You can build applications that display maps and scenes, edit features, and analyze data using data files stored locally on your device.
What is a data file?
A data file is a local file stored on a device that contains data that can be displayed by a layer in a map or scene. Some data files also support editing, querying, and analyzing data.
You use data files to build offline applications that:
- Display maps or scenes without a network connection.
- Can access, display, and analyze data sideloaded onto a device.
- Include data with an application install.
- Collect data on devices that never have access to a network connection.
- Share datasets between applications using peer-to-peer technology.
Data files can be created using a number of tools or scripts, or downloaded from the internet.
How a data file works
To use a data file, you sideload or download it onto a device. You can then access the data file directly from local storage. You typically reference it with a layer and add the layer to a map or scene. Some types of data file support editing, while others are read-only.
The following types of data files are supported directly by the ArcGIS Maps SDKs for Native Apps:
Data file type | Data access API | Layer API | Can query? | Can edit? | License level |
---|---|---|---|---|---|
Vector tile package | Vector Tile Cache | ArcGIS Vector Tile Layer | No | No | Lite |
Image tile package | Tile Cache | Map Tile Layer | No | No | Lite |
Geodatabase. Note: this is limited to Native API geodatabases, typically downloaded as offline data from a feature service, or exported from ArcMap. | Geodatabase | Feature Layer | Yes | Yes | Lite |
Scene Layer Package For display in scenes only | N/A Access a Scene Layer Package (.slpk) file directly from the ArcGIS Scene Layer | ArcGIS Scene Layer | No | No | Lite |
Shapefile | Shapefile Feature Table | Feature Layer | Yes | Yes | Standard |
Local raster file. The following raster formats are supported: ASRP/USRP, CIB, CADRG/ECRG, DTED, GeoPackage Raster, GeoTIFF/TIFF, HFA, HRE, IMG, JPEG, JPEG2000, Mosaic Dataset in SQLite, NITF, PNG, RPF, SRTM, CRF, and MrSID. | Raster | Raster Layer | No | No | Standard |
OGC GeoPackage (feature data) | GeoPackage Feature Table | Feature Layer | Yes | Yes | Standard |
OGC GeoPackage (raster data) | GeoPackage Raster | Raster Layer | No | No | Standard |
OGC KML file | KML Dataset | KML Layer | No | Yes | Standard |
Electronic Nautical Chart (S-57). For display in maps only. Not supported in scenes. | ENC Cell | ENC Layer | No | No | Standard |
Other (e.g. GeoJSON) | Feature Collection | Feature Collection Layer | Yes | Yes | Lite |
Licensing
Files created by ArcGIS (vector tile packages, image tile packages, geodatabases, and scene layer packages) can be used with a free Lite license. Files that require custom parsing code and are used with a Feature Collection Layer can also be used with a free Lite license. Other files require a Standard license.
Steps to display the contents of a data file
The typical high level steps to display the geographic contents of a data file are:
- Sideload or download the data file to device.
- Access the data file with an Native SDK.
- Create a layer referencing the data file.
- Add the layer to a map or scene.
This document covers steps 2-4.
Code examples
Display a shapefile
The steps to display a shapefile are:
- Create a Shapefile Feature Table referencing the shapefile in local storage.
- Create a Feature Layer with the Shapefile Feature Table.
- Add the Feature Layer to a Map displayed in a Map View.
var shapefileTable = new ShapefileFeatureTable("\\path\\to\\shapefile.shp");
var shapefileLayer = new FeatureLayer(shapefileTable);
MainMapView.Map.OperationalLayers.Add(shapefileLayer);
Display features from an OGC GeoPackage
The steps to display features in a GeoPackage are:
- Create a GeoPackage referencing the GeoPackage file in local storage.
- Load the GeoPackage to read its contents.
- Get a GeoPackage Feature Table from the GeoPackage Feature Tables collection in the GeoPackage.
- Create a Feature Layer with the GeoPackage Feature Table.
- Add the Feature Layer to a Map displayed in a Map View.
try
{
var geoPackage = await GeoPackage.OpenAsync("\\path\\to\\geopackage.gpkg");
await geoPackage.LoadAsync();
foreach (var table in geoPackage.GeoPackageFeatureTables)
{
var featureLayer = new FeatureLayer(table);
MainMapView.Map.OperationalLayers.Add(featureLayer);
}
}
catch(Exception ex)
{
// Handle error
}
To display the geopackage contents in 3D, you can add the Feature Layer to a Scene displayed in a Scene View.
Query features in a shapefile (SQL)
The steps to query features in a shapefile using a SQL where clause are:
- Create a Shapefile Feature Table referencing the shapefile in local storage.
- Create a Query Parameters object and set a whereClause.
