Learn how to access local data, such as spending trends, for the United States with the GeoEnrichment service.
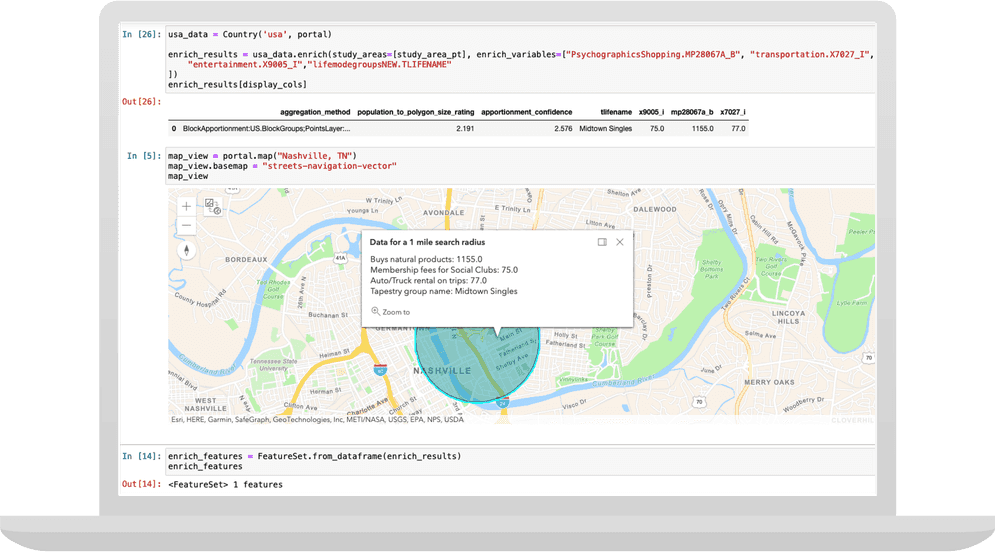
The GeoEnrichment service provides detailed local data for specific countries. Each individual data field is represented by an analysis variable that are organized into data categories such as spending and market behaviors such as 2022 Educational Attainment or 2022 Seen Video Ad at Gas Station Last 30 Days. The data available varies by country and by data provider.
In this tutorial, you use the GeoEnrichment service to display spending trend information for a study area within the United States.
Steps
Import modules and log in
-
Import the
GIS
class from thearcgis.gis
module. TheGIS
class provides helper objects to manage (search, create, and manage) GIS resources such as content, users, and groups. Additionally, import theGeometry
andPoint
classes from thearcgis.geometry
module, theFeature
class fromSet arcgis.features
and theCountry
method from thearcgis.geoenrichment
module.Use dark colors for code blocks from arcgis.gis import GIS from arcgis.features import FeatureSet from arcgis.geoenrichment import Country from arcgis.geometry import Geometry, Point
-
Log in to ArcGIS Online using your credentials. In a hosted notebook, You can use the
home
parameter to use the credentials of the currently logged in account.Use dark colors for code blocks from arcgis.gis import GIS from arcgis.features import FeatureSet from arcgis.geoenrichment import Country from arcgis.geometry import Geometry, Point portal = GIS("home") print(f"Connected to {portal.properties.name} as {portal.properties.user.username}")
Create study area point
-
Use the
Point
class to construct a point geometry by passing in the coordinates and spatial reference representing a location in Nashville, Tennessee.Use dark colors for code blocks portal = GIS("home") print(f"Connected to {portal.properties.name} as {portal.properties.user.username}") study_area_pt = Point({"x": -86.7679, "y": 36.1745, "spatialReference": {"wkid": 4326}})
Get demographic data
-
Use the
Country
class from thearcgis.geoenrichment
module. This provides acces to local variables for a specific country. Pass in the study area point and the enrich variables :Psychographics
,Shopping.MP28067A_ B transportation.X7027_
,I entertainment.X9005_
, andI lifemodegroups
as arrays. To learn more about the data collections and variables, go to the data enrichment section of this guide.NEW.TLIFENAME Use dark colors for code blocks study_area_pt = Point({"x": -86.7679, "y": 36.1745, "spatialReference": {"wkid": 4326}}) usa_data = Country("usa", portal) enrich_results = usa_data.enrich( study_areas=[study_area_pt], enrich_variables=[ "PsychographicsShopping.MP28067A_B", "transportation.X7027_I", "entertainment.X9005_I", "lifemodegroupsNEW.TLIFENAME", ], )
-
To view the values returned from the enrichment, use the
dataframe
object returned from theenrich
