What is a service area?
A service area, also known as an isochrone, is a polygon that represents the distance that can be reached when driving or walking on a street network. This type of analysis is common in real estate search or determining the driving proximity to schools, businesses, or other facilities. For example, you can create a drive time polygon that represents how far you can drive in any direction from the center of a city in 20 minutes.
You can use service areas to build applications that:
-
Visualize and measure the accessibility of locations that provide some kind of service. For example, a three-minute drive-time polygon around a grocery store can determine which residents are able to reach the store within three minutes and are thus more likely to shop there.
-
By generating multiple service areas around one or more locations that can show how accessibility changes with an increase in travel time or travel distance. It can be used, for example, to determine how many hospitals are within 5, 10, and 15 minute drive times of schools.
-
When creating service areas based on travel times, the service can make use of traffic data, which can influence the area that can be reached during different times of the day.
How service areas work
The typical workflow for creating service areas is to define:
- One or more locations around which service areas are generated (facilities).
- The size and number of service areas to generate for each facility.
- Define the type of travel for the analysis.
- Call the service to generate service areas around facilities.
URL requests
You can generate service areas by making an HTTPS request to the Service Area service solve
operation or by using client APIs. Specify the one or more input facilities, and optionally, additional parameters to refine the operation. Some of the most common parameters are described provided below.
Request limits
Limit | Direct | Job |
---|---|---|
Maximum number of facilities: | 100 | 1,000 |
Maximum transaction time: | 5 minutes | 2 hours |
Maximum number of breaks: | No limit | No limit |
Maximum travel time: | 9 hours when walking 5 hours for all other travel modes | 9 hours when walking 5 hours for all other travel modes |
Maximum travel distance: | 27 miles (43.45 kilometers) when walking 300 miles (482.80 kilometers) for all other travel modes | 27 miles (43.45 kilometers) when walking 300 miles (482.80 kilometers) for all other travel modes |
Maximum travel time when generating detailed polygons: | 5 hours when walking 15 minutes for all other travel modes | 5 hours when walking 15 minutes for all other travel modes |
Maximum travel distance when generating detailed polygons: | 15 miles (24.14 kilometers) for all travel modes | 15 miles (24.14 kilometers) for all travel modes |
Maximum travel time when generating service area lines: | 5 hours when walking 15 minutes for all other travel modes | 5 hours when walking 15 minutes for all other travel modes |
Maximum travel distance when generating service area lines: | 15 miles (24.14 kilometers) for all travel modes | 15 miles (24.14 kilometers) for all travel modes |
Maximum number of point barriers: | 250 | 250 |
Maximum number of street features intersected by polyline barriers: | 500 | 500 |
Maximum number of street features intersected by polygon barriers: | 2,000 | 2,000 |
Force hierarchy beyond a travel time of: (If you specify a drive time that exceeds the limit shown here, the analysis uses the hierarchy even if the travel mode defines not to use hierarchy) | 60 minutes The 60-minute limit applies when generating polygons with standard level of detail. ( output ). When walking, the analysis does not force hierarchy even when this limit is exceeded and always returns polygons with standard level of detail. | 4 hours The 4-hour limit applies when generating polygons with standard level of detail. ( polygon_ ). When walking, the analysis does not force hierarchy even when this limit is exceeded and always returns polygons with standard level of detail. |
Force hierarchy beyond a travel distance of: (If you specify a drive distance that exceeds the limit shown here, the analysis uses the hierarchy even if the travel mode defines not to use hierarchy) | 60 miles (96.56 kilometers) The 60-mile limit applies when generating polygons with standard level of detail. ( output ). When walking, the analysis does not force hierarchy even when this limit is exceeded and always returns polygons with standard level of detail. | 240 miles (386.24 kilometers) The 240-mile limit applies when generating polygons with standard level of detail. ( polygon_ ). When walking, the analysis does not force hierarchy even when this limit is exceeded and always returns polygons with standard level of detail. |
Maximum polygon trim distance: | 500 meters | 500 meters |
Maximum snap tolerance: (If the distance between an input point and its nearest traversable street is greater than the distance specified here, the point is excluded from the analysis.) | 12.42 miles (20 kilometers) | 12.42 miles (20 kilometers) |
Maximum number of polygon features that can be returned by the service: | No limit | 10,000 |
Required parameters
Name | Description | Examples |
---|---|---|
f | The format of the data returned. | f=json f=pjson |
token | An API key or OAuth 2.0 access token. Learn how to get an access token in Security and authentication. | token=< token=< |
Direct
Use for shorter transactions with less than 100 facilities that will complete in less than 5 minutes.
https://route-api.arcgis.com/arcgis/rest/services/World/ServiceAreas/NAServer/ServiceArea_World/solveServiceArea?<parameters>
https://route.arcgis.com/arcgis/rest/services/World/ServiceAreas/NAServer/ServiceArea_World/solveServiceArea?<parameters>
Key parameters
Name (Direct) | Description | Examples |
---|---|---|
facilities | One or more locations around which service areas are generated. | facilities=-117,34 |
default | The size and number of service areas to generate for each facility. | default |
travel | The mode of transportation such as driving a car or a truck or walking. | travel JSON Object |
travel | The direction of travel (away from facility or towards facility) that should be used when generating service areas. | travel |
time | The time to depart from or arrive at facilities. You can also specify a value of now , to set the depart or arrive time to current time. | time |
Additional parameters: Exercise fine-grained control on how the service area polygons are generated by specifying output
, split
, overlap
, and merge
parameters.
Job
Use for longer transactions with up to 1,000 facilities that will complete in less than 2 hours.
https://logistics.arcgis.com/arcgis/rest/services/World/ServiceAreas/GPServer/GenerateServiceAreas/submitJob?<parameters>
Key parameters
Name (Job) | Description | Examples |
---|---|---|
facilities | One or more locations around which service areas are generated. | facilities={"features":[{"geometry":{"x":-117,"y":34.5}}]} |
break_ | The size and number of service areas to generate for each facility. | break_ |
travel_ | The mode of transportation such as driving a car or a truck or walking. | travel_ JSON Object |
travel_ | The direction of travel (away from facility or towards facility) that should be used when generating service areas. | travel_ |
time_ | The time to depart from or arrive at facilities. | time_ |
Additional parameters: Exercise fine-grained control on how the service area polygons are generated by specifying polygon_
, polygon_
, and polygons_
parameters.
Code examples
Direct: Find walk distance areas
In this example, use the Service area service to find an area that is within 2.5 kilometers (1.55 miles) of walking distance from an elementary school. Such an area can be used to determine which students are eligible for the school bus service as the students that live within 1.5 miles of walking distance from the school are not eligible.
To find service areas, you need to define one or more facilities around which service areas are generated. The default travel
is driving time, so in this case the travel mode is set to walking distance. The default
are set to 2.5 which is interpreted by the service to be in kilometers since the distance unit of the travel mode's impedance attribute is kilometers. The travel direction is set to esri
to calculate the walk area as students walk towards the school.
The response contains a polygon of the walk area with 1.55 miles that does not qualify for bus transportation.
