What is a dot density style?
The dot density style visualizes the density of a population, or other count, in a polygon data layer by randomly drawing dots within polygon boundaries. Each dot represents a constant numeric value of an attribute or a subset of attributes.
For example, when visualizing population density where each dot represents 100 people, a polygon with a population of 100,000 people would contain 1,000 dots randomly drawn within the polygon.
Unlike choropleth maps, field values used in dot density visualizations don't need to be normalized because the size of the polygon, together with the number of dots rendered within its boundaries, indicate the spatial density of that value.
How a dot density style works
The dot density style is configured with a dot density renderer. A dot density renderer requires the following:
- A list of attributes (minimum of one attribute), matching a data value returned from a field or Arcade expression with a color.
- A
dot
, or the value each dot will represent for each attribute.Value - Optionally (but suggested), a
reference
referring to the view scale.Scale
Code examples
Population density
The following example visualizes population density in the United States.
const dotDensityRenderer = new DotDensityRenderer({
dotValue: 100,
outline: null,
referenceScale: 577790,
legendOptions: {
unit: "people"
},
attributes: [
{
field: "B03002_001E",
color: "#e8ca0d",
label: "Places where people live"
}
]
});
Population density by category
You can specify multiple attributes to visualize subcategories of the data. For example, we can visualize the same layer above, but categorize each dot by race/ethnicity. So each dot of a different color represents 100 people of the respective category (e.g. 1 red dot represents 100 white non-Hispanic) people, each blue dot = 100 African American people, each yellow dot = 100 Hispanic people, etc).
const dotDensityRenderer = new DotDensityRenderer({
dotValue: 100,
outline: null,
referenceScale: 577790, // 1:577,790 view scale
legendOptions: {
unit: "people"
},
attributes: [
{
field: "B03002_003E",
color: "#f23c3f",
label: "White (non-Hispanic)"
},
{
field: "B03002_012E",
color: "#e8ca0d",
label: "Hispanic"
},
{
field: "B03002_004E",
color: "#00b6f1",
label: "Black or African American"
},
{
field: "B03002_006E",
color: "#32ef94",
label: "Asian"
},
{
field: "B03002_005E",
color: "#ff7fe9",
label: "American Indian/Alaskan Native"
},
{
field: "B03002_007E",
color: "#e2c4a5",
label: "Pacific Islander/Hawaiian Native"
},
{
field: "B03002_008E",
color: "#ff6a00",
label: "Other race"
},
{
field: "B03002_009E",
color: "#96f7ef",
label: "Two or more races"
}
]
});
Best practices
The following practices should be followed when creating a dot density visualization.
View scale
This implementation of dot density allows you to linearly scale the dot value based on the view scale. As you zoom in and out of the initial view, the relative density of points remains the same across scales. Always set a reference
to make the visualization work across many scale levels.
In addition to setting a reference
, you should typically set a min
on the layer. Dot density visualizations are difficult to read when dots are no longer distinguishable, either because they coalesce or because they are too dispersed.
Setting a max
on the layer is also important because dot density maps tend to become unreadable at larger scales. Users may start seeing patterns in the random distribution of dots that do not exist in reality. They may also mistakenly interpret the location of each dot as an actual point feature. Users are particularly susceptible to this when the dot
is set to 1. As an example, dot density visualizations on county datasets should only be viewed at the state or regional level.
View spatial reference
You should use equal area spatial references whenever possible, particularly when building dot density visualizations that span global extents. Using heavily distorted spatial references with a dot density renderer is acceptable as long as you restrict the user from comparing the densities of features within the same view at large scales. Try not to compare features across multiple views when equal area projections cannot be used, since significant differences in area distortion may cause the user to misinterpret feature density in heavily distorted areas.
Attributes
Use the attributes
property to specify one or more data values. When multiple attributes are specified, the group of attributes should make logical sense when visualized together, and generally should belong to a parent group. For example, you could map the density of pets, or the density of different kinds of pets, such as dogs or cats. However, it doesn't make sense to map the density of cats and the density of crops within the same renderer.
Use pop-ups to clearly communicate the actual value of the feature, so the user can explore the map visually and interactively.
Tutorials
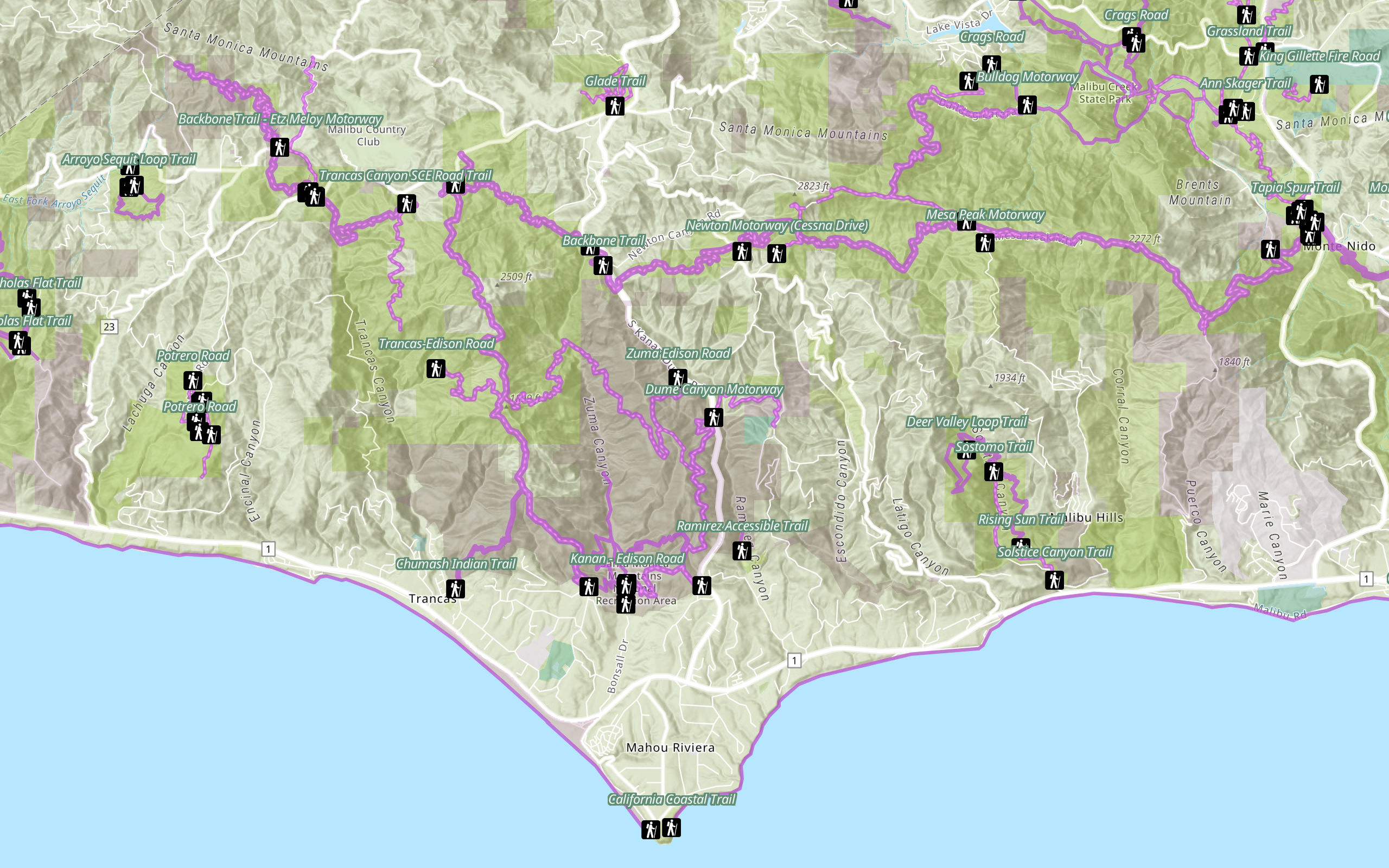
Style a feature layer
Use symbols and renderers to style feature layers.
