require(["esri/symbols/CartographicLineSymbol"], function(CartographicLineSymbol) { /* code goes here */ });
Description
(Added at v1.0)
Line symbols are used to draw linear features on the graphics layer. CartographicLineSymbol, like
SimpleLineSymbol, is either a solid line or a predefined pattern of dashes and dots. Line joins and line caps can be added to further define the line symbol.
Explore the CartographicLineSymbol in the
ArcGIS Symbol Playground. This is a place to explore and learn how to work with various properties and methods before implementing it into custom code. Try out new features, customize them, and copy the generated code into your own application. This sample provides a starting point so as to allow use of these features as quickly as possible.
Samples
Search for
samples that use this class.
Class hierarchy
esri/symbols/Symbol
|_esri/symbols/LineSymbol
|_esri/symbols/SimpleLineSymbol
|_esri/symbols/CartographicLineSymbol
Constructors
Constants
CAP_BUTT | Line ends square at the end point.
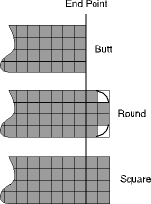 |
CAP_ROUND | Line is rounded just beyond the end point. |
CAP_SQUARE | Line is squared just beyond the end point. |
JOIN_BEVEL | The joined lines are beveled.
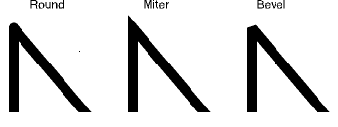 |
JOIN_MITER | The joined lines are not rounded or beveled. |
JOIN_ROUND | The joined lines are rounded. |
STYLE_DASH | The line is made of dashes. |
STYLE_DASHDOT | The line is made of a dash-dot pattern. |
STYLE_DASHDOTDOT | The line is made of a dash-dot-dot pattern. |
STYLE_DOT | The line is made of dots. |
STYLE_LONGDASH | The line is made of a long dash pattern. |
STYLE_LONGDASHDOT | The line is made of a long dash-dot pattern. |
STYLE_NULL | The line has no symbol. |
STYLE_SHORTDASH | The line is made of a short dash pattern. |
STYLE_SHORTDASHDOT | The line is made of a short dash-dot pattern. |
STYLE_SHORTDASHDOTDOT | The line is made of a short dash-dot-dot pattern. |
STYLE_SHORTDOT | The line is made of a short dot pattern. |
STYLE_SOLID | The line is solid. |
Properties
Methods
Constructor Details
Creates a new empty CartographicLineSymbol object.
Sample:
require([
"esri/symbols/CartographicLineSymbol", ...
], function(CartographicLineSymbol, ... ) {
var cls = new CartographicLineSymbol();
...
});
Creates a new CartographicLineSymbol object with parameters.
Parameters:
<String > style |
Optional |
See Constants table for values. |
<Color > color |
Optional |
Symbol color. |
<Number > width |
Optional |
Width of the line in pixels. |
<String > cap |
Optional |
See Constants table for values. |
<String > join |
Optional |
See Constants table for values. |
<String > miterLimit |
Optional |
Size threshold for showing mitered line joins. |
Sample:
require([
"esri/symbols/CartographicLineSymbol", "esri/Color", ...
], function(CartographicLineSymbol, Color, ... ) {
var cls = new CartographicLineSymbol(CartographicLineSymbol.STYLE_SOLID, new Color([255,0,0]), 10, CartographicLineSymbol.CAP_ROUND, CartographicLineSymbol.JOIN_MITER, 5);
...
});
Creates a new CartographicLineSymbol object using a JSON object.
Parameters:
<Object > json |
Required |
JSON object representing the CartographicLineSymbol. View the Symbol Objects (Common data types in ArcGIS) for details
on creating a JSON symbol. Note that when specifying symbol width and height using JSON the values should be entered in points, the JavaScript API then converts the point values to pixels. |
Property Details
The cap style. See the Constants table for valid values and explanations.
Known values: CAP_BUTT|CAP_ROUND|CAP_SQUARE
Default value: CAP_BUTT
The join style. See the Constants table for valid values and explanations.
Known values: JOIN_BEVEL|JOIN_MITER|JOIN_ROUND
Default value: JOIN_MITER
Indicates marker symbols present at the beginning and/or end of a SimpleLineSymbol. See the object specification table in the
setMarker() method for details of the properties of this object.
(Added at v3.23)
Size threshold for showing mitered line joins.
Default value: 10
The line style. See the Constants table for valid values.
Default value: STYLE_SOLID
The type of symbol.
Known values: simplemarkersymbol | picturemarkersymbol | simplelinesymbol | cartographiclinesymbol | simplefillsymbol | picturefillsymbol | textsymbol
Width of line symbol in pixels.
Default value: 1
Method Details
Sets the cap style.
Parameters:
<String > cap |
Required |
Cap style. See the Constants table for valid values. |
Sets the symbol color.
Parameters:
<Color > color |
Required |
Symbol color. |
Sample:
require([
"esri/Color", ...
], function(Color, ... ) {
symbol.setColor(new Color([255,255,0,0.5]));
...
});
Sets the join style.
Parameters:
<String > join |
Required |
Join style. See the Constants table for valid values. |
Sets marker symbols at the beginning and/or end of a SimpleLineSymbol. (Added at v3.23)
Parameters:
<Object > options |
Required |
The options defining the marker style and placement. See the object specification table below. |
Object Specifications: <options
>
<String > placement |
Required |
Indicates where the marker is placed on the line symbol. Valid values are begin , end , and begin-end . |
<String > style |
Required |
Indicates the style of the marker to place on the line symbol. As of version 3.23, the only supported style is arrow . |
Sample:
lineSymbol.setMarker({
style: "arrow",
placement: "end"
});
Sets the size threshold for showing mitered line joins.
Parameters:
<String > miterLimit |
Required |
Miter limit. |
Sets the line symbol style.
Parameters:
<String > style |
Required |
Line style. See the Constants table for valid values. |
Sets the LineSymbol width.
Parameters:
<Number > width |
Required |
Width of line symbol in pixels. |
Sample:
require([
"esri/symbols/SimpleLineSymbol", ...
], function(SimpleLineSymbol, ... ) {
var outline = new SimpleLineSymbol().setWidth(1);
...
});
Converts object to its ArcGIS Server JSON representation.