You can build applications that can display maps or scenes, geocode, and find routes without a network connection using mobile packages.
What is a mobile package?
A mobile package contains one or more maps or scenes. It also contains data content that is referenced by layers in the maps or scenes. Mobile packages are used to display maps and scenes, geocode, reverse geocode, find routes and directions, and analyze data without a network connection.
There are two types of mobile package:
- Mobile map package (MMPK): This type of package contains maps.
- Mobile scene package (MSPK): This type of package contains scenes.
You use mobile packages to build offline applications that:
- Display interactive maps or scenes without a network connection.
- Distribute multiple maps or scenes to mobile workers.
- Geocode addresses and find places offline.
- Get nearby addresses (reverse geocode).
- Calculate turn-by-turn directions offline.
- Include maps and scenes with an application install.
- Distribute maps and scenes that cannot be used after a specific date and time.
You create a mobile package from desktop maps and scenes in ArcGIS Pro. A map or scene in a mobile package shares many capabilities of the desktop map or scene:
- Zoom, pan, and rotate the map or scene.
- Interact with the map's or scene's layers.
- Query and identify features in feature layers.
- Display popups as configured in the desktop map or scene.
- Control feature layer styling.
- Control the visible scale ranges for layers.
- Read preconfigured bookmarks.
How a mobile package works
A mobile package begins with an ArcGIS Pro project containing maps and/or scenes. ArcGIS Pro includes tools to:
- Package up maps and data into a mobile map package file of type
.mmpk
. - Package up scenes and data into a mobile scene package file of type
.mspk
.
You can then sideload or download a mobile package to a device and use it without a network connection.
The typical steps to use a map or scene in a mobile package are:
- Author an ArcGIS Pro project containing maps and/or scenes.
- Create a mobile package using the appropriate ArcGIS Pro tool.
- To create a mobile map package, you can use the Share Mobile Map Package tool or the Create Mobile Map Package tool.
- To create a mobile scene package, you can use the Create Mobile Scene Package tool.
- Sideload or download the mobile package to a device.
- Access and display a map or scene from the mobile package.
This document will cover step 4. For steps 1-3, see the Create a mobile map package tutorial.
Data content
Each map or scene contained in a mobile package can include basemap layers, data layers, and non-spatial tables. A mobile package can include different types of data to support the layers in the package's maps or scenes.
Offline content
A mobile package can include data content for layers and non-spatial tables. Layers in a mobile package's maps or scenes can use this data content without a network connection.
Data content can be included for the following layer types:
- Feature layer
- Vector tile layer
- Map tile layer
The data in a single mobile package can be shared across layers in multiple maps or scenes within that package. This can make mobile packages an efficient way to distribute multiple maps or scenes.
Online content
Layers in a mobile package's maps or scenes can opt to reference online services whenever a network connection is available. This is useful for including live data such as weather or traffic.
If a layer references an online service, it will only display content when there is a network connection. Data content for that layer is not included in the mobile package.
Geocoding, routing, and analysis data
Mobile packages can also contain data to support geocoding, routing, turn-by-turn directions, service area analysis, and closest facility analysis.
The typical steps to geocode using a mobile package are:
- Author an ArcGIS Pro project containing maps and/or scenes, and at least one locator.
- Create a mobile package using the appropriate ArcGIS Pro tool, including a locator. A mobile package can only include one locator, but it can be a composite locator combining multiple locators from the source ArcGIS Pro project.
- Sideload or download the mobile package to a device.
- Access the locator included in the mobile package.
The typical steps to find routes using a mobile package are:
- Author an ArcGIS Pro project containing maps and/or scenes. At least one map/scene should include at least one transportation network dataset.
- Create a mobile package using the appropriate ArcGIS Pro tool.
- Sideload or download the mobile package to a device.
- Access the map or scene in the mobile package that includes the transportation network dataset(s).
- Access a Transportation Network Dataset from the map or scene to create a Route Task.
- Use the Route Task to find a route and directions.
Package expiry
Mobile packages support an expiry date and time. This can be a hard expiry, after which ArcGIS Maps SDKs for Native Apps will not open the mobile package, or a soft expiry after which the Native will issue a warning that an offline application can present to its user.
Code examples
Display a map from a mobile map package
-
Create a Mobile Map Package referencing a
.mmpk
file on disk. -
Load the Mobile Map Package to read its contents.
-
Use one of the maps included in the mobile map package.
try
{
var mobileMapPackage = await MobileMapPackage.OpenAsync(path: "path\\to\\file.mmpk");
await mobileMapPackage.LoadAsync();
MainMapView.Map = mobileMapPackage.Maps.First();
}
catch(Exception ex)
{
// Handle error.
}
Display a scene from a mobile scene package
-
Create a Mobile Scene Package referencing a
.mspk
file on disk. -
Load the Mobile Scene Package to read its contents.
-
Use one of the scenes included in the mobile scene package.
try
{
var mobileScenePackage = await MobileScenePackage.OpenAsync(path: "path\\to\\file.mspk");
await mobileScenePackage.LoadAsync();
MainSceneView.Scene = mobileScenePackage.Scenes.First();
}
catch(Exception ex)
{
// Handle error.
}
Find an address using a mobile map package
-
Create a Mobile Map Package referencing a
.mmpk
file on disk. -
Load the Mobile Map Package to read its contents.
-
Access the Locator Task provided by the Mobile Map Package to perform a geocode.
try
{
var mobileMapPackage = await MobileMapPackage.OpenAsync(path: "\\path\\to\\file.mmpk");
await mobileMapPackage.LoadAsync();
var locatorTask = mobileMapPackage.LocatorTask;
var geocodeResult = await locatorTask.GeocodeAsync("123 Fake Street, Springfield");
if (geocodeResult.FirstOrDefault()?.DisplayLocation is MapPoint resultLocation)
{
await MainMapView.SetViewpointCenterAsync(resultLocation);
}
}
catch (Exception ex)
{
// Handle error
}
Find a route and directions using a mobile map package
-
Create a Mobile Map Package referencing a
.mmpk
file on disk. -
Load the Mobile Map Package to read its contents.
-
Access and load a map which includes a Transportation Network Dataset, and create a Route Task.
-
Access the Route Task to find a route between two points and return turn-by-turn directions.
try
{
var mobileMapPackage = await MobileMapPackage.OpenAsync(path: "\\path\\to\\file.mmpk");
await mobileMapPackage.LoadAsync();
if (mobileMapPackage.Maps.FirstOrDefault() is Map map)
{
await map.LoadAsync();
if (map.TransportationNetworks.FirstOrDefault() is TransportationNetworkDataset networkDataset)
{
var routeTask = await RouteTask.CreateAsync(networkDataset);
var startStop = new Stop(point: fromPoint);
var destinationStop = new Stop(point: toPoint);
var routeParams = await routeTask.CreateDefaultParametersAsync();
routeParams.SetStops(new[] { startStop, destinationStop });
routeParams.ReturnDirections = true;
var routeResult = await routeTask.SolveRouteAsync(routeParams);
// Display the route results in a GraphicsOverlay
}
}
}
catch (Exception ex)
{
// Handle the error
}
Tutorials
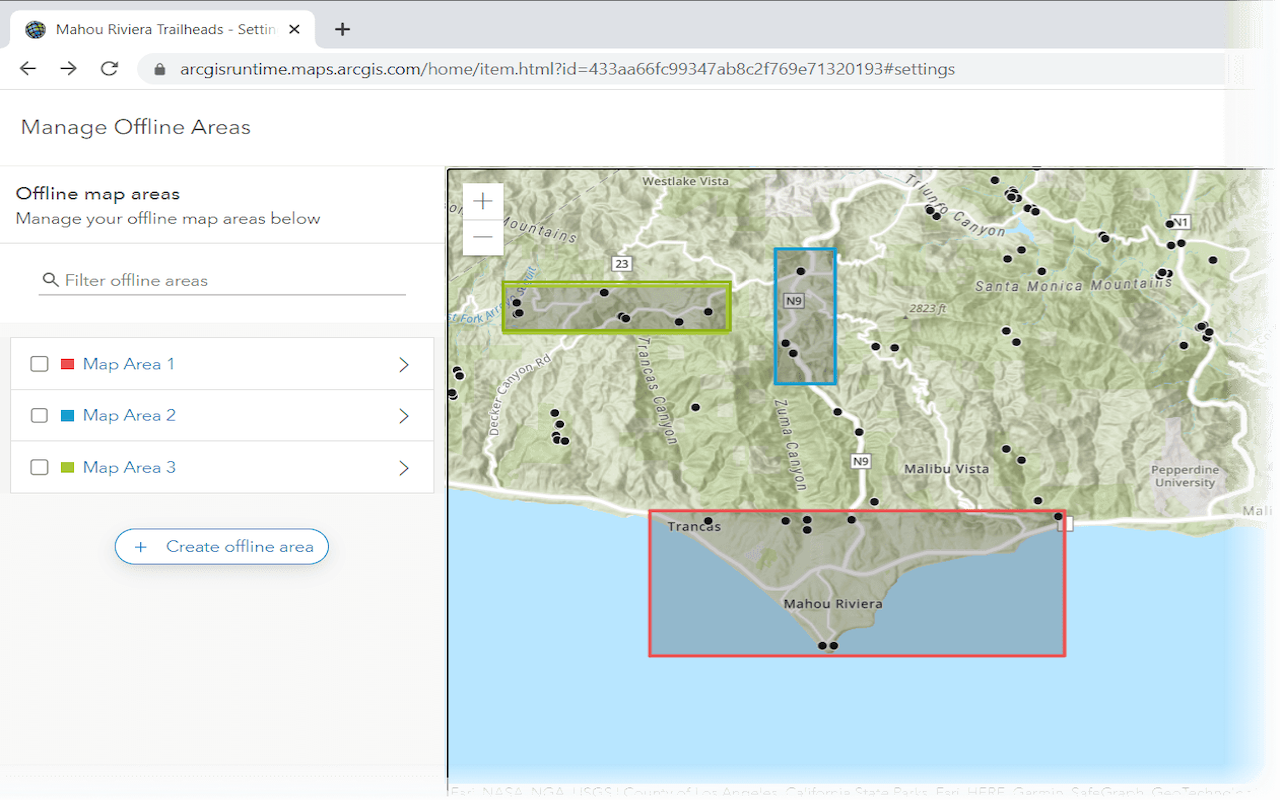
Create an offline map area
Use ArcGIS Online to create an offline map area that can be downloaded by any number of users.
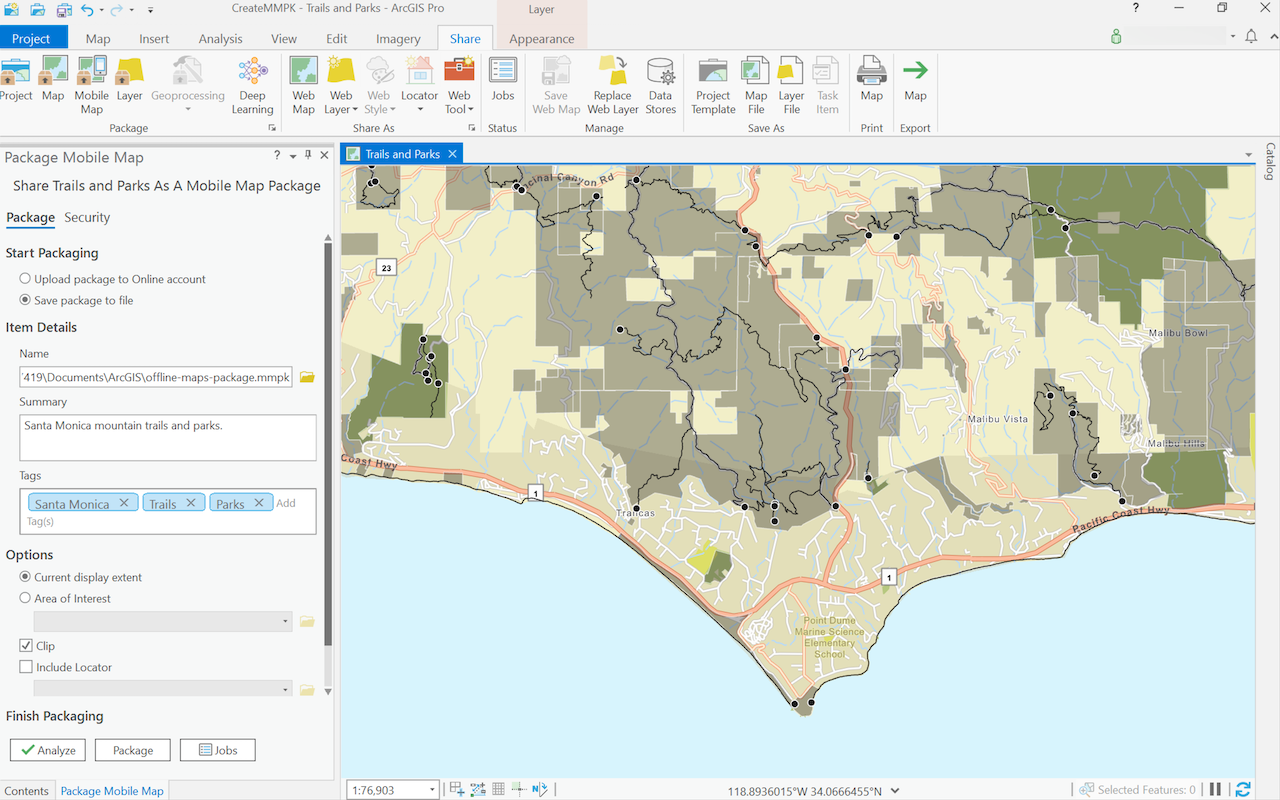
Create a mobile map package
Use ArcGIS Pro to create a mobile map package.
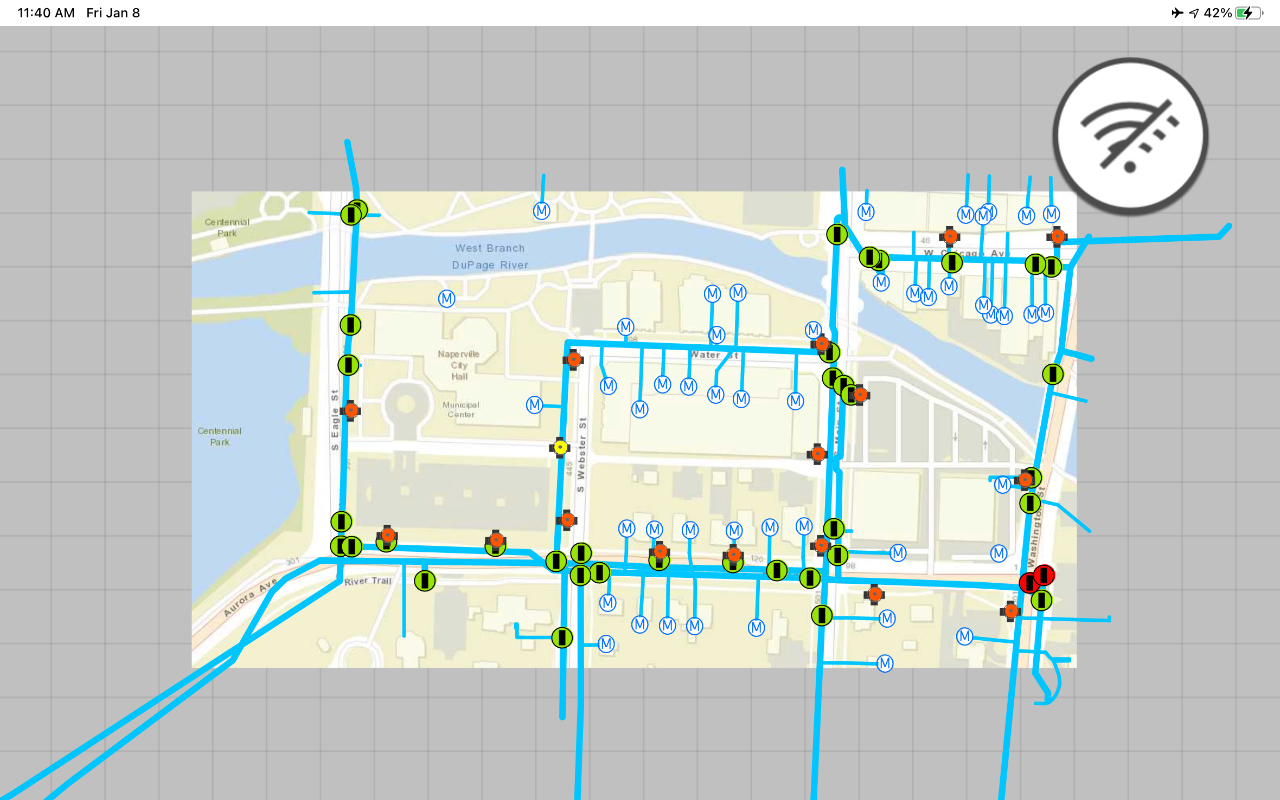
Display an offline map on demand
Download and display an offline map for a custom area of a web map stored in ArcGIS.
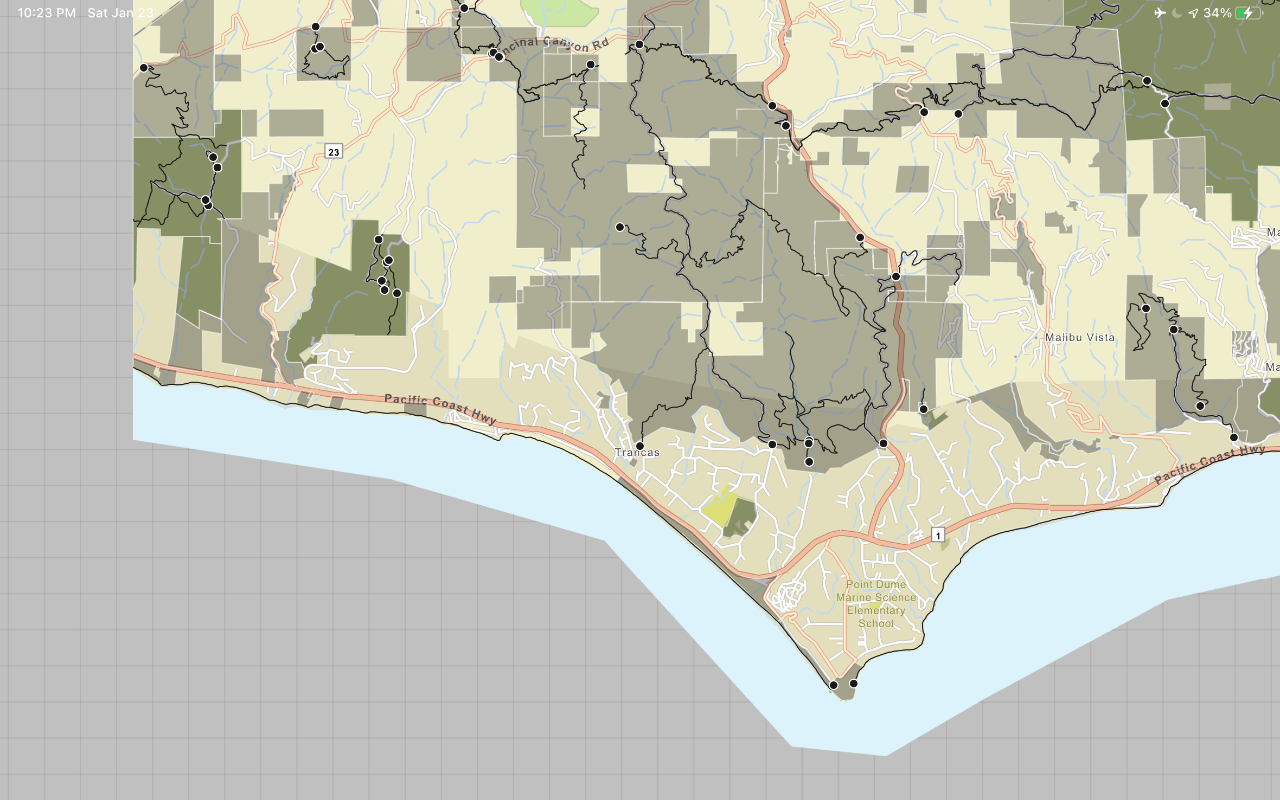
Display a map from a mobile map package
Access and display a map from a mobile map package for offline use.
API support
- 1. Geometry engine
- 2. Manage offline map areas