What is a spatial relationship analysis?
A spatial relationship analysis is the process of determining the topological association between two geometries. For example, you can determine if two geometries contain, cross, overlap, cross, intersect, or touch one another. To execute the analysis, you can use ArcGIS Maps SDK for JavaScript, ArcGIS Maps SDKs for Native Apps, or ArcGIS API for Python. See the code examples below.
How to determine the spatial relationship
To determine if a spatial relationship exists between two shapes, you have to create the geometries and test for a specific type of relationship. Most client APIs have specific methods for each operation. All operations return true
or false
.
To determine if a spatial relationship exists:
- Create two geometries with the same spatial reference.
- Use an operation to test for a specific type of spatial relationship. For example,
intersects
. - Evaluate the return value (
true
orfalse
).
Most APIs use the Dimensionally Extended 9-Intersection Model (DE-9IM) to evaluate if two geometries have a specific type of relationship. To do so, the following geometry properties are evaluated:
- Interior: The entire shape, except for its boundary. All geometry types have interiors.
- Boundary: The endpoints of all linear parts for line features, or the linear outline of a polygon. Only polylines and polygons have boundaries.
- Exterior: The outside area of a shape. All geometry types have exteriors.
If the interior, boundary, and exterior of the geometries satisfy the operation, it returns true, and the geometries support the spatial relationship.
Types of spatial relationship operations
The table below includes operations for determining common spatial relationships between two geometries. They return true or false indicating if the geometries satisfy the relation or not.
Operation | Description | Relation (yellow = true) |
---|---|---|
Contains | Returns true if one geometry contains another geometry. | The left geometry contains the top geometry. |
Disjoint | Returns true if one geometry does not intersect another geometry. | The left geometry is disjoint from the top geometry. |
Intersects | Returns true if one geometry intersects another geometry. | The left geometry intersects the top geometry. |
Overlaps | Returns true if one geometry overlaps another geometry. NOTE: Only valid for geometries of the same type or dimension. | The left geometry overlaps the top geometry. |
Touches | Returns true if one geometry touches another geometry. | The left geometry touches the top geometry. |
Within | Returns true if one geometry is fully within another geometry. | The left geometry is within the top geometry. |
Relate | Returns true if input geometries satisfy the specified DE-9IM spatial relation. You can provide any combination of the supported DE-9IM operations. |
Custom spatial relationships
You can test for a custom spatial relationship by defining your own relation. For example, you can create a relation that tests if two geometries intersect and touch. To do so, see the Relate example below.
Code examples
Contains
Determine if one geometry contains another geometry.
const contains = geometryEngine.contains(squareOutside, squareInside);
if (contains) {
console.log("The outer square contains the inner square.");
}
Disjoint
Determine if two geometries are disjoint.
const disjoint = geometryEngine.disjoint(leftSquare, rightSquare);
if (disjoint) {
console.log("The squares are disjoint.");
}
Intersects
Determine if two geometries intersect.
const intersects = geometryEngine.intersects(line, square);
if (intersects) {
console.log("The line intersects the square.");
}
Overlaps
Determine if two geometries overlap.
const overlaps = geometryEngine.overlaps(rectangle, square);
if (overlaps) {
console.log("The rectangle and square overlap.");
}
Touches
Determine if two geometries touch.
const touches = geometryEngine.touches(triangle, square);
if (touches) {
console.log("The triangle touches the square.");
}
Within
Determine if one geometry is fully within another.
const within = geometryEngine.within(square, rectangle);
if (within) {
console.log("The square is within the rectangle.");
}
Relate
Determine if the geometries satisfy a custom DE-9IM relation. The test below is for a polyline that intersects and touches the a part of the square. The test is: "FFT****".
// Default DE-91M relations
// touches FF*FT****
// intersects ****T****
// Custom relation: touches + intersects = *F*FT****
const related = geometryEngine.relate(polyline, square, "*F*FT****");
if (related) {
console.log("The line touches and intersects the square.");
}
Tutorials
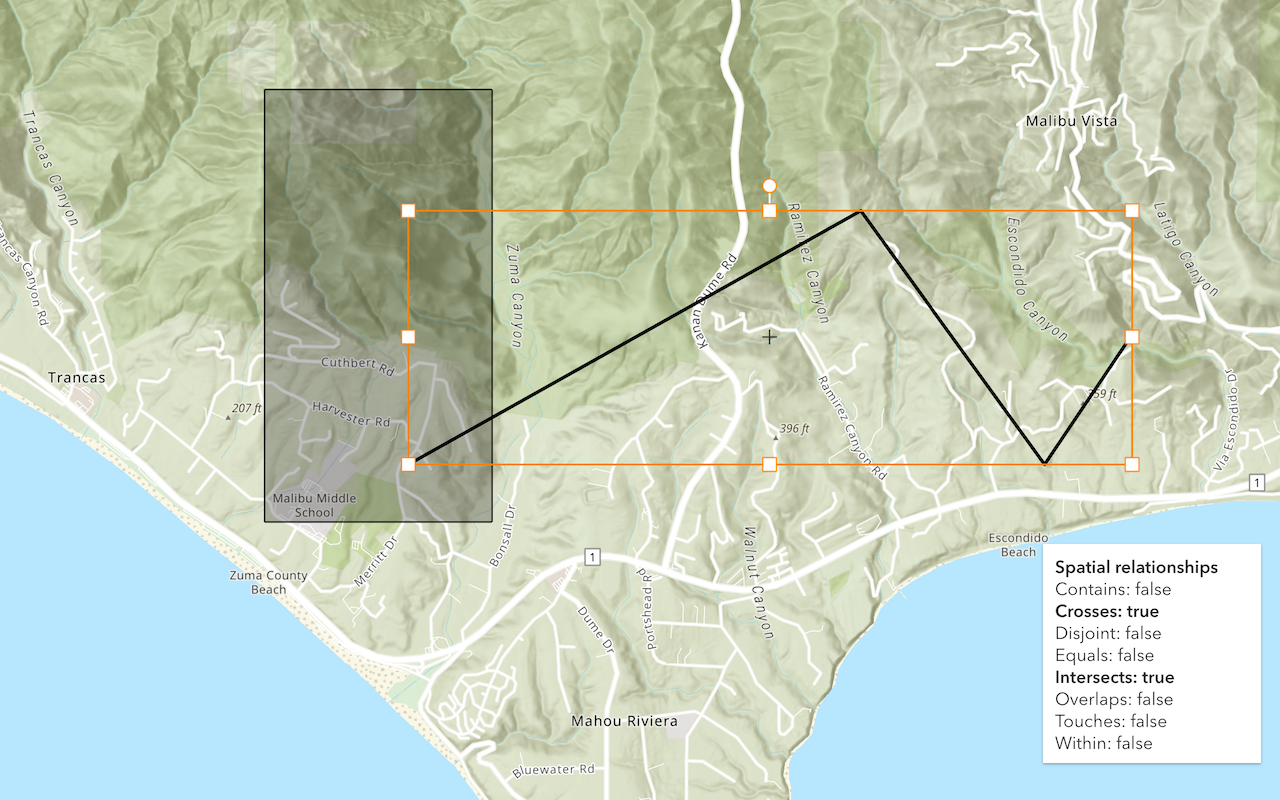
Find spatial relationships
Determine the spatial relationship between two geometries.
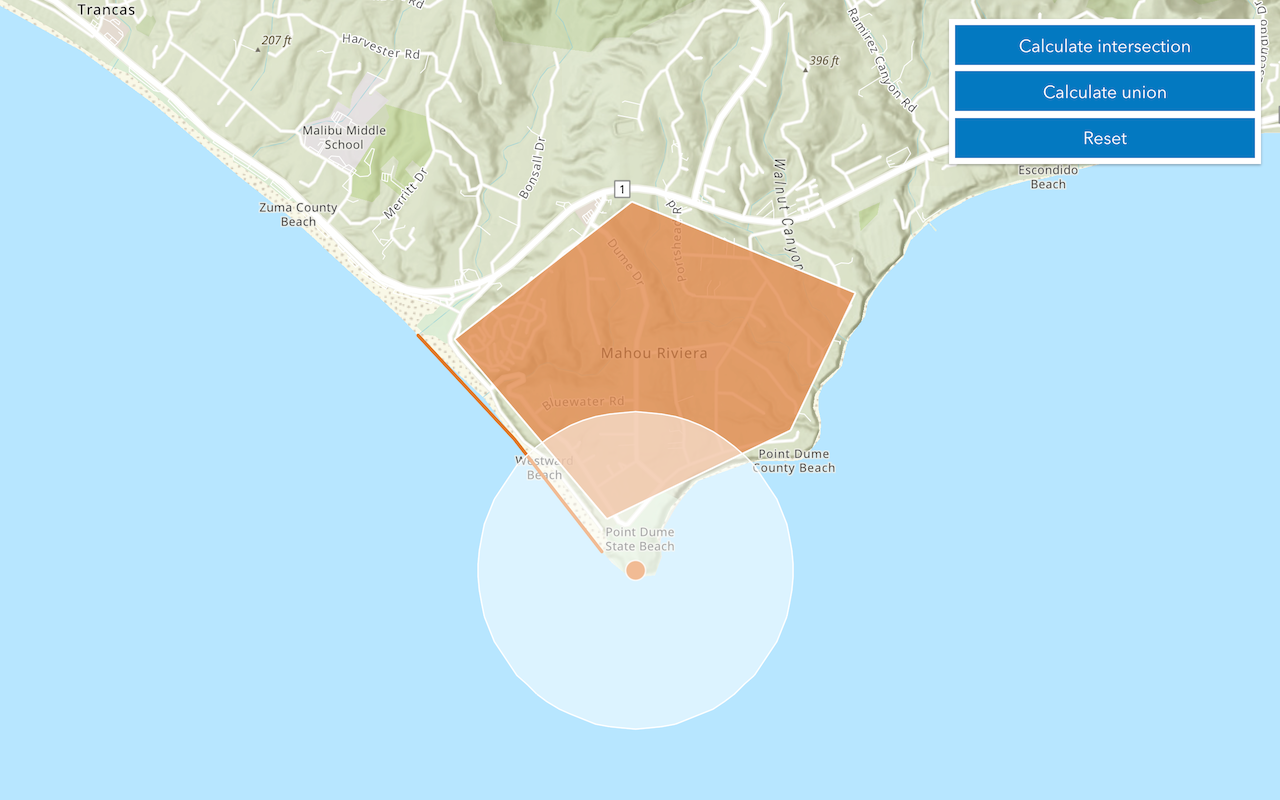
Calculate geometries
Perform buffer, intersect, union, and other geometric operations.
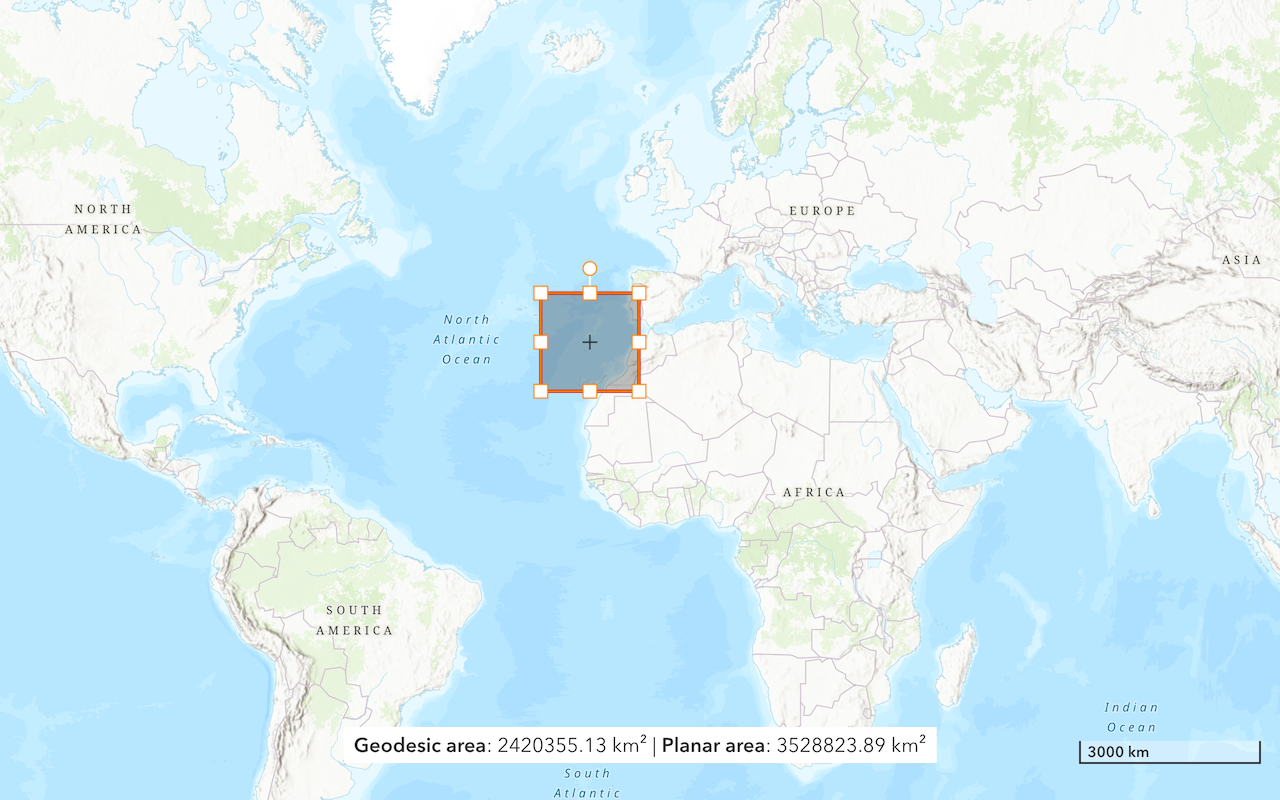
Find length and area
Get the length of a line and the area of a polygon.
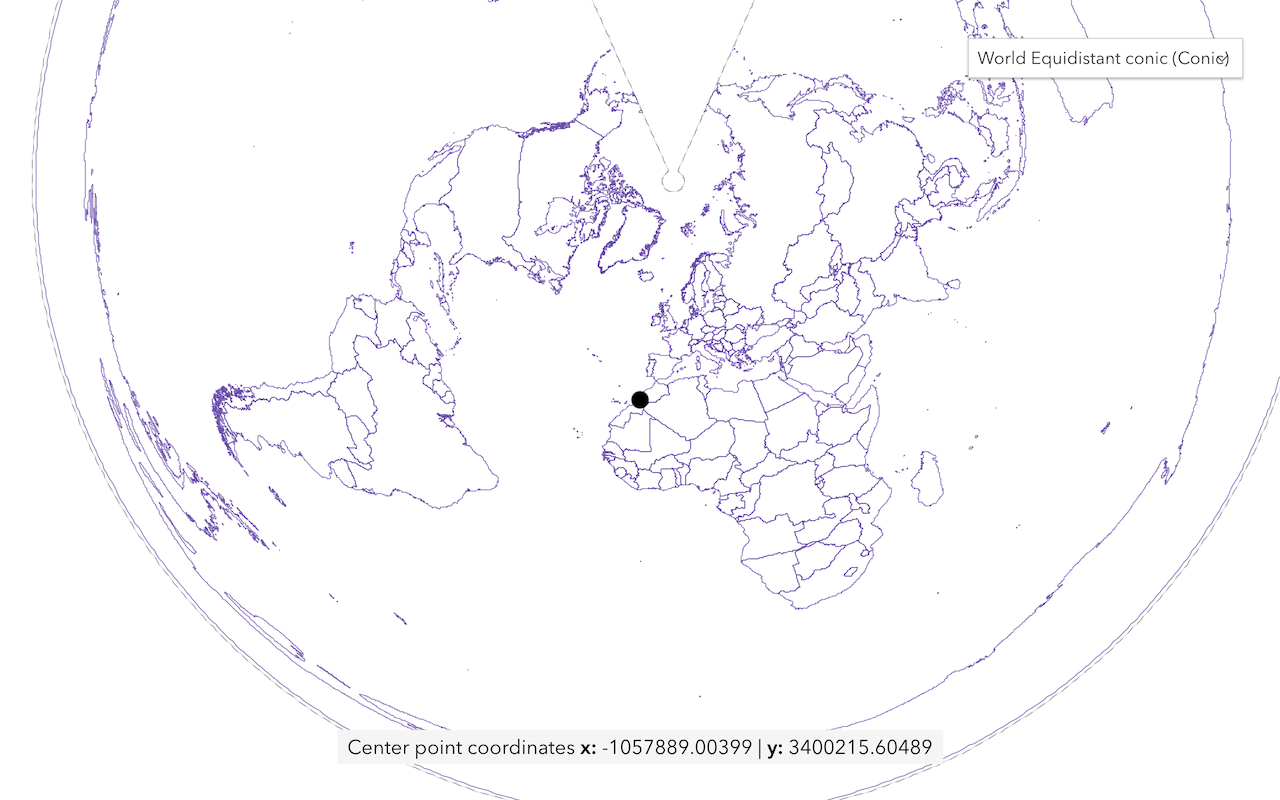
Display projected geometries
Project geometries to a new spatial reference in a map.
API support
Spatial relationship | Geometric calculation | Length and area | Projection | |
---|---|---|---|---|
ArcGIS Maps SDK for JavaScript | ||||
ArcGIS Maps SDK for .NET | ||||
ArcGIS Maps SDK for Kotlin | ||||
ArcGIS Maps SDK for Swift | ||||
ArcGIS Maps SDK for Java | ||||
ArcGIS Maps SDK for Qt | ||||
ArcGIS API for Python | ||||
ArcGIS REST JS | ||||
Esri Leaflet | ||||
MapBox GL JS | ||||
OpenLayers |