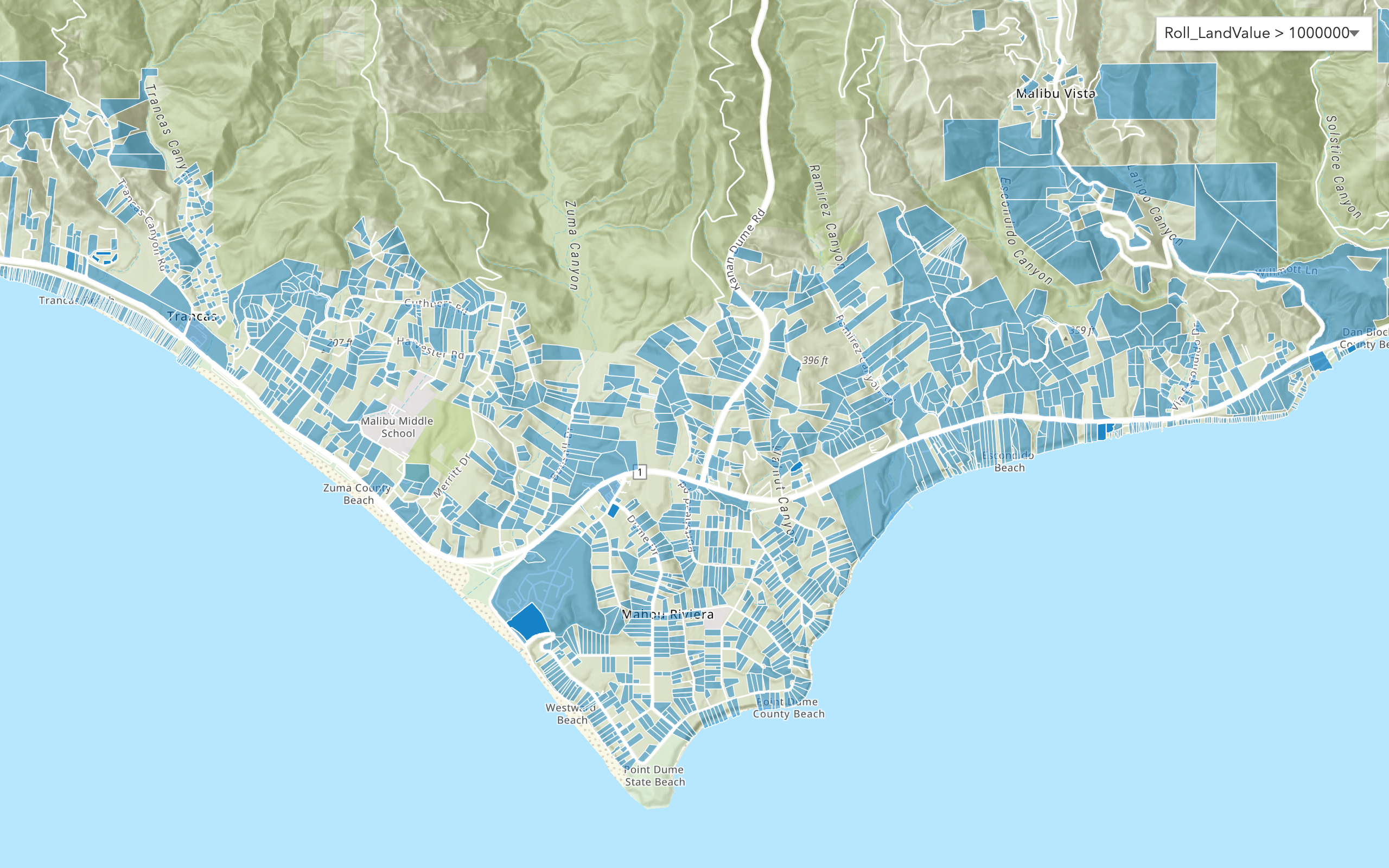
Query a feature layer (SQL)
Execute a SQL query to access polygon features from a feature layer.
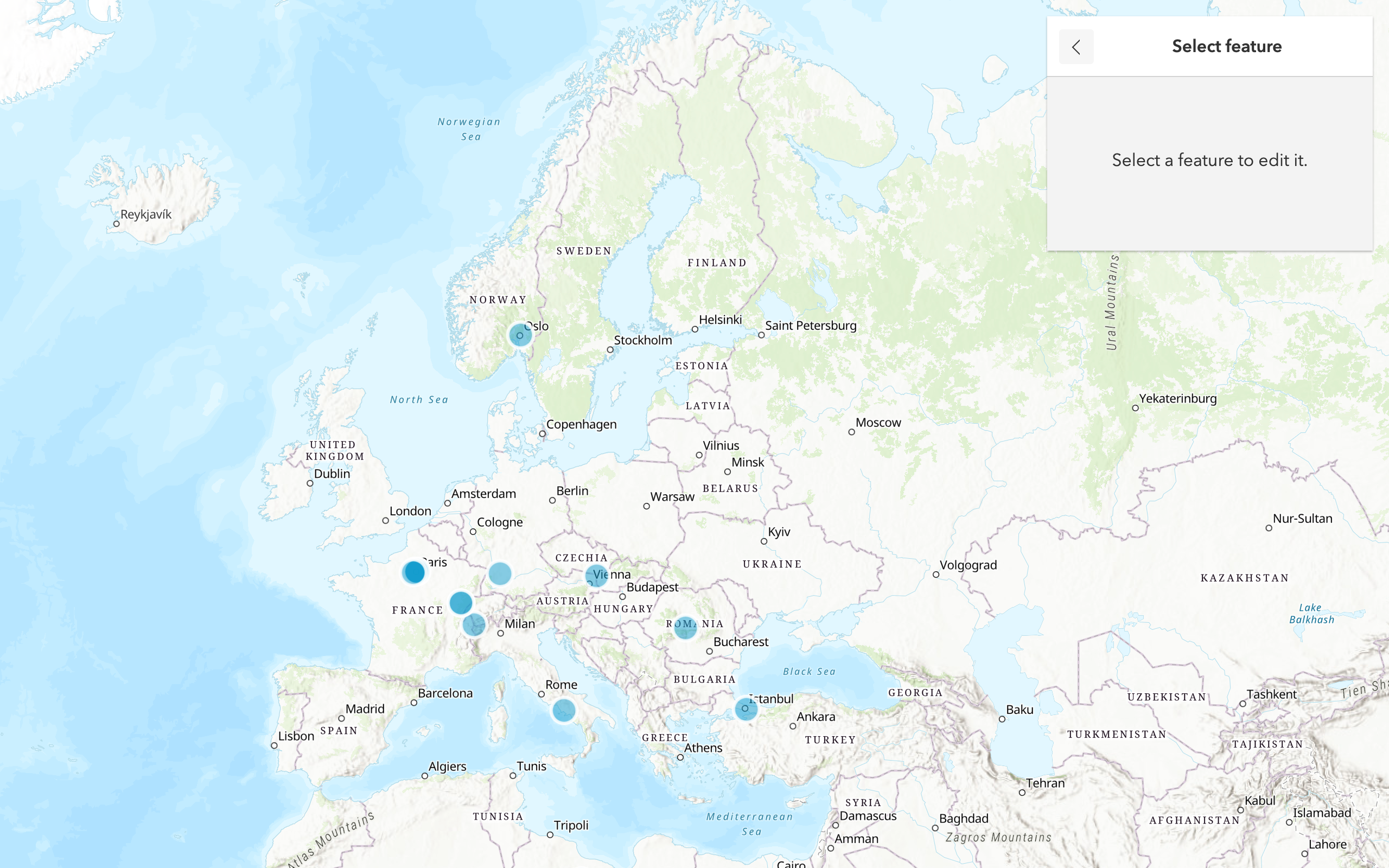
Edit feature data
Add, update, and delete features in a feature service.
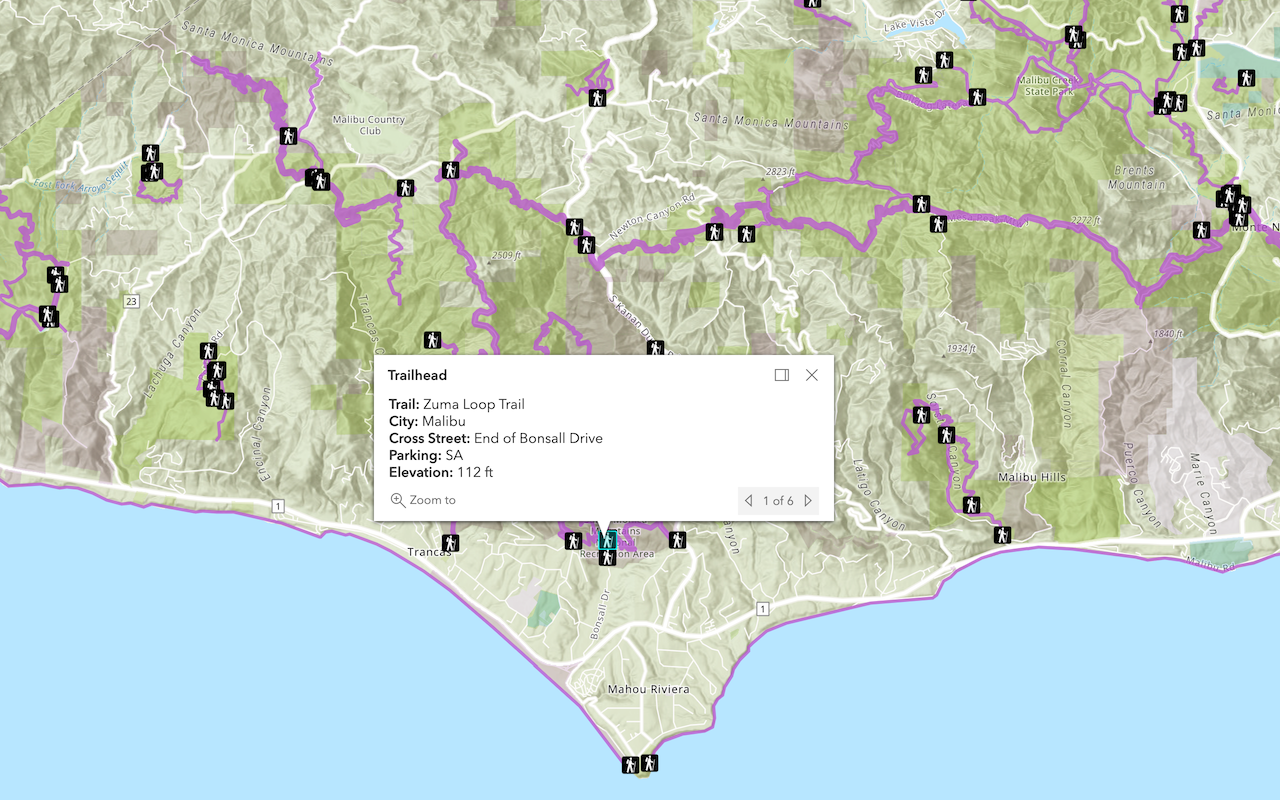
Display a popup
Format a popup to show attributes in a feature layer.