In addition to geocoding addresses, the geocoding service can also be used to find points of interest (POI) of several different kinds. In Part 3, we will explain briefly about category
parameter of geocode()
function and its applications.
Getting started
First, Let's look at some Quickstart examples, which show how the category
attributes change based on the search string.
Search for administrative place names
The geocode()
method supports single field and multi-field searches for administrative place names. This includes searches for neighborhoods, cities, counties, states, provinces, or countries. If a search for a city name results in multiple matches with the same name, the World Geocoding Service will sort the candidates in order of their relative importance to each other (as indicated by the value of the Rank output field), with priority generally based on population and capital status.
For example, there are many cities in the world named Liverpool
, so a search for "Liverpool" results in several equivalent matches; Liverpool, UK
will always be the top candidate since it has the greatest population.
from arcgis.geocoding import geocode
from arcgis.gis import GIS
gis = GIS("home")
address = "Liverpool"
map1 = gis.map("United Kingdom", 6)
map1
liverpool = geocode(address)[0]
map1.draw(liverpool['location'])
However, rank alone is not always enough to distinguish between administrative places. Also, you may not necessarily want to find the highest-ranked feature for a particular search. It may be necessary to remove ambiguity by refining searches with additional information. For example, a search for Liverpool
returns Liverpool, UK
as the top candidate based on rank. If you instead want to find the town of Liverpool, New York, it is necessary to add the state information to the search.
address = {
"Address" : "Liverpool",
"Region" : "NY"
}
map2 = gis.map("Onondaga County, New York, United States")
map2
liverpool = geocode(address)[0]
map2.draw(liverpool['location'])
Example of finding landmarks
The geocode()
method can be used to find famous landmarks. The example below geocodes and maps Mt. Everest in Asia, Eiffel Tower in Europe, and the Statue of Liberty in North America:
landmarks = ["Mt. Everest", "Eiffel Tower", "Statue of Liberty"]
map4 = gis.map()
map4
for lm in landmarks:
lm_res = geocode(lm)[0]
map4.draw(lm_res['location'])
Search for postal codes
The geocode()
method supports searches for postal codes and postal code extensions. When searching for postal codes, it is important to note that the same code can be valid in more than one country. For the best results, it may be necessary to include additional information with the postal code, such as city or country.
address = {
"Postal" : 110001,
"CountryCode" : "India"
}
map3 = gis.map("New Delhi, India")
map3
pincode = geocode(address)[0]
map3.draw(pincode['location'])
The matched address contains several attributes that provide values for the various output fields supported by the geocoder, as listed below:
pincode['attributes']
{'Loc_name': 'World', 'Status': 'M', 'Score': 100, 'Match_addr': '110001', 'LongLabel': '110001, New Delhi, Delhi, IND', 'ShortLabel': '110001', 'Addr_type': 'Postal', 'Type': '', 'PlaceName': '110001', 'Place_addr': 'New Delhi, Delhi, 110001', 'Phone': '', 'URL': '', 'Rank': 5, 'AddBldg': '', 'AddNum': '', 'AddNumFrom': '', 'AddNumTo': '', 'AddRange': '', 'Side': '', 'StPreDir': '', 'StPreType': '', 'StName': '', 'StType': '', 'StDir': '', 'BldgType': '', 'BldgName': '', 'LevelType': '', 'LevelName': '', 'UnitType': '', 'UnitName': '', 'SubAddr': '', 'StAddr': '', 'Block': '', 'Sector': '', 'Nbrhd': '', 'District': '', 'City': 'New Delhi', 'MetroArea': '', 'Subregion': 'New Delhi', 'Region': 'Delhi', 'RegionAbbr': 'DL', 'Territory': '', 'Zone': '', 'Postal': '110001', 'PostalExt': '', 'Country': 'IND', 'CntryName': 'India', 'LangCode': 'ENG', 'Distance': 0, 'X': 77.21866000000006, 'Y': 28.623395000000073, 'DisplayX': 77.21866000000006, 'DisplayY': 28.623395000000073, 'Xmin': 77.18466000000005, 'Xmax': 77.25266000000006, 'Ymin': 28.589395000000074, 'Ymax': 28.657395000000072, 'ExInfo': ''}
Note that if users want to get exact administrative boundaries, they can do so by using geoenrichment as shown here.
