What is a class breaks style?
The class breaks style allows you to visualize numeric ranges of data. It involves defining meaningful ranges of numbers into two or more classes, representing each class with a unique symbol. You can use this style to answer questions beginning with how much?
While a class break's symbol is distinct from another class break's symbol, symbols are typically similar to one another in all aspects with the exception of one property, such as color.
How a class breaks style works
This style is configured with a class breaks renderer. This renderer requires the following:
- A reference to a data value either from a field name, or an Arcade expression.
- A list of class break info objects that match a unique symbol with a range of expected values returned from the field or expression.
For visualizations with light basemaps or backgrounds, classes representing smaller numbers are typically represented with light colors and classes representing larger numbers are represented with dark colors.
There are several classification methods that can be employed in the creation of this renderer, but defining class breaks in code typically involves manual classification.
Classified visualizations are ideal for maps where predefined breaks hold meaning to the end user. The range for each class and the number of classes should be deliberate choices made after careful consideration.
Code examples
Normalize by attribute
This example demonstrates how to visualize ranges of numeric data values normalized by another data attribute. In the vast majority of cases, you should not normalize total counts with polygon data. These values should be normalized by a base value or the area of the polygon.
This app visualizes the percentage of people age 25 and older that did not attend any high school classes.
- Create a class breaks renderer.
- Reference a numeric field name containing the total number of people that did not complete high school.
- Reference a
normalization
, in this case, the total number of people age 25 and older. The value ofField field
will be divided by the value ofnormalization
.Field - Create four class break info objects and assign a symbol to each range of values.
- You can optionally add a default symbol to represent all values that don't fall within one of the breaks.
const renderer = {
type: "class-breaks",
field: "NOHS_CY",
normalizationField: "EDUCBASECY",
legendOptions: {
title: "% of adults with no high school education"
},
defaultSymbol: {
type: "simple-fill",
color: "black",
style: "backward-diagonal",
outline: {
width: 0.5,
color: [50, 50, 50, 0.6]
}
},
defaultLabel: "no data",
classBreakInfos: [
{
minValue: 0,
maxValue: 0.04999,
symbol: createSymbol("#edf8fb"),
label: "< 5%"
},
{
minValue: 0.05,
maxValue: 0.14999,
symbol: createSymbol("#b3cde3"),
label: "5 - 15%"
},
{
minValue: 0.15,
maxValue: 0.24999,
symbol: createSymbol("#8c96c6"),
label: "15 - 25%"
},
{
minValue: 0.25,
maxValue: 1.0,
symbol: createSymbol("#88419d"),
label: "> 25%"
}
]
};
Normalize by area
This example demonstrates how to visualize ranges of values normalized by the area of each polygon. This app visualizes the number of households per square mile. This value is calculated client-side using an Arcade expression. First, write the Arcade expression.
$feature.TOTHH_CY / AreaGeodetic($feature, 'square-miles');
- Create a class breaks renderer.
- Reference the Arcade expression in the
value
property.Expression - Create four class break info objects and assign a symbol to each range of values.
- You can optionally add a default symbol to represent all values that don't fall within one of the breaks.
const renderer = {
type: "class-breaks",
valueExpression: `
$feature.TOTHH_CY / AreaGeodetic($feature, 'square-miles');
`,
valueExpressionTitle: "Households per square mile",
classBreakInfos: [
{
minValue: 0,
maxValue: 2500,
symbol: createSymbol("#edf8fb"),
label: "< 2,500"
},
{
minValue: 2500,
maxValue: 5000,
symbol: createSymbol("#b3cde3"),
label: "2,500 - 5,000"
},
{
minValue: 5000,
maxValue: 10000,
symbol: createSymbol("#8c96c6"),
label: "5000 - 10,000"
},
{
minValue: 10000,
maxValue: 1000000,
symbol: createSymbol("#88419d"),
label: "> 10,000"
}
]
};
Graduated point symbols in 3D
This example visualizes earthquakes on a globe based on their magnitude. The goal of this map is to show the major earthquakes that cause severe damage, the moderate ones that cause damage only to buildings which are not earthquake proof, and small earthquakes that don't cause any serious damage. A class breaks renderer with three classes based on the magnitude ranges is the most suitable to see these types of earthquakes at a glance.
Steps
- Create three classes for high magnitude earthquakes (greater than 7), moderate magnitude (greater than 5 and less than 7) and small magnitude (less than 5).
- Assign an intuitive symbol to each of the classes.
- Set these classes on a class breaks renderer.
