Learn how to create and display a map with a basemap layer.
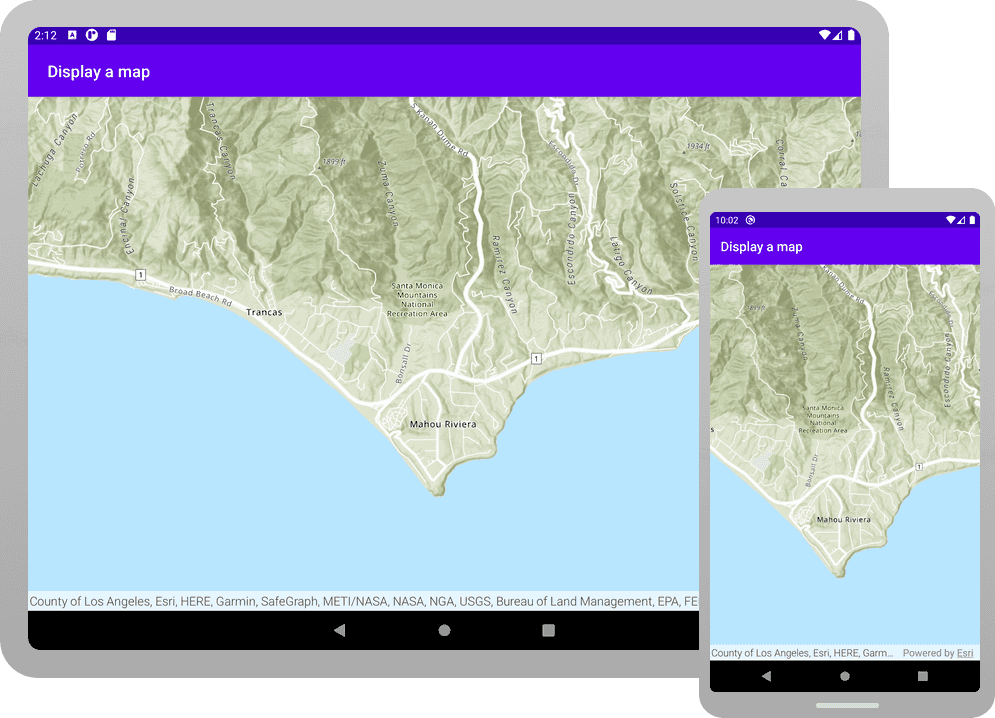
A map contains layers of geographic data. A map contains a basemap layer and, optionally, one or more data layers. You can display a specific area of a map by using a map view and setting the location and zoom level.
In this tutorial, you create and display a map of the Santa Monica Mountains in California using the topographic basemap layer.
The map and code will be used as the starting point for other 2D tutorials.
Prerequisites
The following are required for this tutorial:
- An ArcGIS account to access API keys. If you don't have an account, sign up for free.
- Confirm that your system meets the system requirements.
- An IDE for Android development in Kotlin.
Steps
Create a new Android Studio project
Use Android Studio to create an app and configure it to reference the API.
-
Open Android Studio.
- In the menu bar, click File > New > New Project....
- In the Create New Project window, make sure Phone and Tablet tab is selected, and then select Empty Activity. Click Next.
Gradle is the default build tool in Android Studio. If you are unable to use Gradle, see Getting the API Manually.
- In the Configure your project window, set the following configuration options:
- Name:
Display a map
. - Package name: Change to
com.example.app
. Or change to match your organization. - Save location: Set to a new folder.
- Language: Kotlin
- Minimum SDK: API 23: Android 6.0 (Marshmallow)
- Name:
-
In the Project tool window, make sure that your current view is Android. These tutorial instructions refer to that view.
If your view name is something other than Android (such as Project or Packages), click on the leftmost control in the title bar of the Project tool window, and select Android from the list.
