What is routing?
Routing, also known as simple routing, is the process of finding the best path from an origin to a destination for an individual or single vehicle. Routing takes into consideration many different data parameters in the street network such as speed limit, number of lanes, and time of day. Routing can also take into consideration other real-time data such as road conditions, accidents, and other barriers.
You can use routing to build applications that:
- Find the shortest path from an origin to a destination
- Find the quickest path to multiple destinations
- Generate driving directions in multiple languages
How routing works
The typical workflow for creating a route is to:
- Define the origin, and stops, and destination.
- Define the type of travel for the route.
- Call the service to find the route features and directions.
You can create simple or optimized routes using the routing service.
-
A simple route is the shortest path between stops.
-
An optimized route is the most efficient path between stops.
To create an optimized route, you will need to use the find
parameter. Using the parameter will result in the stops being reordered to return the most efficient path between them.
How to navigate a route
Once you have a route, if you want to use your current device's location to track progress and provide navigation instructions (voice guidance) as you traverse the route, the best way to accomplish this is with the ArcGIS Maps SDKs for Native Apps.
URL requests
You can find a route and directions by making an HTTPS request to the routing service solve
operation or by using client APIs. Specify the origin, destination, and optionally, additional parameters to refine the results with directions. Some of the most common parameters are described provided below.
Request limits
Limit | Direct | Job |
---|---|---|
Maximum number of stops | 150 | 10,000 |
Maximum transaction time: | 5 minutes | 60 minutes |
Maximum number of point barriers: | 250 | 250 |
Maximum number of street features intersected by polyline barriers: | 500 | 500 |
Maximum number of street features intersected by polygon barriers: | 2,000 | 2,000 |
Maximum straight-line distance for the walking travel mode: (If the straight-line distance between any stops is greater than this limit, the analysis will fail when the walking restriction is used.) | 27 miles (43.45 kilometers) | 27 miles (43.45 kilometers) |
Force hierarchy beyond a straight-line distance of: (If the straight-line distance between any stops is greater than the limit shown here, the analysis uses hierarchy, even if the travel_ defines not to use hierarchy.) | 50 miles (80.46 kilometers) | 50 miles (80.46 kilometers) |
Maximum snap tolerance: (If the distance between an input point and its nearest traversable street is greater than the distance specified here, the point is excluded from the analysis.) | 12.42 miles (20 kilometers) | 12.42 miles (20 kilometers) |
Maximum number of directions features that can be returned: | No limit | 1,000,000 |
Maximum number of route edges that can be returned: | Not available | 1,000,000 |
Required parameters
Name | Description | Examples |
---|---|---|
f | The format of the data returned. | f=json f=pjson f=geojson f=html |
token | An API key or OAuth 2.0 access token. Learn how to get an access token in Security and authentication. | token=< token=< |
stops | The two or more locations that need to be visited in the route. | stops=-117,34; -117.5,34.5 |
find | Specify whether the service should find the best sequence when visiting multiple destinations. Note: Set this parameter to true to generate an optimized route. | find |
Direct
Use for shorter transactions with less than 150 stops that will complete in less than 5 minutes.
https://route-api.arcgis.com/arcgis/rest/services/World/Route/NAServer/Route_World/solve?<parameters>
https://route.arcgis.com/arcgis/rest/services/World/Route/NAServer/Route_World/solve?<parameters>
Key parameters
Name (Direct) | Description | Examples |
---|---|---|
travel | The mode of transportation such as driving a car or a truck or walking. | travel JSON Object |
start | The time at which travel begins from the input stops. You can also specify a value of now , to set the depart time to the current time. | start |
return | Generate driving directions for each route. | return |
directions | The language to be used when generating driving directions. | directions |
Additional parameters: Set additional constraints for a route such as temporary slowdowns, using polygon
, or set output
to specify the type of route feature created by the service.
Job
Use for longer transactions with up to 10,000 stops that will complete in less than 60 minutes.
https://logistics.arcgis.com/arcgis/rest/services/World/Route/GPServer/FindRoutes/submitJob?<parameters>
Required parameters
Name | Description | Examples |
---|---|---|
f | The format of the data returned. | f=json f=pjson f=geojson f=html |
token | An API key or OAuth 2.0 access token. Learn how to get an access token in Security and authentication. | token=< token=< |
stops | The two or more locations that need to be visited in the route. | stops=-117,34; -117.5,34.5 |
reorder_ | Specify whether the service should find the best sequence when visiting multiple destinations. Note:Set this parameter to true to return an optimized route. | reorder_ |
Key parameters
Name (Job) | Description | Examples |
---|---|---|
travel_ | The mode of transportation such as driving a car or a truck or walking. | travel_ JSON Object |
time_ | The time at which travel begins from the input stops. | time_ |
populate_ | Generate driving directions for each route. | populate_ |
directions_ | The language to be used when generating driving directions. | directions_ |
Additional parameters: Set additional constraints such as temporary slowdowns, using polygon_
, route_
to specify the type of route feature created by the service, or set return_
if the start and end location for your route is same.
Code examples
Direct: Find a route and directions
In this example, use the routing service to find a route between a set of stops that accounts for current traffic conditions and generate driving directions in Spanish.
To find a route, you need to define at least two stops to visit. The default travel
is driving time by vehicle, but you can use walk or trucking travel modes as well. It is always good to provide the start
to get the best results. In this example, we specify start
as now
which sets the route departure time as the current time and also indicates to the service that the route should use the current traffic conditions. We also set the directions
to generate driving directions in our language of choice.
The response contains a set of ordered stops to visit, the route segments, and turn-by-turn text directions.
APIs
function getRoute() {
const routeUrl = "https://route-api.arcgis.com/arcgis/rest/services/World/Route/NAServer/Route_World";
const routeParams = new RouteParameters({
stops: new FeatureSet({
features: view.graphics.toArray()
}),
returnDirections: true,
directionsLanguage: "es"
});
route.solve(routeUrl, routeParams)
.then((data)=> {
if (data.routeResults.length > 0) {
showRoute(data.routeResults[0].route);
showDirections(data.routeResults[0].directions.features);
}
})
.catch((error)=>{
console.log(error);
})
}
REST API
curl https://route-api.arcgis.com/arcgis/rest/services/World/Route/NAServer/Route_World/solve? \
-d "f=json"
-d "token=<ACCESS_TOKEN>"
-d "stops=-122.68782,45.51238;-122.690176,45.522054;-122.614995,45.526201"
-d "startTime=now"
-d "returnDirections=true"
-d "directionsLanguage=es"
{
"routes": {
"fieldAliases": {
// one or more field aliases
},
"geometryType": "esriGeometryPolyline",
"spatialReference": {
"wkid": 4326,
"latestWkid": 4326
},
"features": [
{
"attributes": {
"Name": "Location 1 - Location 3",
"Total_TravelTime": 14.758,
"Total_Miles": 5.491,
"Total_Kilometers": 8.837
// more field values
},
"geometry": {
"paths": [
[
[
-122.68838,
45.51262
]
// additional points that make up the path geometry between location 1 and location 2
],
[
[
-122.69019,
45.52206
]
// additional points that make up the path geometry between location 2 and location 3
]
]
}
}
]
},
"directions": [
{
"routeId": 1,
"routeName": "Location 1 - Location 3",
"summary": {
"totalLength": 5.491,
"totalTime": 14.758,
"totalDriveTime": 14.758,
"envelope": {
"xmin": -122.69257,
"ymin": 45.51262,
"xmax": -122.61497,
"ymax": 45.53422,
"spatialReference": {
"wkid": 4326,
"latestWkid": 4326
}
}
},
"features": [
{
"attributes": {
"length": 0,
"time": 0,
"text": "Salga desde Location 1",
"ETA": 1607894119000,
"arriveTimeUTC": 1607922919000,
"maneuverType": "esriDMTDepart"
},
"compressedGeometry": "+gm5a9-1vvvvvh+nnn9tf+0+0",
"strings": [
{
"string": "9:15 PM",
"stringType": "esriDSTEstimatedArrivalTime"
},
{
"string": "Location 1",
"stringType": "esriDSTStreetName"
}
]
},
{
"attributes": {
"length": 0.024,
"time": 0.054,
"text": "Vaya hacia el noreste",
"ETA": 1607894119000,
"arriveTimeUTC": 1607922919000,
"maneuverType": "esriDMTStraight"
},
"compressedGeometry": "+gm5a9-1vvvvvh+nnn9tf+8a+j6+1h7+59i",
"strings": [
{
"string": "9:15 PM",
"stringType": "esriDSTEstimatedArrivalTime"
}
]
}
// more direction features
]
}
]
}
Job: Find multiple routes
In this example, you will find the travel time and travel distance for multiple routes operated by a long-haul logistics company.
-
We group a pair of stops that define a route by assigning them a unique
Route
value. This allows the service to find all the routes in a single request as compared to sending multiple requests for each route, thus improving the overall performance.Name -
We specify the Trucking Time
travel_
since we are finding routes for trucks and not cars.mode -
Since we are only interested in finding the travel time and travel distance for our routes and do not need the route geometry, we set the
route_
toshape None
. -
We also do not need driving directions for our routes. So we set the
populate_
todirections false
.
APIs
# Connect to the routing service
api_key = "YOUR_API_KEY"
arcgis.GIS("https://www.arcgis.com", api_key=api_key)
# Get the trucking time travel mode defined for the portal. Fail if the travel mode is not found.
truck_time_travel_mode = ""
for feature in arcgis.network.analysis.get_travel_modes().supported_travel_modes.features:
attributes = feature.attributes
if attributes["AltName"] == "Trucking Time":
truck_time_travel_mode = attributes["TravelMode"]
break
assert truck_time_travel_mode, "Trucking Time travel mode not found"
# Call the routing service
result = arcgis.network.analysis.find_routes(stops=stops,
travel_mode=truck_time_travel_mode,
populate_directions=False,
route_shape="None")
print_result(result)
REST API
Unlike Direct request type which allows you to make a request and get back all the results in the response, when working with a Job request type, you need to follow a three step workflow:
- Make the
submit
request with the appropriate request parameters to get a job id.Job - Using the job id, check the job status to see if the job completed successfully.
- Use the job id to get one or more results.
curl https://logistics.arcgis.com/arcgis/rest/services/World/Route/GPServer/FindRoutes/submitJob? \
-d "f=json" \
-d "token=<ACCESS_TOKEN>" \
-d "stops={'features':[{'geometry':{'x':-118.268579,'y':34.052291},'attributes':{'Name':'Los Angeles, CA','RouteName':'Route A'}},{'geometry':{'x':-74.009023,'y':40.709975},'attributes':{'Name':'New York, NY','RouteName':'Route A'}},{'geometry':{'x':-87.648549,'y':41.758688},'attributes':{'Name':'Chicago, IL','RouteName':'Route B'}},{'geometry':{'x':-122.320028,'y':47.591046},'attributes':{'Name':'Seattle, WA','RouteName':'Route B'}},{'geometry':{'x':-71.057477,'y':42.359483},'attributes':{'Name':'Boston, MA','RouteName':'Route C'}},{'geometry':{'x':-95.368848,'y':29.759222},'attributes':{'Name':'Houston, TX','RouteName':'Route C'}},{'geometry':{'x':-80.220762,'y':25.761156},'attributes':{'Name':'Miami, FL','RouteName':'Route D'}},{'geometry':{'x':-122.651334,'y':45.51567},'attributes':{'Name':'Portland, OR','RouteName':'Route D'}},{'geometry':{'x':-112.074247,'y':33.446554},'attributes':{'Name':'Phoenix, AZ','RouteName':'Route E'}},{'geometry':{'x':-75.161557,'y':39.952057},'attributes':{'Name':'Philadelphia, PA','RouteName':'Route E'}}]}" \
-d "travel_mode={'attributeParameterValues':[{'attributeName':'Use Preferred Truck Routes','parameterName':'Restriction Usage','value':'PREFER_HIGH'},{'attributeName':'Avoid Unpaved Roads','parameterName':'Restriction Usage','value':'AVOID_HIGH'},{'attributeName':'Avoid Private Roads','parameterName':'Restriction Usage','value':'AVOID_MEDIUM'},{'attributeName':'Driving a Truck','parameterName':'Restriction Usage','value':'PROHIBITED'},{'attributeName':'Roads Under Construction Prohibited','parameterName':'Restriction Usage','value':'PROHIBITED'},{'attributeName':'Avoid Gates','parameterName':'Restriction Usage','value':'AVOID_MEDIUM'},{'attributeName':'Avoid Express Lanes','parameterName':'Restriction Usage','value':'PROHIBITED'},{'attributeName':'Avoid Carpool Roads','parameterName':'Restriction Usage','value':'PROHIBITED'},{'attributeName':'Avoid Truck Restricted Roads','parameterName':'Restriction Usage','value':'AVOID_HIGH'},{'attributeName':'TruckTravelTime','parameterName':'Vehicle Maximum Speed (km/h)','value':0}],'description':'Models basic truck travel by preferring designated truck routes, and finds solutions that optimize travel time. Routes must obey one-way roads, avoid illegal turns, and so on. When you specify a start time, dynamic travel speeds based on traffic are used where it is available, up to the legal truck speed limit.','distanceAttributeName':'Kilometers','id':'ZzzRtYcPLjXFBKwr','impedanceAttributeName':'TruckTravelTime','name':'Trucking Time','restrictionAttributeNames':['Avoid Carpool Roads','Avoid Express Lanes','Avoid Gates','Avoid Private Roads','Avoid Truck Restricted Roads','Avoid Unpaved Roads','Driving a Truck','Roads Under Construction Prohibited','Use Preferred Truck Routes'],'simplificationTolerance':10,'simplificationToleranceUnits':'esriMeters','timeAttributeName':'TruckTravelTime','type':'TRUCK','useHierarchy':true,'uturnAtJunctions':'esriNFSBNoBacktrack'}" \
-d "populate_directions=false" \
-d "route_shape=None" \
Response (JSON)
{
"jobId": "j2b41de060e5d42d082b888d3be029253",
"jobStatus": "esriJobSubmitted"
}
Request
curl https://logistics.arcgis.com/arcgis/rest/services/World/Route/GPServer/FindRoutes/jobs/j2b41de060e5d42d082b888d3be029253? \
-d "f=json" \
-d "token=<ACCESS_TOKEN>"
Response (JSON)
{
"jobId": "j2b41de060e5d42d082b888d3be029253",
"jobStatus": "esriJobSucceeded",
"results": {
"Solve_Succeeded": {
"paramUrl": "results/Solve_Succeeded"
},
"Output_Routes": {
"paramUrl": "results/Output_Routes"
},
"Output_Route_Edges": {
"paramUrl": "results/Output_Route_Edges"
},
"Output_Directions": {
"paramUrl": "results/Output_Directions"
},
"Output_Stops": {
"paramUrl": "results/Output_Stops"
},
"Output_Network_Analysis_Layer": {
Request
curl https://logistics.arcgis.com/arcgis/rest/services/World/Route/GPServer/FindRoutes/jobs/j2b41de060e5d42d082b888d3be029253/results/Output_Routes? \
-d "f=json" \
-d "token=<ACCESS_TOKEN>"
Response (JSON)
{
"paramName": "Output_Routes",
"value": {
"fields": [
// One or more field definitions
],
"features": [
{
"attributes": {
"Name": "Route A",
"Total_Miles": 2802.1727702808216,
"Total_Kilometers": 4509.6689541527276,
"Total_Minutes": 2569.5486409677055
// more field values
}
},
{
"attributes": {
"Name": "Route B",
"Total_Miles": 2123.5459789541665,
"Total_Kilometers": 3417.5228149996442,
"Total_Minutes": 1942.2562740678086
// more field values
}
},
{
"attributes": {
"Name": "Route C",
"Total_Miles": 1858.4600032557835,
"Total_Kilometers": 2990.9074372945502,
"Total_Minutes": 1741.9289972140964
// more field values
}
},
{
"attributes": {
"Name": "Route D",
"Total_Miles": 3270.1929177872121,
"Total_Kilometers": 5262.8758768350972,
"Total_Minutes": 2975.6605207300813
// more field values
}
},
{
"attributes": {
"Name": "Route E",
"Total_Miles": 2392.4891536441355,
"Total_Kilometers": 3850.3457651737981,
"Total_Minutes": 2128.3869138049135
// more field values
}
}
],
"exceededTransferLimit": false
}
}
Tutorials
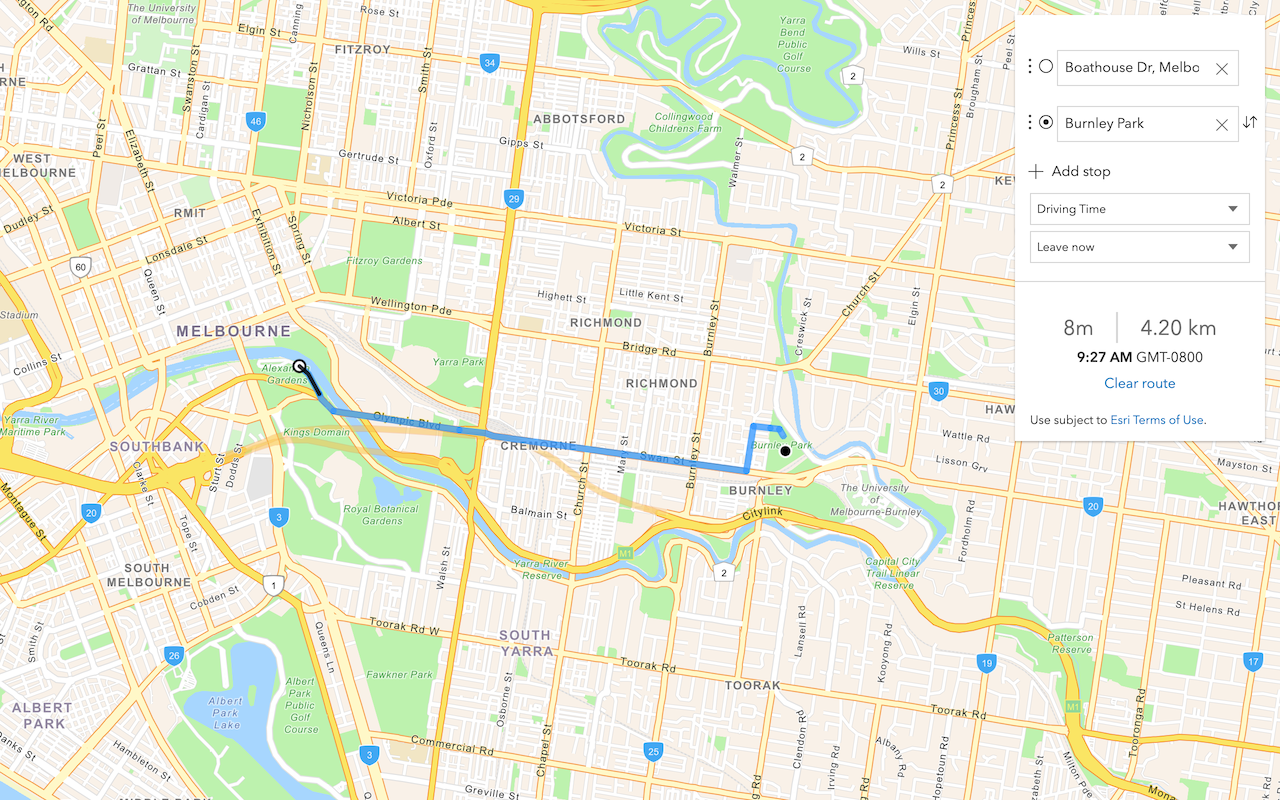
Find a route and directions
Find a route and directions with the routing service.
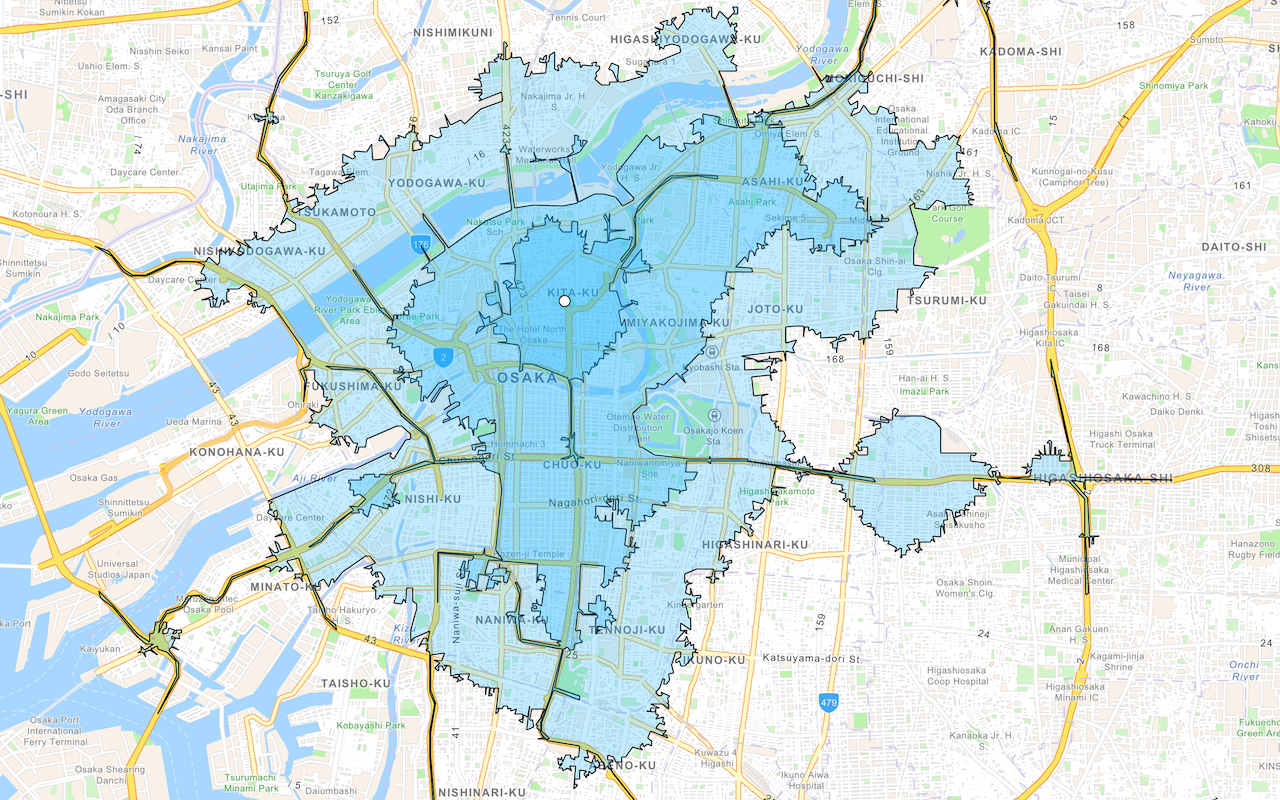
Find service areas
Create an isochrone with driving distance with the routing service.
Services
Routing service
Get turn-by-turn directions and solve advanced routing problems.
API support
Routing | Optimized Routing | Fleet routing | Closest facility routing | Service areas | Location-allocation | Travel cost matrix | |
---|---|---|---|---|---|---|---|
ArcGIS Maps SDK for JavaScript | 1 | 1 | 1 | ||||
ArcGIS Maps SDK for .NET | 1 | 1 | 1 | ||||
ArcGIS Maps SDK for Kotlin | 1 | 1 | 1 | ||||
ArcGIS Maps SDK for Swift | 1 | 1 | 1 | ||||
ArcGIS Maps SDK for Java | 1 | 1 | 1 | ||||
ArcGIS Maps SDK for Qt | 1 | 1 | 1 | ||||
ArcGIS API for Python | |||||||
ArcGIS REST JS | |||||||
Esri Leaflet | 2 | 2 | 2 | 2 | 2 | 2 | 2 |
MapLibre GL JS | 2 | 2 | 2 | 2 | 2 | 2 | 2 |
OpenLayers | 2 | 2 | 2 | 2 | 2 | 2 | 2 |
- 1. Access with geoprocessing task.
- 2. Access via ArcGIS REST JS.
Tools
Developer dashboard
Manage API keys, service usage, and data with the ArcGIS Developers website.
ArcGIS.com
Create, manage, and share content and data with GIS tools.
Map Viewer
Create, explore, and share web maps for 2D applications.
Scene Viewer
Create, explore, and share web scenes for 3D applications.
ArcGIS Pro
Explore, visualize, and analyze both 2D and 3D data with desktop GIS tools.