Introduction
The reverse_geocode()
function in the arcgis.geocoding
module determines the address at a particular x/y
location. You can pass the coordinates of a point location to the geocoder
and get returned the address that is closest (or nearest) to the location.
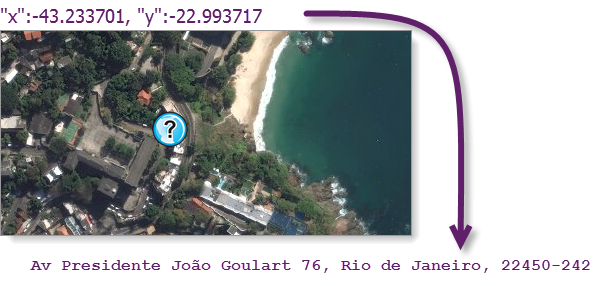
from arcgis.geocoding import reverse_geocode
help(reverse_geocode)
Help on function reverse_geocode in module arcgis.geocoding._functions: reverse_geocode(location, distance=None, out_sr=None, lang_code=None, return_intersection=False, for_storage=False, geocoder=None, feature_types=None, roof_top='street') The reverse_geocode operation determines the address at a particular x/y location. You pass the coordinates of a point location to the geocoding service, and the service returns the address that is closest to the location. =================== ==================================================== **Argument** **Description** ------------------- ---------------------------------------------------- location required list/Point Geometry ------------------- ---------------------------------------------------- distance optional float, radial distance in meteres to search for an address. The default is 100 meters. ------------------- ---------------------------------------------------- out_sr optional integer, spatial reference of the x/y coordinate returned. ------------------- ---------------------------------------------------- lang_code optional string. Sets the language in which geocode results are returned. This is useful for ensuring that results are returned in the expected language. If the lang_code parameter isn't included in a request, or if it is included but there are no matching features with the input language code, the resultant match is returned in the language code of the primary matched components from the input search string. ------------------- ---------------------------------------------------- return_intersection optional Boolean, which specifies whether the service should return the nearest street intersection or the nearest address to the input location ------------------- ---------------------------------------------------- for_storage optional boolean, specifies whether the results of the operation will be persisted ------------------- ---------------------------------------------------- geocoder optional geocoder, the geocoder to be used. If not specified, the active GIS's first geocoder is used. ------------------- ---------------------------------------------------- feature_types Optional String. Limits the possible match types performed by the `reverse_geocode` method. If a single value is included, the search tolerance for the input feature type is 500 meters. If multiple values (separated by a comma, with no spaces) are included, the default search distances specified in the feature type hierarchy table are applied. Values: StreetInt, DistanceMarker, StreetAddress, StreetName, POI, PointAddress, Postal, and Locality ------------------- ---------------------------------------------------- location_type Optional string. Specifies whether the rooftop point or street entrance is used as the output geometry of point address matches. By default, street is used, which is useful in routing scenarios, as the rooftop location of some addresses may be offset from a street by a large distance. However, for map display purposes, you may want to use rooftop instead, especially when large buildings or landmarks are geocoded. The location_type parameter only affects the location object in the JSON response and does not change the x,y or DisplayX/DisplayY attribute values. Values: street, rooftop =================== ==================================================== :returns: dictionary
The required location
parameter
As shown in the help output above, the only required input parameter of reverse_geocode()
is the location
parameter, which is the point from which to search for the closest address. The point can be represented as a simple list
of coordinates ([x, y] or [longitude, latitude]), a dict
object (with or without spatial reference) or JSON point object, or a Point Geometry
object.
The spatial reference of the list of coordinates is always WGS84
(in decimal degress), the same coordinate system as the World Geocoding Service.
Use JSON formatting to specify any other coordinate system for the input location. Specifically, set the spatial reference using its well-known ID (WKID)
value. For a list of valid WKID values, see Projected Coordinate Systems and Geographic Coordinate Systems.
Example using simple syntax and the default WGS84
spatial reference:
location=[103.8767227,1.3330736]
Example using JSON and the default WGS84
spatial reference:
location={"x": 103.876722, "y": 1.3330736}
Example using JSON and specifying a spatial reference (WGS84 Web Mercator Auxiliary Sphere
):
location= { "x": 11563503, "y": 148410, "spatialReference": { "wkid": 3857 } }
The simple reverse geocode examples
Next, we will look at different kinds of input for the location
parameter - (1) list, (2) dict, (3) dict with sr, and (4) Point object. Note that, when composing a list containing x/y coordinates, the order is X,Y and not Y,X as in lat, long.
from arcgis.gis import GIS
from arcgis.geocoding import reverse_geocode
gis = GIS(profile="your_online_profile")
Example 1 - location input as list
results = reverse_geocode([2.2945, 48.8583])
type(results)
dict
results
{'address': {'Match_addr': 'Salle Gustave Eiffel', 'LongLabel': 'Salle Gustave Eiffel, Avenue Gustave Eiffel, 75007, 7e Arrondissement, Paris, Île-de-France, FRA', 'ShortLabel': 'Salle Gustave Eiffel', 'Addr_type': 'POI', 'Type': 'Convention Center', 'PlaceName': 'Salle Gustave Eiffel', 'AddNum': '', 'Address': 'Avenue Gustave Eiffel', 'Block': '', 'Sector': '', 'Neighborhood': 'Gros Caillou', 'District': '7e Arrondissement', 'City': 'Paris', 'MetroArea': '', 'Subregion': 'Paris', 'Region': 'Île-de-France', 'Territory': '', 'Postal': '75007', 'PostalExt': '', 'CountryCode': 'FRA'}, 'location': {'x': 2.2951599583073303, 'y': 48.85784000836726, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}}}
Example 2 - location input as dict
results = reverse_geocode(location={"x": 103.876722, "y": 1.3330736})
results
{'address': {'Match_addr': 'Sennett Estate, 40 Lichi Avenue, 348814, Singapore', 'LongLabel': 'Sennett Estate, 40 Lichi Avenue, 348814, Singapore, SGP', 'ShortLabel': 'Sennett Estate, 40 Lichi Avenue', 'Addr_type': 'PointAddress', 'Type': '', 'PlaceName': '', 'AddNum': '40', 'Address': 'Sennett Estate, 40 Lichi Avenue', 'Block': '', 'Sector': '', 'Neighborhood': '', 'District': '', 'City': 'Singapore', 'MetroArea': '', 'Subregion': '', 'Region': 'Singapore', 'Territory': '', 'Postal': '348814', 'PostalExt': '', 'CountryCode': 'SGP'}, 'location': {'x': 103.87671885261159, 'y': 1.333058719212687, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}}}
Example 3 - location input as dict with sr
results = reverse_geocode(location= { "x": 11563503, "y": 148410, "spatialReference": { "wkid": 3857 } })
results
{'address': {'Match_addr': 'Sennett Estate, 40 Lichi Avenue, 348814, Singapore', 'LongLabel': 'Sennett Estate, 40 Lichi Avenue, 348814, Singapore, SGP', 'ShortLabel': 'Sennett Estate, 40 Lichi Avenue', 'Addr_type': 'PointAddress', 'Type': '', 'PlaceName': '', 'AddNum': '40', 'Address': 'Sennett Estate, 40 Lichi Avenue', 'Block': '', 'Sector': '', 'Neighborhood': '', 'District': '', 'City': 'Singapore', 'MetroArea': '', 'Subregion': '', 'Region': 'Singapore', 'Territory': '', 'Postal': '348814', 'PostalExt': '', 'CountryCode': 'SGP'}, 'location': {'x': 103.87671885261159, 'y': 1.333058719212687, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}}}
Example 4 - location input as Point Geometry object
from arcgis.geometry import Geometry
pt = Geometry({
"x": 11563503,
"y": 148410,
"spatialReference": {
"wkid": 3857
}
})
results = reverse_geocode(pt)
type(results)
dict
results
{'address': {'Match_addr': 'Sennett Estate, 40 Lichi Avenue, 348814, Singapore', 'LongLabel': 'Sennett Estate, 40 Lichi Avenue, 348814, Singapore, SGP', 'ShortLabel': 'Sennett Estate, 40 Lichi Avenue', 'Addr_type': 'PointAddress', 'Type': '', 'PlaceName': '', 'AddNum': '40', 'Address': 'Sennett Estate, 40 Lichi Avenue', 'Block': '', 'Sector': '', 'Neighborhood': '', 'District': '', 'City': 'Singapore', 'MetroArea': '', 'Subregion': '', 'Region': 'Singapore', 'Territory': '', 'Postal': '348814', 'PostalExt': '', 'CountryCode': 'SGP'}, 'location': {'x': 103.87671885261159, 'y': 1.333058719212687, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}}}
Reverse geocode a location clicked on the map
There are situations when the input location is not known beforehand, and instead relies on the user to click a location. The example below illustrates how to define a handler function (e.g. find_addr()
) and an event listener (per user clicking on the map e.g. on_click()
) to handle these instances.
map1 = gis.map('Redlands, CA', 14)
map1

The corresponding addresses for the four clicked points shown above are listed here:
207 Redlands Mall, Redlands, California, 92373
125 W Vine St, Redlands, California, 92373
Redlands Fire Department Station 261
137 E Vine St, Redlands, California, 92373
def find_addr(m, g):
try:
geocoded = reverse_geocode(g)
m.draw(g)
print(geocoded['address']['Match_addr'])
except:
print("Couldn't match address. Try another place...")
map1.on_click(find_addr)
Get result as intersection instead of address (return_intersection
param)
Facts about the return_intersection
parameter:
- A Boolean which specifies whether the service should return the nearest street intersection or the nearest address to the input location.
- If True, then the closest intersection to the input location is returned;
- if False, then the closest address to the input location is returned.
- The default value is False.
# Let's take a look at the intersection of W. Vine St & S 4th St in the city of Redlands, CA

from arcgis.geocoding import geocode
intersect_loc = geocode("S 4th St & W Vine, Redlands, CA")[0]['location']
print(intersect_loc)
{'x': -117.18361987601203, 'y': 34.0546600260095}
When the return_intersection
parameter is not specified, or set to False, then the closest address to the input location is returned. We can see below that the returned address is a StreetAddress
, instead of intersection, and that the matched addresses are of Street number 130 to 134 on the S 4th St
.
reverse_geocode(intersect_loc)
{'address': {'Match_addr': '130-134 S 4th St, Redlands, California, 92373', 'LongLabel': '130-134 S 4th St, Redlands, CA, 92373, USA', 'ShortLabel': '130-134 S 4th St', 'Addr_type': 'StreetAddress', 'Type': '', 'PlaceName': '', 'AddNum': '130', 'Address': '130 S 4th St', 'Block': '', 'Sector': '', 'Neighborhood': 'South Redlands', 'District': '', 'City': 'Redlands', 'MetroArea': 'Inland Empire', 'Subregion': 'San Bernardino County', 'Region': 'California', 'Territory': '', 'Postal': '92373', 'PostalExt': '', 'CountryCode': 'USA'}, 'location': {'x': -117.18367019351942, 'y': 34.054620721017486, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}}}
On the other hand, when set to True
, then the closest intersection to the input location is returned. We can see this in the Match_addr
and Addr_Type
output fields shown below:
reverse_geocode(intersect_loc,
return_intersection = True)
{'address': {'Match_addr': 'S 4th St & W Vine St, Redlands, California, 92373', 'LongLabel': 'S 4th St & W Vine St, Redlands, CA, 92373, USA', 'ShortLabel': 'S 4th St & W Vine St', 'Addr_type': 'StreetInt', 'Type': '', 'PlaceName': '', 'AddNum': '', 'Address': 'S 4th St & W Vine St', 'Block': '', 'Sector': '', 'Neighborhood': 'South Redlands', 'District': '', 'City': 'Redlands', 'MetroArea': 'Inland Empire', 'Subregion': 'San Bernardino County', 'Region': 'California', 'Territory': '', 'Postal': '92373', 'PostalExt': '', 'CountryCode': 'USA'}, 'location': {'x': -117.18361987601203, 'y': 34.05466002600951, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}}}
Get results in desired SR
Facts about the out_sr
parameter:
- The spatial reference of the x/y coordinates returned by a geocode request. This is useful for applications using a map with a spatial reference different than that of the geocode service.
