A set of functions for working with FeatureSets.
Area
Area(features, unit?) -> Number
Function bundle: Data Access
Returns the area of the input FeatureSet in the given units. This is a planar measurement using Cartesian mathematics.
Feature geometries in the visualization and labeling profiles are generalized according to the view's scale resolution to improve drawing performance. Therefore, using a feature's geometry (i.e. $feature
) as input to any geometry function in these contexts will return different results at each scale level. Other profiles, such as popup, provide the full resolution geometry.
Parameters
- features: FeatureSet - The FeatureSet for which to calculate the planar area.
- unit (Optional): Text | Number - Measurement unit of the return value. For the visualization, labeling, and popup profiles, the default unit is the map's spatial reference. In other profiles, like field calculation, the default is based on the spatial reference of the data.
Possible values:acres
|square-feet
|hectares
|square-kilometers
|square-miles
|square-nautical-miles
|square-meters
|square-yards
Return value: Number
Example
Returns the area of the layer in square kilometers
Area($layer, 'square-kilometers')
AreaGeodetic
AreaGeodetic(features, unit?) -> Number
Function bundle: Data Access
Returns the geodetic area of the input FeatureSet in the given units. This is more reliable measurement of area than Area()
because it takes into account the Earth's curvature. Support is limited to geometries with a Web Mercator (wkid 3857) or a WGS 84 (wkid 4326) spatial reference.
Feature geometries in the visualization and labeling profiles are generalized according to the view's scale resolution to improve drawing performance. Therefore, using a feature's geometry (i.e. $feature
) as input to any geometry function in these contexts will return different results at each scale level. Other profiles, such as popup, provide the full resolution geometry.
Parameters
- features: FeatureSet - The FeatureSet for which to calculate the geodetic area.
- unit (Optional): Text | Number - Measurement unit of the return value. For the visualization, labeling, and popup profiles, the default unit is the map's spatial reference. In other profiles, like field calculation, the default is based on the spatial reference of the data.
Possible values:acres
|square-feet
|hectares
|square-kilometers
|square-miles
|square-nautical-miles
|square-meters
|square-yards
Return value: Number
Example
Returns the geodetic area of the layer in square kilometers
AreaGeodetic($layer, 'square-kilometers')
Attachments
Attachments(inputFeature, options?) -> Array<Attachment>
Function bundle: Data Access
Profiles: Field Calculation | Form Calculation | Attribute Rules | Popups | Tasks
Returns a list of attachments associated with the input feature. Each result includes the name of the attachment, the content type, id, and size in bytes. Only applicable to features originating from a feature service.
Parameters
-
inputFeature: Feature - Attachments associated with this feature will be fetched from the service.
-
options (Optional): Dictionary - Settings for the request. Dictionary properties:
- types: Array<Text> - An array of text values representing the file types to fetch.
Possible Values:bmp
,ecw
,emf
,eps
,ps
,gif
,img
,jp2
,jpc
,j2k
,jpf
,jpg
,jpeg
,jpe
,png
,psd
,raw
,sid
,tif, tiff
,wmf
,wps
,avi
,mpg
,mpe
,mpeg
,mov
,wmv
,aif
,mid
,rmi
,mp2
,mp3
,mp4
,pma
,mpv2
,qt
,ra, ram
,wav
,wma
,doc
,docx
,dot
,xls
,xlsx
,xlt
,pdf
,ppt
,pptx
,txt
,zip
,7z
,gz
,gtar
,tar
,tgz, vrml
,gml
,json
,xml
,mdb
,geodatabase
- minsize: Number - The minimum file size of the attachment in bytes.
- maxsize: Number - The maximum file size of the attachment in bytes.
- metadata (Optional): Boolean - Indicates whether to include attachment metadata in the function return. Only Exif metadata for images is currently supported.
- types: Array<Text> - An array of text values representing the file types to fetch.
Return value: Array<Attachment>
Example
Returns the number of attachments associated with the feature
// Returns the number of attachments associated with the feature
Count(Attachments($feature))
Average
Average(features, fieldNameOrSQLExpression) -> Number
Function bundle: Data Access
Returns the average value of a given numeric field in a FeatureSet.
Parameters
- features: FeatureSet - A FeatureSet on which to perform the operation.
- fieldNameOrSQLExpression: Text - Specifies the name of a numeric field or SQL92 expression for which the statistic will be calculated from the input FeatureSet.
