Learn how to import data into your portal using ArcGIS API for Python.
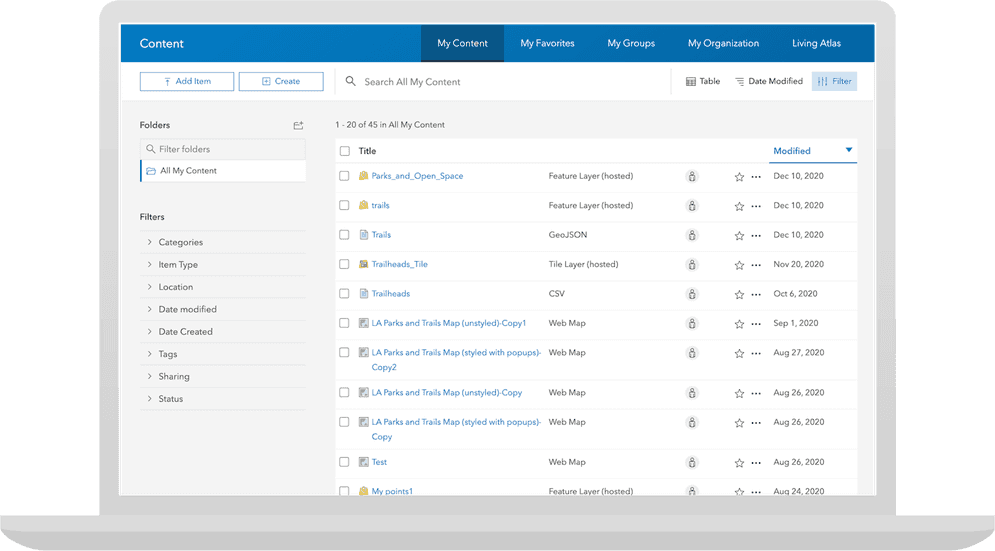
With an ArcGIS Online account, ArcGIS Location Platform account, or ArcGIS Enterprise account you can upload geographic data to your portal in several formats, including CSV, XLS, GPX, GeoJSON, or Shapefiles. Once imported, you can publish your data as a feature layer, and then call the underlying ArcGIS REST Feature Service to edit and make spatial queries on that layer.
Initially your feature layer is private, but you can change the sharing level of the layer in your portal (only for ArcGIS Online and ArcGIS Enterprise accounts).
With the ArcGIS API for Python you can automate the process of importing and then publishing feature data as a web layer. The import process is a fast and easy way to turn static data into live web services that can be displayed, filtered, and edited within your applications.
In this tutorial, you will import different file types as items stored in ArcGIS.
Prerequisites
The ArcGIS API for Python tutorials use Jupyter Notebooks to execute Python code. If you are new to this environment, please see the guide to install the API and use notebooks locally.
Be sure you have completed the Download data tutorial or have downloaded the LA_Hub_Datasets from ArcGIS Online.