- Call Query Features With Parameters on the Shapefile Feature Table.
try
{
var shapefileTable = await ShapefileFeatureTable.OpenAsync("\\path\\to\\shapefile");
var queryParameters = new QueryParameters { WhereClause = "height < 12" };
var queryResult = await shapefileTable.QueryFeaturesAsync(queryParameters);
foreach(var feature in queryResult)
{
double height = (double)feature.Attributes["height"];
// do something with height value
}
}
catch (Exception ex)
{
// Handle error
}
Tutorials
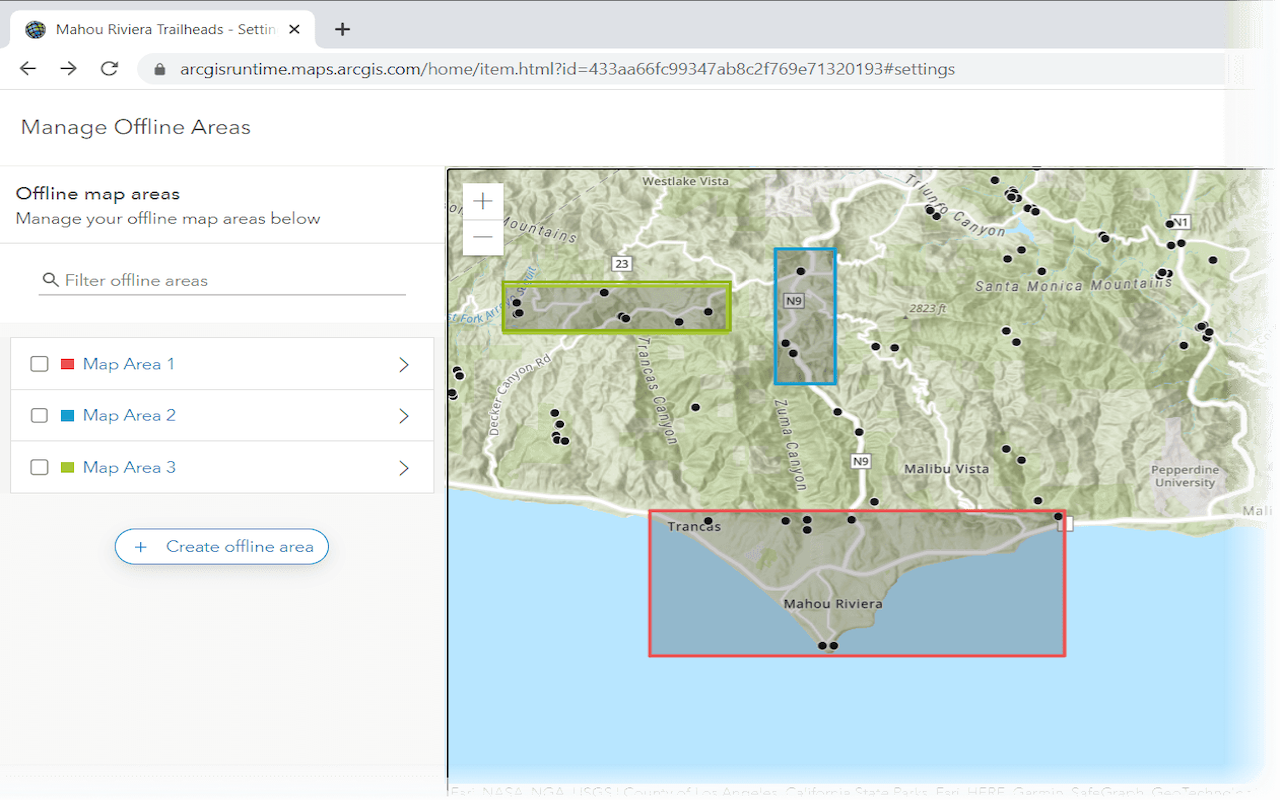
Create an offline map area
Use ArcGIS Online to create an offline map area that can be downloaded by any number of users.
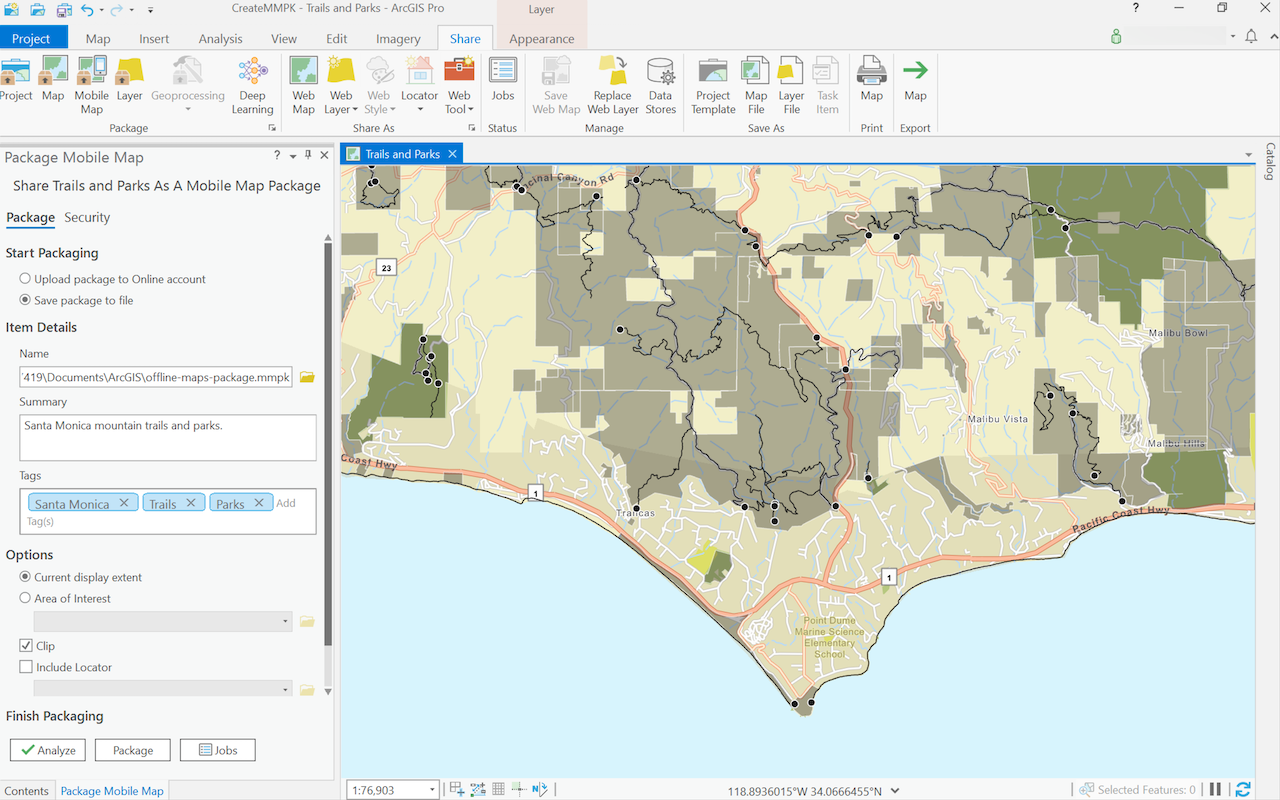
Create a mobile map package
Use ArcGIS Pro to create a mobile map package.
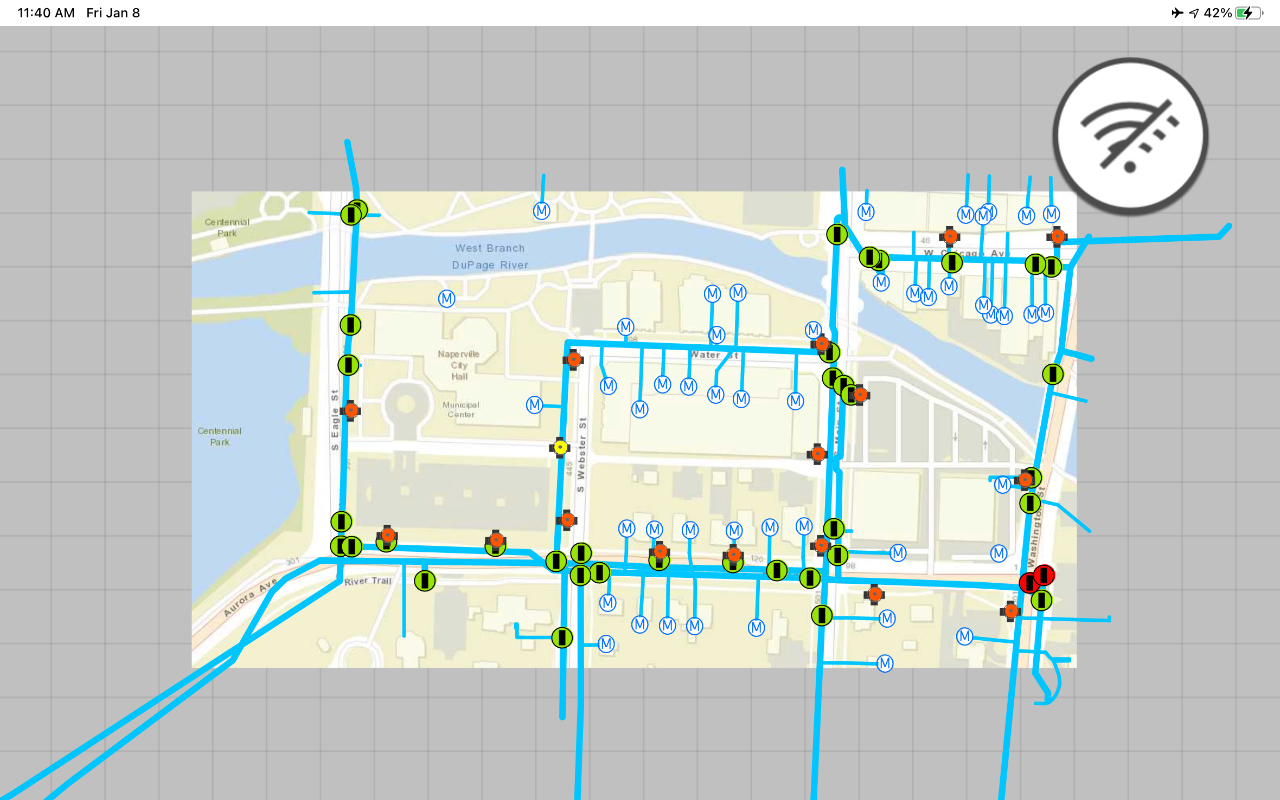
Display an offline map on demand
Download and display an offline map for a custom area of a web map stored in ArcGIS.