Example of finding multiple categories
In the example below, we search for Indian and Thai Food in Los Angeles, and plot their locations using different symbols based on the Type
attribute:
categories = "Indian Food, Thai Food"
dtla = geocode("Downtown, Los Angeles, CA")[0]
map5 = gis.map(dtla)
map5
# find and plot up to 100 Indian and Thai restaurants in DTLA
restaurants = geocode(None, dtla['extent'], category=categories, max_locations=100)
thai_symbol = {
"type": "esriSMS",
"style": "esriSMSSquare",
"color": [76,115,0,255],
"size": 8,
"angle": 0,
"xoffset": 0,
"yoffset": 0,
"outline":
{
"color": [152,230,0,255],
"width": 1
}
}
indian_symbol = {
"type": "esriSMS",
"style": "esriSMSCircle",
"color": [115,0,76,255],
"size": 8,
"angle": 0,
"xoffset": 0,
"yoffset": 0,
"outline":
{
"color": [152,230,0,255],
"width": 1
}
}
for restaurant in restaurants:
popup = {
"title" : restaurant['address'],
"content" : "Phone: " + restaurant['attributes']['Phone']
}
if restaurant['attributes']['Type'] == 'Thai Food':
map5.draw(restaurant['location'], popup, thai_symbol) # use a green square symbol for Thai food
else:
map5.draw(restaurant['location'], popup, indian_symbol)
Example of finding restaurants within a polygon drawn on map
In the example above, we have learned to search for restaurants in downtown LA. Next we will design a call-back method that could get the geometry of the drawn sketch entered by user and use it as the customized search_extent
for the geocode()
method.
map6 = gis.map()
map6.extent = { 'spatialReference': {'latestWkid': 3857, 'wkid': 102100},
'xmin': -8235706.664189668,
'ymin': 4977993.551288029,
'xmax': -8233351.448255569,
'ymax': 4978949.014141619}
map6
Searching within the area of drawn polygon...
{'xmin': -8237203.157884028, 'ymin': 4975541.833547482, 'xmax': -8234069.23972434, 'ymax': 4977544.483455284, 'spatialReference': {'latestWkid': 3857, 'wkid': 102100}}
Paris Baguette
Melt Bakery
Farmstand
Liquiteria
Sushi Express
Playa Bowls
Ovenly
Starbucks
Toby's Estate Coffee
Sushi On Jones
from arcgis.geometry import Polygon, project, Geometry
# Define the callback function that search within the area.
drawn_polygon = None
def find_restaurants(map1, g):
global drawn_polygon
drawn_polygon = g
print("Searching within the area of drawn polygon...")
search_area = Polygon(g)
search_area_extent = { 'xmin': search_area.extent[0],
'ymin': search_area.extent[1],
'xmax': search_area.extent[2],
'ymax': search_area.extent[3],
'spatialReference': {'latestWkid': 3857, 'wkid': 102100}}
print(search_area_extent)
restaurants = geocode(None, search_extent=search_area_extent, category="Food", max_locations=10)
print(restaurants)
for restaurant in restaurants:
popup = {
"title" : restaurant['address'],
"content" : "Phone: " + restaurant['attributes']['Phone']
}
map1.draw(restaurant['location'], popup)
print(restaurant['address'])
# Set as the callback function to be invoked when a polygon is drawn on the map
map6.on_draw_end(find_restaurants)
# Either use the geometry defined below
search_area_dict = {'type': 'Polygon', 'coordinates': [[[-73.95949251594702, 40.763214654993785],
[-73.97567231593547, 40.74551709428493],
[-74.00064117977167, 40.76103030464491],
[-73.95949251594702, 40.763214654993785]]]}
search_area = Geometry(search_area_dict)
map6.draw(search_area)
# Or draw your own polygon
#map6.draw("polygon")
Example of finding hospitals within 10 mile buffer around Esri HQ and distance to each
Next, let's walk through the example of finding hospitals within a 10-mile buffer around Esri Headquarter (HQ) and computing distances from Esri to each hospital. The steps of implementation in this section would include:
- Creating a Point object for Esri HQ, use the
geometry
module to build abuffer
, and then applying it assearch_extent
parameter. - Getting
geocode()
results as aFeatureSet
, iterating through each feature within, and usingdistance()
to compute the distance from the originalPoint
to the feature. - Presenting results as a
DataFrame
showing different columns along with distance, and plotting the distance as a bar chart. - Plotting the
FeatureSet
on the map with appropriate symbols (using the Python symbol picker for this, or programmatically create these symbols).