Steps
Import modules and log in
-
Use the
GIS
class to log in to your portal using your ArcGIS Location Platform account, ArcGIS Online account, or ArcGIS Enterprise account.Use dark colors for code blocks from arcgis.gis import GIS, ItemProperties, ItemTypeEnum portal = GIS(username="<YOUR_USERNAME>")
Import the Trailheads CSV file
-
Create an
Item
object to store the metadata for the CSV file. Use the fieldsProperties title
,description
,tags
, anditem
._type Use dark colors for code blocks portal = GIS(username="<YOUR_USERNAME>") trailhead_item_properties = ItemProperties( item_type = ItemTypeEnum.CSV, title="Trailheads", description = "Trailheads CSV file imported using the ArcGIS API for Python", tags="la, trailheads, csv, python, los, angeles" ) trailhead_item_properties
-
Upload the file by calling the
add
method on theFolder
class. Pass in the local path of the file and theItem
object. TheProperties add
method is run asynchronously, so it return aFuture
object. Use theresult()
method to return the resultingItem
object.Use dark colors for code blocks trailhead_item_properties = ItemProperties( item_type = ItemTypeEnum.CSV, title="Trailheads", description = "Trailheads CSV file imported using the ArcGIS API for Python", tags="la, trailheads, csv, python, los, angeles" ) trailhead_item_properties csv_file = './data/LA_Hub_datasets/LA_Hub_datasets/Trailheads.csv' # get the root folder, i.e. "MyContent" > "All my content" folder = portal.content.folders.get() folder csv_item = folder.add(item_properties = trailhead_properties, file = csv_file).result()
-
Call the
publish
method on the returnedItem
to publish a feature layer. The results will be a newItem
representing the published service.Use dark colors for code blocks trailhead_item_properties = ItemProperties( item_type = ItemTypeEnum.CSV, title="Trailheads", description = "Trailheads CSV file imported using the ArcGIS API for Python", tags="la, trailheads, csv, python, los, angeles" ) trailhead_item_properties csv_file = './data/LA_Hub_datasets/LA_Hub_datasets/Trailheads.csv' # get the root folder, i.e. "MyContent" > "All my content" folder = portal.content.folders.get() folder csv_item = folder.add(item_properties = trailhead_properties, file = csv_file).result() trailhead_service = csv_item.publish() trailhead_service.url
Import the Trails GeoJSON file
-
Create an
Item
object to store the metadata for the GeoJSON file. Use the fieldsProperties title
,description
,tags
, anditem
._type Use dark colors for code blocks trailhead_service = csv_item.publish() trailhead_service.url trails_properties = ItemProperties( title = "Trails", description = "Trails GeoJSON file imported using the ArcGIS API for Python", tags = "la, trails, geojson, python, los, angeles", item_type = ItemTypeEnum.GEOJSON ) trails_properties
-
Upload the local GeoJSON file by calling the
add
method on theFolder
class. Pass in the local file path and theItem
object. Since the add method runs asynchronously, it will return aProperties Future
object. Use theresult()
method to access the resultingItem
object.Use dark colors for code blocks trails_properties = ItemProperties( title = "Trails", description = "Trails GeoJSON file imported using the ArcGIS API for Python", tags = "la, trails, geojson, python, los, angeles", item_type = ItemTypeEnum.GEOJSON ) trails_properties geojson_file = './data/LA_Hub_datasets/LA_Hub_datasets/Trails.geojson' geojson_item = folder.add(item_properties = trails_properties, file = geojson_file).result()
-
Call the
publish
method on theItem
to publish the service. This will return a newItem
representing the published service.Use dark colors for code blocks trails_properties = ItemProperties( title = "Trails", description = "Trails GeoJSON file imported using the ArcGIS API for Python", tags = "la, trails, geojson, python, los, angeles", item_type = ItemTypeEnum.GEOJSON ) trails_properties geojson_file = './data/LA_Hub_datasets/LA_Hub_datasets/Trails.geojson' geojson_item = folder.add(item_properties = trails_properties, file = geojson_file).result() trails_service = geojson_item.publish() trails_service
Import the Parks and Open Spaces Shapefile
-
Create an
Item
object to store the metadata for the ZIP file. Use the fieldsProperties title
,description
,tags
, anditem
._type Use dark colors for code blocks trails_service = geojson_item.publish() trails_service parks_properties = { 'title': 'Parks and Open space', 'tags':'parks, open data, tutorials', 'type': 'Shapefile' }
-
Upload the local ZIP file by calling the
add
method on theFolder
class. Pass in the local file path and theItem
object. Since the add method runs asynchronously, it will return aProperties Future
object. Use theresult()
method to access the resultingItem
object.Use dark colors for code blocks parks_properties = { 'title': 'Parks and Open space', 'tags':'parks, open data, tutorials', 'type': 'Shapefile' } zip_file = './data/Parks_and_Open_Space.zip' # relative path to notebook shp_item = folder.add(item_properties = parks_properties, file = zip_file).result() shp_item
-
Call the
publish
method on the returnedItem
to publish the service. This will return newItem
representing the published service.Use dark colors for code blocks parks_properties = { 'title': 'Parks and Open space', 'tags':'parks, open data, tutorials', 'type': 'Shapefile' } zip_file = './data/Parks_and_Open_Space.zip' # relative path to notebook shp_item = folder.add(item_properties = parks_properties, file = zip_file).result() shp_item parks_service = shp_item.publish() parks_service
Identify the Item Ids and URLs
-
Use the
url
andid
properties of each of the published service items to get the item ID and service URL. You can use these to access your data in other tutorials and projects.Use dark colors for code blocks print(f"Trailheads (points) item id: {trailhead_service.id} , feature layer url : {trailhead_service.url}") print(f"Trails (polylines) item id: {trails_service.id}, feature layer url: {trails_service.url}") print(f"Parks (polygons) item id: {parks_service.id}, feature layer url: {parks_service.url}")
What's next?
