What is a basemap?
A basemap, also known as a basemap layer, is a layer that provides the overall visual context for a map or scene. It typically contains geographic features and labels for continents, lakes, administrative boundaries, streets, cities, and places. The most common data sources for a basemap are the basemap styles service and data services.
You can use a basemap layer to:
- Display different types of geographic data of the world for both maps and scenes.
- Display basemap styles such as ArcGIS streets, navigation, or light gray canvas, or OSM streets.
- Display custom basemap styles with your own colors, glyphs, and fonts.
- Display vector tile layers for streets or navigation.
- Display map tile layers for satellite imagery or hillshade.
- Display your own data services with their own spatial references.
How a basemap works
A basemap layer provides the visual foundation for a mapping application. It typically contains data with global coverage and is the first layer added to a map or scene. When a view displays a map, the basemap layer is the first layer to draw, followed by data layers, and then graphics.
Figure 1: A basemap layer provides visual context for a map or scene.
Types of data sources
Two common data sources for a basemap are the basemap styles service and data services.
Basemap styles service
The basemap styles service is a location service that provides basemap styles and data for the extent of the world. Each basemap style has a unique set of visual properties for geographic features and labels. The service includes two data providers: ArcGIS and OSM. The data providers supports default basemap styles such as streets, navigation, light gray canvas, and imagery. The data for each style is provided through vector tile layers and map tile layers hosted in ArcGIS and is stored in the web mercator spatial reference.
The general steps to use the basemap style service are:
-
Explore the default basemap styles available with the basemap styles service.
-
Reference the service, data provider, and style.
- Data providers:
ArcGIS
orOSM
. - Basemap styles: E.g.
streets
,imagery
,navigation
,topography
,light gray canvas
, oroutdoors
.
- Data providers:
-
Display the basemap.
The ArcGIS Maps SDKs provide enumerations or helper classes to access each style. When using open source libraries, however, you need to reference the basemap styles service or the underlying vector tile or map tile layers directly.
esriConfig.apiKey = "YOUR_API_KEY";
const map = new Map({
basemap: "arcgis-navigation"
});
Data services
Data services such as feature services, vector tile services, and map tile services are services hosted in ArcGIS.com or ArcGIS Enterprise that contain your data. In most cases, any data service supported by a client API can be used as a data source for a basemap. Data services can be accessed and managed with a hosted layer (item) in ArcGIS.
The general steps to use a data service are:
- Find or create a hosted layer and data service:
- Find existing services in ArcGIS Online and ArcGIS Living Atlas of the World.
- Learn how to create your own data services in Data hosting.
- Get the layer item ID or service URL. For example:
- Layer item:
https:
//www.arcgis.com/home/item.html?id=4d9fb5c0a6344407aec56f47a11482b5 - Item ID:
4d9fb5c0a6344407aec56f47a11482b5
- Service URL:
https:
//services2.arcgis.com/Fia PA4ga0i QKduv3/arcgis/rest/services/State_ Geologic_ Map_ Compilation_ %E2%80%93_ Geology/Feature Server/0
- Layer item:
- Display the basemap.
ArcGIS Maps SDKs access data services with a hosted layer item ID or service URL. Open source libraries however access data services with the service URL.
const featureLayer = new FeatureLayer({
portalItem: {
id: "4d9fb5c0a6344407aec56f47a11482b5" // State Geologic Map Compilation – Geology
}
});
const basemap = new Basemap({
baseLayers: [featureLayer]
});
Code examples
Display a basemap style
This example shows how to use the basemap styles service as a data source for a basemap. To do so, you use one of the default basemap styles. To see all of the styles, go to Basemap styles service (v2).
Steps
- Create a map.
- Reference a style from the basemap styles service.
- Add the basemap to the map.
esriConfig.apiKey = "YOUR_API_KEY"
const map = new Map({
basemap: "arcgis/streets",
//basemap: "arcgis/navigation"
//basemap: "arcgis/topographic"
//basemap: "arcgis/outdoor"
//basemap: "arcgis/light-gray"
//basemap: "arcgis/imagery"
//basemap: "osm/standard"
//basemap: "osm/navigation"
//basemap: "osm/blueprint"
})
const view = new MapView({
map: map,
center: [-118.805, 34.027],
zoom: 12,
container: "viewDiv",
constraints: {
snapToZoom: false,
},
})
ArcGIS/Streets
ArcGIS/Navigation
ArcGIS/Topographic
ArcGIS/Outdoor
ArcGIS/Light gray canvas
ArcGIS/Imagery
OSM/Standard
OSM/Navigation
OSM/Blueprint
Display localized place labels
The basemap styles service displays English language labels by default. This example shows how to display the OSM styles with localized and language-based place labels.
Steps
- Create a map or scene.
- Reference a style from the basemap styles service (v2).
- In the style URL, set the
language
parameter with a language code. - Add the basemap to the map.
Localized place labels (local)
This example uses the arcgis/light-gray
map style. By default, place labels display global place names. To render localized place labels, set the language
parameter to local
. The localized labels will render after zoom level 10.
const apiKey = "YOUR_API_KEY";
const map = L.map("map").setView([2.35, 48.856], 6);
L.esri.Vector.vectorBasemapLayer("arcgis/light-gray", {
apikey: apiKey,
language: 'local',
version: 2
}).addTo(map);
Language-based place labels (global)
This example uses the arcgis/dark-gray
map style. It sets the language
parameter with a language code, in this case ja
, so that all place labels at varying zoom levels are rendered in Japanese.
const apiKey = "YOUR_API_KEY";
const map = L.map("map").setView([2.35, 48.856], 6);
L.esri.Vector.vectorBasemapLayer("arcgis/dark-gray", {
apikey: apiKey,
language: 'ja',
version: 2
}).addTo(map);
Display a worldview
The basemap styles service displays country boundaries and labels using the default global worldview. This example shows how to display basemap borders and labels based on a specific view of a country. Worldviews are only available for some ArcGIS basemap styles such as navigation
, streets
, and community
. OSM styles are not supported.
Steps
- Create a map or scene.
- Reference a basemap layer from the basemap styles service (v2).
- In the style URL, set the
worldview
parameter with a supported worldview name. - Add the basemap to the map.
This example uses the arcgis/light-gray
map style and sets the worldview
for boundaries and labels to morocco
. To find all worldview
options, go to Basemap styles service (v2).
const worldView = "morocco"
const map = new maplibregl.Map({
container: "map", // ID of the div element
style: `https://basemapstyles-api.arcgis.com/arcgis/rest/services/styles/v2/styles/arcgis/light-gray?token=${apiKey}&worldview=${worldView}`,
zoom: 3,
center: [-7.09, 31], // Starting location [longitude, latitude]
})
Display a hosted layer (data service)
This example shows how to use a data service in ArcGIS as the data source for a basemap. The data is a hosted feature layer that shows geology across the USA. To do so you need to reference the item IDs for the hosted layer.
Steps
- Find the hosted layer item ID.
- Create a basemap and add the layer as base layers.
- Create a map and use the basemap.
const featureLayer = new FeatureLayer({
portalItem: {
id: "4d9fb5c0a6344407aec56f47a11482b5" // State Geologic Map Compilation – Geology
}
});
const basemap = new Basemap({
baseLayers: [featureLayer]
});
Tutorials

Display a map
Create and display a map with the basemap styles service.