Step 1. Create a Point object for Esri HQ, and build a buffer
esrihq_fset = geocode("Esri", as_featureset=True)
esrihq_fset
<FeatureSet> 20 features
esri_geom = esrihq_fset.features[0]
esri_geom.geometry.JSON
'{"x": -117.19569523299998, "y": 34.05608640000003, "spatialReference": {"wkid": 4326, "latestWkid": 4326}}'
from arcgis.features import Feature, FeatureSet
from arcgis.geometry import buffer
esri_buffer = buffer([esri_geom.geometry],
in_sr = 102100, buffer_sr=102100,
distances=0.1, unit=9001)[0]
esri_buffer_f = Feature(geometry=esri_buffer)
esri_buffer_fset = FeatureSet([esri_buffer_f])
esri_buffer_fset
<FeatureSet> 1 features
# need to change the `type` from `MultiPolygon` to `Polygon`
esri_buffer_f_geom_dict = {"type": "Polygon",
"coordinates": esri_buffer_f.geometry.coordinates().tolist()}
Step 2. Geocode and compute distance
map7 = gis.map("Redlands, CA")
map7
esri_buffer_geom = Geometry(esri_buffer_f_geom_dict)
esri_buffer_geom.extent
(-117.29569523299998, 33.956086400000025, -117.09569523299999, 34.15608640000003)
fill_symbol = {"type": "esriSFS",
"style": "esriSFSNull",
"outline":{"color": [0,0,0,255]}}
map7.draw(esri_buffer_geom, symbol=fill_symbol)
house_symbol = {"angle":0,"xoffset":0,"yoffset":0,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/Shapes/RedStarLargeB.png",
"contentType":"image/png","width":24,"height":24}
map7.draw(esri_geom.geometry, symbol=house_symbol)
search_area_extent = { 'xmin': esri_buffer_geom.extent[0],
'ymin': esri_buffer_geom.extent[1],
'xmax': esri_buffer_geom.extent[2],
'ymax': esri_buffer_geom.extent[3],
'spatialReference': {'latestWkid': 4326, 'wkid': 102100}}
hospitals = geocode('hospital', search_extent=search_area_extent, max_locations=50)
len(hospitals)
6
hospital_symbol = {"angle":0,"xoffset":0,"yoffset":0,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/SafetyHealth/Hospital.png",
"contentType":"image/png","width":24,"height":24}
neighborhood_data_dict = {}
neighborhood_data_dict['hospitals'] = []
for place in hospitals:
popup={"title" : place['attributes']['PlaceName'],
"content" : place['attributes']['Place_addr']}
map7.draw(place['location'], symbol=hospital_symbol, popup=popup)
neighborhood_data_dict['hospitals'].append(place['attributes']['PlaceName'])
for place in hospitals:
print(place["location"])
{'x': -117.27639999999997, 'y': 34.13462000000004, 'type': 'point', 'spatialReference': {'wkid': 4326}} {'x': -117.27727999999996, 'y': 34.13429000000008, 'type': 'point', 'spatialReference': {'wkid': 4326}} {'x': -117.20486999999997, 'y': 34.03696000000008, 'type': 'point', 'spatialReference': {'wkid': 4326}} {'x': -117.20439999999996, 'y': 34.03624000000008, 'type': 'point', 'spatialReference': {'wkid': 4326}} {'x': -117.26324001673439, 'y': 34.0497600366438, 'type': 'point', 'spatialReference': {'wkid': 4326}} {'x': -117.26249999999999, 'y': 34.048730000000035, 'type': 'point', 'spatialReference': {'wkid': 4326}}
from arcgis.geometry import Point, distance
neighborhood_data_dict['distance'] = []
for place in hospitals:
dis = distance( spatial_ref=4326,
geometry1=Point(place["location"]),
geometry2=esri_geom.geometry,
geodesic=False,
gis=gis)
neighborhood_data_dict['distance'].append(dis['distance'])
Step 3. Present results in tables and bar charts
Note: The distance column is to displayed in units of 100 miles.