- Assign the renderer to the earthquake layer.
const renderer = {
type: "class-breaks",
field: "mag",
legendOptions: {
title: "Legend",
},
classBreakInfos: [
{
minValue: -2,
maxValue: 5,
symbol: {
type: "point-3d",
symbolLayers: [baseSymbolLayer],
},
label: "Magnitude < 5",
},
{
minValue: 5,
maxValue: 7,
symbol: {
type: "point-3d",
symbolLayers: [baseSymbolLayer, secondSymbolLayer],
},
label: "Magnitude between 5 and 7",
},
{
minValue: 7,
maxValue: 10,
symbol: {
type: "point-3d",
symbolLayers: [
baseSymbolLayer,
secondSymbolLayer,
thirdSymbolLayer,
],
},
label: "Magnitude larger than 7",
},
],
};
earthquakesLayer.renderer = renderer;
Tutorials
Learn how to build and style layers with step-by-step tutorials.
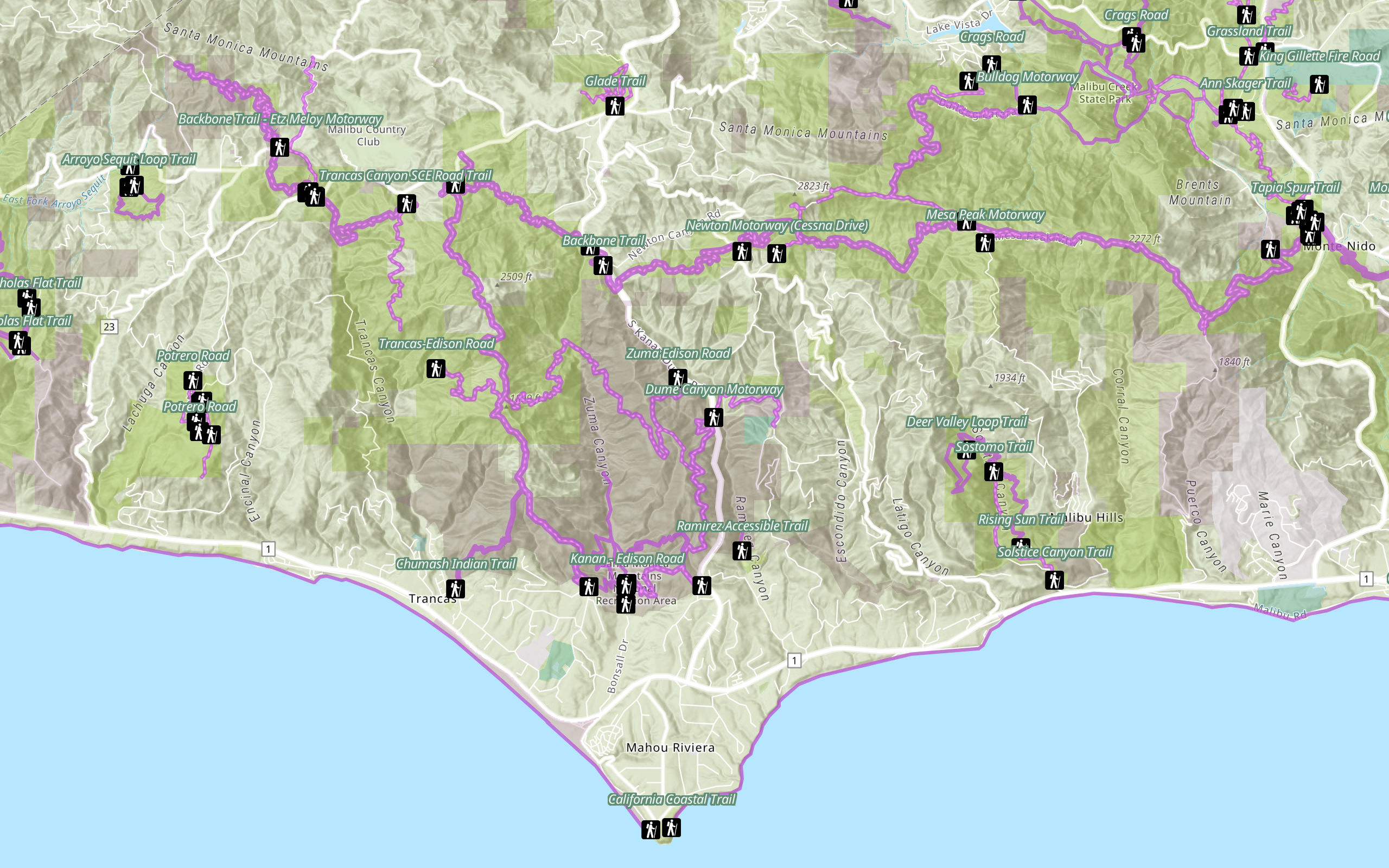
Style a feature layer
Use symbols and renderers to style feature layers.
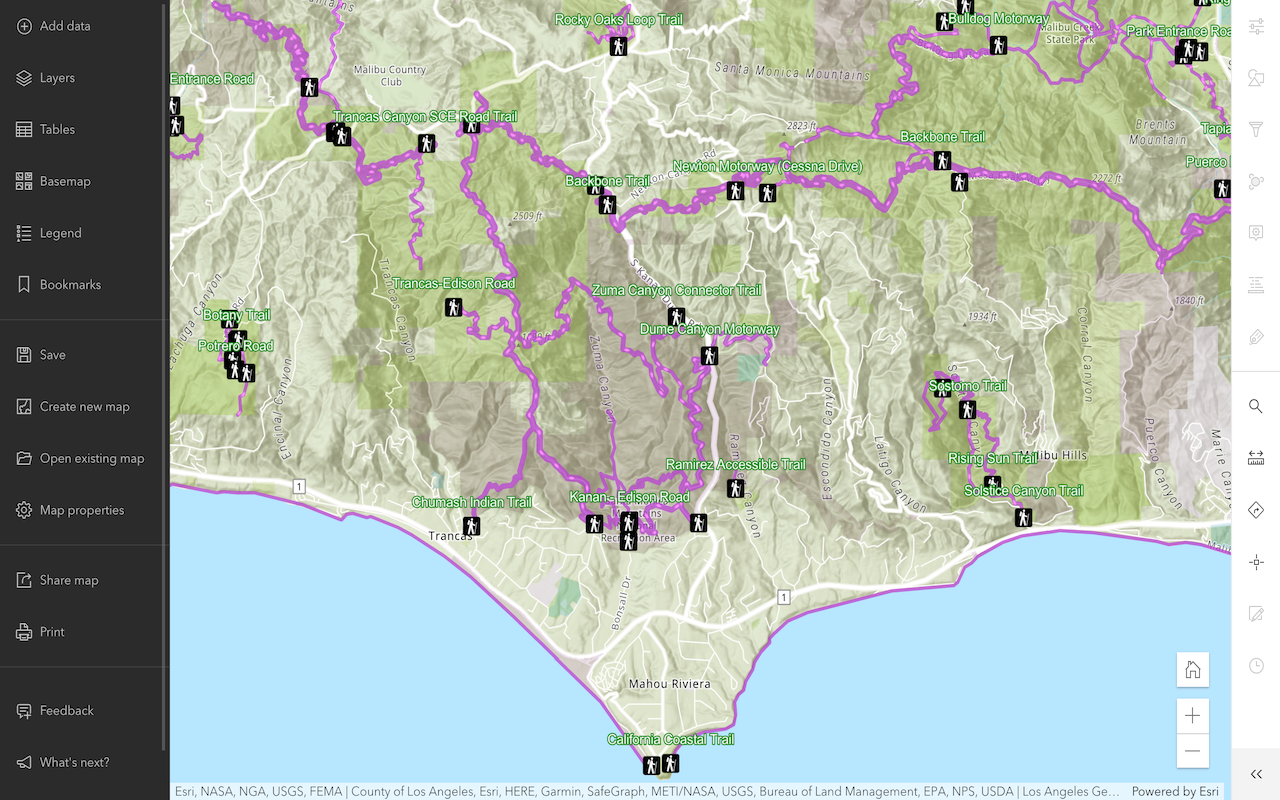
Style layers in a web map
Use Map Viewer to style layers in a web map.
Services
Different APIs have different levels of support for data-driven visualization.
Unique types | Class breaks | Visual variables | Time | Multivariate | Predominance | Dot density | Relationship | |
---|---|---|---|---|---|---|---|---|
ArcGIS Maps SDK for JavaScript | ||||||||
ArcGIS Maps SDK for Kotlin | ||||||||
ArcGIS Maps SDK for Swift | ||||||||
ArcGIS Maps SDK for Java | ||||||||
ArcGIS Maps SDK for .NET | ||||||||
ArcGIS Maps SDK for Qt | ||||||||
ArcGIS API for Python |
API support
Different APIs have different levels of support for data-driven visualization.
Unique types | Class breaks | Visual variables | Time | Multivariate | Predominance | Dot density | Relationship | |
---|---|---|---|---|---|---|---|---|
ArcGIS Maps SDK for JavaScript | ||||||||
ArcGIS Maps SDK for Kotlin | ||||||||
ArcGIS Maps SDK for Swift | ||||||||
ArcGIS Maps SDK for Java | ||||||||
ArcGIS Maps SDK for .NET | ||||||||
ArcGIS Maps SDK for Qt | ||||||||
ArcGIS API for Python |
Tools
Use tools such as Map Viewer and Scene Viewer to create and style web maps and web scenes for your applications.