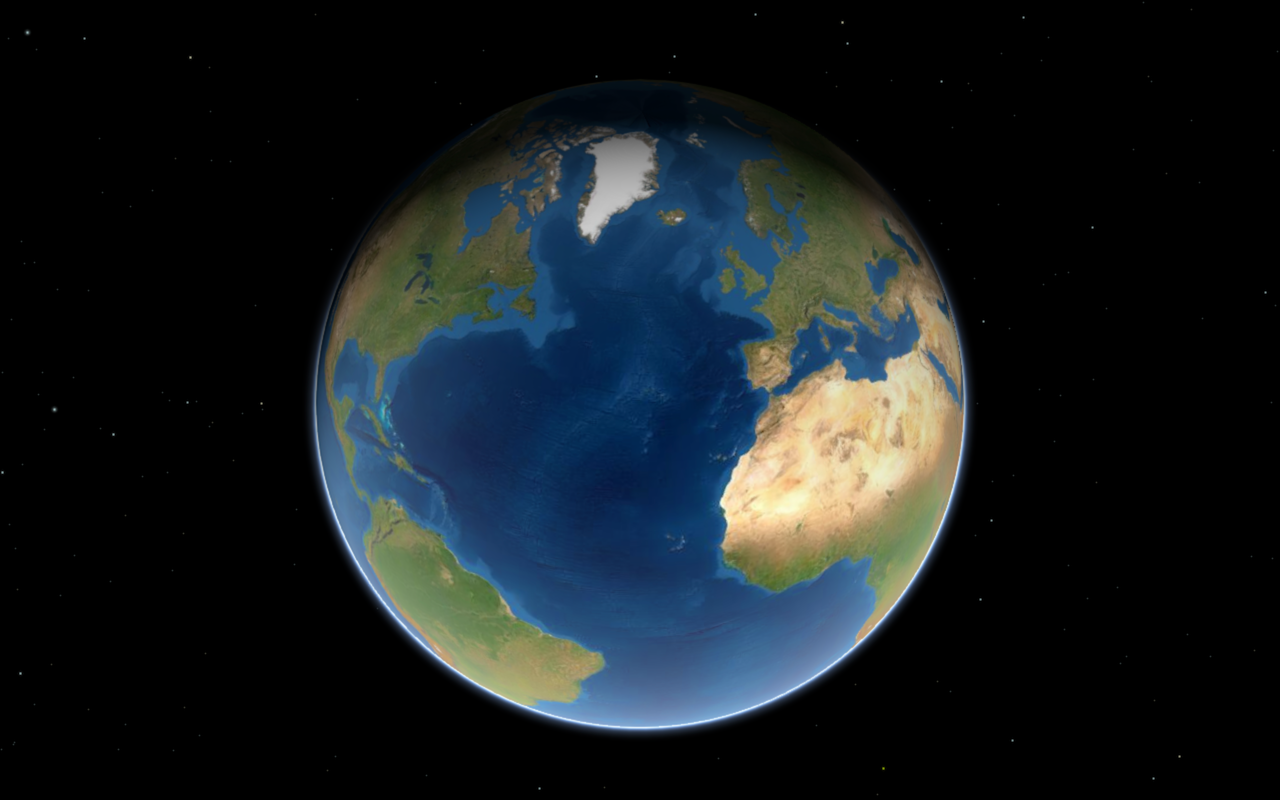
Display a scene
Display a scene with the basemap styles service.
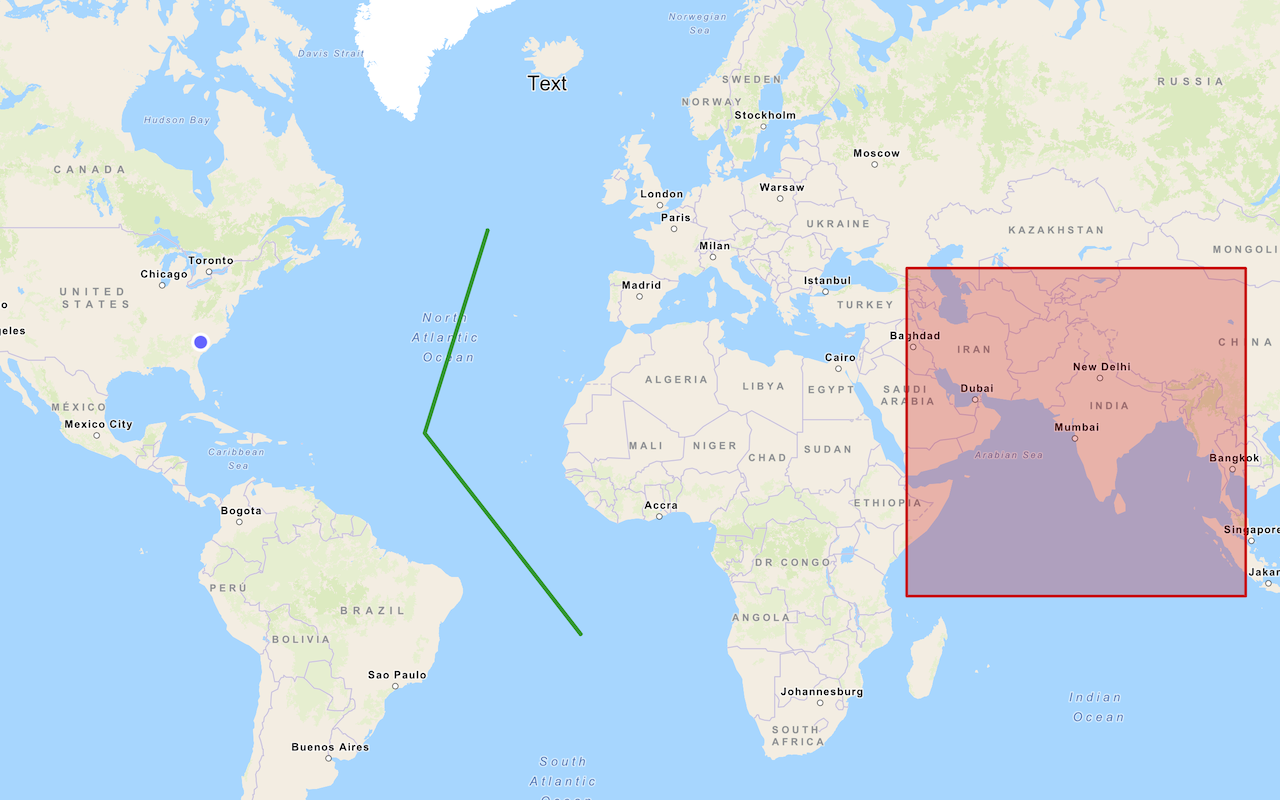
Add a point, line, and polygon
Display point, line, and polygon graphics in a map.
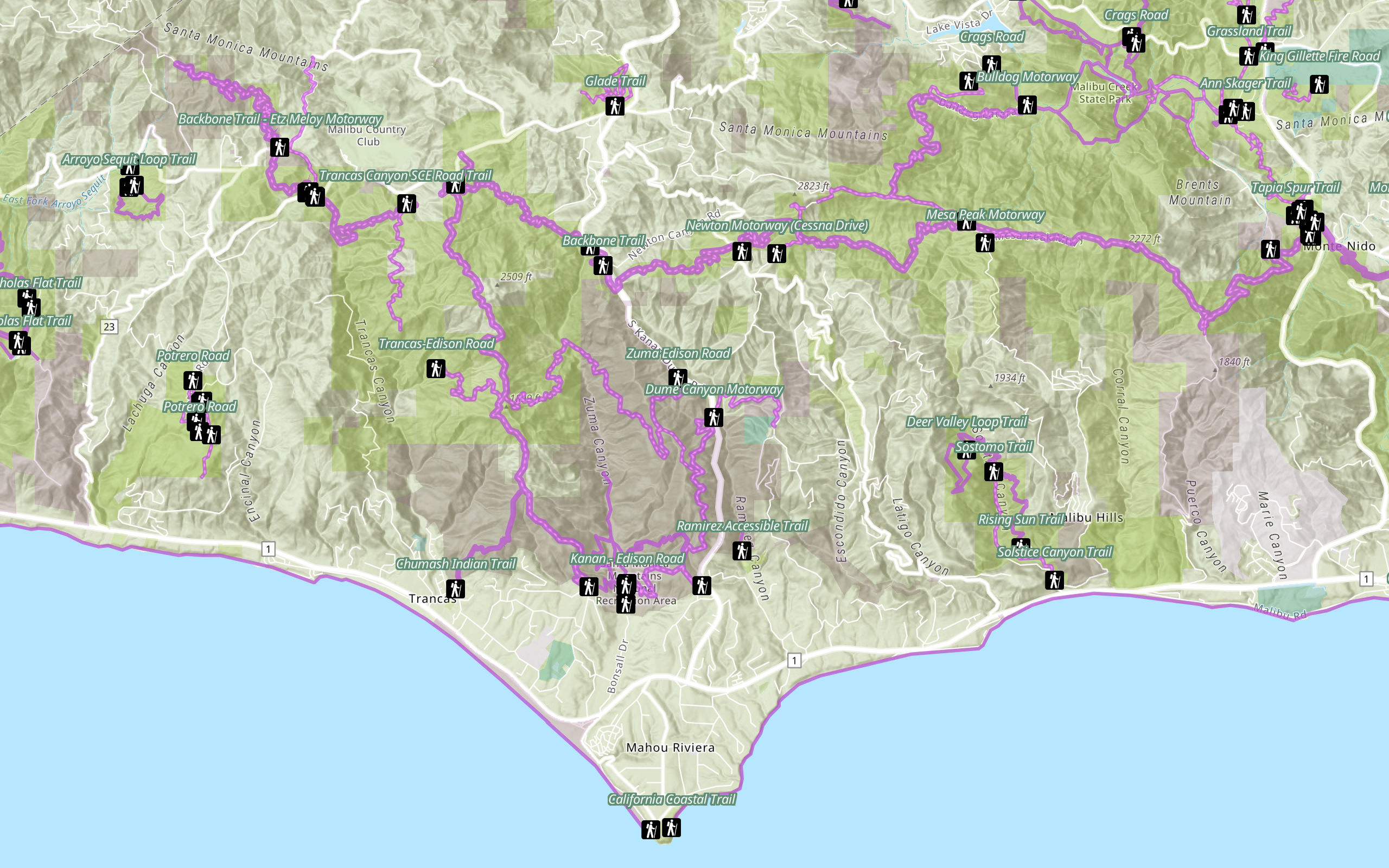
Add a feature layer
Access and display point, line, and polygon features from a feature service.
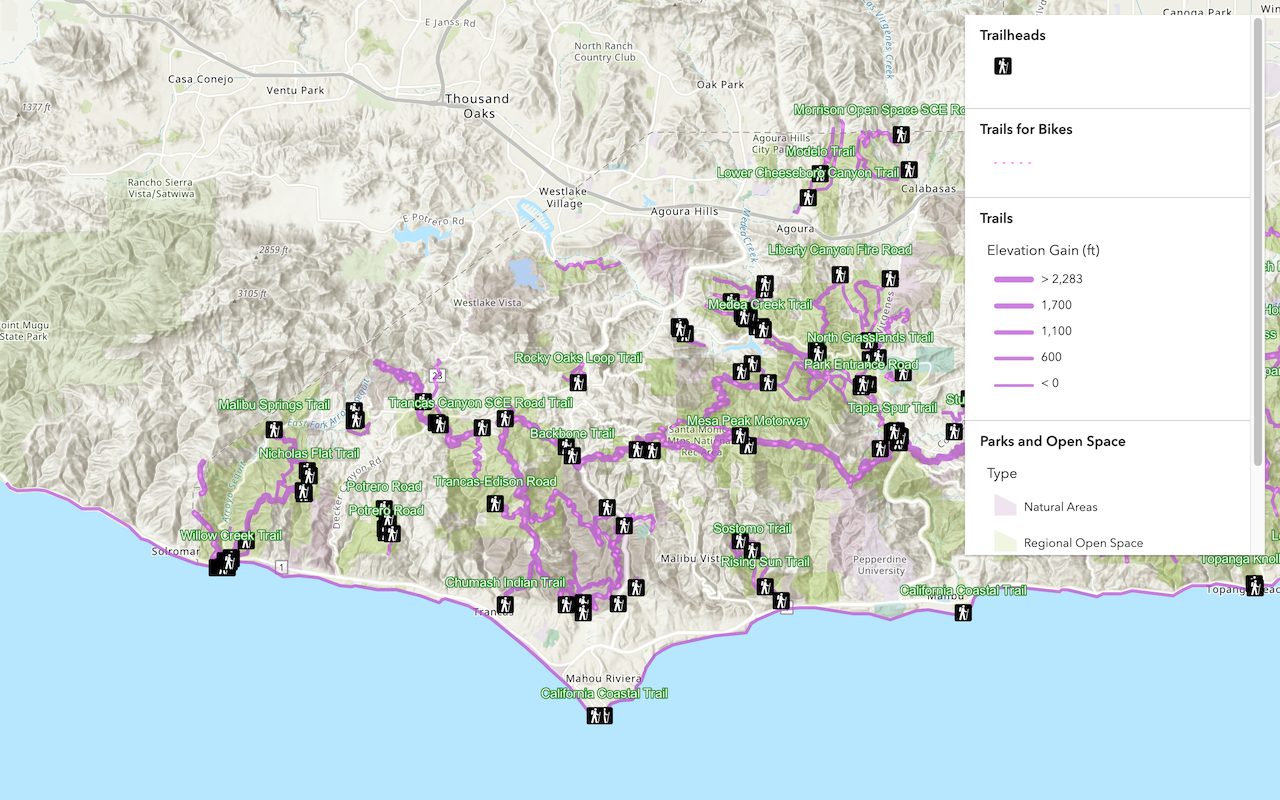
Display a web map
Create and display a map from a web map.