- The spatial reference can be specified as either a
well-known ID (WKID)
or asa JSON spatial reference object
. Ifout_sr
is not specified, the spatial reference of the output locations is the same as that of the service. The World Geocoding Service spatial reference isWGS84 (WKID = 4326)
.
Example (102100
is the WKID
for the Web Mercator projection):
out_sr=102100
The first example below shows a varied version of Example 4, when our_sr
is specified different than WGS84:
reverse_geocode(location = { 'x': 103.87671885261159,
'y': 1.333058719212687,
'spatialReference': {'wkid': 4326, 'latestWkid': 4326}},
out_sr = 3857)
{'address': {'Match_addr': 'Sennett Estate, 40 Lichi Avenue, 348814, Singapore', 'LongLabel': 'Sennett Estate, 40 Lichi Avenue, 348814, Singapore, SGP', 'ShortLabel': 'Sennett Estate, 40 Lichi Avenue', 'Addr_type': 'PointAddress', 'Type': '', 'PlaceName': '', 'AddNum': '40', 'Address': 'Sennett Estate, 40 Lichi Avenue', 'Block': '', 'Sector': '', 'Neighborhood': '', 'District': '', 'City': 'Singapore', 'MetroArea': '', 'Subregion': '', 'Region': 'Singapore', 'Territory': '', 'Postal': '348814', 'PostalExt': '', 'CountryCode': 'SGP'}, 'location': {'x': 11563503.447948763, 'y': 148408.80784300127, 'spatialReference': {'wkid': 102100, 'latestWkid': 3857}}}
Get results in desired language (lang_code
param)
Facts about the lang_code
parameter:
- Sets the language in which reverse-geocoded addresses are returned. Addresses in many countries are available in more than one language. In these cases, the
lang_code
parameter can be used to specify which language should be used for addresses returned by thereverse_geocode()
method. This is useful for ensuring that addresses are returned in the expected language by reverse geocoding functionality in an application. For example, a web application could be designed to get the browser language and then pass it as thelang_code
parameter value in areverse_geocode
request. - See the table of supported countries for valid language code values in each country.