-
From the Project tool window, open Gradle Scripts > build.gradle (Project: Display_a_map). Replace the contents of the file with the following code:
build.gradle (Project: Display_a_map)Use dark colors for code blocks Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line // Top-level build file where you can add configuration options common to all sub-projects/modules. plugins { id 'com.android.application' version '7.2.1' apply false id 'com.android.library' version '7.2.1' apply false id 'org.jetbrains.kotlin.android' version '1.7.0' apply false } task clean(type: Delete) { delete rootProject.buildDir }
-
From the Project tool window, open Gradle Scripts > build.gradle (Module: Display_a_map.app). Replace the contents of the file with the following code:
build.gradle (Module: app)Use dark colors for code blocks Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line plugins { id 'com.android.application' id 'org.jetbrains.kotlin.android' } android { compileSdkVersion 32 defaultConfig { applicationId "com.example.app" minSdkVersion 23 targetSdkVersion 32 versionCode 1 versionName "1.0" } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro' } } compileOptions { sourceCompatibility JavaVersion.VERSION_1_8 targetCompatibility JavaVersion.VERSION_1_8 } kotlinOptions { jvmTarget = JavaVersion.VERSION_1_8.toString() } buildFeatures { viewBinding true } packagingOptions { exclude 'META-INF/DEPENDENCIES' } } dependencies { implementation fileTree(dir: "libs", include: ["*.jar"]) implementation 'androidx.appcompat:appcompat:1.4.2' implementation 'androidx.constraintlayout:constraintlayout:2.1.4' implementation 'androidx.multidex:multidex:2.0.1' implementation 'com.google.android.material:material:1.6.1' implementation 'com.esri.arcgisruntime:arcgis-android:100.15.0' }
-
From the Project tool window, open Gradle Scripts > settings.gradle. Replace the contents of the file with the following code:
settings.gradle (Display a map)Use dark colors for code blocks Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line Change line import org.gradle.api.initialization.resolve.RepositoriesMode pluginManagement { repositories { gradlePluginPortal() google() mavenCentral() } } dependencyResolutionManagement { repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS) repositories { google() mavenCentral() maven { url 'https://esri.jfrog.io/artifactory/arcgis' } } } rootProject.name = "Display a map" include ':app'
-
Sync the Gradle changes. Click the Sync now prompt or click the refresh icon (Sync Project with Gradle Files) in the toolbar. This may take several minutes.
-
From the Project tool window, open app > manifests > AndroidManifest.xml. Update the Android manifest to allow network access, and also to indicate the app uses OpenGL 2.0 or above.
Insert these new elements within the
manifest
element. Do not alter or remove any other statements.Depending on what ArcGIS functionality you add in future tutorials, it is likely you will need to add additional permissions to your manifest.
AndroidManifest.xmlUse dark colors for code blocks Add line. Add line. Add line. Add line. <manifest xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" package="com.example.app"> <uses-permission android:name="android.permission.INTERNET" /> <uses-feature android:glEsVersion="0x00020000" android:required="true" />
Add import statements
Open app > java > com.example.app MainActivity.kt, and add import statements to reference the ArcGIS Runtime API.
package com.example.app
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import com.esri.arcgisruntime.ArcGISRuntimeEnvironment
import com.esri.arcgisruntime.mapping.ArcGISMap
import com.esri.arcgisruntime.mapping.BasemapStyle
import com.esri.arcgisruntime.mapping.Viewpoint
import com.esri.arcgisruntime.mapping.view.MapView
import com.example.app.databinding.ActivityMainBinding
class MainActivity : AppCompatActivity() {
Add a UI for the map view
A map view is a UI component that displays a map. It also handles user interactions with the map, including navigating with touch gestures. Use XML to add a map view to the UI and make it available to the main activity source code.
-
In app > res > layout > activity_main.xml, replace the entire
Text
element with aView Map
element.View If you do not see the XML code, select the Code tab to switch out of design mode and display the XML code in the editor.