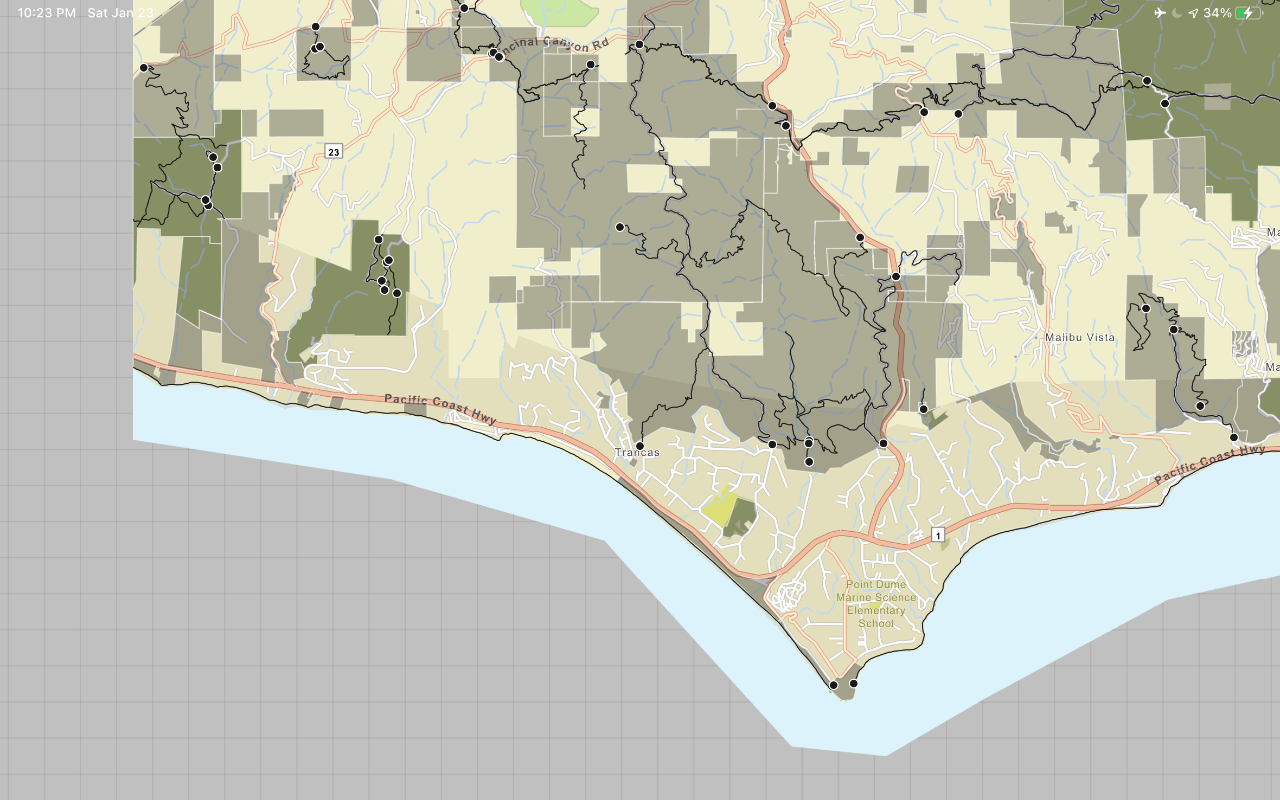
Display a map from a mobile map package
Access and display a map from a mobile map package for offline use.
API support
- 1. Geometry engine
- 2. Manage offline map areas