APIs
async function findServiceArea(locationFeature) {
if (!travelMode) {
const networkDescription = await networkService.fetchServiceDescription(url);
travelMode = networkDescription.supportedTravelModes.find(
(travelMode) => travelMode.name === "Walking Distance"
);
}
const serviceAreaParameters = new ServiceAreaParameters({
facilities: new FeatureSet({
features: [locationFeature]
}),
defaultBreaks: [2.5], // km
travelMode,
travelDirection: "to-facility",
outSpatialReference: view.spatialReference,
trimOuterPolygon: true
});
const { serviceAreaPolygons } = await serviceArea.solve(url, serviceAreaParameters);
showServiceAreas(serviceAreaPolygons);
}
REST API
curl https://route-api.arcgis.com/arcgis/rest/services/World/ServiceAreas/NAServer/ServiceArea_World/solveServiceArea? \
-d "f=json" \
-d "token=<ACCESS_TOKEN>" \
-d "facilities=-117.133163,34.022445" \
-d "defaultBreaks=2.5" \
-d "travelDirection=esriNATravelDirectionToFacility" \
-d "travelMode={'attributeParameterValues':[{'attributeName':'Avoid Private Roads','parameterName':'Restriction Usage','value':'AVOID_MEDIUM'},{'attributeName':'Walking','parameterName':'Restriction Usage','value':'PROHIBITED'},{'attributeName':'Preferred for Pedestrians','parameterName':'Restriction Usage','value':'PREFER_LOW'},{'attributeName':'WalkTime','parameterName':'Walking Speed (km/h)','value':5},{'attributeName':'Avoid Roads Unsuitable for Pedestrians','parameterName':'Restriction Usage','value':'AVOID_HIGH'}],'description':'Follows paths and roads that allow pedestrian traffic and finds solutions that optimize travel distance.','distanceAttributeName':'Kilometers','id':'yFuMFwIYblqKEefX','impedanceAttributeName':'Kilometers','name':'Walking Distance','restrictionAttributeNames':['Avoid Private Roads','Avoid Roads Unsuitable for Pedestrians','Preferred for Pedestrians','Walking'],'simplificationTolerance':2,'simplificationToleranceUnits':'esriMeters','timeAttributeName':'WalkTime','type':'WALK','useHierarchy':false,'uturnAtJunctions':'esriNFSBAllowBacktrack'}"
{
"messages": [],
"saPolygons": {
"fieldAliases": {
"Name": "Name"
// more field aliases
},
"geometryType": "esriGeometryPolygon",
"spatialReference": {
"wkid": 4326,
"latestWkid": 4326
},
"features": [
{
"attributes": {
"Name": "Location 1 : 0 - 2.5",
"FromBreak": 0,
"ToBreak": 2.5
// more field values
},
"geometry": {
"rings": [
[
[
-117.13887868499995,
34.04079974800004
],
[
-117.13820495099998,
34.040350593000028
]
// additional points that make up the ring for the polygon
]
]
}
}
]
}
}
Job: Find drive-time areas
In this example, you will find the 5, 10, and 15 minute drive-time polygons around all locations of a grocery store chain in a city. The chain wants to use these drive-time areas to see how many customers can easily drive to their stores and thus are more likely to shop there.
The example illustrates how to measure the time it takes potential customers to reach each store. To do so, the travel_
is set to Towards Facility
. Attributes for each store are also provided such as the Store
, Store
, and Address
. These will be appended to the drive-time areas to correlate the output drive-time area with the store.
APIs
# Connect to the Service area service
api_key = "YOUR_API_KEY"
arcgis.GIS("https://www.arcgis.com", api_key=api_key)
# Call the Service Area service
result = arcgis.network.analysis.generate_service_areas(facilities=facilities,
break_values="5 10 15",
travel_direction="Towards Facility")
print_result(result)
REST API
Unlike Direct request type which allows you to make a request and get back all the results in the response, when working with a Job request type, you need to follow a three step workflow:
- Make the
submit
request with the appropriate request parameters to get a job id.Job - Using the job id, check the job status to see if the job completed successfully.
- Use the job id to get one or more results.