Your
Map
element creates an instance of theView Map
class from the ArcGIS Runtime API for Android.View In your main activity source code, you can access that
Map
instance using an implicit property, which is declared in the value of theView android:
attribute. In this case, the property will be namedid map
.View activity_main.xmlUse dark colors for code blocks Change line Change line Change line Change line Change line Change line Change line Change line <?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <com.esri.arcgisruntime.mapping.view.MapView android:id="@+id/mapView" android:layout_width="0dp" android:layout_height="0dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> </androidx.constraintlayout.widget.ConstraintLayout>
-
In MainActivity.kt, create a read-only local variable named
activity
referencing the generated Android classMain Binding Activity
.Main Binding MainActivity.ktUse dark colors for code blocks Add line. Add line. Add line. class MainActivity : AppCompatActivity() { private val activityMainBinding by lazy { ActivityMainBinding.inflate(layoutInflater) }
-
Create a read-only variable named
map
and bind it to theView map
created in activity_main.xml.View MainActivity.ktUse dark colors for code blocks Add line. Add line. Add line. class MainActivity : AppCompatActivity() { private val activityMainBinding by lazy { ActivityMainBinding.inflate(layoutInflater) } private val mapView: MapView by lazy { activityMainBinding.mapView }
Add a map
Use the map view to display a map centered on the Santa Monica Mountains in California. The map will contain a topographic basemap layer.
-
In MainActivity.kt, add a new
setup
method in yourMap() Main
class. In the method, create an ArcGISMap.Activity Configure
ArcGISMap
using a specific basemap style namedARCGIS_
.TOPOGRAPHIC MainActivity.ktUse dark colors for code blocks // set up your map here. You will call this method from onCreate() private fun setupMap() { // create a map with the BasemapStyle streets val map = ArcGISMap(BasemapStyle.ARCGIS_TOPOGRAPHIC) }
-
Set the
map
property ofmap
to the newView ArcGISMap
. Then create aView
and add it to thePoint map
.View MainActivity.ktUse dark colors for code blocks // set up your map here. You will call this method from onCreate() private fun setupMap() { // create a map with the BasemapStyle streets val map = ArcGISMap(BasemapStyle.ARCGIS_TOPOGRAPHIC) // set the map to be displayed in the layout's MapView mapView.map = map // set the viewpoint, Viewpoint(latitude, longitude, scale) mapView.setViewpoint(Viewpoint(34.0270, -118.8050, 72000.0)) }
-
In the
o
method, replace the Android Studio default calln Create() set
withContent View(R.layout.activity_ main) set
. Then callContent View(activity Main Binding.root) setup
.Map() Creating a new Android Studio project for Kotlin or Java automatically uses
set
. You can run the map view from theContent View(R.layout.activity_ main) activity_
layout, but this requires a separate extension in the gradle script.main MainActivity.ktUse dark colors for code blocks Change line Add line. override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(activityMainBinding.root) setupMap() }
-
Override the
o
,n Pause() o
, andn Resume() o
methods of then Destroy() Main
class.Activity The
Map
needs to know when your app goes to the background or is restored from background in order to properly manage the view. When the view is no longer needed, its resources should be released with a call to dispose.View You can place these methods anywhere inside your
Main
class definition.Activity MainActivity.ktUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. override fun onPause() { mapView.pause() super.onPause() } override fun onResume() { super.onResume() mapView.resume() } override fun onDestroy() { mapView.dispose() super.onDestroy() }
Set your API key
An API Key enables access to services, web maps, and web scenes hosted in ArcGIS Online.
-
Go to your developer dashboard to get your API key. For these tutorials, use your default API key. It is scoped to include all of the services demonstrated in the tutorials.
-
Create the
set
method, in which you set theA p i K e y F o r App() api
property on theKey ArcGISRuntime
using the API Key. Paste your API key inside the quotes, replacing YOUR_API_KEY.Environment MainActivity.ktUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. override fun onDestroy() { mapView.dispose() super.onDestroy() } private fun setApiKeyForApp(){ // set your API key // Note: it is not best practice to store API keys in source code. The API key is referenced // here for the convenience of this tutorial. ArcGISRuntimeEnvironment.setApiKey("YOUR_API_KEY") }
-
Call
set
in theA p i K e y F o r App() o
lifecycle method.n Create() MainActivity.ktUse dark colors for code blocks Add line. override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(activityMainBinding.root) setApiKeyForApp() setupMap() }
-
Click Run > Run > app to run the app.
The Android Emulator should display and run your app in the Android Virtual Devcie (AVD) selected in the Android Studio toolbar:
If your app builds but no AVD displays, you need to add one. Click Tools > AVD Manager > Create Virtual Device...
You should see a map with the topographic basemap layer centered on the Santa Monica Mountains in California. Pinch, drag, and double-tap the map view to explore the map.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: