The most common approach to perform a geometry analysis is to use a client-side API to create a geometry (point, multipoint, polyline, polygon and others) and then execute one or more geometry operations to solve a problem. When you use two or more operations for geometry analysis, this is known as chaining. For example, you can buffer a point, then calculate the area of the result, and then determine if a line intersects the buffer.
Geometry data is typically created through user interaction or by accessing features in a hosted feature layer. When the operations are complete, the results are typically displayed in a map or used as input for other analyses.
Steps
The general steps to perform a geometry analysis are:
- Determine the geometry operation(s) required to solve the problem.
- Create one or more geometries with the same spatial reference.
- Execute the geometry operations.
- Display the results in a map or use as input for another analysis.
Example
Buffer, calculate area, and intersect
This example shows how to chain together a number of geometry operations to perform a geometry analysis. It creates a point and then buffers the point, calculates the area, and then determines if the area (polygon) intersects a line.
A real-world example of this might be to determine the potential flood area (buffer) that will affect a road and if the road will intersect the flood area.
const point = Geometry.fromJSON({
type: "point",
x: -3.83565819758263,
y: 40.408142411681396,
spatialReference: SpatialReference.WGS84,
});
const buffer = geometryEngine.geodesicBuffer(point, 1000, "meters");
const area = geometryEngine.geodesicArea(buffer);
console.log("The area is:" + area);
const line = Geometry.fromJSON({
type: "polyline",
paths: [
[
[-3.7611339616121, 40.39446064625446],
[-3.6992634462656, 40.42167509407656],
[-3.65292023890635, 40.39553309352415],
],
],
spatialReference: SpatialReference.WGS84,
});
const intersects = geometryEngine.intersects(buffer, line);
console.log("The geometries intersect: " + intersects);
Tutorials
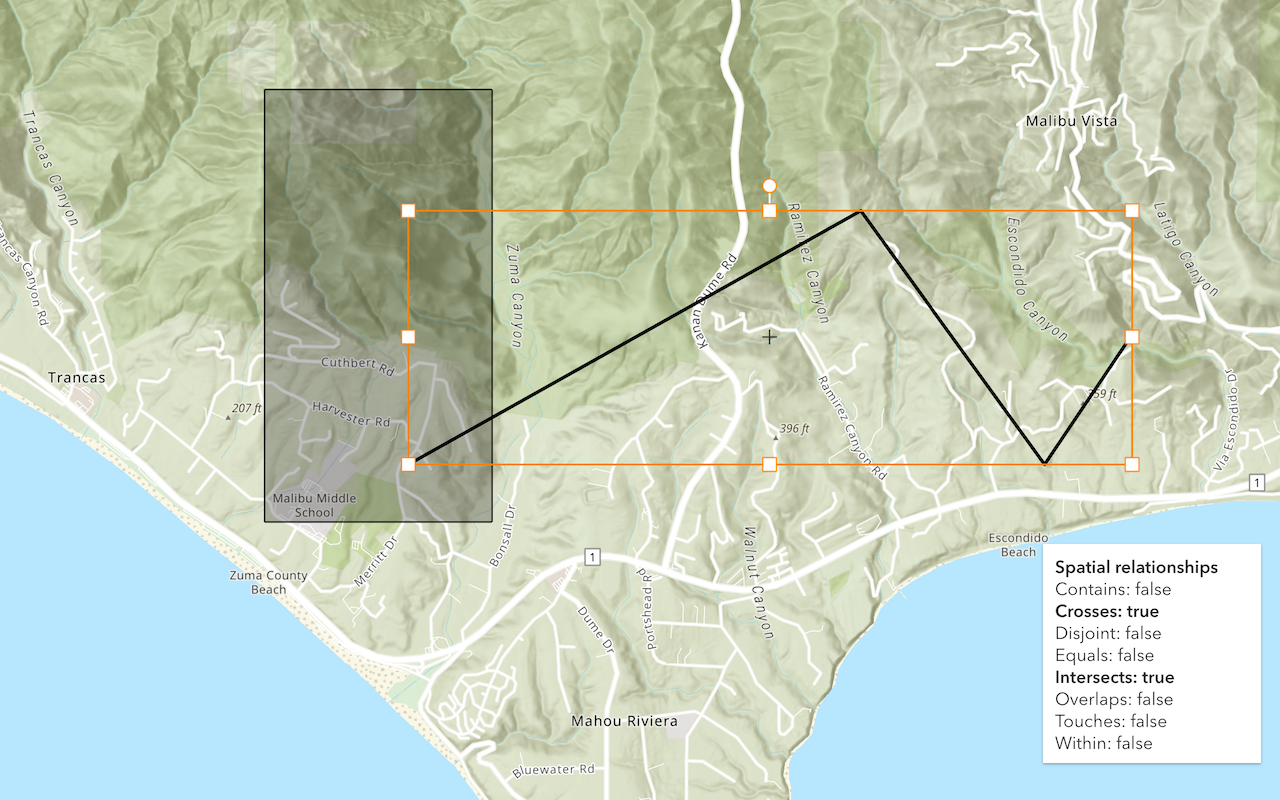
Find spatial relationships
Determine the spatial relationship between two geometries.
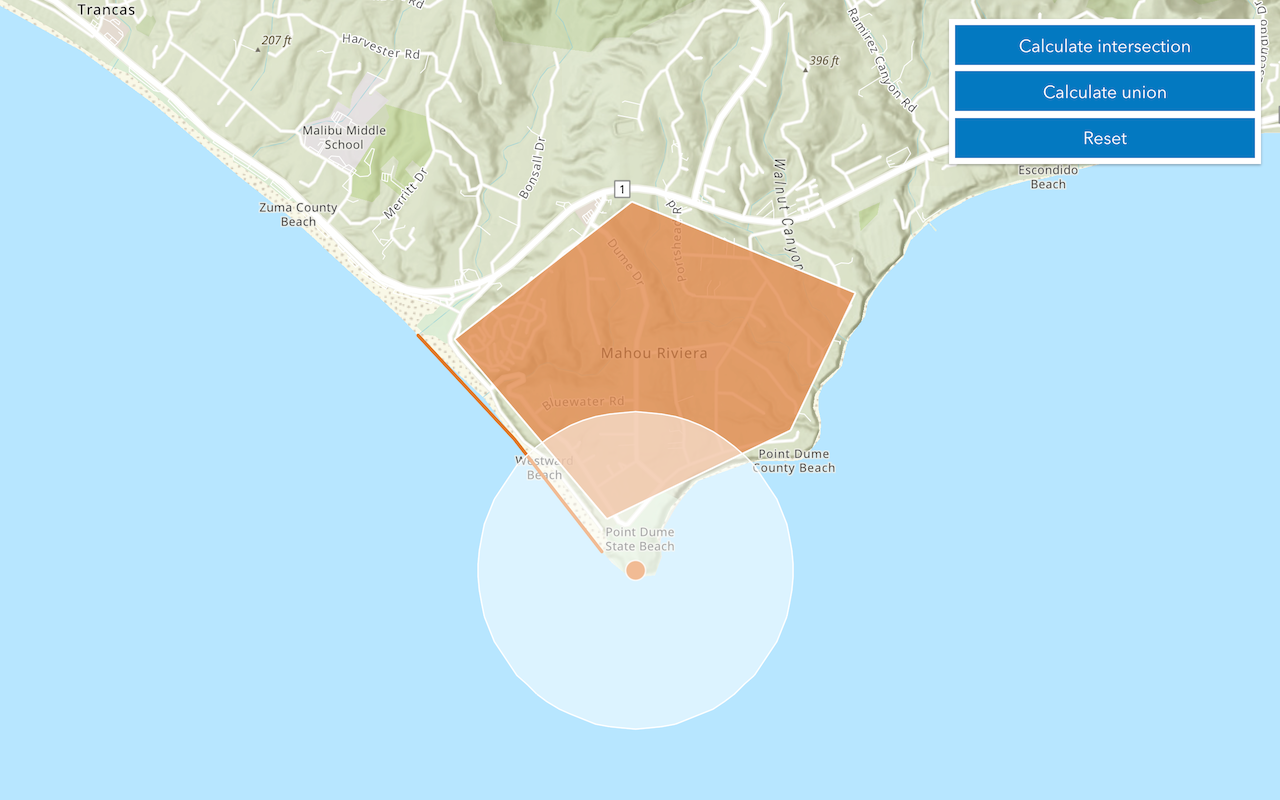
Calculate geometries
Perform buffer, intersect, union, and other geometric operations.
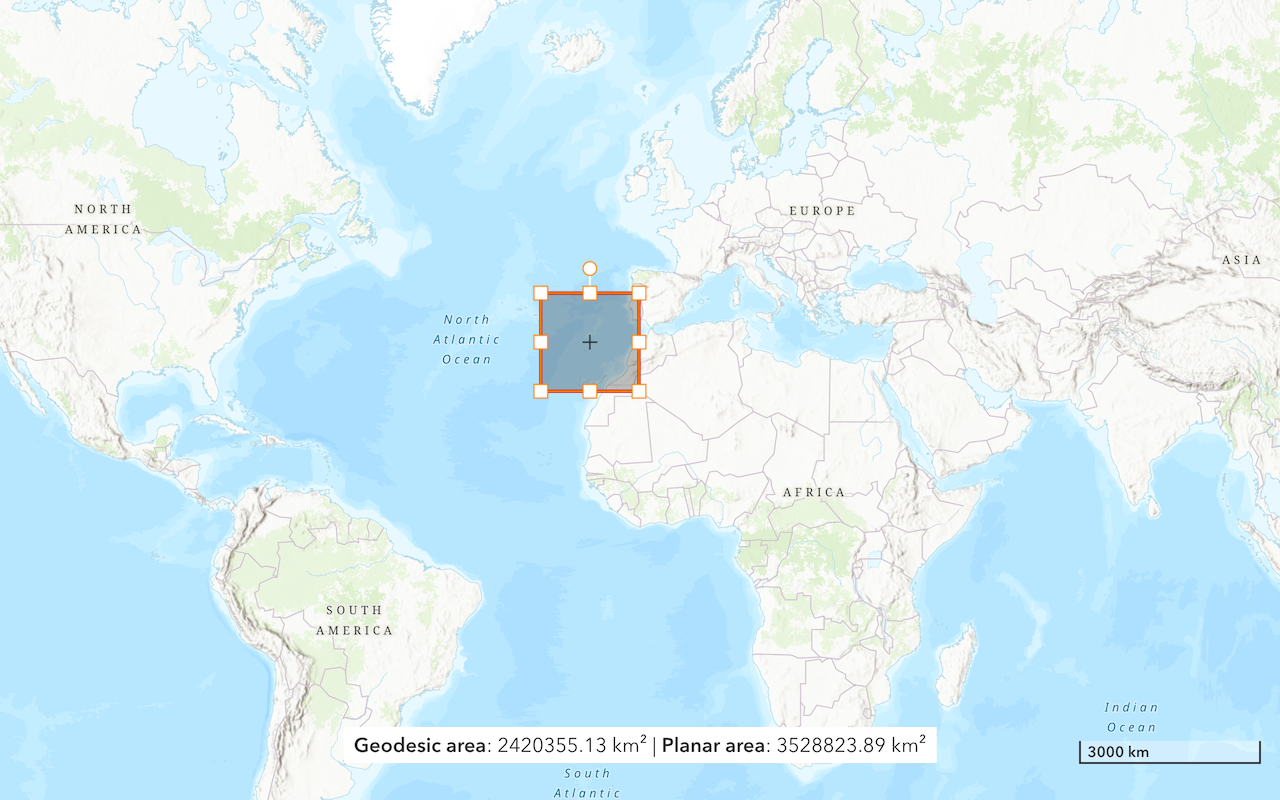
Find length and area
Get the length of a line and the area of a polygon.
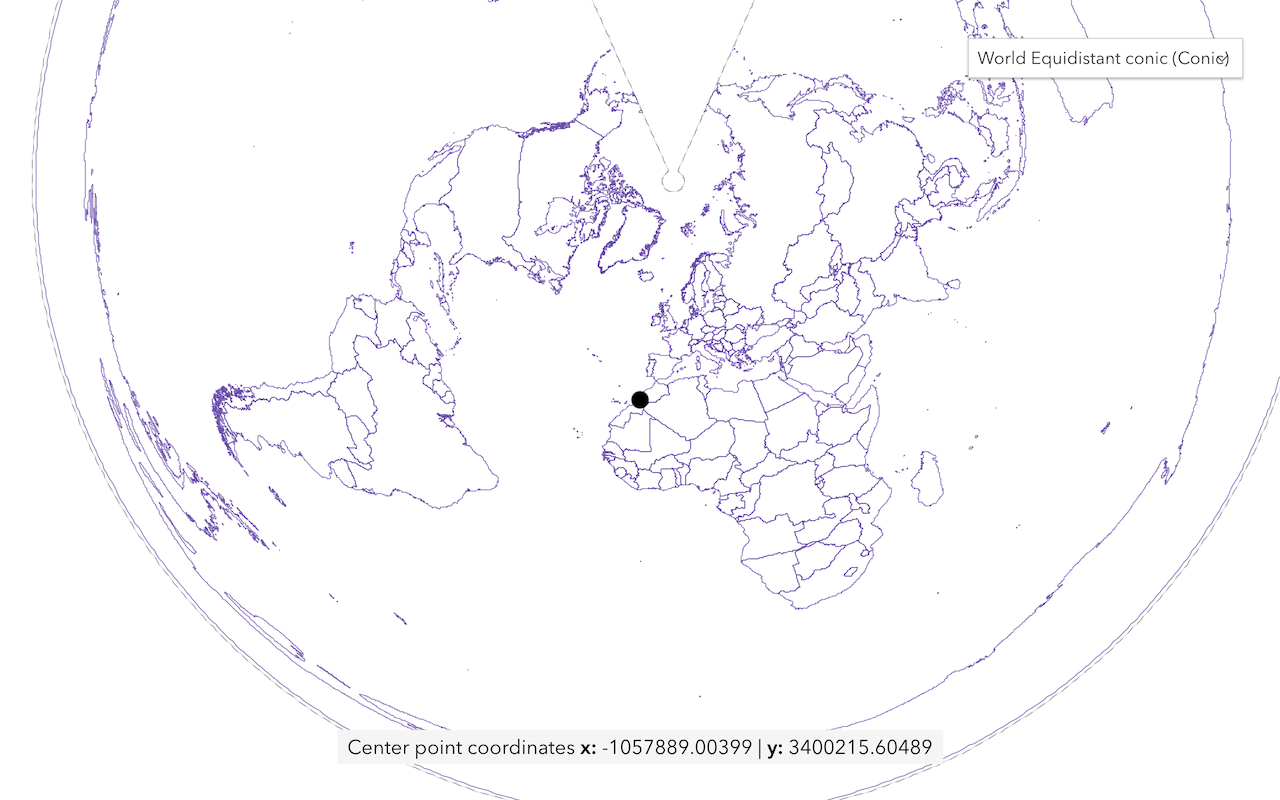
Display projected geometries
Project geometries to a new spatial reference in a map.
Services
Spatial analysis service
Process spatial datasets to discover relationships and patterns.
API support
Spatial relationship | Geometric calculation | Length and area | Projection | |
---|---|---|---|---|
ArcGIS Maps SDK for JavaScript | ||||
ArcGIS Maps SDK for .NET | ||||
ArcGIS Maps SDK for Kotlin | ||||
ArcGIS Maps SDK for Swift | ||||
ArcGIS Maps SDK for Java | ||||
ArcGIS Maps SDK for Qt | ||||
ArcGIS API for Python | ||||
ArcGIS REST JS | ||||
Esri Leaflet | ||||
MapBox GL JS | ||||
OpenLayers |