Learn how to use additional functionality in these tutorials:

Download data
Automate downloading data from the cloud using the ArcGIS API for Python.
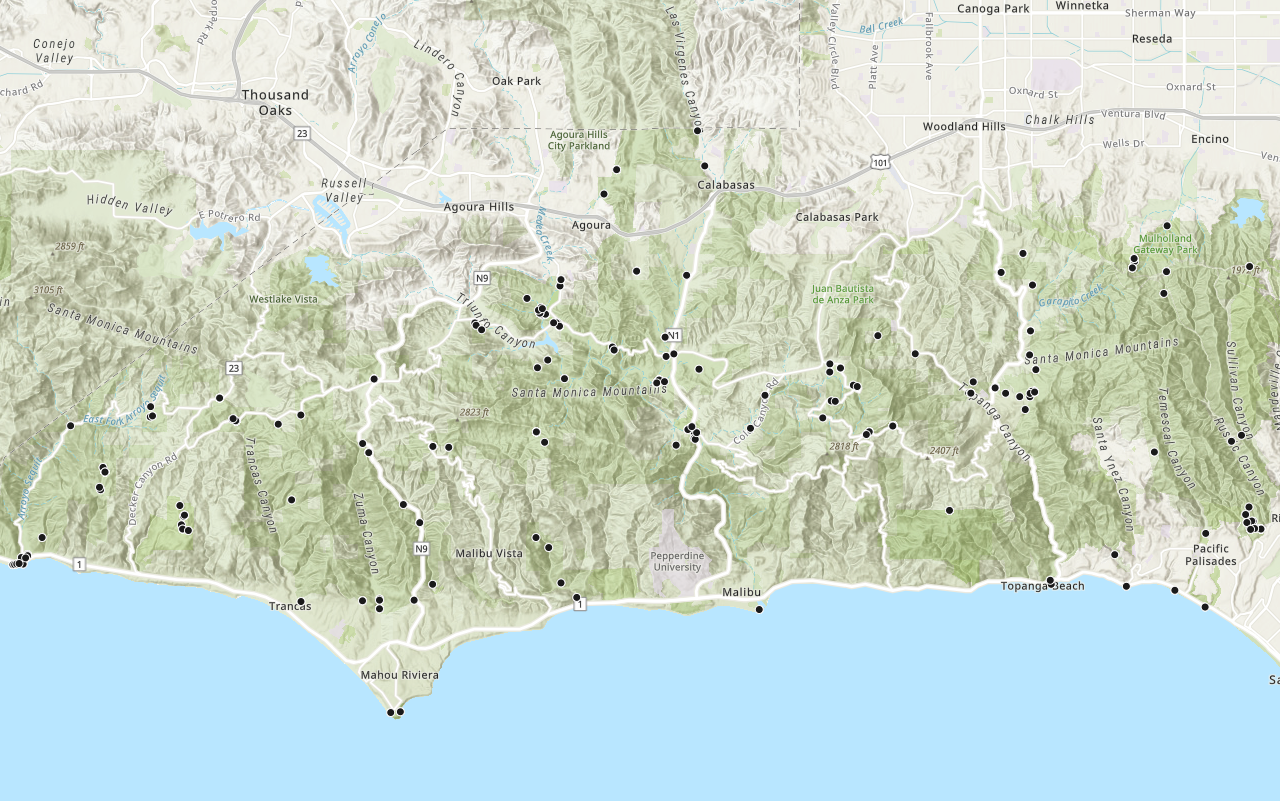
Add a layer from a portal item
Learn how to use a portal item to access and display point features from a feature service.