Now let's look at an example of reverse_geocode()
using the land_code
parameter applied to the starting point of Great Wall
in China, and the Taj Marble
in India:
# returning Simplified Chinese
reverse_geocode(location = {'x': 119.79533000000004, 'y': 39.96675000000005},
lang_code = "ZH")
{'address': {'Match_addr': '河北省秦皇岛市山海关区', 'LongLabel': '河北省秦皇岛市山海关区', 'ShortLabel': '河北省秦皇岛市山海关区', 'CountryCode': 'CHN', 'City': '秦皇岛市', 'Neighborhood': '山海关区', 'Region': '河北省', 'Address': '河北省秦皇岛市山海关区', 'Addr_type': 'Locality', 'Type': '', 'PlaceName': '', 'AddNum': '', 'Block': '', 'Sector': '', 'District': '', 'MetroArea': '', 'Subregion': '', 'Territory': '', 'Postal': '', 'PostalExt': ''}, 'location': {'x': 119.744545, 'y': 40.018464, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}}}
# returns in Hindi
reverse_geocode({'x': 74.81476000000004, 'y': 34.02453000000003},
lang_code="HIN")
{'address': {'Match_addr': 'एनएच-1ए, नातिपुरा, श्रीनगर, जम्मू और कश्मीर, 190015', 'LongLabel': 'एनएच-1ए, नातिपुरा, श्रीनगर, जम्मू और कश्मीर, 190015, IND', 'ShortLabel': 'एनएच-1ए', 'Addr_type': 'StreetName', 'Type': '', 'PlaceName': '', 'AddNum': '', 'Address': 'एनएच-1ए', 'Block': '', 'Sector': '', 'Neighborhood': '', 'District': 'नातिपुरा', 'City': 'श्रीनगर', 'MetroArea': '', 'Subregion': 'श्रीनगर', 'Region': 'जम्मू और कश्मीर', 'Territory': '', 'Postal': '190015', 'PostalExt': '', 'CountryCode': 'IND'}, 'location': {'x': 74.81476450480069, 'y': 34.024511515995734, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}}}
Customize the address type of searches (feature_types
param)
feature_types
parameter:
- Limits the possible match types performed by the
reverse_geocode
method. - If a single value is included, the search tolerance for the input feature type is
500
meters. - If multiple values (separated by a comma, with no spaces) are included, the default search distances specified in the feature type hierarchy table are applied.
- The list below includes the valid values for the
feature_types
parameter:- StreetInt, DistanceMarker, StreetAddress, StreetName, POI, PointAddress, Postal, and Locality
Let's see an example of reverse geocoding for the Yosemite National Park
(at {'x':-119.59032605870676 , 'y':37.746595069113496 }
) and how changing the feature_types
from POI
to StreetAddress
, or other types in getting navigable address for GPS, will be different. Note that there will be different output of the X,Y coordinates in the returned dict:
map3 = gis.map("Yosemite National Park")
map3.center = {'spatialReference': {'latestWkid': 3857, 'wkid': 102100},
'x': -13312740.491088409,
'y': 4543687.396149781}
map3.zoom = 19
map3

park_xy = {'x':-119.59032605870676 , 'y':37.746595069113496 }
map3.draw(park_xy)
# without specifying the `feature_types`
park_a = reverse_geocode(park_xy)
map3.draw(park_a["location"],
symbol = {"angle":0,"xoffset":0,"yoffset":8.15625,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/AtoZ/blueA.png",
"contentType":"image/png","width":15.75,"height":21.75})
display(park_a)
{'address': {'Match_addr': 'Northside Dr, Yosemite National Park, California, 95389', 'LongLabel': 'Northside Dr, Yosemite National Park, CA, 95389, USA', 'ShortLabel': 'Northside Dr', 'Addr_type': 'StreetName', 'Type': '', 'PlaceName': '', 'AddNum': '', 'Address': 'Northside Dr', 'Block': '', 'Sector': '', 'Neighborhood': '', 'District': '', 'City': 'Yosemite National Park', 'MetroArea': '', 'Subregion': 'Mariposa County', 'Region': 'California', 'Territory': '', 'Postal': '95389', 'PostalExt': '', 'CountryCode': 'USA'}, 'location': {'x': -119.59032552840779, 'y': 37.74658950402821, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}, 'type': 'point'}}
# search tolerance = 25 meters
park_b = reverse_geocode(park_xy, feature_types = "POI")
map3.draw(park_b["location"],
symbol = {"angle":0,"xoffset":0,"yoffset":8.15625,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/AtoZ/blueB.png",
"contentType":"image/png","width":15.75,"height":21.75})
display(park_b)
{'address': {'Match_addr': 'Lower Yosemite Fall', 'LongLabel': 'Lower Yosemite Fall, Northside Dr, Yosemite National Park, CA, 95389, USA', 'ShortLabel': 'Lower Yosemite Fall', 'Addr_type': 'POI', 'Type': 'Tourist Attraction', 'PlaceName': 'Lower Yosemite Fall', 'AddNum': '', 'Address': 'Northside Dr', 'Block': '', 'Sector': '', 'Neighborhood': '', 'District': '', 'City': 'Yosemite National Park', 'MetroArea': '', 'Subregion': 'Mariposa County', 'Region': 'California', 'Territory': '', 'Postal': '95389', 'PostalExt': '', 'CountryCode': 'USA'}, 'location': {'x': -119.59128999999996, 'y': 37.746380000000045, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}, 'type': 'point'}}
# search_tolerance = 10 meters
park_c = reverse_geocode(park_xy, feature_types = "StreetInt")
map3.draw(park_c["location"],
symbol = {"angle":0,"xoffset":0,"yoffset":8.15625,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/AtoZ/blueC.png",
"contentType":"image/png","width":15.75,"height":21.75})
display(park_c)
{'address': {'Match_addr': 'Northside Dr & Village Dr, Yosemite National Park, California, 95389', 'LongLabel': 'Northside Dr & Village Dr, Yosemite National Park, CA, 95389, USA', 'ShortLabel': 'Northside Dr & Village Dr', 'Addr_type': 'StreetInt', 'Type': '', 'PlaceName': '', 'AddNum': '', 'Address': 'Northside Dr & Village Dr', 'Block': '', 'Sector': '', 'Neighborhood': '', 'District': '', 'City': 'Yosemite National Park', 'MetroArea': '', 'Subregion': 'Mariposa County', 'Region': 'California', 'Territory': '', 'Postal': '95389', 'PostalExt': '', 'CountryCode': 'USA'}, 'location': {'x': -119.59052989240291, 'y': 37.7465700301093, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}, 'type': 'point'}}
# search_tolerance = 3 meters
park_d = reverse_geocode(park_xy, feature_types = "StreetAddress,DistanceMarker,StreetName")
map3.draw(park_d["location"],
symbol = {"angle":0,"xoffset":0,"yoffset":8.15625,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/AtoZ/blueD.png",
"contentType":"image/png","width":15.75,"height":21.75})
display(park_d)
{'address': {'Match_addr': 'Northside Dr, Yosemite National Park, California, 95389', 'LongLabel': 'Northside Dr, Yosemite National Park, CA, 95389, USA', 'ShortLabel': 'Northside Dr', 'Addr_type': 'StreetName', 'Type': '', 'PlaceName': '', 'AddNum': '', 'Address': 'Northside Dr', 'Block': '', 'Sector': '', 'Neighborhood': '', 'District': '', 'City': 'Yosemite National Park', 'MetroArea': '', 'Subregion': 'Mariposa County', 'Region': 'California', 'Territory': '', 'Postal': '95389', 'PostalExt': '', 'CountryCode': 'USA'}, 'location': {'x': -119.59032552840779, 'y': 37.74658950402821, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}, 'type': 'point'}}
# search_tolerance = within boundaries
# If the input location intersects multiple boundaries, the feature with the smallest area is returned.
park_e = reverse_geocode(park_xy, feature_types = "Locality,Postal")
map3.draw(park_e["location"],
symbol = {"angle":0,"xoffset":0,"yoffset":8.15625,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/AtoZ/blueE.png",
"contentType":"image/png","width":15.75,"height":21.75})
display(park_e)
{'address': {'Match_addr': '95389, Yosemite National Park, California', 'LongLabel': '95389, Yosemite National Park, CA, USA', 'ShortLabel': '95389', 'Addr_type': 'Postal', 'Type': '', 'PlaceName': '95389', 'AddNum': '', 'Address': '', 'Block': '', 'Sector': '', 'Neighborhood': '', 'District': '', 'City': 'Yosemite National Park', 'MetroArea': '', 'Subregion': 'Mariposa County', 'Region': 'California', 'Territory': '', 'Postal': '95389', 'PostalExt': '', 'CountryCode': 'USA'}, 'location': {'x': -119.59032605870676, 'y': 37.746595069113496, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}, 'type': 'point'}}
park_f = reverse_geocode(park_xy, feature_types = "POI area")
map3.draw(park_f["location"],
symbol = {"angle":0,"xoffset":0,"yoffset":8.15625,"type":"esriPMS",
"url":"http://static.arcgis.com/images/Symbols/AtoZ/blueF.png",
"contentType":"image/png","width":15.75,"height":21.75})
display(park_f)
{'address': {'Match_addr': 'Yosemite National Park', 'LongLabel': 'Yosemite National Park, Village Dr, Yosemite National Park, CA, 95389, USA', 'ShortLabel': 'Yosemite National Park', 'Addr_type': 'POI', 'Type': 'Park', 'PlaceName': 'Yosemite National Park', 'AddNum': '', 'Address': 'Village Dr', 'Block': '', 'Sector': '', 'Neighborhood': '', 'District': '', 'City': 'Yosemite National Park', 'MetroArea': '', 'Subregion': 'Mariposa County', 'Region': 'California', 'Territory': '', 'Postal': '95389', 'PostalExt': '', 'CountryCode': 'USA'}, 'location': {'x': -119.59032605870676, 'y': 37.746595069113496, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}, 'type': 'point'}}
As we can see from the map above, park_a
, park_d
, park_e
and park_f
all point to the same location (the centroid of Yosemite National Park), while park_b
and park_c
are slightly offset from the centroid.
Limit searches by distance
param
Here, note that default distance varies by feature_types and more can be learnt from here
distance
parameter
- The optional distance parameter allows you to specify a radial distance in meters to search for an address from the specified location. If no distance value is specified, then the value is assumed to be 100 meters.
Example:
distance=50
# search_tolerance = 10 meters
reverse_geocode({'x': -87.62330985,'y':41.88270585},
feature_types = "POI",
distance=10)
{'address': {'Match_addr': 'The Bean', 'LongLabel': 'The Bean, Chicago, IL, 60603, USA', 'ShortLabel': 'The Bean', 'Addr_type': 'POI', 'Type': 'Tourist Attraction', 'PlaceName': 'The Bean', 'AddNum': '', 'Address': '', 'Block': '', 'Sector': '', 'Neighborhood': 'Loop', 'District': '', 'City': 'Chicago', 'MetroArea': 'Chicagoland', 'Subregion': 'Cook County', 'Region': 'Illinois', 'Territory': '', 'Postal': '60603', 'PostalExt': '', 'CountryCode': 'USA'}, 'location': {'x': -87.62351981667449, 'y': 41.88258590496559, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}}}
# search_tolerance = 500 meters
reverse_geocode({'x': -87.62330995,'y':41.88270595},
distance=500)
{'address': {'Match_addr': 'Park Cafe', 'LongLabel': 'Park Cafe, 11 N Michigan Ave, Chicago, IL, 60602, USA', 'ShortLabel': 'Park Cafe', 'Addr_type': 'POI', 'Type': 'Restaurant', 'PlaceName': 'Park Cafe', 'AddNum': '11', 'Address': '11 N Michigan Ave', 'Block': '', 'Sector': '', 'Neighborhood': '', 'District': '', 'City': 'Chicago', 'MetroArea': '', 'Subregion': 'Cook County', 'Region': 'Illinois', 'Territory': '', 'Postal': '60602', 'PostalExt': '', 'CountryCode': 'USA'}, 'location': {'x': -87.62330499999996, 'y': 41.88267500000006, 'spatialReference': {'wkid': 4326, 'latestWkid': 4326}}}
Conclusions
In Part 5 of the Series, we have explored how to perform reverse_geocode()
with different input (e.g. JSON, List
object, or Point Geometry
object) and optional parameters to limit the search results. The optional parameters used included lang_code
, distance
, feature_types
, etc. In the next notebook, we will discuss how to work with a customized geocoder
.