Return value: Number
Examples
calculates the difference between the feature's population and the average population of all features in the layer
$feature.population - Average($layer, 'population')
calculates the average population per square mile of all features in the layer
Average($layer, 'population / area')
Count
Count(features) -> Number
Function bundle: Data Access
Returns the number of features in a FeatureSet.
Parameter
- features: FeatureSet - A FeatureSet from which to count the number of features
Return value: Number
Example
Returns the number of features in a layer
Count($layer)
Crosses
Crosses(features, crossingGeometry) -> FeatureSet
Function bundle: Data Access
Returns features from a FeatureSet that cross the input geometry. In the graphic below, the red highlight illustrates the spatial relationships where the function will return features.
Feature geometries in the visualization and labeling profiles are generalized according to the view's scale resolution to improve drawing performance. Therefore, using a feature's geometry (i.e. $feature
) as input to any geometry function in these contexts will return different results at each scale level. Other profiles, such as popup, provide the full resolution geometry.
Parameters
- features: FeatureSet - The features to test the crosses relationship with the input
crossing
.Geometry - crossingGeometry: Geometry | Feature - The geometry being crossed.
Return value: FeatureSet
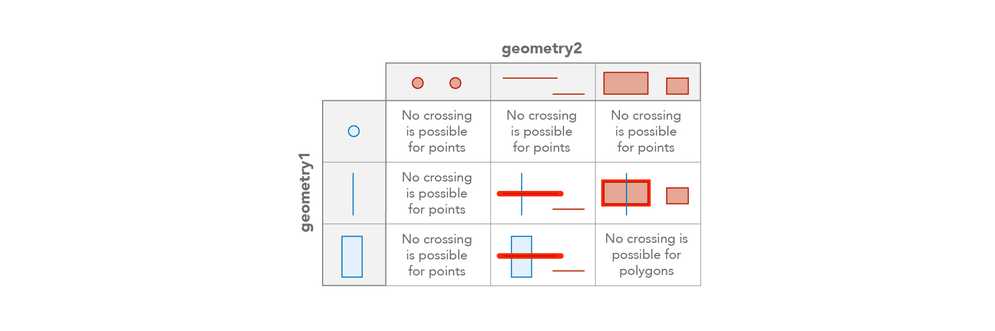
Example
Returns the number of features in the FeatureSet that cross the given polygon
var geom2 = Polygon({ ... });
Count( Crosses($layer, geom2) );
Distinct
Distinct(features, fields) -> FeatureSet
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Field Calculation | Form Calculation | Popups | Tasks
Returns a set of distinct, or unique, values from a FeatureSet.
Parameters
-
features: FeatureSet - A FeatureSet from which to return distinct values.
-
fields: Text | Dictionary | Array<Text> | Array<Dictionary> - The field(s) and/or expression(s) from which to determine unique values. This parameter can be an array of field names, an array of expressions, or a dictionary or array of dictionary that specify output column names where unique values will be stored. If a dictionary is specified, the folowing specification must be used:
Return value: FeatureSet
Examples
Returns a FeatureSet with a 'Status' column. Each row of the FeatureSet contains a unique status value
Distinct($layer, 'Status')
Returns a FeatureSet with a 'Status' and a 'Type' column. Each row of the FeatureSet contains a unique combination of 'Status' and 'Type' values
Distinct($layer, ['Status', 'Type'])
Returns FeatureSet with a Density column with rows that may contain values of Low, High, or N/A
Distinct($layer, {
name: "Density",
expression: "CASE WHEN PopDensity < 100 THEN 'Low' WHEN PopDensity >= 100 THEN 'High' ELSE 'N/A' END"
})
Returns FeatureSet with a Score and a Type column
Distinct($layer, [{
name: 'Score',
expression: 'POPULATION_DENSITY * 0.65 + Status_Code * 0.35'
}, {
name: 'Type',
expression: 'Category'
}])
Domain
Domain(features, fieldName, subtype?) -> Dictionary
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Popups | Field Calculation | Form Calculation | Tasks
Returns the domain assigned to the given field of the provided feature
. If the feature
belongs to a class with a subtype, this returns the domain assigned to the subtype.
Parameters
- features: FeatureSet - The FeatureSet whose features contain a field that has a domain.
- fieldName: Text - The name of the field (not the alias of the field) containing the domain.
- subtype (Optional): Text | Number - The coded value for the subtype if the feature supports subtypes.
Return value: Dictionary
Returns a dictionary described by the properties below.
- type: Text - The type of domain - either
coded
orValue range
. - name: Text - The domain name.
- dataType: Text - The data type of the domain field. It can be one of the following values:
esri
,Field Type Small Integer esri
,Field Type Integer esri
,Field Type B i g Integer esri
,Field Type Single esri
,Field Type Double esri
,Field Type String esri
,Field Type Date esri
,Field Type OID esri
,Field Type Geometry esri
,Field Type Blob esri
,Field Type Raster esri
,Field Type GUID esri
,Field Type Global I D esri
.Field Type XML - min: Number - Only applicable to
range
domains. The minimum value of the domain. - max: Number - Only applicable to
range
domains. The maximum value of the domain. - codedValues: Array<Dictionary> - Only applicable to
coded
domains. An array of dictionaries describing the valid values for the field. Each dictionary has aValue code
property, which contains the actual field value, and aname
property containing a user-friendly description of the value (e.g.{ code:
).1, name: "pavement" }
Example
The domain assigned to the feature's subtype
var fsPole = FeatureSetByName($layer, "Pole", 1);
var d = Domain(fsPole, "poleType")
// the poleType field has a coded value domain called poleTypes
// the value of d will be
// {
// type: "codedValue" ,
// name: "poleTypesThreePhase",
// dataType: "number",
// codedValues: [
// { name: "Unknown", code: 0 },
// { name: "Wood", code: 1 },
// { name: "Steel", code: 2 }
// { name: "Reinforced Steel", code: 3 }
// ]
// }
DomainCode
DomainCode(features, fieldName, value, subtype?) -> Number | Text
Function bundle: Data Access
Returns the code of an associated domain description in a FeatureSet.
Parameters
- features: FeatureSet - The feature set with a field that has a domain.
- fieldName: Text - The name of the field (not the alias of the field) containing the domain.
- value: Text - The value to be converted back into a code. The returned code comes from the service metadata.
- subtype (Optional): Number | Text - The coded number or name for the subtype if the feature set supports subtyping.
Example
Prints the domain description for the field referenced.
DomainCode($layer, 'Enabled', 'True', subtype)
DomainName
DomainName(features, fieldName, code?, subtype?) -> Text
Function bundle: Data Access
Returns the descriptive name for a domain code in a FeatureSet.
Parameters
- features: FeatureSet - A FeatureSet with a field that has a domain.
- fieldName: Text - The name of the field (not the alias of the field) containing the domain.
- code (Optional): Number | Text - The code associated with the desired descriptive name. The returned code comes from the service metadata.
- subtype (Optional): Number | Text - The coded number or name of the subtype if the FeatureSet supports subtyping.
Return value: Text
Example
prints the domain description for the referenced field
DomainName($layer, 'fieldName')
EnvelopeIntersects
EnvelopeIntersects(features, envelope) -> FeatureSet
Function bundle: Data Access
Returns features from a FeatureSet where the envelopes (or extent) of a set of features intersect the envelope of another geometry. In the graphic below, the red highlight illustrates the spatial relationships where the function will return features.
Feature geometries in the visualization and labeling profiles are generalized according to the view's scale resolution to improve drawing performance. Therefore, using a feature's geometry (i.e. $feature
) as input to any geometry function in these contexts will return different results at each scale level. Other profiles, such as popup, provide the full resolution geometry.
Parameters
- features: FeatureSet - The FeatureSet that is tested for the intersects relationship to the input
envelope
. - envelope: Geometry | Feature - The envelope being intersected.
Return value: FeatureSet
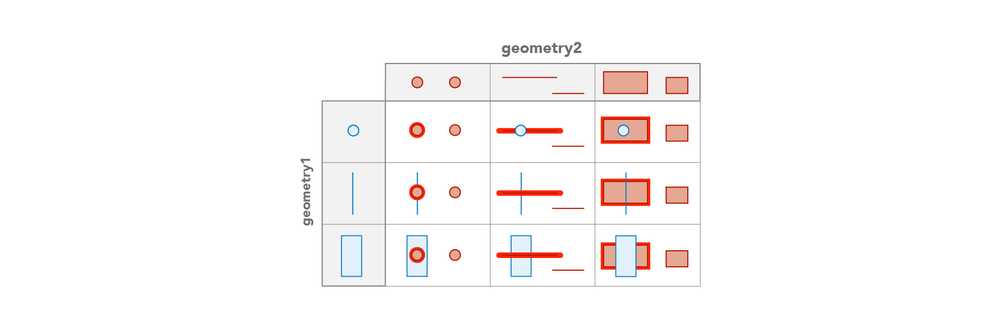
Example
Returns the number of features that intersect the envelope of geom2
var geom2 = Polygon({ ... });
Count( EnvelopeIntersects($layer, geom2) );
Expects
Expects(features, field1, [field2, ..., fieldN]?) -> Null
Function bundle: Data Access
Requests additional attributes for the given FeatureSet.
Parameters
- features: FeatureSet - The feature set to which the requested fields will be attached.
- field1: Text - A field name to request for the given feature. List only fields required for use in the expression. If necessary, you can request all fields using the wildcard
*
character. However, this should be avoided to prevent loading an unnecessary amount of data that can negatively impact app performance. - [field2, ..., fieldN] (Optional): Text - An ongoing list of field names to request for the given feature. List only fields required for use in the expression.
Return value: Null
Example
Requests the POPULATION field for the features in the cluster
// If the layer is clustered based on count,
// only the OBJECTID field is requested by default.
// To display the sum of the POPULATION field
// for all features in the cluster, we must
// explicitly request the POPULATION data.
Expects($aggregatedFeatures, 'POPULATION')
Text(Sum($aggregatedFeatures, 'POPULATION'), '#,###')
FeatureSet
This function has 2 signatures:
FeatureSet(definition) -> FeatureSet
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Popups | Field Calculation | Form Calculation | Tasks
Creates a new FeatureSet from JSON according to the ArcGIS REST spec. See the snippet below for an example of this.
Parameter
- definition: Text - The JSON describing a set of features. The JSON must be serialized as a text value.
Return value: FeatureSet
Example
Create a FeatureSet from JSON.
// JSON representation of the feature used in the snippet below
// {
// 'fields': [{
// 'alias': 'RANK',
// 'name': 'RANK',
// 'type': 'esriFieldTypeInteger'
// }, {
// 'alias': 'ELEV_m',
// 'name': 'ELEV_m',
// 'type': 'esriFieldTypeInteger'
// }],
// 'spatialReference': { 'wkid': 4326 },
// 'geometryType': 'esriGeometryPoint',
// 'features': [{
// 'geometry': {
// 'spatialReference': { 'wkid': 4326 },
// 'x': -151.0063,
// 'y': 63.069
// },
// 'attributes': {
// 'RANK': 1,
// 'ELEV_m': 6168
// }
// }]
// };
// The Dictionary representation of the FeatureSet must be a serialized text value
var features = FeatureSet('{"fields":[{"alias":"RANK","name":"RANK","type":"esriFieldTypeInteger"},{"alias":"ELEV_m","name":"ELEV_m","type":"esriFieldTypeInteger"}],"spatialReference":{"wkid":4326},"geometryType":"esriGeometryPoint","features":[{"geometry":{"spatialReference":{"wkid":4326},"x":-151.0063,"y":63.069},"attributes":{"RANK":1,"ELEV_m":6168}}]}')
FeatureSet(definition) -> FeatureSet
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Popups | Field Calculation | Form Calculation | Tasks
Creates a new FeatureSet from a Dictionary representing JSON according to the ArcGIS REST spec. See the snippet below for an example of this.
Parameter
- definition: Dictionary - A Dictionary describing a set of features.
Return value: FeatureSet
Example
Create a FeatureSet from dictionary.
// JSON representation of the feature used in the snippet below
var d = {
fields: [{
alias: 'RANK',
name: 'RANK',
type: 'esriFieldTypeInteger'
}, {
alias: 'ELEV_m',
name: 'ELEV_m',
type: 'esriFieldTypeInteger'
}],
spatialReference: { wkid: 4326 },
geometryType: 'esriGeometryPoint',
features: [{
geometry: {
spatialReference: { wkid: 4326 },
x: -151.0063,
y: 63.069
},
attributes: {
RANK: 1,
ELEV_m: 6168
}
}]
};
var features = FeatureSet(d)
FeatureSetByAssociation
FeatureSetByAssociation(inputFeature, associationType, terminalName?) -> FeatureSet
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Popups | Tasks
Returns all the features associated with the input feature as a FeatureSet. This is specific to Utility Network workflows.
Parameters
- inputFeature: Feature - The feature from which to query for all associated features. This feature must be coming from a feature service; feature collections are not supported.
- associationType: Text - The type of association with the feature to be returned.
Possible Values:connected
|container
|content
|structure
|attached
Possible Values added at version 1.10:junction
|Edge midspan
- terminalName (Optional): Text - Only applicable to
connected
association types.
Return value: FeatureSet
Returns a FeatureSet containing features with the field specification described in the table below.
- className: Text - The class name based on the value of
TONETWORKSOURCEID
orFROMNETWORKSOURCEID
. - globalId: Text - The Global ID of the feature in the other table (i.e. either the value of
TOGLOBALID
orFROMGLOBALID
). - isContentVisible: Number - Can either be a value of
1
(visible) or0
(not visible). This value represents the visibility of the associated content and is only applicable for containment associations. - objectId: Text - The ObjectID of the row in the association table.
- percentAlong: Number - Applies to
midspan
association types. Returns a floating point number from 0-1 indicating the location (as a ratio) of the junction along the edge. - side: Text - Applies to
junction
association types. Indicates which side the junction is on.Edge
Possible values: from
or to
Examples
Returns all assets that have connectivity associations with the low side terminal of the transformer.
FeatureSetByAssociation($feature, 'connected', 'Low');
Returns the number of electric devices associated with the feature
var allContent = FeatureSetByAssociation ($feature, "content");
var devicesRows = Filter(allContent, "className = 'Electric Device'");
var devicesCount = Count(devicesRows);
return devicesCount;
FeatureSetById
FeatureSetById(featureSetCollection, id, fields?, includeGeometry?) -> FeatureSet
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Popups | Field Calculation | Form Calculation | Tasks
Creates a FeatureSet from a Feature Layer based on its layer ID within a map or feature service. Limiting the number of fields in the request and excluding the geometry can improve the performance of the script.
Parameters
- featureSetCollection: FeatureSetCollection - The map or feature service containing one or more layers from which to create a FeatureSet. Typically, this value is the
$map
or$datastore
global. - id: Text - The ID of the layer within the given
map
. This layer must be created from a feature service; feature collections are not supported. Please note that this value must be a text literal. - fields (Optional): Array<Text> - The fields to include in the FeatureSet. By default, all fields are included. To request all fields in the layer, set this value to
['*']
. Limiting the number of fields improves the performance of the script. - includeGeometry (Optional): Boolean - Indicates whether to include the geometry in the features. By default, this is
true
. For performance reasons, you should only request the geometry if necessary, such as for use in geometry functions.
Return value: FeatureSet
Example
Returns the number of features in the layer with the id DemoLayerWM_1117 in the given map.
var features = FeatureSetById($map,'DemoLayerWM_1117', ['*'], true);
Count( features );
FeatureSetByName
FeatureSetByName(featureSetCollection, title, fields?, includeGeometry?) -> FeatureSet
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Popups | Field Calculation | Form Calculation | Tasks
Creates a FeatureSet from a Feature Layer based on its name within a map or feature service. Keep in mind this name is not necessarily unique. It is therefore more appropriate to create a FeatureSet using Feature
. Limiting the number of fields in the FeatureSet and excluding the geometry can improve the performance of the script.
Parameters
- featureSetCollection: FeatureSetCollection - The map or feature service containing one or more layers from which to create a FeatureSet. Typically, this value is the
$map
or$datastore
global. - title: Text - The title of the layer within the given
map
. This layer must be created from a feature service; feature collections are not supported. Please note that this value must be a text literal. - fields (Optional): Array<Text> - The fields to include in the FeatureSet. By default, all fields are included. To request all fields in the layer, set this value to
['*']
. Limiting the number of fields improves the performance of the script. - includeGeometry (Optional): Boolean - Indicates whether to include the geometry in the features. By default, this is
true
. For performance reasons, you should only request the geometry if necessary, such as for use in geometry functions.
Return value: FeatureSet
Example
Returns the number of features in the layer with the title 'Bike routes' in the given map.
var features = FeatureSetByName($map,'Bike routes', ['*'], true);
Count(features);
FeatureSetByRelationshipClass
FeatureSetByRelationshipClass(inputFeature, relationshipClass, fieldNames?, includeGeometry?) -> FeatureSet
Function bundle: Data Access
Profiles: Attribute Rules
Returns the related records for a given feature based on the name of a given relationship class.
Parameters
- inputFeature: Feature - The feature from which to fetch related records.
- relationshipClass: Text - The name of the relationship class as defined in the data source.
- fieldNames (Optional): Array<Text> - The fields to return in the FeatureSet. This list includes fields from both the relationship table and the input Feature.
- includeGeometry (Optional): Boolean - Indicates whether to return the geometry for the resulting features.
Return value: FeatureSet
Additional resources
Example
Returns the count of pole inspection related records
// A calculation rule that returns the count of a pole inspection records.
