What is a join analysis?
A join analysis is the process of combining the attributes from one feature dataset to another based on spatial and/or attribute relationships. The join data can be a feature layer or table. To execute the analysis, use the spatial analysis service and the Join
operation.
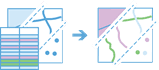
When features are joined, you can use the attributes to calculate statistics, classify the data, show additional data in popups, or visualize the data using renderers.
Real-world examples of this analysis include the following:
- Joining a table of flood data to a feature layer of county boundaries to visualize affected counties.
- Joining the attributes of a land use data with zoning data to see discrepencies between zoning and use for each parcel.
- Combining valve inspection records to fire hydrant locations to determine which hydrants are due for inspection.
How to perform a join analysis
- Review the parameters for the
Join
operation.Features - Send a request to get the spatial analysis service URL.
- Execute a job request with the following URL and parameters:
- URL:
https:
//< YOUR_ ANALYSIS_ SERVICE>/arcgis/rest/services/tasks/GPServer/Join Features/submit Job - Parameters:
input
: The point, line, polygon, or table hosted feature layer that will have attributes from the joinLayer parameter appended to its table.Layer join
: The point, line, polygon, or table hosted feature layer that will be joined to the targetLayer parameter.Layer attribute
: Defines an attribute relationship used to join features. Features are matched when the field values in the join layer are equal to field values in the target layer.Relationship output
: A string representing the name of the hosted feature layer to reutrn with the results.Name
- URL:
- Check the status.
- Get the output layer results.
To see examples using ArcGIS API for Python, ArcGIS REST JS, and the ArcGIS REST API, go to Examples below.
URL request
http://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/JoinFeatures/SubmitJob?<parameters>
Required parameters
Name | Description | Examples |
---|---|---|
f | The format of the data returned | f=json f=pjson |
token | An OAuth 2.0 access token. Learn how to get an access token in Security and authentication. | token=< |
target | The point, line, polygon, or table layer that will have attributes from the join parameter appended to its table. | {"url": < |
join | The point, line, polygon, or table layer that will be joined to the target parameter. | {"url": < |
Key parameters
Name | Description | Examples |
---|---|---|
attribute | Defines an attribute relationship used to join features. Features are matched when the field values in the join layer are equal to field values in the target layer. | [{"target |
join | A string representing the type of join (JoinOneToOne or JoinOneToMany) that will be applied. | "join |
output | A string representing the name of the hosted feature layer to return with the results. NOTE: If you do not include this parameter, the results are returned as a feature collection (JSON). | {"service |
Code examples
Join attributes from a table
This example uses the Join
operation to visualize counties with the highest recreation acreages. A feature table of recreation area values is joined to a polygon hosted feature layer by matching the Area_
field values present in both feature layers.
In the analysis, the target
value is the North Carolina regions hosted feature layer. The join
value is the North Carolina resources table. The attributes of the join
are appended to the target
based on the values provided in the attribute
parameter.
APIs
targetLayer = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/nc_regions/FeatureServer/0"
joinLayer = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/nc_resource_table/FeatureServer/0"
results = join_features(
target_layer=targetLayer,
join_layer=joinLayer,
join_operation="joinOneToOne",
attribute_relationship=[
{
"targetField": "county",
"operator": "equal",
"joinField": "Area_Name",
}
],
# Outputs results as a hosted feature layer.
output_name="Join features results",
)
print(f"New item created : {results.itemid}")
Service requests
Request
POST arcgis.com/sharing/rest/portals/self HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"helperServices": {
// Other parameters
"analysis": {
"url": "https://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer"
},
"geoenrichment": {
"url": "https://geoenrich.arcgis.com/arcgis/rest/services/World/GeoenrichmentServer"
}
}
}
Request
POST <ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/JoinFeatures/submitJob HTTP/1.1
Content-Type: application/x-www-form-urlencoded
f=json
&token=<ACCESS_TOKEN>
&targetLayer={"url":"https://services.arcgis.com/P3ePLMYs2RVChkJx/arcgis/rest/services/USA_Counties_Generalized/FeatureServer/0","filter":"STATE_NAME = 'North Carolina'","name":"County Polygons"}
&joinLayer={"url":"https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/environment_recreation_and_resources_linc/FeatureServer/0","filter":"(Area_Type = 'County') AND (Year = 2022)","name":"Environment-Recreation-Resources"}
&joinOperation=joinOneToOne
&attributeRelationship=["{"targetField":"NAME","operator":"equal","joinField":"Area_Name"}"]
&recordsToMatch={"groupByFields":"","orderByFields":"ObjectId ASC","topCount":1}
&joinType=inner
&outputName={"serviceProperties":{"name":"Join features sql result"}}
&context={"extent":{"xmin":-9745081.305501556,"ymin":3688856.7703679274,"xmax":-7938721.45306675,"ymax":4734515.317308861,"spatialReference":{"wkid":102100,"latestWkid":3857}}}
Response (JSON)
{
"jobId": "<JOB_ID>",
"jobStatus": "esriJobSubmitted",
"results": {},
"inputs": {},
"messages": []
}
Request
POST <ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/JoinFeatures/jobs/j347a8b21b5d943fbb4600772f3b2043e HTTP/1.1
Content-Type: application/x-www-form-urlencoded
f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"jobId": "<JOB_ID>",
"jobStatus": "esriJobSucceeded",
"results": {
"outputLayer": {
"paramUrl": "results/outputLayer"
}
},
"inputs": {},
"messages": []
}
Request
POST <ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/JoinFeatures/jobs/j347a8b21b5d943fbb4600772f3b2043e/results/outputLayer HTTP/1.1
Content-Type: application/x-www-form-urlencoded
f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"paramName": "outputLayer",
"dataType": "GPString",
"value": {
"url": "<RESULT_ITEM_URL>",
"itemId": "<RESULT_ITEM_ID>"
}
}
Join attributes from a feature layer
This example uses the Join
operation to join home value data to census blocks. The attributes of the points that intersect
with the polygons are joined, allowing you to visualize the census blocks by the average home value (AVGVAL_
) field.
In the analysis, the target
value is the Census Blocks
hosted feature layer. The join
is the San Francisco housing data hosted feature layer. The spatial
parameter is set to intersects
.
APIs
targetLayer = "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Census_blocks_within_San_Francisco/FeatureServer/0"
joinLayer = {
"url": "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Centroids_for_San_Francisco_housing/FeatureServer/0",
"filter": "AVGVAL_CY > 0",
}
results = join_features(
target_layer=targetLayer,
join_layer=joinLayer,
join_operation="joinOneToOne",
join_type="inner",
spatial_relationship="intersects",
# Outputs results as a hosted feature layer.
output_name="Join features results",
)
print(f"New item created : {results.itemid}")
Service requests
Request
POST arcgis.com/sharing/rest/portals/self HTTP/1.1
Content-Type: application/x-www-form-urlencoded
&f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"helperServices": {
// Other parameters
"analysis": {
"url": "https://<YOUR_ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer"
},
"geoenrichment": {
"url": "https://geoenrich.arcgis.com/arcgis/rest/services/World/GeoenrichmentServer"
}
}
}
Request
POST /arcgis/rest/services/tasks/GPServer/JoinFeatures/submitJob HTTP/1.1
Host: analysis3.arcgis.com
f=json
&token=<ACCESS_TOKEN
&targetLayer=%7B%22url%22%3A%22https%3A%2F%2Fservices3.arcgis.com%2FGVgbJbqm8hXASVYi%2Farcgis%2Frest%2Fservices%2FCensus_blocks_within_San_Francisco%2FFeatureServer%2F0%22%7D
&joinLayer=%7B%22url%22%3A%22https%3A%2F%2Fservices3.arcgis.com%2FGVgbJbqm8hXASVYi%2Farcgis%2Frest%2Fservices%2FCentroids_for_San_Francisco_housing%2FFeatureServer%2F0%22%2C%20%22filter%22%3A%22AVGVAL_CY%20%3E%200%22%7D
&joinOperation=joinOneToOne
&joinType=inner
&spatialRelationship=intersects
&outputName={"serviceProperties":{"name":"Join features spatial result"}}
Response (JSON)
{
"jobId": "<JOB_ID>",
"jobStatus": "esriJobSubmitted",
"results": {},
"inputs": {},
"messages": []
}
Request
POST <ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/JoinFeatures/jobs/j347a8b21b5d943fbb4600772f3b2043e HTTP/1.1
Content-Type: application/x-www-form-urlencoded
f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"jobId": "<JOB_ID>",
"jobStatus": "esriJobSucceeded",
"results": {
"outputLayer": {
"paramUrl": "results/outputLayer"
}
},
"inputs": {},
"messages": []
}
Request
POST <ANALYSIS_SERVICE>/arcgis/rest/services/tasks/GPServer/JoinFeatures/jobs/j347a8b21b5d943fbb4600772f3b2043e/results/outputLayer HTTP/1.1
Content-Type: application/x-www-form-urlencoded
f=json
&token=<ACCESS_TOKEN>
Response (JSON)
{
"paramName": "outputLayer",
"dataType": "GPString",
"value": {
"url": "<RESULT_ITEM_URL>",
"itemId": "<RESULT_ITEM_ID>"
}
}
Tutorials
Learn how to perform related analyses interactively with Map Viewer and programmatically with ArcGIS API for Python, ArcGIS REST JS, and ArcGIS REST API.
Services
Spatial analysis service
Process spatial datasets to discover relationships and patterns.
API support
- 1. Access with geoprocessing task
- 2. Access via ArcGIS REST JS
Tools
Developer dashboard
Manage API keys, service usage, and data with the ArcGIS Developers website.
ArcGIS.com
Create, manage, and share content and data with GIS tools.
Map Viewer
Create, explore, and share web maps for 2D applications.
ArcGIS Pro
Explore, visualize, and analyze both 2D and 3D data with desktop GIS tools.