import pandas as pd
neighborhood_df = pd.DataFrame.from_dict(neighborhood_data_dict, orient='index')
neighborhood_df = neighborhood_df.transpose()
neighborhood_df
hospitals | distance | |
---|---|---|
0 | St Bernardine Medical Center | 0.112609 |
1 | St Bernardine Medical Center-ER | 0.113013 |
2 | Redlands Community Hospital | 0.021213 |
3 | Redlands Community Hospital-ER | 0.021671 |
4 | Loma Linda University Medical Center | 0.06784 |
5 | Loma Linda University Medical Center-ER | 0.067209 |
The bar plot below displays the real address on the x-axis for each entry of hospitals on the x-axis.
import matplotlib.pyplot as plt
fig = plt.figure()
ax = fig.add_axes([0,0,1,1])
addrs = neighborhood_data_dict["hospitals"]
scores = neighborhood_data_dict["distance"]
ax.bar(addrs,scores)
plt.xticks(rotation=90)
plt.show()

Finding POIs using category filtering
We have seen selective examples with the category
parameter being used in the geocode()
function above. The steps below show the users how to get the list of all categories the geocoder knows and some usage examples:
Get a list of available categories and sub-categories with the current geocoder
from arcgis.geocoding import get_geocoders
geocoder = get_geocoders(gis)[0]
def list_categories(obj, depth = 0):
for category in obj['categories']:
print('\t'*depth + category['name'])
if 'categories' in category:
list_categories(category, depth + 1)
list_categories(geocoder.properties)
Address Subaddress Point Address Street Address Distance Marker Intersection Street Midblock Street Name Postal Primary Postal Postal Locality Postal Extension Coordinate System LatLong XY YX MGRS USNG Populated Place Block Sector Neighborhood District City Metro Area Subregion Region Territory Country Zone POI Arts and Entertainment Amusement Park Aquarium Art Gallery Art Museum Billiards Bowling Alley Casino Cinema Historical Monument History Museum Indoor Sports Jazz Club Landmark Live Music Museum Other Arts and Entertainment Performing Arts Ruin Science Museum Tourist Attraction Wild Animal Park Zoo Education College Fine Arts School Other Education School Vocational School Food African Food American Food Argentinean Food Australian Food Austrian Food Bakery Balkan Food BBQ and Southern Food Belgian Food Bistro Brazilian Food Breakfast Brewpub British Isles Food Burgers Cajun and Creole Food Californian Food Caribbean Food Chicken Restaurant Chilean Food Chinese Food Coffee Shop Continental Food Creperie East European Food Fast Food Filipino Food Fondue French Food Fusion Food German Food Greek Food Grill Hawaiian Food Ice Cream Shop Indian Food Indonesian Food International Food Irish Food Italian Food Japanese Food Korean Food Kosher Food Latin American Food Malaysian Food Mexican Food Middle Eastern Food Moroccan Food Other Restaurant Pastries Pizza Polish Food Portuguese Food Restaurant Russian Food Sandwich Shop Scandinavian Food Seafood Snacks South American Food Southeast Asian Food Southwestern Food Spanish Food Steak House Sushi Swiss Food Tapas Thai Food Turkish Food Vegetarian Food Vietnamese Food Winery Land Features Atoll Basin Butte Canyon Cape Cave Cliff Continent Desert Dune Flat Forest Glacier Grassland Hill Island Isthmus Lava Marsh Meadow Mesa Mountain Mountain Range Oasis Other Land Feature Peninsula Plain Plateau Point Ravine Ridge Rock Scrubland Swamp Valley Volcano Wetland Nightlife Spot Bar or Pub Dancing Karaoke Night Club Nightlife Parks and Outdoors Basketball Beach Campground Diving Center Fishing Garden Golf Course Golf Driving Range Harbor Hockey Ice Skating Rink Nature Reserve Other Parks and Outdoors Park Racetrack Scenic Overlook Shooting Range Ski Lift Ski Resort Soccer Sports Center Sports Field Swimming Pool Tennis Court Trail Wildlife Reserve Professional and Other Places Ashram Banquet Hall Border Crossing Building Business Facility Cemetery Church City Hall Civic Center Convention Center Court House Dentist Doctor Embassy Factory Farm Fire Station Government Office Gurdwara Hospital Industrial Zone Library Livestock Medical Clinic Military Base Mine Mosque Observatory Oil Facility Orchard Other Professional Place Other Religious Place Place of Worship Plantation Police Station Post Office Power Station Prison Public Restroom Radio Station Ranch Recreation Facility Religious Center Scientific Research Shrine Storage Synagogue Telecom Temple Tower Veterinarian Vineyard Warehouse Water Tank Water Treatment Residence Estate House Nursing Home Residential Area Shops and Service ATM Auto Dealership Auto Maintenance Auto Parts Bank Beauty Salon Bookstore Butcher Candy Store Car Wash Childrens Apparel Clothing Store Consumer Electronics Store Convenience Store Delivery Service Department Store Electrical Fitness Center Flea Market Food and Beverage Shop Footwear Furniture Store Gas Station Grocery Jewelry Laundry Home Improvement Store Market Mens Apparel Mobile Phone Shop Motorcycle Shop Office Supplies Store Optical Other Shops and Service Pet Store Pharmacy Plumbing Repair Services Shopping Center Spa Specialty Store Sporting Goods Store Tire Store Toy Store Used Car Dealership Wholesale Warehouse Wine and Liquor Womens Apparel Travel and Transport Airport Bed and Breakfast Bridge Bus Station Cargo Center Dock Ferry Heliport Highway Exit Hostel Hotel Marina Metro Station Motel Other Travel Parking Pier Port Rental Cars Railyard Resort Rest Area Taxi Tollbooth Tourist Information Train Station Transportation Service Travel Agency Truck Stop Tunnel Weigh Station Water Features Abyssal Plain Bay Canal Channel Continental Rise Continental Shelf Continental Slope Cove Dam Delta Estuary Fjord Fracture Zone Gulf Hot Spring Irrigation Jetty Lagoon Lake Ocean Ocean Bank Oceanic Basin Oceanic Plateau Oceanic Ridge Other Water Feature Reef Reservoir Sea Seamount Shoal Sound Spring Strait Stream Submarine Canyon Submarine Cliff Submarine Fan Submarine Hill Submarine Terrace Submarine Valley Trench Undersea Feature Waterfall Well Wharf
An example of finding restaurants around a given location
Now let us find restaurants near Time Square (with a maximum of returned results set to 100, since there are way too many restaurants in the specified location).
time_square = geocode("Time Square, NYC")[0]
map8 = gis.map(time_square)
map8
restaurants = geocode(None, time_square['extent'], category="Food", max_locations=100)
for restaurant in restaurants:
popup = {
"title" : restaurant['address'],
"content" : "Phone: " + restaurant['attributes']['Phone']
}
map8.draw(restaurant['location'], popup)
How to refine the results by sub-category
Still using the last request to search for restaurants near Time Square, we will further refine the search by sub-categories, e.g. Indian, Chinese, Burgers, Thai.. etc.
The category
parameter is used to specify a place or address type which can be used to filter results. The parameter supports input of single category values
or multiple comma-separated values
. Its usage (shown below) is applicable for all other categories that have sub-categories, besides food. For instance, Education
would include College
, Fine Arts School
, Other Education
, School
and Vocational School
. For more categories and sub-categories, please refer to API Reference.
map9 = gis.map(time_square)
map9
categories = "Indian Food, Chinese Food, Burgers, Thai Food"
chinese_symbol = {
"type": "esriSMS",
"style": "esriSMSSquare",
"color": [115,100,76,55],
"size": 8,
"angle": 0,
"xoffset": 0,
"yoffset": 0,
"outline":
{
"color": [152,230,0,255],
"width": 1
}
}
burgers_symbol = {
"type": "esriSMS",
"style": "esriSMSCircle",
"color": [15,0,176,255],
"size": 8,
"angle": 0,
"xoffset": 0,
"yoffset": 0,
"outline":
{
"color": [152,230,0,255],
"width": 1
}
}
restaurants = geocode(None, time_square['extent'], category=categories, max_locations=100)
for restaurant in restaurants:
popup = {
"title" : restaurant['address'],
"content" : "Phone: " + restaurant['attributes']['Phone']
}
if restaurant['attributes']['Type'] == 'Thai Food':
map9.draw(restaurant['location'], popup, thai_symbol) # green square
elif restaurant['attributes']['Type'] == 'Indian Food':
map9.draw(restaurant['location'], popup, indian_symbol) # dark red circle
elif restaurant['attributes']['Type'] == 'Chinese Food':
map9.draw(restaurant['location'], popup, chinese_symbol) # mint square
else:
map9.draw(restaurant['location'], popup, burgers_symbol) # blue circle
Example of finding gas stations, bars, and other facilities near a given location
The example below showcases how to find gas stations, bars, libraries, schools, parks, and grocery stores around a given location, based on the previous sample in which we look for hospitals near Esri Headquarter.
Step 1. Create symbols for facilities
(You can get your symbols using this online tool.)
symbols = {"groceries": {"angle":0,"xoffset":0,"yoffset":0,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/PeoplePlaces/Shopping.png",
"contentType":"image/png","width":12,"height":12},
"coffee": {"angle":0,"xoffset":0,"yoffset":0,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/PeoplePlaces/Coffee.png",
"contentType":"image/png","width":12,"height":12},
"restaurant": {"angle":0,"xoffset":0,"yoffset":0,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/PeoplePlaces/Dining.png",
"contentType":"image/png","width":12,"height":12},
"bar": {"angle":0,"xoffset":0,"yoffset":0,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/PeoplePlaces/Bar.png",
"contentType":"image/png","width":12,"height":12},
"gas": {"angle":0,"xoffset":0,"yoffset":0,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/Transportation/esriBusinessMarker_72.png",
"contentType":"image/png","width":12,"height":12},
"park": {"angle":0,"xoffset":0,"yoffset":0,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/OutdoorRecreation/RestArea.png",
"contentType":"image/png","width":10,"height":10},
"school": {"angle":0,"xoffset":0,"yoffset":0,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/PeoplePlaces/Note.png",
"contentType":"image/png","width":10,"height":10},
"library": {"angle":0,"xoffset":0,"yoffset":0,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/PeoplePlaces/LiveShow.png",
"contentType":"image/png","width":12,"height":12}}
list(symbols.keys())
['groceries', 'coffee', 'restaurant', 'bar', 'gas', 'park', 'school', 'library']
Step 2. Define your own geocode function
Next, let's define a function to use the ArcGIS Geocoding service in search of facilities around the Esri Headquarter, based on the kind of facilities (e.g. groceries) you are looking for:
def search_and_map(in_map, kind="groceries"):
per_kind = geocode(kind, search_extent=search_area_extent,
max_locations=20, as_featureset=True)
neighborhood_data_dict[kind] = []
for place in per_kind:
popup={"title" : place.attributes['PlaceName'],
"content" : place.attributes['Place_addr']}
in_map.draw(place.geometry, symbol=symbols[kind], popup=popup)
neighborhood_data_dict[kind].append(place.attributes['PlaceName'])
Now, we are ready to loop through the list of facility types and perform geocoding for each kind, then map the results with the customized symbols:
map7.zoom = 13
map7
for kind in list(symbols.keys()) :
search_and_map(map7, kind)
Step 3. Tabularize the results
Last but not least, let's present the results in a table:
neighborhood_df = pd.DataFrame.from_dict(neighborhood_data_dict, orient='index')
neighborhood_df = neighborhood_df.transpose()
neighborhood_df
hospitals | distance | groceries | coffee | restaurant | bar | gas | park | school | library | |
---|---|---|---|---|---|---|---|---|---|---|
0 | St Bernardine Medical Center | 0.112609 | E D M Supermarket | Starbucks | SJL tacos | The Brandin' Iron Country Nightclub | Mobil | Meadowbrook Fields | Public Safety Academy | Howard Rowe Public Library |
1 | St Bernardine Medical Center-ER | 0.113013 | Food 4 Less | Starbucks | Ala Eh Ihaw Ihaw | Arrowhead Country Club | Golden Petroleum | Meadowbrook Park | Victoria Elementary School | San Bernardino County Library |
2 | Redlands Community Hospital | 0.021213 | S M Seafood & Asian Market | Starbucks | Mu | Dingers Sports Bar and Grill | Chevron | Seccombe Lake | La Petite Academy | Country Law Library |
3 | Redlands Community Hospital-ER | 0.021671 | Shop Rite No 3 Market | Starbucks | Breakfast Shack | Sportspage | Chevron Extra Mile | Palm Park | Crafton Elementary School | Coddington Library |
4 | Loma Linda University Medical Center | 0.06784 | Stater Bros. Markets | Starbucks | The Royal Falconer Pub & Restaurant | Trenia's Bed | ARCO | Perris Hill Park | Hope Christian Elementary School | Norman Feldheym Central Library |
5 | Loma Linda University Medical Center-ER | 0.067209 | Rocky Market | Starbucks | Caprice Cafe | Gold Ball Billiards | ARCO | Mill Park | Franklin Elementary School | San Bernardino Public Library |
6 | None | None | Mother's Nutritional Centers | Starbucks | Don Orange Tacos | Pool Club | California Tool & Welding Supply | Redlands Sports Park | Tender Care for Kids | AK Smiley Public Library |
7 | None | None | Ninety Nine Njr Outlet | Starbucks | The Gourmet Pizza Shoppe | South Side Saloon | Shell Oil | Sylvan Park | Target Community Schools | Highland Branch Library |
8 | None | None | Cardenas Markets | Starbucks | The State | Taker Over Tuesdays | ARCO | Community Park | Lugonia Elementary School | Loma Linda Branch Library |
9 | None | None | Gate City Beverage Bear Trucking | The Coffee Bean & Tea Leaf | Darby's American Cantina | La Luna | Valero Energy | Israel Beal Park | Judson & Brown Elementary School | A K Smiley Public Library |
10 | None | None | La Surtidora Cash & Carry | Starbucks | Downtown Redlands | The Blue Swing | Chevron | Crafton Park | Clement Middle School | Armacost Library |
11 | None | None | Design Checkstands | Starbucks | Redlands Oyster Cult | Ahalena Hookah Lounge | Food'n Fuel | Redlands Dog Park | Ahrens Child Care Center | Division Two Library California State Court of... |
12 | None | None | Cott Beverages USA | Starbucks | McDuff's | Gotham | Costco Gasoline | Ford Park | Target Community School | Rowe Branch San Bernardino Public Library |
13 | None | None | Interstate Brands | Starbucks | Ilegal 1895 | Y Lounge | ARCO | Prospect Park | Redlands Christian School | San Bernardino County Library |
14 | None | None | ALDI | Starbucks | Tokyo Restaurant | The Finish Line Bar | 7-Eleven Fuel | Smiley Park | Redlands High School | San Bernardino Public Library |
15 | None | None | Rudolph Foods West | Starbucks | Burgertown USA | Kluddes Kitchen Bar | Sam's Club Fuel Center | Caroline Park | Kids Land Academy | San Bernardino Sun Library |
16 | None | None | Summit Cash & Carry | Starbucks | Twin Dragon | Redlands Country Club | Orange Show Food & Fuel | Brookside Park | Moore Middle School | San Bernardino County Law Library |
17 | None | None | A K Food Store | Boba Tea House | Carolyn's Cafe | Vault Martini Bar & Grill | World | Jennie Davis Park | Kingsbury Elementary School | Del E Webb Memorial Library |
18 | None | None | Todd's Market | Starbucks | Brew N Chew | Tartan of Redlands | Orange Show Food Mart | Texonia Park | Cope Middle School | Loma Linda Branch San Bernardino County Library |
19 | None | None | Inland Empire Cash & Carry | Starbucks | The District | Copehouse Bar & Bistro | Orange Oil | East Highlands Ranch Community Park | Smiley Elementary School | Highland Branch San Bernardino County Library |
neighborhood_df.count().plot(kind='bar')
plt.title('Facilities within 10 miles of Esri')
Text(0.5, 1.0, 'Facilities within 10 miles of Esri')
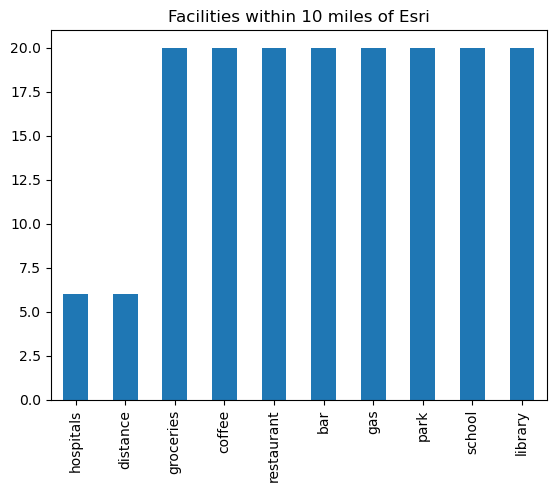
Conclusions
In Part 3, we have walked through different user scenarios using the category
parameter within the geocode()
function to search and filter geocoded results. In the last scenario, because home buyers often look for access to facilities, such as groceries, restaurants, schools, emergency, and health care, near prospective neighborhoods when shortlisting properties, we used the geocoding
module to search for these facilities, build a table for each property, and map the deliverables.