Introduction
ArcGIS pretrained models automate the task of digitizing and extracting geographical features from imagery and point cloud datasets. Manually extracting features from raw data, such as digitizing cars, is time consuming. Deep learning automates the process and minimizes the manual interaction necessary to complete these tasks. However, training a deep learning model can be complicated as it needs large amounts of data, computing resources, and knowledge of how deep learning works.
With ArcGIS pretrained models, we do not need to invest time and energy into training a deep learning model. The ArcGIS models have been trained on data from a variety of geographies and work well across them. These pretrained models are available on ArcGIS Living Atlas of the World to anyone with an ArcGIS account.
Car Detection-USA is used to detect cars in high-resolution drone or aerial imagery. Car detection can be used for applications such as traffic management and analysis, parking lot utilization, urban planning, and more. It can also be used as a proxy for deriving economic indicators and estimating retail sales. High-resolution aerial and drone imagery can be used for car detection due to its high spatiotemporal coverage.
Necessary imports
import arcgis
from arcgis.gis import GIS
from arcgis.learn import detect_objects
from arcgis.raster.functions import equal_to
import pandas as pd
from datetime import datetime as dt
from ipywidgets import HBox, VBox, Label, Layout
Connect to your GIS
gis = GIS("home")
First, search for your imagery layer in ArcGIS Online. We can search for content shared by users outside our organization by setting outside_org
to True.
Get data and model for analysis
We have downloaded aerial imagery from OpenAerialMap and published it as a dynamic imagery layer, allowing us to get it below.
We will use three items for our analysis. We can get the uploaded imageries using their item id.
site_1 = gis.content.get('3409a289b12740c1a8cd0aea6a6da409')
site_2 = gis.content.get('29ddf4b2463c4a77a79197d6d2f97801')
site_3 = gis.content.get('84ca1eb102164e1c9d2208dc4efdce6e')
To count the detected cars in multiple imageries, create a list of imageries.
imagery_list = [site_1, site_2, site_3]
Visualize the imagery using layers attribute and indexing.
imagery_list[2].layers[0]
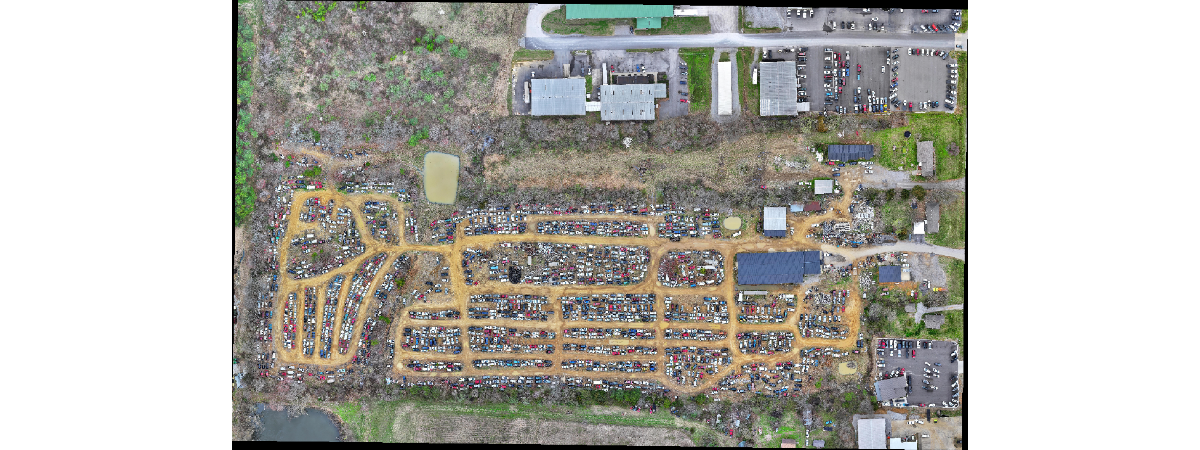
Search for the pretrained model item in ArcGIS Living Atlas of the World
.
model = gis.content.search(
"Car Detection-USA owner: esri_analytics", "Deep Learning Package", outside_org=True
)[0]
model
Detect and count cars
The following code runs detect_objects
function over each imagery and calculates the total count of cars detected. It then stores the item name and and its cars count in a dictionary named num_cars
.
Create a dictionary num_cars
to save the number of cars detected in each imagery.
num_cars = {}
Create a for loop to iterate through each imagery.
for i in range(len(imagery_list)):
# Get the imagery using indexing
raster = imagery_list[i].layers[0]
# Call the function detect_objects and assign values to its parameters
detected_cars = detect_objects (
# The input image used to detect objects.
input_raster = raster,
# The deep learning model to use to detect objects.
model = model,
# The output detected features file name.
output_name = "detected_cars" + str(dt.now().microsecond),
# The name value pairs of arguments and their values that can be customized by the clients.
model_arguments = {
"padding": "100",
"batch_size": "16", # Change batch size as per GPU specifications
"threshold": "0.9", # Get detections greater than 90% confidence
"return_bboxes": "False",
"tile_size": "224",
},
# Context allows to set environment settings that affect task operation.
context = {
"processorType": "GPU" # The specified processor (CPU or GPU) will be used for the analysis.
}
)
# Save the number of cars detected in an imagery with its file name before iterating to next imagery.
num_cars[imagery_list[i].name] = len(detected_cars.query(as_df=True))
Visualize detections on map
Let's visualize the detected cars in one of the aerial images.
# Create a map object
map1 = gis.map()
# Add input imagery to map object
map1.add_layer(imagery_list[2])
# Create another map object to see the detected features simultaneously
map2 = gis.map()
# Add both input imagery and detected features to second map object
map2.add_layer(imagery_list[2])
map2.add_layer(detected_cars)
Sync both the maps.
map1.sync_navigation(map2)
Create layout to visualize both the map objects. Keep running the below cells. Maps will automatically update here.
hbox_layout = Layout()
hbox_layout.justify_content = "space-around"
hb1 = HBox([Label("Input Imagery"), Label("Input Imagery with Detected Features")])
hb1.layout = hbox_layout
VBox([hb1, HBox([map1, map2])])

Zoom to the extent of detected features using zoom_to_layer
attribute
map1.zoom_to_layer(detected_cars)
Set the extent of the map to your desired extent.
map1.extent = {
'spatialReference': {'latestWkid': 3857, 'wkid': 102100},
'xmin': -9868132.37436656,
'ymin': 4407536.042181445,
'xmax': -9868058.923159715,
'ymax': 4407595.758609775
}
Now you must be seeing the added imagery layer and detected features over the map in the desired extent.
Check the count of cars in each imagery by displaying the output of num_cars
dictionary created in above steps.
num_cars # shows number of cars detected in each imagery layer.
{'car_image1': 780, 'car_image_2': 83, 'car_image_3': 1744}
Conclusion
This sample demonstrated how Car Detection-USA pretrained model can be used to detect cars in an aerial imagery and get the cars count in an area.
References
- Imagery from OpenAerialMap is licensed under CC-BY 4.0