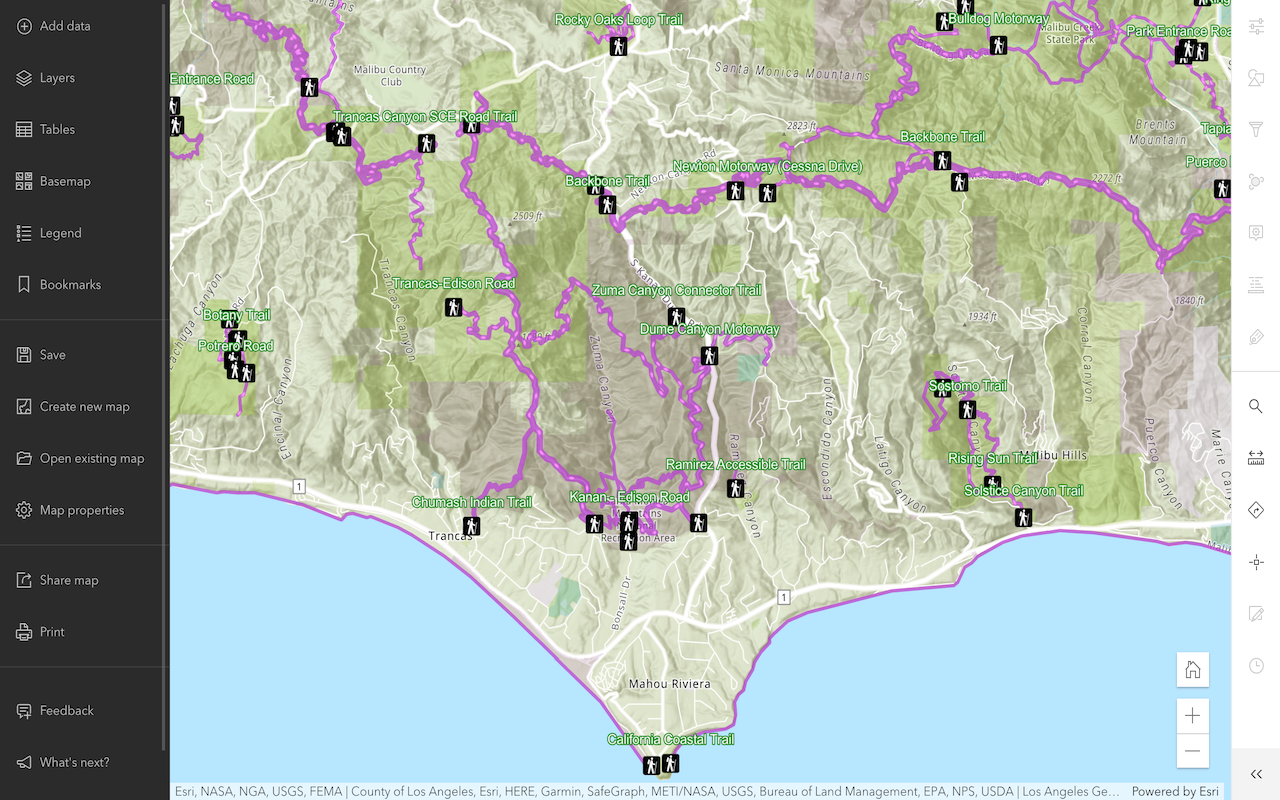
Style layers in a web map
Use Map Viewer to style layers in a web map.
Services
Feature service
Add, update, delete, and query feature data.
API support
Different APIs have different levels of support for data-driven visualization.
Unique types | Class breaks | Visual variables | Time | Multivariate | Predominance | Dot density | Relationship | |
---|---|---|---|---|---|---|---|---|
ArcGIS Maps SDK for JavaScript | ||||||||
ArcGIS Maps SDK for Kotlin | ||||||||
ArcGIS Maps SDK for Swift | ||||||||
ArcGIS Maps SDK for Java | ||||||||
ArcGIS Maps SDK for .NET | ||||||||
ArcGIS Maps SDK for Qt | ||||||||
ArcGIS API for Python |