What is an interpolation analysis?
An interpolation analysis is the process of estimating and predicting the location of new features and attribute values by using point features with numeric fields. To execute the analysis, use the spatial analysis service and the Interpolate
.
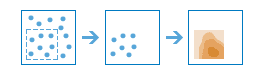
Real-world examples of this analysis include the following:
- Interpolating air quality, soil nutrients, and rainfall levels.
- Predicting heavy metal concentrations.
- Predicting earthquake magnitudes.
How to perform an interpolation analysis
The general steps to performing an interpolation analysis are as follows:
- Review the parameters for the
Interpolate
operation.Points - Send a request to get the spatial analysis service URL.
- Execute a job request with the following URL and parameters:
- URL:
https:
//< YOUR_ ANALYSIS_ SERVICE>/arcgis/rest/services/tasks/GPServer/Interpolate Points/submit Job - Parameters:
input
: Your points dataset as a hosted feature layer or feature collection.Layer field
: The numeric field containing the values to interpolate.output
: A string representing the name of the hosted feature layer to reutrn with the results.Name
- URL:
- Check the status.
- Get the output layer results.
To see examples using ArcGIS API for Python, ArcGIS REST JS, and the ArcGIS REST API, go to Examples below.
URL request
https://analysis3.arcgis.com/arcgis/rest/services/tasks/GPServer/CalculateDensity/submitJob?<parameters>
Required parameters
Name | Description | Examples |
---|---|---|
f | The format of the data returned. | f=json f=pjson |
token | An OAuth 2.0 access token. Learn how to get an access token in Security and authentication. | token=< |
input | The point feature collection or hosted feature layer. | {url: |
field | The numeric field containing the values to interpolate. | Elevation |
Key parameters
Name | Description | Examples |
---|---|---|
interpolate | A value declaring the preference for speed versus accuracy. The default is 5 . | 1 , 5 , 9 |
classification | Determines how the predicted values will be classified into areas. The default is Geometric . | Equal , Equal , Geometric , Manual |
class | If the classification is Manual , then provide the class break values as a list. | [1,3,5] |
bounding | The layer specifying the polygons in which you want values to be interpolated. | {url: |
output | A string representing the name of the hosted feature layer to return with the results. NOTE: If you do not include this parameter, the results are returned as a feature collection (JSON). | {"service |
context | A bounding box or output spatial reference for the analysis. | "extent":{"xmin: |
Code examples
Predict air quality
This example uses the Interpolate
operation to predict the air quality index (AQI) based on particle values that are 10 micrometers or smaller.
In the analysis, the input
value is the AQI PM10 hosted feature layer. The DAILY_
is the field
used to interpolate values.
APIs
inputLayer = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/aqi_pm10/FeatureServer/0"
aoiLayer = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/California_State_Boundary/FeatureServer/0"
results = interpolate_points(
input_layer=inputLayer,
field="DAILY_AQI_VALUE",
interpolate_option=5,
classification_type="GeometricInterval",
num_classes=10,
bounding_polygon_layer=aoiLayer,
output_prediction_error=False,
#Outputs results as a hosted feature layer.
output_name="Interpolate points result",
)
result_features = results["result_layer"].query()
print(
f"The interpolate points layer has {len(result_features.features)} new records"
)
Service requests
Request
POST arcgis.com/sharing/rest/portals/self HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"helperServices": {
// Other parameters
"analysis": {
"url": "https://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer"
},
"geoenrichment": {
"url": "https://geoenrich.arcgis.com/arcgis/rest/services/World/GeoenrichmentServer"
}
}
}
Request
POST <YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/InterpolatePoints/submitJob HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
&inputLayer={"url":"https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/aqi_pm10/FeatureServer/0"}
&field=DAILY_AQI_VALUE
&interpolateOption=5
&classificationType=GeometricInterval
&numClasses=10'\
&boundingPolygonLayer={{"url":"https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/California_State_Boundary/FeatureServer/0"}}'
&outputPredictionError=false
&returnFeatureCollection=false
&outputName={"serviceProperties":{"name":"Interpolate points results"}}
&context={}
Response (JSON)
{
"jobId": "<JOB_ID>",
"jobStatus": "esriJobSubmitted",
"results": {},
"inputs": {},
"messages": []
}
Request
POST <YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/InterpolatePoints/jobs/<JOB_ID> HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
Response (JSON)
// Executing job
{
"jobId": "<JOB_ID>",
"jobStatus": "esriJobExecuting",
"results": {},
"inputs": {},
"messages": []
}
// Job succeeded
{
"jobId": "<JOB_ID>",
"jobStatus": "esriJobSucceeded",
"results": {
"pointClustersResultLayer": {
"paramUrl": "results/resultLayer"
},
},
"inputs": {},
"messages": []
}
Request
POST <YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/InterpolatePoints/jobs/<JOB_ID>/results/outliersResultLayer HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&returnType=data
&token=<ACCESS_TOKEN>
Response (JSON)
{
"paramName": "resultLayer",
"dataType": "GPString",
"value": {
"url": "<SERVICE_URL>",
"itemId": "<ITEM_ID>"
}
}
Predict fuel prices
This example uses the Interpolate
operation to predict fuel prices in Madrid.
In the analysis, the input
value is the Gasolineras hosted feature layer and the Precio_
is the field
used to interpolate values.
APIs
results = interpolate_points(
input_layer="https://services1.arcgis.com/nCKYwcSONQTkPA4K/arcgis/rest/services/Gasolineras_Pro/FeatureServer/0",
field="Precio_gas",
interpolate_option=5,
classification_type="GeometricInterval",
num_classes=10,
#Outputs results as a hosted feature layer.
output_name="Interpolate points results",
context={
"extent": {
"xmin": -438873.975499999,
"ymin": 4903874.0387,
"xmax": -375084.7903,
"ymax": 4945862.018,
"spatialReference": {"wkid": 102100},
},
},
)
print(f"New item created : {results.itemid}")
Service requests
Request
POST arcgis.com/sharing/rest/portals/self HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"helperServices": {
// Other parameters
"analysis": {
"url": "https://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer"
},
"geoenrichment": {
"url": "https://geoenrich.arcgis.com/arcgis/rest/services/World/GeoenrichmentServer"
}
}
}
Request
POST <YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/InterpolatePoints/submitJob HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
&inputLayer={"url":"https://services1.arcgis.com/nCKYwcSONQTkPA4K/arcgis/rest/services/Gasolineras_Pro/FeatureServer/0"}
&field=Precio_gas
&interpolateOption=5
&classificationType=GeometricInterval
&numClasses=10'
&outputPredictionError=false
&returnFeatureCollection=false
&outputName={"serviceProperties":{"name":"Interpolate points results"}}
&context={"extent":{"xmin":-439205.7832022423,"ymin":4903730.811446736,"xmax":-375075.11647106585,"ymax":4945924.051060095,"spatialReference":{"wkid":102100,"latestWkid":3857}}}
Response (JSON)
{
"jobId": "<JOB_ID>",
"jobStatus": "esriJobSubmitted",
"results": {},
"inputs": {},
"messages": []
}
Request
POST <YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/InterpolatePoints/jobs/<JOB_ID> HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
Response (JSON)
// Executing job
{
"jobId": "<JOB_ID>",
"jobStatus": "esriJobExecuting",
"results": {},
"inputs": {},
"messages": []
}
// Job succeeded
{
"jobId": "<JOB_ID>",
"jobStatus": "esriJobSucceeded",
"results": {
"pointClustersResultLayer": {
"paramUrl": "results/resultLayer"
},
},
"inputs": {},
"messages": []
}
Request
POST <YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/InterpolatePoints/jobs/<JOB_ID>/results/outliersResultLayer HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&returnType=data
&token=<ACCESS_TOKEN>
Response (JSON)
{
"paramName": "resultLayer",
"dataType": "GPString",
"value": {
"url": "<SERVICE_URL>",
"itemId": "<ITEM_ID>"
}
}
Tutorials
Learn how to perform related analyses interactively with Map Viewer and programmatically with ArcGIS API for Python, ArcGIS REST JS, and ArcGIS REST API.
Services
Spatial analysis service
Process spatial datasets to discover relationships and patterns.
API support
Find hot spots | Find outliers | Find point clusters | Calculate density | Interpolate points | |
---|---|---|---|---|---|
ArcGIS Maps SDK for JavaScript | 1 | 1 | 1 | 1 | 1 |
ArcGIS Maps SDK for .NET | |||||
ArcGIS Maps SDK for Kotlin | |||||
ArcGIS Maps SDK for Swift | |||||
ArcGIS Maps SDK for Java | |||||
ArcGIS Maps SDK for Qt | |||||
ArcGIS API for Python | |||||
ArcGIS REST JS | 1 | 1 | 1 | 1 | 1 |
Esri Leaflet | 2 | 2 | 2 | 2 | 2 |
MapBox GL JS | 2 | 2 | 2 | 2 | 2 |
OpenLayers | 2 | 2 | 2 | 2 | 2 |
- 1. Access with geoprocessing task
- 2. Access via ArcGIS REST JS