curl https://logistics.arcgis.com/arcgis/rest/services/World/ServiceAreas/GPServer/GenerateServiceAreas/submitJob? \
-d "f=json" \
-d "token=<ACCESS_TOKEN>" \
-d "facilities={'displayFieldName':'','fieldAliases':{'StoreName':'Store Name','Address':'Address','StoreId':'Store ID'},'geometryType':'esriGeometryPoint','spatialReference':{'wkid':4326,'latestWkid':4326},'fields':[{'name':'StoreName','type':'esriFieldTypeString','alias':'Name','length':50},{'name':'Address','type':'esriFieldTypeString','alias':'Name','length':256},{'name':'StoreId','type':'esriFieldTypeString','alias':'Store ID','length':16}],'features':[{'attributes':{'StoreName':'Store 1','Address':'1775 E Lugonia Ave, Redlands, CA 92374','StoreId':'120'},'geometry':{'x':-117.14002999994386,'y':34.071219999994128}},{'attributes':{'StoreName':'Store 2','Address':'1536 Barton Rd, Redlands, CA 92373','StoreId':'130'},'geometry':{'x':-117.207329999671,'y':34.047980000203609}},{'attributes':{'StoreName':'Store 3','Address':'11 E Colton Ave, Redlands, CA 92374','StoreId':'121'},'geometry':{'x':-117.18194000041973,'y':34.06351999976232}}]}" \
-d "break_values=5 10 15" \
-d "travel_direction='Towards Facility'"
Response (JSON)
{
"jobId": "jdb99eec215ab41a9961a2563d7933956",
"jobStatus": "esriJobSubmitted"
}
Request
curl https://logistics.arcgis.com/arcgis/rest/services/World/ServiceAreas/GPServer/GenerateServiceAreas/jobs/jdb99eec215ab41a9961a2563d7933956? \
-d "f=json" \
-d "token=<ACCESS_TOKEN>"
Response (JSON)
{
"jobId": "jdb99eec215ab41a9961a2563d7933956",
"jobStatus": "esriJobSucceeded",
"results": {
"Service_Areas": {
"paramUrl": "results/Service_Areas"
},
"Solve_Succeeded": {
"paramUrl": "results/Solve_Succeeded"