method to display the results as a table. Pass in an array of field names to limit the results to a subset of columns.Use dark colors for code blocks usa_data = Country("usa", portal) enrich_results = usa_data.enrich( study_areas=[study_area_pt], enrich_variables=[ "PsychographicsShopping.MP28067A_B", "transportation.X7027_I", "entertainment.X9005_I", "lifemodegroupsNEW.TLIFENAME", ], ) display_cols = [ "aggregation_method", "population_to_polygon_size_rating", "apportionment_confidence", "tlifename", "x9005_i", "mp28067a_b", "x7027_i", ] enrich_results[display_cols]
Display the results on a map
-
Use the
map
property from theGIS
class to create a map. To set the initial extent of the map, pass in the stringNashville, TN
This will set the map to an extent that is appropriate for the results. Set the basemap of the map tostreets-navigation-vector
.Use dark colors for code blocks display_cols = [ "aggregation_method", "population_to_polygon_size_rating", "apportionment_confidence", "tlifename", "x9005_i", "mp28067a_b", "x7027_i", ] enrich_results[display_cols] map_view = portal.map("Nashville, TN") map_view.basemap = "streets-navigation-vector" map_view
-
Use the
Feature
class to convert the returned Pandas DataFrame into a feature set. Create a popup object that will display formatted attribute values when a feature is clicked in the map.Set Use dark colors for code blocks map_view = portal.map("Nashville, TN") map_view.basemap = "streets-navigation-vector" map_view enrich_features = FeatureSet.from_dataframe(enrich_results) enrich_features popup_data = [ f"Buys natural products: {enrich_features.features[0].attributes['mp28067a_b']}", f"Membership fees for Social Clubs: {enrich_features.features[0].attributes['x9005_i']}", f"Auto/Truck rental on trips: {enrich_features.features[0].attributes['x7027_i']}", f"Tapestry group name: {enrich_features.features[0].attributes['tlifename']}", ] popup_info = { "title": "<b>Data for a 1 mile search radius</b>", "content": "<br>".join(popup_data), }
-
Use the
clear_
method to remove any previous results from the _Mapping. Then use thegraphics() draw
method and pass in the enriched geomety and popup info to add the results to the map. Set theextent
of the map to the result geometry's extent.Use dark colors for code blocks enrich_features = FeatureSet.from_dataframe(enrich_results) enrich_features popup_data = [ f"Buys natural products: {enrich_features.features[0].attributes['mp28067a_b']}", f"Membership fees for Social Clubs: {enrich_features.features[0].attributes['x9005_i']}", f"Auto/Truck rental on trips: {enrich_features.features[0].attributes['x7027_i']}", f"Tapestry group name: {enrich_features.features[0].attributes['tlifename']}", ] popup_info = { "title": "<b>Data for a 1 mile search radius</b>", "content": "<br>".join(popup_data), } map_view.clear_graphics() map_view.draw(enrich_features.features[0].geometry, popup=popup_info) map_view.extent = Geometry(enrich_features.features[0].geometry).extent
You should see a map centered around Nashville, TN with a graphic representing a 1 mile radius around the study area point. Click on the graphic to display a popup containing local demographic values.
What's next?
Learn how to use additional functionality in these tutorials: