Geocode Address
The geocode()
function supports searching for places and addresses either in a single field format or in a multi-field format with the address components separated into multiple parameters.
The code below imports the geocode
function and displays its signature and parameters, along with a brief description:
from arcgis.geocoding import geocode
help(geocode)
Help on function geocode in module arcgis.geocoding._functions: geocode(address, search_extent=None, location=None, distance=None, out_sr=None, category=None, out_fields='*', max_locations=20, magic_key=None, for_storage=False, geocoder=None, as_featureset=False, match_out_of_range=True, location_type='street', lang_code=None, source_country=None)
Single-line address
geocode()
can be called with one required parameter address
or can be used with optional parameters to fine-tune the search results such as search_extent
, as seen in this example.
- The
address
parameter specifies the location to be geocoded. This can be a string containing the single line address, i.e street address, place name, postal code, or POI.
from arcgis.gis import GIS
gis = GIS(profile = "your_enterprise_profile") # log in with your own enterprise or AGOL profile configuration
As seen in Part 1, geocode
performed upon a single line address returns matched geocoded results. The more details provided onto the address string, the more refined result set becomes.
single_line_address = "380 New York Street, Redlands, CA 92373"
# geocode the single line address
esrihq = geocode(single_line_address)
len(esrihq)
1
If we loosen up on the single-line address definition, e.g. remove the city and state name, then the length of the returned result set increases to 20.
single_line_address = "380 New York Street"
# geocode the single line address
esrihq = geocode(single_line_address)
len(esrihq)
20
Example of World Series POI
Another example of such is to define the single line address of search to be Disneyland, USA
, as compared to Disneyland
. The former returns matched results in USA only while the latter is returning all in the world.
disney = geocode("Disneyland, USA")
len(disney)
3
for i in range(3):
print(disney[i]['attributes']['LongLabel'])
print(" - ",disney[i]['attributes']['Score'],
" - ", disney[i]['attributes']['Addr_type'],
" - ", disney[i]['attributes']['Type'])
Disneyland, 1313 S Disneyland Dr, Anaheim, CA, 92802, USA - 100 - POI - Amusement Park Disneyland, Anaheim, CA, USA - 100 - Locality - Neighborhood Disneyland, 1313 S Harbor Blvd, Anaheim, CA, 92802, USA - 100 - POI - Business Facility
disney = geocode("Disneyland")
len(disney)
20
for i in range(20):
if disney[i]['attributes']['Type']== "Amusement Park":
print(disney[i]['attributes']['LongLabel'])
print(" - ",disney[i]['attributes']['Score'],
" - ", disney[i]['attributes']['Addr_type'])
Disneyland, 1313 S Disneyland Dr, Anaheim, CA, 92802, USA - 100 - POI Disney Land, 7th Street, Gandhipuram, Coimbatore, Tamil Nadu, 641012, IND - 100 - POI Disney Land, Kalawad Road, Mota Mava, Rajkot, Gujarat, 360005, IND - 100 - POI Disneyland, Boulevard du Parc, 77700, Coupvray, Seine-et-Marne, Ile-de-France, FRA - 100 - POI
Multi-field address
Alternatively, the address can be specified in a multi-field format using a dict
containing the various address fields accepted by the corresponding geocoding service.
In order to provide a way to find addresses in many different countries, which may use different addressing formats, the geocode()
method uses standardized field names for submitting address components. In understanding what specific fields are needed to enter for your address, you can check out the addressField
introduction for more information.
The Geocoder's address
field property specifies the various address fields accepted by it when geocoding addresses. The neighborhood, city, subregion, and region parameters represent typical administrative divisions within a country. They may have different contexts for different countries, and not all administrative divisions are used in all countries. For instance, with addresses in the United States, only the city
(city) and region
(state) parameters are used; for addresses in Mexico, the neighborhood
parameter is used for districts
(colonias) within a city, city
for municipalities
(municipios), and the region
parameter for states
(estados); Spain uses all four administrative divisions.
For example, if the address
field of a geocoding service resource includes fields with the following names: Address, City, Region and Postal
, then the address argument is of the form below.
multi_field_address = {
"Address" : "380 N Y St",
"City" : "Redlands",
"Region" : "CA",
"Postal" : 92373
}
# geocode the multi_field_address
esrihq1 = geocode(multi_field_address)
len(esrihq1)
2
The returned result set contains two dict
objects, and we can see from below that each dict
object contains 'address', 'location', 'score', 'attributes', and 'extent' keys. The x
and y
coordinates can be found in both location
and attributes
properties.
esrihq1[0].keys()
dict_keys(['address', 'location', 'score', 'attributes', 'extent'])
esrihq1[0]['location'], esrihq1[0]['extent']
({'x': -117.19568252432872, 'y': 34.05723700023128}, {'xmin': -117.19587199429185, 'ymin': 34.056237000231285, 'xmax': -117.19387199429184, 'ymax': 34.05823700023128})
We can also go one level deeper and check out the address type
, X
and Y
coordinates from its attributes
field for more details.
esrihq1[0]['attributes']['Addr_type'], esrihq1[0]['attributes']['X'], esrihq1[0]['attributes']['Y']
('PointAddress', -117.19568252432872, 34.05723700023128)
esrihq1[1]['attributes']['Addr_type'], esrihq1[1]['attributes']['X'], esrihq1[1]['attributes']['Y']
('StreetAddress', -117.18686179838916, 34.05653921319909)
Example of an Indian address
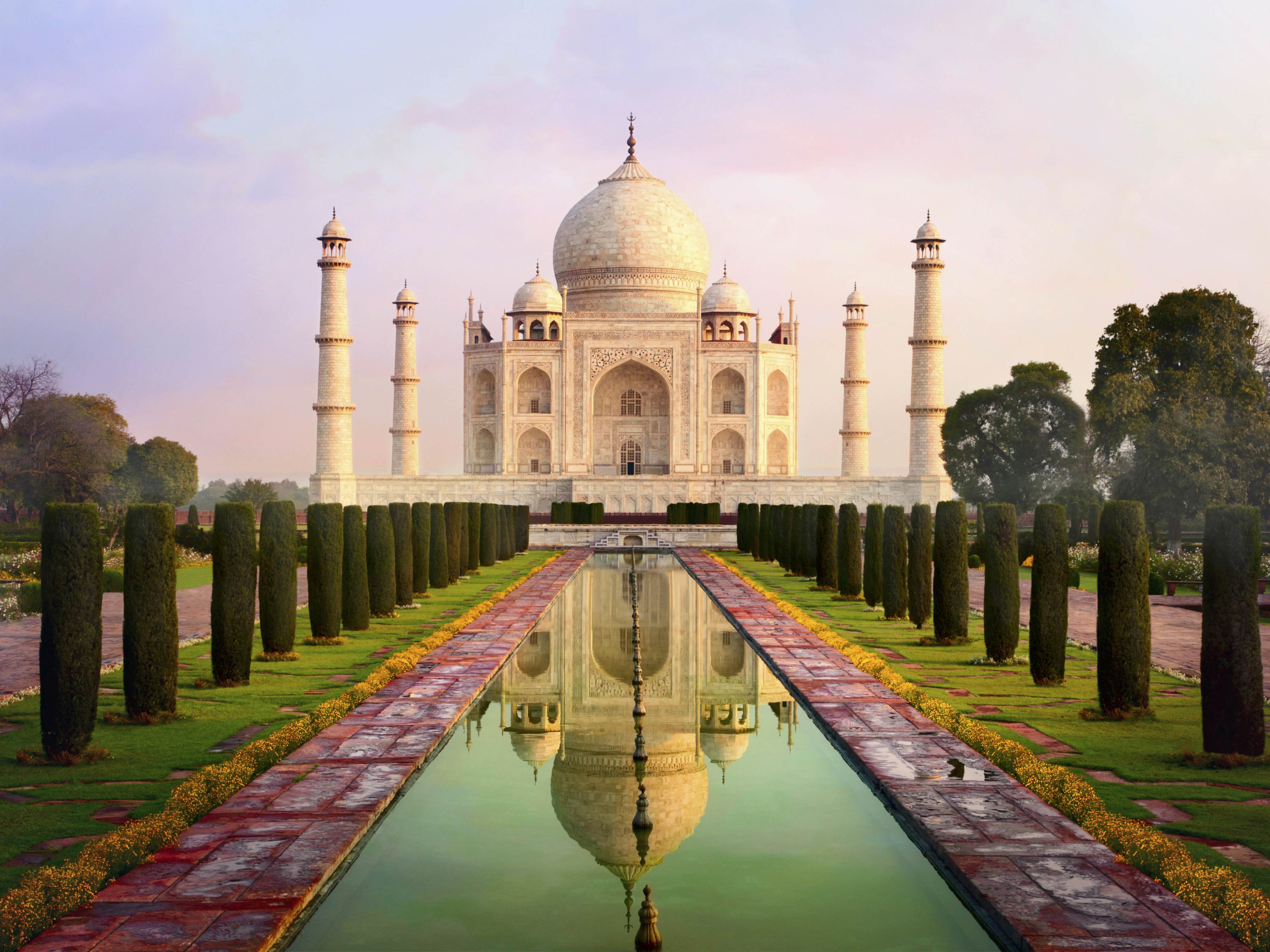
Photo of Taj Mahal (Source: Lonely Planet)
india_mfield_address = {
"Address" : "Taj Mahal",
"City": "Agra",
'District': 'Taj Ganj',
"Region" : 'Uttar Pradesh',
"Country": "IND",
"Postal" : 282001
}
# geocode the multi_field_address
taj_mahal = geocode(india_mfield_address)
len(taj_mahal)
7
Example of a Mexican address

Photo of San Miguel de Allende (Source: National Geography)
mex_mfield_address = {
"Address" : "San Miguel de Allende",
"City": 'San Miguel de Allende',
"Region" : 'Guanajuato',
"Country": "MEX"
}
# geocode the multi_field_address
san_miguel = geocode(mex_mfield_address)
len(san_miguel)
20
Example of a Spanish Address

Photo of Sagrada Familia (Source: https://www.culturalplaces.com/)
esp_mfield_address = {
"Address" : "Sagrada Familia",
'Nbrhd': 'Sagrada Familia',
'District': 'Barcelona',
'City': 'Barcelona',
'Region': 'Catalunya',
"Country": "ESP"
}
# geocode the multi_field_address
sag_fam = geocode(esp_mfield_address)
len(sag_fam)
20
Search for street intersections
The following example illustrates how to search for a street intersection. An intersection is where two streets cross each other, and hence an intersection search consists of the intersecting street names plus the containing administrative division or postal code. For example, redlands blvd and new york st 92373
is a valid intersection search, as is redlands blvd & new york st redlands ca
.
intersection = "redlands blvd and new york st 92373"
multi_field_intersection = {
"Address" : "redlands blvd & new york st",
"City" : "Redlands",
"Region" : "CA"
}
map2 = gis.map("Esri, Redlands, CA", 15)
map2

# geocode the intersection address and plot the location of the first geocode result on the map
# either of the two intersection address formats can be used, they give itentical results:
# intersection_result = geocode(intersection)[0]
intersection_result = geocode(multi_field_intersection)[0]
popup = {
"title" : "redlands blvd and new york st",
"content" : intersection_result['address']
}
map2.draw(intersection_result['location'], popup)
Understanding the geocoded result
The output fields
The geocode()
method returns a list of dict
object, and we can look at the first entry of the list (e.g. intersection_result
) to determine what the included keys of the dict
object are:
intersection_result.keys()
dict_keys(['address', 'location', 'score', 'attributes', 'extent'])
intersection_result['attributes'].keys()
dict_keys(['Loc_name', 'Status', 'Score', 'Match_addr', 'LongLabel', 'ShortLabel', 'Addr_type', 'Type', 'PlaceName', 'Place_addr', 'Phone', 'URL', 'Rank', 'AddBldg', 'AddNum', 'AddNumFrom', 'AddNumTo', 'AddRange', 'Side', 'StPreDir', 'StPreType', 'StName', 'StType', 'StDir', 'StPreDir1', 'StPreType1', 'StName1', 'StType1', 'StDir1', 'StPreDir2', 'StPreType2', 'StName2', 'StType2', 'StDir2', 'BldgType', 'BldgName', 'LevelType', 'LevelName', 'UnitType', 'UnitName', 'SubAddr', 'StAddr', 'Block', 'Sector', 'Nbrhd', 'District', 'City', 'MetroArea', 'Subregion', 'Region', 'RegionAbbr', 'Territory', 'Zone', 'Postal', 'PostalExt', 'Country', 'LangCode', 'Distance', 'X', 'Y', 'DisplayX', 'DisplayY', 'Xmin', 'Xmax', 'Ymin', 'Ymax', 'ExInfo'])
See below for the descriptions for all of the fields that can be returned by geocode()
:
address
: Complete matching address returned forfindAddressCandidates
andgeocodeAddresses
geocode requests.location
: The point coordinates of the output match location as specified by the x and y properties. The spatial reference of the x and y coordinates is defined by thespatialReference
output field. Always returned by default forfindAddressCandidates
andgeocodeAddresses
geocode requests only.score
: A number from 1–100 indicating the degree to which the input tokens in a geocoding request match the address components in a candidate record. A score of 100 represents a perfect match, while lower scores represent decreasing match accuracy.attributes
: Adict
object containingLoc_name
,Status
,Score
,Match_addr
,LongLabel
,ShortLabel
,Addr_type
etc. key-value pairs.extent
: the display extent of a feature returned by the geocoding service.
Read into a DataFrame
The previous example explained how to get results as a FeatureSet
using as_featureset = True
parameter, and map the FeatureSet
on the Map Widget. Next, we will convert the FeatureSet
to a DataFrame
and see how the results look.
disney_fset = geocode("Disneyland", as_featureset = True)
disney_fset
<FeatureSet> 20 features
disney_fset.sdf.head()
Loc_name | Status | Score | Match_addr | LongLabel | ShortLabel | Addr_type | Type | PlaceName | Place_addr | ... | Y | DisplayX | DisplayY | Xmin | Xmax | Ymin | Ymax | ExInfo | OBJECTID | SHAPE | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | World | M | 100 | Disneyland | Disneyland, 1313 S Disneyland Dr, Anaheim, CA,... | Disneyland | POI | Amusement Park | Disneyland | 1313 S Disneyland Dr, Anaheim, California, 92802 | ... | 33.81533 | -117.91895 | 33.81277 | -117.92395 | -117.91395 | 33.80777 | 33.81777 | 1 | {"x": -117.92369994070933, "y": 33.81532991704... | |
1 | World | M | 100 | Disneyland, Anaheim, California | Disneyland, Anaheim, CA, USA | Disneyland | Locality | Neighborhood | Disneyland | Anaheim, California | ... | 33.81530 | -117.92370 | 33.81530 | -117.93570 | -117.91170 | 33.80330 | 33.82730 | 2 | {"x": -117.92369999999994, "y": 33.81530000000... | |
2 | World | T | 100 | Disney Land | Disney Land, 7th Street, Gandhipuram, Coimbato... | Disney Land | POI | Amusement Park | Disney Land | 7th Street, Gandhipuram, Coimbatore, Tamil Nad... | ... | 11.01845 | 76.96622 | 11.01845 | 76.96122 | 76.97122 | 11.01345 | 11.02345 | 3 | {"x": 76.96622000000008, "y": 11.0184500000000... | |
3 | World | T | 100 | Disney Land | Disney Land, Guntur Road, Prakasam, Andhra Pra... | Disney Land | POI | Convention Center | Disney Land | Guntur Road, Prakasam, Andhra Pradesh, 523001 | ... | 15.51213 | 80.04665 | 15.51213 | 80.04165 | 80.05165 | 15.50713 | 15.51713 | 4 | {"x": 80.04665000000006, "y": 15.5121300000000... | |
4 | World | T | 100 | Disney Land | Disney Land, Kalawad Road, Mota Mava, Rajkot, ... | Disney Land | POI | Amusement Park | Disney Land | Kalawad Road, Mota Mava, Rajkot, Gujarat, 360005 | ... | 22.27105 | 70.74842 | 22.27105 | 70.74342 | 70.75342 | 22.26605 | 22.27605 | 5 | {"x": 70.74842000000007, "y": 22.2710500000000... |
5 rows × 59 columns
disney_fset.sdf.Addr_type
0 POI 1 Locality 2 POI 3 POI 4 POI 5 POI 6 POI 7 POI 8 POI 9 POI 10 POI 11 POI 12 POI 13 POI 14 POI 15 POI 16 POI 17 POI 18 POI 19 POI Name: Addr_type, dtype: object
disney_fset.sdf.Score
0 100 1 100 2 100 3 100 4 100 5 100 6 100 7 100 8 100 9 100 10 100 11 100 12 100 13 100 14 100 15 100 16 100 17 100 18 100 19 100 Name: Score, dtype: int64
Plot the accuracy of different results as a bar plot
Take the four entries shown in the disney_fset.sdf
for example. Since a score of 100 represents a perfect match, while lower scores represent decreasing match accuracy, the first entry is listed with the highest score, representing the best accuracy among all results.
import matplotlib.pyplot as plt
fig = plt.figure()
ax = fig.add_axes([0,0,1,1])
addrs = disney_fset.sdf.Match_addr
scores = disney_fset.sdf.Score
ax.bar(addrs,scores)
plt.show()

Advanced Options
Advanced options for the geocode()
method to be used for configuring and fine-tuning the search results are listed below:
- Limit searches to a set of bounding boxes (
search_extent
param) - Defining an origin point location of the search to be used with distance (
location
&distance
parameters) - Customizing number of geocode results (
max_locations
param) - Limit searches to exact address range (
match_out_of_range
param) - Limit searches to a particular country (
source_country
param) - Getting results in desired co-ordinate system (
out_sr
param) - Customizing output fields in the geocoded results (
out_fields
param) - Street coordinates vs Rooftop coordinates (
location_type
param) - Geocoding in languages other than English (
lang_code
) - Whether the results of the operation will be persisted (
for_storage
param) magic_key
param. More explanations can be found in part 7.
search_extent
parameter
search_extent
: An optional string of a set of bounding box coordinates that limit the search area to a specific region. This is especially useful for applications in which a user will search for places and addresses only within the current map extent.
You can specify the spatial reference of the search_extent
coordinates, which is necessary if the map spatial reference is different than that of the geocoding service; otherwise, the spatial reference of the coordinates is assumed to be the same as that of the geocoding service.
The input can either be a comma-separated list of coordinates defining the bounding box or a JSON envelope object. The spatial reference of the bounding box coordinates can be included if an envelope object is used.
as_featureset
parameter
as_featureset
: An optional boolean. If True, the result set is returned as a FeatureSet
object. Otherwise, it is a dictionary.
Example using search_extent
& etc.
The example below uses Esri headquarter's bbox as search_extent
, and the geocoded results got filtered down to 1 (from the original count of 20).
# geocode the single line address
esrihq_fset = geocode(single_line_address,
search_extent = {'xmin': -117.19587199429185,
'ymin': 34.056237000231285,
'xmax': -117.19387199429184,
'ymax': 34.05823700023128},
as_featureset = True)
esrihq_fset
<FeatureSet> 1 features
esrihq_fset.features[0].attributes["Match_addr"]
'380 New York St, Redlands, California, 92373'
map0 = gis.map("Redlands, CA")
map0

# plot the location of the first geocode result on the map
map0.draw(esrihq_fset)
location
parameter
location
: Optional [x,y] defining an origin point location that is used with the distance parameter to sort geocoding candidates based upon their proximity to the location.
The location
parameter defines an origin point location that is used with the distance parameter to sort geocoding candidates based upon their proximity to the location. The distance parameter specifies the radial distance from the location in meters. The priority of candidates within this radius is boosted relative to those outside the radius.
This is useful in mobile applications where a user will want to search for places in the vicinity of their current GPS location; the location and distance parameters can be used in this scenario.
The location parameter can be specified without specifying a distance. If distance is not specified, it defaults to 50000 meters.
The location can be represented with a simple comma-separated syntax (x,y), or as a JSON point object. If the comma-separated syntax is used, the spatial reference of the coordinates must be WGS84. Otherwise, the spatial reference of the point coordinates can be defined in the JSON object.
Example using simple syntax (WGS84): location=-117.196,34.056
JSON example with a spatial reference: location= { "x": -13046165.572, "y": 4036389.847, "spatialReference": { "wkid": 102100 } }
distance
parameter
The distance
parameter specifies the radius of an area around a point location that is used to boost the rank of geocoding candidates so that candidates closest to the location are returned first. The distance value is in meters.
If the distance parameter is specified, then the location parameter must be specified as well.
It is important to note that unlike the searchExtent parameter, the location and distance parameters allow searches to extend beyond the specified search radius. They are not used to filter results, but rather to rank resulting candidates based on their distance from a location. You must pass a searchExtent value in addition to location and distance if you want to confine the search results to a specific area.
Example of searching within two miles of the current extent: distance=3218.69
Example using location
and distance
# geocode the multi_field_address
esrihq1_fset = geocode(multi_field_address,
location={"x":-117.19568252432872, "y":34.05723700023128},
distance=0,
as_featureset=True)
esrihq1_fset
<FeatureSet> 4 features
map1 = gis.map("Redlands, CA")
map1

map1.draw(esrihq1_fset)
max_locations
parameter
The max_locations
parameter is the maximum number of locations to be returned by a search, up to the maximum number allowed by the geocoder. If not specified, then all matching candidates up to the maximum are returned.
The World Geocoding Service allows up to 20 candidates to be returned for a single request. Note that up to 50 POI candidates can be returned.
Example:
max_locations=10
category
parameter
The category
parameter is a place or address type which can be used to filter geocoding results. The parameter supports input of single category values or multiple comma-separated values. The category parameter can be passed in a request with or without a single line address input. More categories can be found in Part 1.
Example of category filtering with a single category:
category="Address"
Example of category filtering with multiple categories:
category="Address,Postal"
Note: The `category` parameter is only functional when used with single line address input. It does not work with multi field addresses; specifically the address, neighborhood, city, region, subregion, countryCode, and postal parameters.
unionsquare = geocode("Union Square, San Francisco, CA")[0]
map3 = gis.map(unionsquare)
map3

# find and plot upto 10 Indian restaurants around Union Square in San Francisco, CA
restaurants = geocode(None,
search_extent = unionsquare['extent'],
category="Indian Food",
max_locations=10)
for restaurant in restaurants:
popup = {
"title" : restaurant['address'],
"content" : "Phone: " + restaurant['attributes']['Phone']
}
map3.draw(restaurant['location'], popup)
for_storage
parameter
This parameter specifies whether the results of the operation will be persisted. The default value is False
, which indicates the results of the operation can't be stored, but they can be temporarily displayed on a map for instance. If you store the results, in a database for example, you need to set this parameter to True
.
Applications are contractually prohibited from storing the results of geocoding transactions unless they make the request by passing the for_storage
parameter with a value of true.
ArcGIS Online service credits are deducted from the organization account for each geocode transaction that includes the for_storage
parameter with a value of true. Refer to the ArcGIS Online service credits overview page for more information on how credits are charged.
To learn more about free and paid geocoding operations, see this help topic.
Example:
for_storage=True
starbucks_fset = geocode("Starbucks", unionsquare['extent'],
max_locations=10,
as_featureset=True,
for_storage=True)
starbucks_fset
<FeatureSet> 10 features
out_sr
parameter
The spatial reference of the x/y coordinates returned by the geocode method. This is useful for applications using a map with a spatial reference different than that of the geocoder.
The spatial reference can be specified as either a well-known ID (WKID) or as a JSON spatial reference object. If outSR is not specified, the spatial reference of the output locations is the same as that of the geocoder. The World Geocoding Service spatial reference is WGS84 (WKID = 4326).
For a list of valid WKID values, see Projected Coordinate Systems and Geographic Coordinate Systems.
Example (102100 is the WKID for the Web Mercator projection):
out_sr=102100
out_fields
parameter
The list of fields to be returned in the response. Descriptions for each of these fields are available in the Output fields section of this document.
The returned address, x/y coordinates of the match location, match score, spatial reference, extent of the output feature, and the addr_type (match level) are returned by default.
Example that returns all output fields:
out_fields=*
Example that returns the specified fields only:
out_fields="AddrNum,StName,City"
Example using out_sr
and out_fields
Next, let's look at an example in which we output the result set in a different spatial reference than the default sr
, and let's limit the output fields to be only containing Addr_type,AddrNum,StName,City,X,Y columns.
esrihq2 = geocode(multi_field_address,
out_sr={
"wkid" : 3857,
"latestWkid" : 102100
},
out_fields="Addr_type,AddrNum,StName,City,X,Y")
len(esrihq2)
2
esrihq2[0]['location'], esrihq2[0]['extent']
({'x': -13046163.701778423, 'y': 4036490.1486023655}, {'xmin': -13046184.79347824, 'ymin': 4036355.7832219717, 'xmax': -13045962.154496653, 'ymax': 4036624.5155679826})
We can compare the output cell shown above to the output without specifying out_sr
and out_fields
:
({'x': -117.19568252432872, 'y': 34.05723700023128},
{'xmin': -117.19587199429185,
'ymin': 34.056237000231285,
'xmax': -117.19387199429184,
'ymax': 34.05823700023128})
It is obvious that the x
, y
coordinates represented in location
field, and the bbox
as shown in the extents
field, have been reprojected. However, if we look at the attributes
field of the result, the X
and Y
responses are still the same as the non-projected ones.
esrihq2[0]['attributes']['Addr_type'], esrihq2[0]['attributes']['X'], esrihq2[0]['attributes']['Y']
('PointAddress', -117.19568252432872, 34.05723700023128)
On the other hand, if querying for an attribute key that is not in out_fields
, then the user will see KeyError
shown as what's being returned here:
esrihq2[0]['attributes']['Postal']
---------------------------------------------------------------------------KeyError Traceback (most recent call last)<ipython-input-78-c83a961fdcfb> in <module>
----> 1 esrihq2[0]['attributes']['Postal'], esrihq2[0]['attributes']['Xmin'], esrihq2[0]['attributes']['Ymin']
KeyError: 'Postal'
source_country
parameter
source_country
limits the returned candidates to the specified country or countries for either single-field or multifield requests. Three-character country codes are the acceptable values for this parameter. A list of supported countries and codes is available in Geocode coverage. Multiple country codes can be specified (comma-separated with no spaces) to return results for more than one country.
Example
sourceCountry=FRA,DEU,ESP
lang_code
parameter
lang_code
sets the language in which geocode results are returned. This is useful for ensuring that results are returned in the expected language. If the langCode parameter isn't included in a request, or if it is included but there are no matching features with the input language code, then the resultant match is returned in the language code of the primary matched components from the input search string. See the table of supported countries for more information and valid language code values in each country. Note that full language names cannot be used in the lang_code
parameter.
Example
langCode=fr
Now let's look at an example of geocoding at 'Western Wall'
. We will specify the geocode to be performed inside the country of Israel and to return the results in Hebrew.
# when `lang_code` is not set, default to return results in English
western_wall_en = geocode("Western Wall", source_country = "ISR")
western_wall_en[0]['attributes']['LongLabel']
'The Western Wall, Rahvat HaKotel, Yerushalayim, ISR'
western_wall = geocode("Western Wall", source_country = "ISR", lang_code="HE")
western_wall[0]['attributes']['LongLabel']
'הכותל המערבי, רחבת הכותל, ירושלים'
Similarly, here we revisit a previous example of geocoding at 'Taj Mahal'
, only this time, we also specify the geocode to be performed inside the country of India and to return the result in Hindi:
# when `lang_code` is not set, default to return results in English
taj_ind_en = geocode("Taj Mahal", source_country="IND")
taj_ind_en[0]['attributes']['LongLabel']
'Taj Mahal, Taj Ganj, Agra, Uttar Pradesh, 282001, IND'
taj_ind = geocode("Taj Mahal", source_country="IND", lang_code="HI")
taj_ind[0]['attributes']['LongLabel']
'ताज महल, ताज गंज, आगरा, उत्तर प्रदेश, 282001, IND'
match_out_of_range
parameter
The match_out_of_range
parameter provides better spatial accuracy for inexact street addresses by specifying whether matches should be returned when the input number is outside of the house range defined for the input street. Out of range matches
will be defined as Addr_type=StreetAddressExt
. Input house numbers that exceed the range on a street segment by more than 100
will not result in streetAddressExt matches. For streets with smaller house numbers, the maxOutOfRange tolerance is less than 100. The default value of this parameter is true. Check out more on this topic at Esri Community.
Values: True | False
When match_out_of_range
is not set or set to True, geocode
will need to include the inexact estreet addresses as matched results. When set to False, then the results outside of the house range can be excluded.
res_list = geocode("109 Main Street, Fairfax, VA", match_out_of_range=False)
print(len(res_list), " potential matches:")
for res in res_list:
print(res['attributes']['LongLabel'], " | ", res['attributes']['Addr_type'])
20 potential matches: Main St, Fairfax, VA, 22032, USA | StreetName Main St, Fairfax, VA, 22031, USA | StreetName Main St, Fairfax, VA, 22030, USA | StreetName Main St, Alexandria, VA, 22309, USA | StreetName Main St, Clifton, VA, 20124, USA | StreetName 109 Main St, Fairfax, IA, 52228, USA | StreetAddress 109 S Main St, Fairfax, OK, 74637, USA | StreetAddress 109 N Main St, Fairfax, OK, 74637, USA | StreetAddress 109 E Main St, Fairfax, MO, 64446, USA | StreetAddress 109 Main St W, Fairfax, SC, 29827, USA | StreetAddress 109 Main St E, Fairfax, SC, 29827, USA | StreetAddress 109 Main Dr, Fairfax, SC, 29827, USA | StreetAddress Main Dr, Herndon, VA, 20170, USA | StreetName The Main Street Pub, 7140 Main St, Clifton, VA, 20124, USA | POI MainStreet Bank, 4029 Chain Bridge Rd, Fairfax, VA, 22030, USA | POI MainStreet Bank, 10089 Fairfax Blvd, Fairfax, VA, 22030, USA | POI Main Street OB Gyn, 10875 Main St, Fairfax, VA, 22030, USA | POI Main Street Shell, 9956 Main St, Fairfax, VA, 22031, USA | POI 109 Main St, Cambridge, VT, 05444, USA | StreetAddress MainStreet Bank, 1354 Old Chain Bridge Rd, Mc Lean, VA, 22101, USA | POI
location_type
parameter
The location_type
parameter specifies whether the rooftop point
or street entrance
should be the output geometry of PointAddress matches. By default, street
is used, which can be useful for routing scenarios, as the rooftop location of some addresses may be offset from a street by a large distance. However, for map display purposes, it may be desirable to use rooftop instead, especially when large buildings or landmarks are geocoded.
The location parameter only affects the location object in the JSON response and does not change the x,y or DisplayX/DisplayY attribute values.
As what will see in the example below, when location_type="rooftop"
, map rendering of the specific point uses rooftop, which reflects the detailed location of PointAddress
better. When location_type="street"
, map rendering of the returned geocoded result usually polts the point at the street.
res_fset = geocode("380 New York St, 92373", location_type="rooftop", as_featureset=True)
res_fset2 = geocode("380 New York St, 92373", location_type="street", as_featureset=True)
from ipywidgets import HBox, VBox, Label, Layout
# create 3 map widgets
map1 = gis.map("Redlands, CA", 14)
map2 = gis.map("Redlands, CA", 14)
# set hbox layout preferences
hbox_layout = Layout()
hbox_layout.justify_content = 'space-around'
# create 2 hbox - one for title, another for maps
hb1 = HBox([Label('rooftop'),Label('street')])
hb2 = HBox([map1, map2])
hb1.layout,hb2.layout = hbox_layout, hbox_layout
# sync all maps
map1.sync_navigation(map2)
# each hbox is a row in the vbox
VBox([hb1,hb2])

map1.draw(res_fset) #shown on the left panel, as point on the rooftop of a building
map2.draw(res_fset2) #shown on the right, as point at the street
Commonly Seen Errors
Error messages (and/or error codes) are returned when the geocoding service cannot complete a request. This can be due to invalid parameters being included in the request or insufficient user permissions. Some potential errors that may be encountered with the service are described below.
Exception: Invalid Token (Error Code: 498)
Possible causes:- The token associated with the current GIS object might be expired or invalid. You can try signing in to the GIS object again.
Unable to complete operation (Error Code: 400)
Possible causes:<parameter name> parameter value exceeds the maximum length of <maximum length> characters allowed for the parameter value.
This error is returned if the input parameter value contains too many characters. For example, if the SingleLine parameter value exceeds 100 characters, this error is returned.Category parameter value entered is not supported
This error is returned when an invalid category parameter value is included in a request.
Cannot perform query. Invalid query parameters. (Error Code: 400)
Possible causes:Unable to find address for the specified location.
Returned when no match can be found for a reverseGeocode operation.
Invalid or missing input parameters. (Error Code: 400)
Possible causes:- This error is returned if a request is missing a required parameter.
Other error messages can be found in the help on geocoding service output.
Conclusions
In Part 2, we have walked through various approaches of performing geocode()
to locate addresses using single line address, or multi-field address, with or without advanced parameters. Next in Part 3, we will talk about how to geocode point of interests.