},
"Output_Network_Analysis_Layer": {
"paramUrl": "results/Output_Network_Analysis_Layer"
},
"Output_Facilities": {
"paramUrl": "results/Output_Facilities"
},
"Output_Service_Area_Lines": {
"paramUrl": "results/Output_Service_Area_Lines"
},
"Output_Result_File": {
Request
curl https://logistics.arcgis.com/arcgis/rest/services/World/ServiceAreas/GPServer/GenerateServiceAreas/jobs/jdb99eec215ab41a9961a2563d7933956/results/Service_Areas? \
-d "f=json" \
-d "token=<ACCESS_TOKEN>"
Response (JSON)
{
"paramName": "Service_Areas",
"value": {
"geometryType": "esriGeometryPolygon",
"spatialReference": {
"wkid": 4326,
"latestWkid": 4326
},
"fields": [
// one or more field definitions
],
"features": [
{
"attributes": {
"FromBreak": 10,
"ToBreak": 15,
"StoreName": "Store 2",
"Address": "1536 Barton Rd, Redlands, CA 92373",
"StoreId": "130"
//more field values
},
"geometry": {
"rings": [
[
[
-117.18199762099999,
34.170830291000073
]
// additional points that make up the ring for the polygon
],
[
[
-117.15662033099994,
33.956358498000043
]
// additional points that make up the ring for the polygon
]
// additional rings that make up the polygon
]
}
},
{
"attributes": {
"FromBreak": 5,
"ToBreak": 10,
"StoreName": "Store 2",
"Address": "1536 Barton Rd, Redlands, CA 92373",
"StoreId": "130"
// more field values
},
"geometry": {
"rings": [
// one or more rings that make up the polygon
]
}
},
{
"attributes": {
"FromBreak": 0,
"ToBreak": 5,
"StoreName": "Store 2",
"Address": "1536 Barton Rd, Redlands, CA 92373",
"StoreId": "130"
// more field values
},
Tutorials
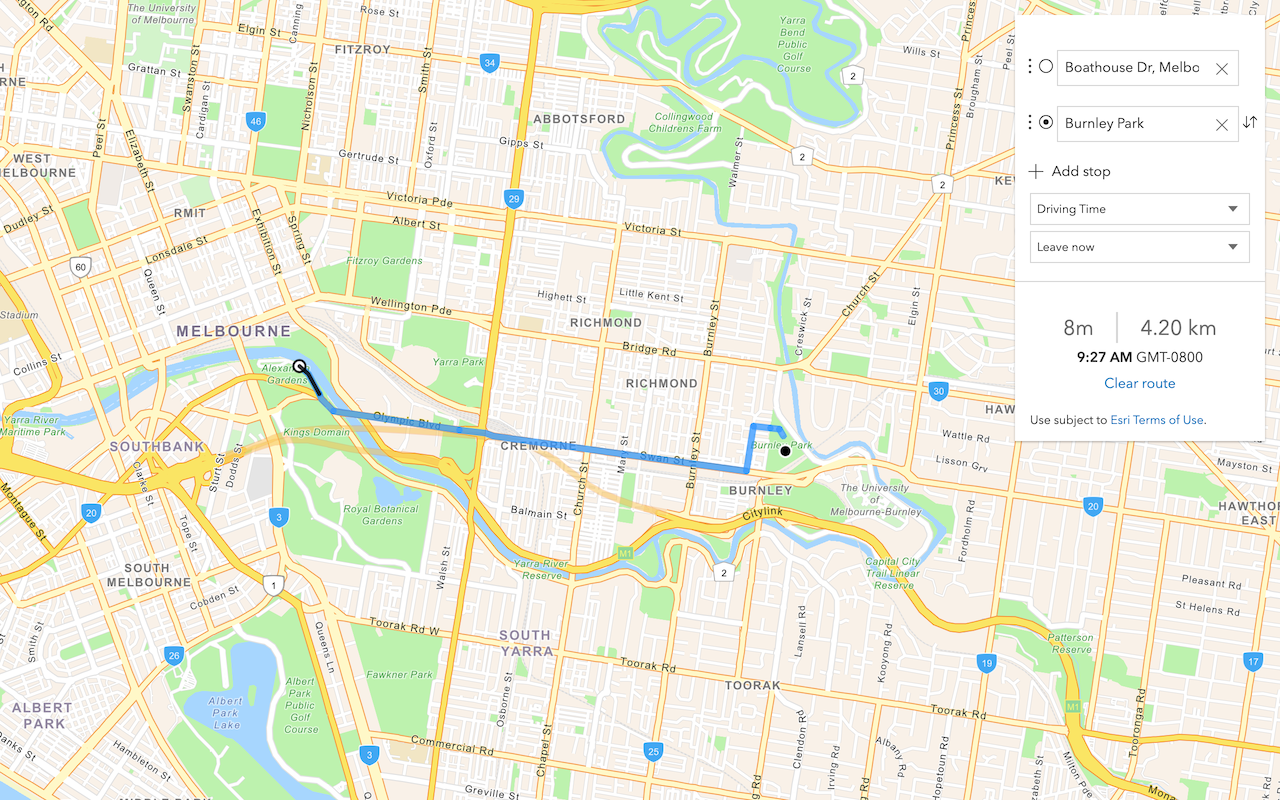
Find a route and directions
Find a route and directions with the routing service.
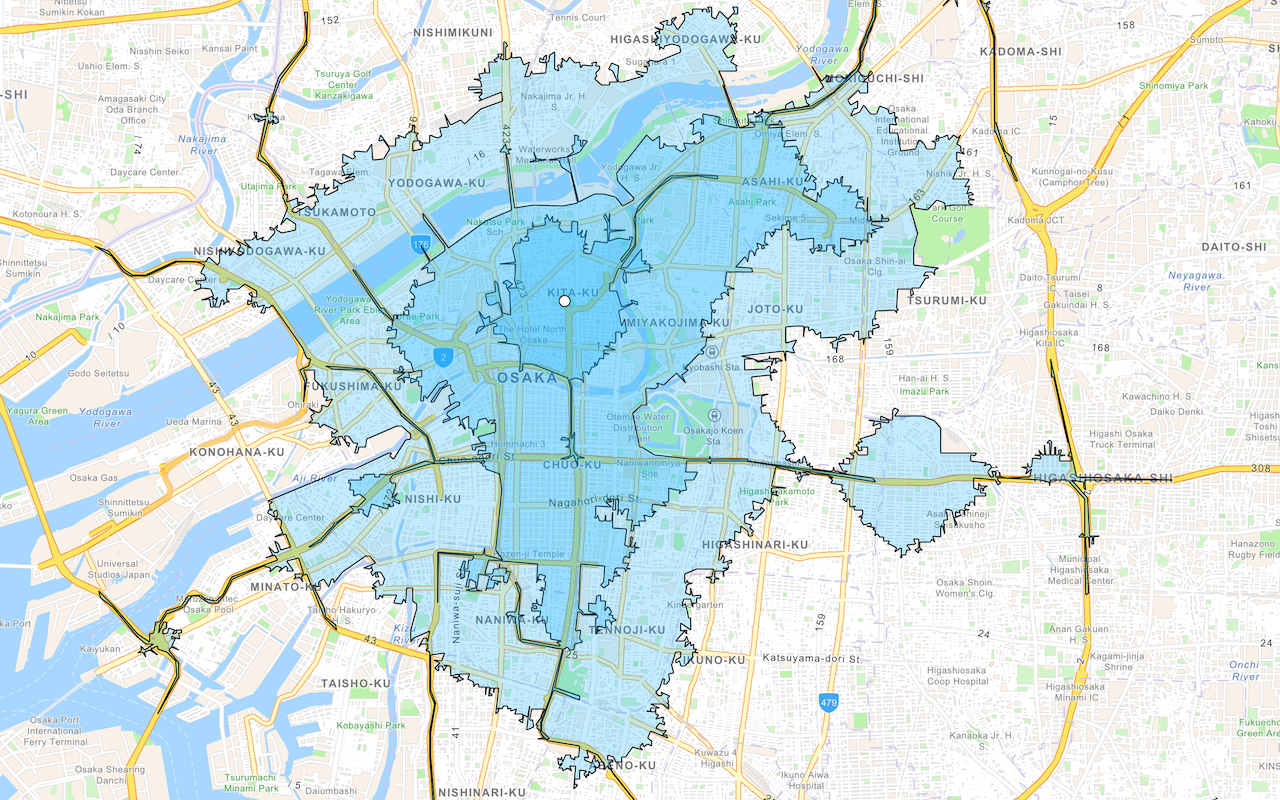
Find service areas
Create an isochrone with driving distance with the routing service.
Services
Routing service
Get turn-by-turn directions and solve advanced routing problems.
API support
Routing | Optimized Routing | Fleet routing | Closest facility routing | Service areas | Location-allocation | Travel cost matrix | |
---|---|---|---|---|---|---|---|
ArcGIS Maps SDK for JavaScript | 1 | 1 | 1 | ||||
ArcGIS Maps SDK for .NET | 1 | 1 | 1 | ||||
ArcGIS Maps SDK for Kotlin | 1 | 1 | 1 | ||||
ArcGIS Maps SDK for Swift | 1 | 1 | 1 | ||||
ArcGIS Maps SDK for Java | 1 | 1 | 1 | ||||
ArcGIS Maps SDK for Qt | 1 | 1 | 1 | ||||
ArcGIS API for Python | |||||||
ArcGIS REST JS | |||||||
Esri Leaflet | 2 | 2 | 2 | 2 | 2 | 2 | 2 |
MapLibre GL JS | 2 | 2 | 2 | 2 | 2 | 2 | 2 |
OpenLayers | 2 | 2 | 2 | 2 | 2 | 2 | 2 |
- 1. Access with geoprocessing task.
- 2. Access via ArcGIS REST JS.
Tools
Developer dashboard
Manage API keys, service usage, and data with the ArcGIS Developers website.
ArcGIS.com
Create, manage, and share content and data with GIS tools.
Map Viewer
Create, explore, and share web maps for 2D applications.
Scene Viewer
Create, explore, and share web scenes for 3D applications.
ArcGIS Pro
Explore, visualize, and analyze both 2D and 3D data with desktop GIS tools.