// When a pole feature is updated the calculation rule reads all its related inspections records from the comments field and returns the total inspection count for that feature.
var fsinspected = FeatureSetByRelationshipClass($feature, “pole_inspection”, [“comments”], false);
return Count(fsinspected);
FeatureSetByRelationshipName
FeatureSetByRelationshipName(inputFeature, relationshipName, fieldNames?, includeGeometry?) -> FeatureSet
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Field Calculation | Form Calculation | Popups | Tasks
Returns the related records for a given feature as a FeatureSet.
Parameters
- inputFeature: Feature - The feature for which to fetch related records.
- relationshipName: Text - The name of the relationship according to the feature service associated with the given feature.
- fieldNames (Optional): Array<Text> - The fields to return in the FeatureSet. This list includes fields from both the relationship table and the input Feature.
- includeGeometry (Optional): Boolean - Indicates whether to return the geometry for the resulting features.
Return value: FeatureSet
Example
Returns the sum of several fields across all related records
var results = FeatureSetByRelationshipName($feature, 'Election_Results', ['*'], false)
Sum(results, 'democrat + republican + other')
Filter
Filter(features, sqlExpression) -> FeatureSet
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Popups | Field Calculation | Form Calculation | Tasks
Creates a new FeatureSet with all the features that pass the SQL92 expression filter.
Parameters
- features: FeatureSet - The FeatureSet, or layer, to filter.
- sqlExpression: Text - The SQL92 expression used to filter features in the layer. This expression can substitute an Arcade variable using the
@
character. See the snippet below for an example.
Return value: FeatureSet
Examples
Filter features using a SQL92 expression
// Returns all features with a Population greater than 10,000
var result = Filter($layer, 'POPULATION > 10000');
Filter features using a SQL92 expression with a variable substitute
// Returns all features with a Population greater than the dataset average
var averageValue = Average($layer, 'POPULATION')
var result = Filter($layer, 'POPULATION > @averageValue');
FilterBySubtypeCode
FilterBySubtypeCode(features, subtypeCode) -> FeatureSet
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Field Calculation | Form Calculation | Popups | Tasks
Creates a new FeatureSet with all the features matching the given subtype code.
Parameters
- features: FeatureSet - The FeatureSet, or layer, to filter.
- subtypeCode: Number - The subtype code used to filter features in the FeatureSet or layer.
Return value: FeatureSet
Example
Filter features with the subtype code
// Returns all features that have the given subtype code
FilterBySubtypeCode($layer, 5)
First
First(features) -> Feature
Function bundle: Data Access
Returns the first feature in a FeatureSet. Returns null
if the FeatureSet is empty.
Parameter
- features: FeatureSet - The FeatureSet from which to return the first feature.
Return value: Feature
Example
returns the area of the first feature in the layer.
Area( First($layer) )
GdbVersion
GdbVersion(features) -> Text
Function bundle: Data Access
Returns the name of the current geodatabase version for branch or versioned data. When the data is not in a multi-user geodatabase, an empty text value will be returned.
Parameter
- features: FeatureSet - A FeatureSet from which to return the current geodatabase version.
Return value: Text
Additional resources
Example
Returns the geodatabase version of the given FeatureSet
GdbVersion($layer)
GetFeatureSet
GetFeatureSet(inputFeature, source?) -> FeatureSet
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Popups | Field Calculation | Form Calculation | Tasks
Gets the FeatureSet in which the input feature belongs. The returned FeatureSet represents all features from the input feature's parent/root layer or table.
Parameters
-
inputFeature: Feature - The feature belonging to a parent or root FeatureSet.
-
source (Optional): Text - Indicates the source FeatureSet to return.
Possible Values:datasource
: (default) Returns all the features from the input feature's data source without any filters or definition expressions as a FeatureSet.root
: Returns the initial FeatureSet to which the input feature belongs. This may be a filtered subset of all the features in the data source.parent
: Returns the parent FeatureSet of the input feature. This can be a smaller set of features than the original data source or root FeatureSet.
Return value: FeatureSet
Examples
Returns a FeatureSet representing all the features in the data source.
// The data source for the 'Bike routes' layer has 2,000 features.
// The map sets a definition expression on the 'Bike routes' layer and filters it to 100 features.
var fs1 = FeatureSetByName($map, 'Bike routes', ['*'], true);
var fs2 = top(fs1, 10)
var f = First(fs2)
GetFeatureSet(f)
// returns a FeatureSet representing the data source with 2,000 features
Returns the root FeatureSet of the feature.
// The data source for the 'Bike routes' layer has 2,000 features.
// The map sets a definition expression on the 'Bike routes' layer and filters it to 100 features.
var fs1 = FeatureSetByName($map, 'Bike routes', ['*'], true);
var fs2 = top(fs1, 10)
var f = First(fs2)
GetFeatureSet(f, 'root')
// returns the root FeatureSet representing 100 features
Returns the parent FeatureSet of the feature.
// The data source for the 'Bike routes' layer has 2,000 features.
// The map sets a definition expression on the 'Bike routes' layer and filters it to 100 features.
var fs1 = FeatureSetByName($map, 'Bike routes', ['*'], true);
var fs2 = top(fs1, 10)
var f = First(fs2)
GetFeatureSet(f, 'parent')
// returns the parent FeatureSet representing 10 features
Returns the number of features in the data source table within 1 mile of the feature.
var fullFeatureSet = GetFeatureSet($feature);
var featuresOneMile = Intersects(fullFeatureSet, BufferGeodetic($feature, 1, 'miles'))
Count(featuresOneMile)
GetFeatureSetInfo
GetFeatureSetInfo(inputFeatureSet) -> Dictionary
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Popups | Field Calculation | Form Calculation | Tasks
Returns metadata for the original source database and service (if applicable) of a FeatureSet.
Parameter
- inputFeatureSet: FeatureSet - The FeatureSet from which to fetch metadata.
Return value: Dictionary
Returns a dictionary described by the properties below.
- layerId: Number - The layerId of the feature service. Only applicable to FeatureSets created from feature services. This value will be
null
for sde/filegdb/mobile workspaces. - layerName: Text - The layer name of the feature service. Only applicable to FeatureSets created from feature services. This value will be
null
for sde/filegdb/mobile workspaces. - itemId: Text - The portal item ID of the feature service. Only applicable to FeatureSets created from feature services that have an associated portal item. This value will be
null
for sde/filegdb/mobile workspaces. - serviceLayerUrl: Text - The url of the feature service layer. Only applicable to FeatureSets created from feature services. This value will be
null
for sde/filegdb/mobile workspaces. - webMapLayerId: Text - The layerId of the associated layer within the context of a web map. Only applicable to FeatureSets created from feature service layers that are contained within a web map. This value will be
null
for sde/filegdb/mobile workspaces. - webMapLayerTitle: Text - The title of the associated layer within the context of a web map. Only applicable to FeatureSets created from feature service layers that are contained within a web map. This value will be
null
for sde/filegdb/mobile workspaces. - className: Text - The name of the underlying feature class. Only applicable to FeatureSets created from feature classes in filegdb/mobile workspaces.
- objectClassId: Number - The objectClassId. Only applicable to FeatureSets created from feature classes in filegdb workspaces.
Examples
Metadata returned from a FeatureSet connected to an underlying feature service
// $layer originates from a feature service layer in a web map
GetFeatureSetInfo($layer);
// returns the following:
{
"layerId": 7,
"layerName": "My Table",
"itemId": "dda795cf2af44d2bb7af2827963b76e8",
"serviceLayerUrl": "https://utilitynetwork.esri.com/server/rest/services/ClassicNapervilleElectric_Postgres/FeatureServer/100",
"webMapLayerId": 1,
"webMapLayerTitle": "MyTable1",
"className": null,
"objectClassId": null
}
Metadata returned from a FeatureSet originating from a filegdb or mobilegdb
// $featureset originates from a feature class in a filegdb or mobilegdb
GetFeatureSetInfo($featureset);
// returns the following:
{
"layerId": null,
"layerName": null,
"itemId": null,
"serviceLayerUrl": null,
"webMapLayerId": null,
"webMapLayerTitle": null,
"className": "myTable",
"objectClassId": 7
}
Metadata returned from a FeatureSet connected to an sde workspace (client server direct connection)
// Client server direct connection (sqlserver/oracle/etc.)
GetFeatureSetInfo($featureset);
// returns the following:
{
"layerId": null,
"layerName": null,
"itemId": null,
"serviceLayerUrl": null,
"webMapLayerId": null,
"webMapLayerTitle": null,
"className": "owner.myTable",
"objectClassId": 7
}
GetUser
This function has 2 signatures:
GetUser(features, username?) -> Dictionary
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Popups | Field Calculation | Form Calculation | Tasks
Returns the current user from the workspace. For data from a service, either the Portal user or Server user is returned. For data from a database connection, the database user is returned. When no user is associated with the workspace, such as a file geodatabase, a null
value will be returned.