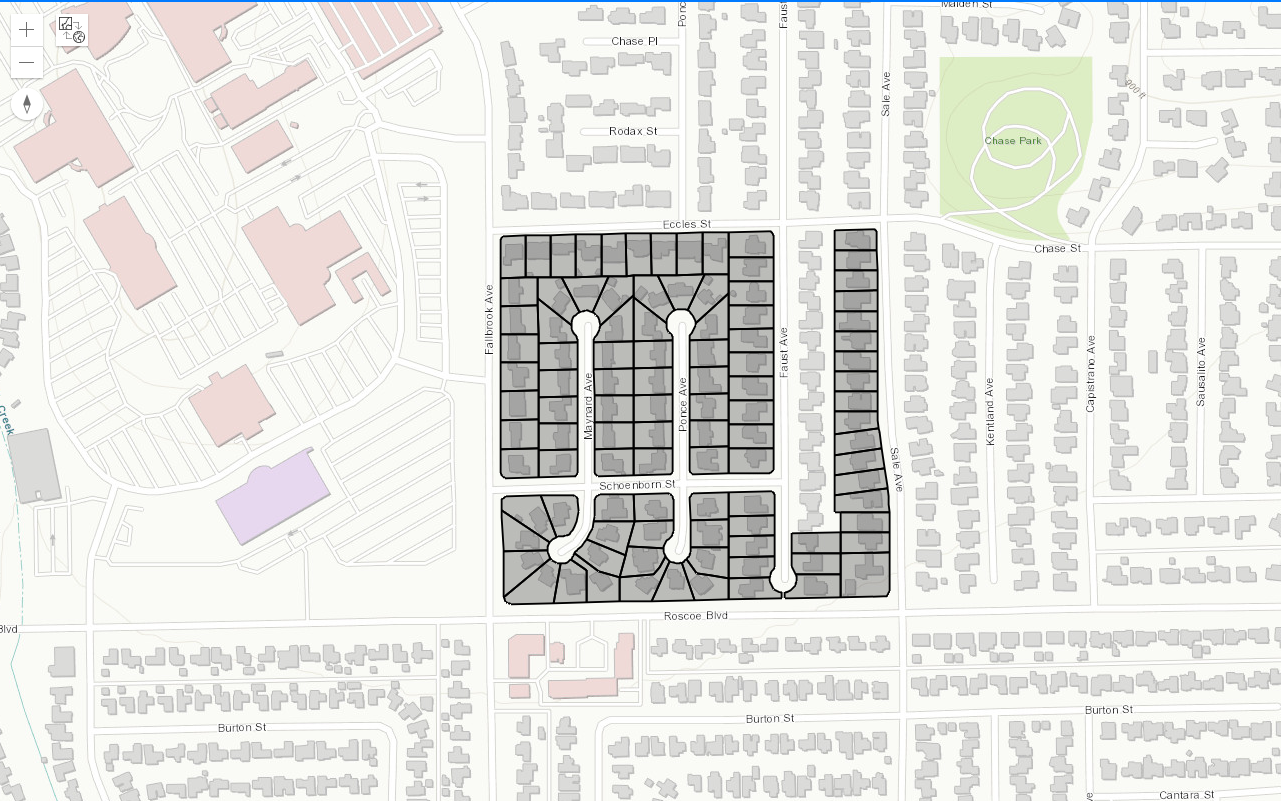
Query a feature layer (SQL)
Learn how to execute a SQL query to access polygon features from feature services.