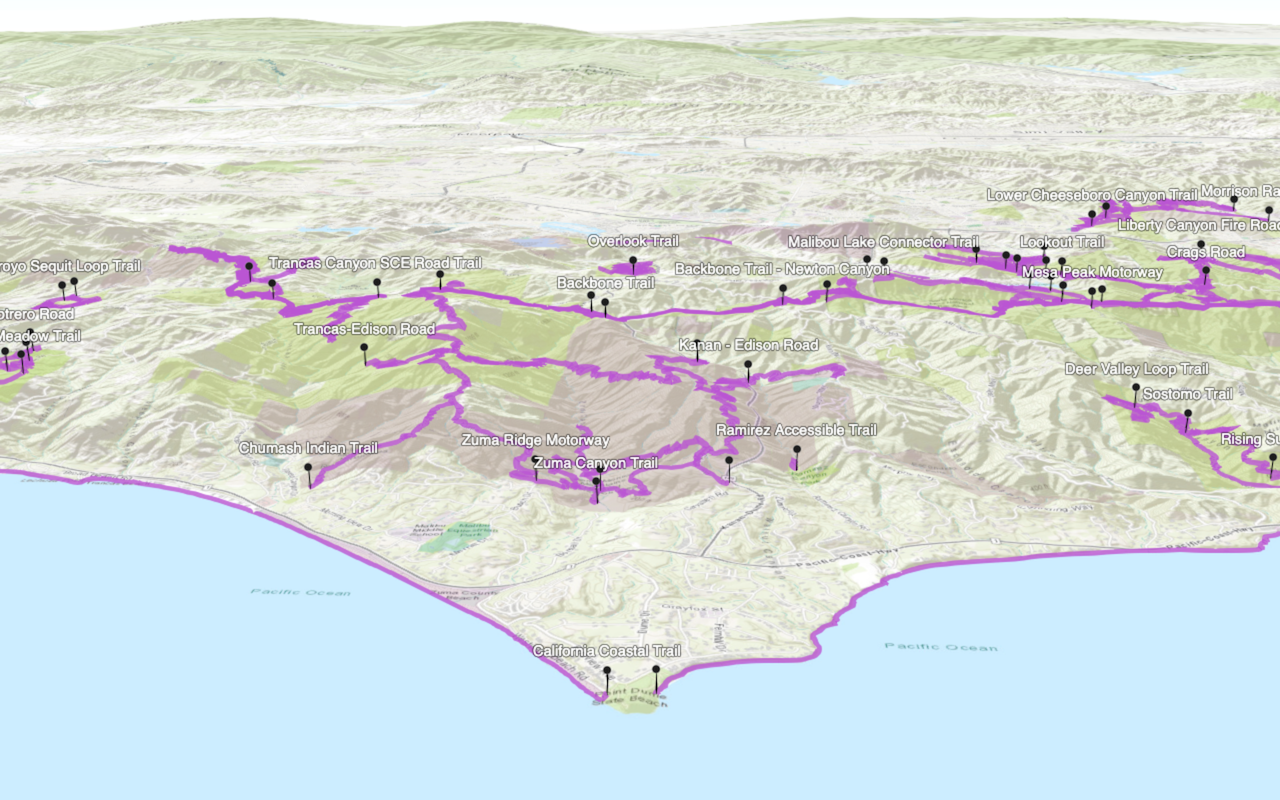
Display a web scene
Create and display a scene from a web scene.
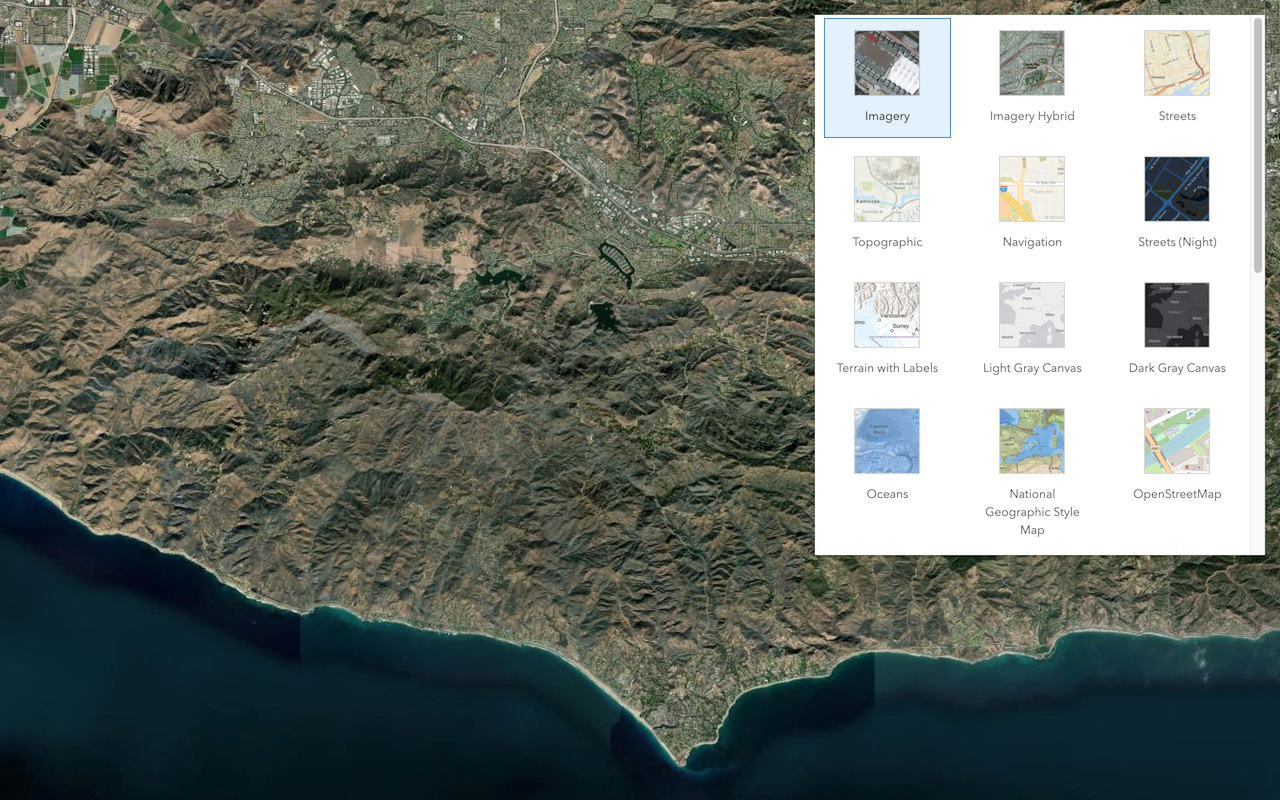
Change the basemap layer
Switch a basemap layer from streets to satellite imagery.
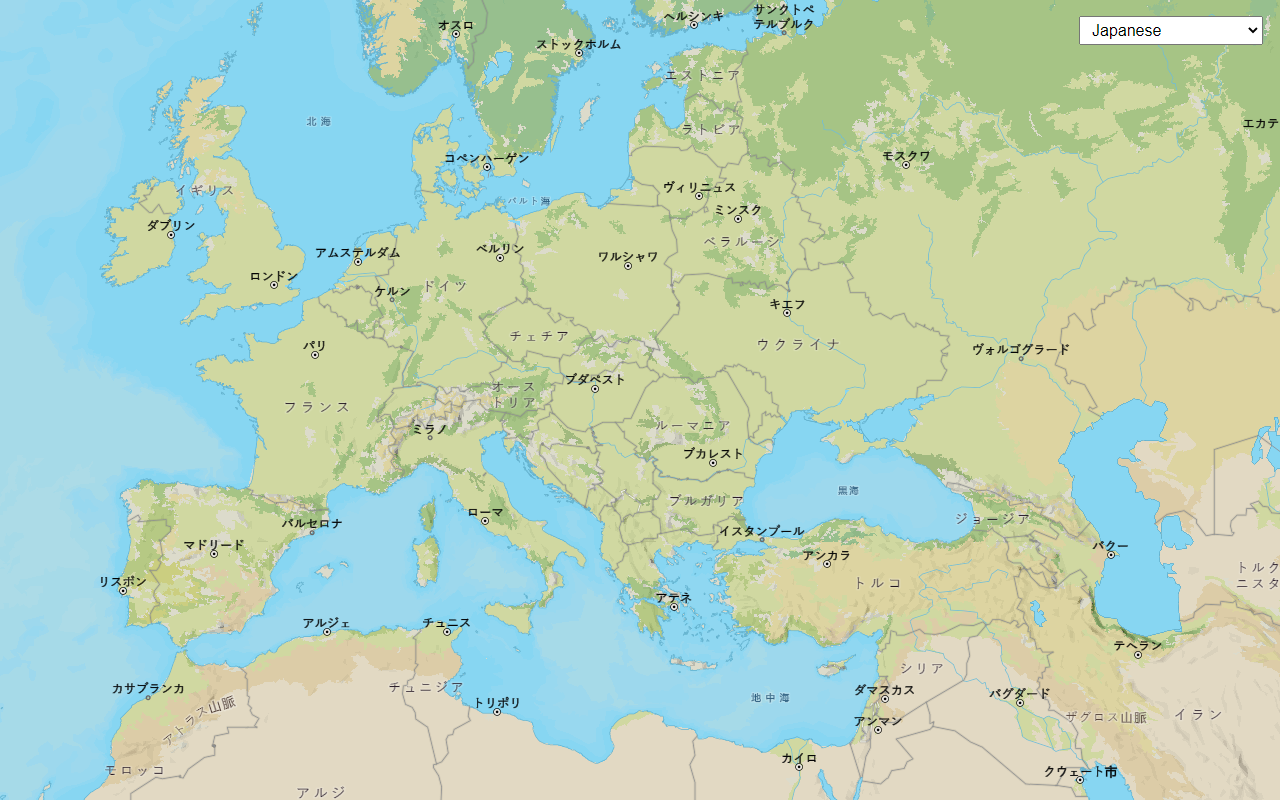
Change the place label language
Switch the language of place labels on a basemap.
Services
Basemap styles service (v1)
Access basemap styles such as streets, navigation, and imagery for maps and scenes.
Basemap styles service (v2)
Access basemap styles and additional features such as localized languages, worldviews, and places.
Feature service
Add, update, delete, and query feature data.
Vector tile service
Store and access vector tile data.
Map tile service
Store and access map tile data.
API support
2D Display | 3D Display | Basemap layers | Basemap places | Data layers | Graphics | Web maps | Web scenes | |
---|---|---|---|---|---|---|---|---|
ArcGIS Maps SDK for JavaScript | 1 | |||||||
ArcGIS Maps SDK for .NET | ||||||||
ArcGIS Maps SDK for Kotlin | ||||||||
ArcGIS Maps SDK for Swift | ||||||||
ArcGIS Maps SDK for Java | ||||||||
ArcGIS Maps SDK for Qt | ||||||||
ArcGIS API for Python | ||||||||
ArcGIS REST JS | 2 | 2 | ||||||
Esri Leaflet | 3 | 4 | ||||||
MapLibre GL JS | 3 | 4 | ||||||
OpenLayers | 1 | 3 | 4 | |||||
CesiumJS | 3 | 4 |
- 1. Display places only.
- 2. Access via HTTP request and authentication.
- 3. Access via Feature layer or Map tile layer.
- 4. Access via layers.