Parameters
- features: FeatureSet - A FeatureSet from which to return the current user.
- username (Optional): Text - The username of the user you want to return. Only limited information will be returned based on your permissions when making the request.
Return value: Dictionary
Returns a dictionary described by the properties below.
- id: Text - The user id of the returned user.
- username: Text - The username of the returned user.
- fullName: Text - The user's first and last name.
- email: Text - The email address associated with the user's account.
- groups: Array<Text> - An array of groups that the user belongs to.
- role: Text - The role that the user plays within their organization (e.g. Administrator, Publisher, User, Viewer, or Custom).
- privileges: Array<Text> - An array of privileges that the user has within their organization (e.g. edit, view, etc).
Examples
Returns information about the user "tester".
GetUser($layer, "tester")
// returns {"id": "12", "username": "tester", "name":"Testy Tester", "email": "tester@example.com", ...}
Returns username for the currently logged in user of the active portal. If no user is associated with the portal, this will return null
.
var userInfo = GetUser($layer);
if(HasValue(userInfo, "username")){
return userInfo.username;
}
GetUser(features, extensions?) -> Dictionary
Function bundle: Data Access
Profiles: Attribute Rules | Popups | Field Calculation | Form Calculation | Tasks
Returns the current user from the workspace. For data from a service, either the Portal user or Server user is returned. For data from a database connection, the database user is returned. When no user is associated with the workspace, such as a file geodatabase, a null
value will be returned.
Parameters
- features: FeatureSet - A FeatureSet from which to return the current user.
- extensions (Optional): Boolean - Determines if the
user
will be returned in the dictionary.License Type Extensions
Return value: Dictionary
Returns a dictionary described by the properties below.
- id: Text - The user id of the returned user.
- username: Text - The username of the returned user.
- fullName: Text - The user's first and last name.
- email: Text - The email address associated with the user's account.
- groups: Array<Text> - An array of groups that the user belongs to.
- role: Text - The role that the user plays within their organization (e.g. Administrator, Publisher, User, Viewer, or Custom).
- privileges: Array<Text> - An array of privileges that the user has within their organization (e.g. edit, view, etc).
- userLicenseTypeExtensions: Array<Text> - An array of the license type extensions associated with the user's account (e.g. "Utility Network", "Parcel Fabric", etc). The
extensions
parameter must be set totrue
in order for this to be returned.
Example
Returns information about the user currently logged in based on the workspace connection from a layer with user extensions.
GetUser($layer, true)
GroupBy
GroupBy(features, groupByFields, statistics) -> FeatureSet
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Field Calculation | Form Calculation | Popups | Tasks
Returns statistics as a FeatureSet for a set of grouped or distinct values.
Parameters
-
features: FeatureSet - A FeatureSet from which to return statistics for unique values returned from a given set of fields and/or expressions.
-
groupByFields: Text | Array<Text> | Array<Dictionary> - The field(s) and/or expression(s) from which to group statistics by unique values. This parameter can be a single field name, an array of field names, or an array of objects that specify column names paired with an expression (typically the field name) for the output FeatureSet. If an array of objects is specified, the following specification must be followed for each object:
-
statistics: Dictionary | Array<Dictionary> - The summary statistics to calculate for each group. This parameter can be a dictionary or array of dictionary that specify output statistics to return for each group. The following specification must be used:
- name: Text - The name of the column to store the result of the given statistical query in the output FeatureSet.
- expression: Text - A SQL-92 expression or field name from which to query statistics.
- statistic: Text - The statistic type to query for the given field or expression.
Possible Values: SUM | COUNT | MIN | MAX | AVG | STDEV | VAR
Return value: FeatureSet
Examples
Returns the count of each tree type
var treeStats = GroupBy($layer, 'TreeType', { name: 'NumTrees', expression: '1', statistic: 'COUNT' });
// treeStats contains features with columns TreeType and NumTrees
// Each unique tree type will have a count
Returns the count and the average height of each tree type
var treeStats = GroupBy($layer,
[ // fields/expressions to group statistics by
{ name: 'Type', expression: 'TreeType'},
{ name: 'Status', expression: 'TreeStatus'}
],
[ // statistics to return for each unique category
{ name: 'Total', expression: '1', statistic: 'COUNT' },
{ name: 'AvgHeight', expression: 'Height', statistic: 'AVG' },
{ name: 'MaxPercentCoverage', expression: 'CoverageRatio * 100', statistic: 'MAX' }
]
);
// treeStats contains features with columns Type, Status, Total, AvgHeight, MaxPercentCoverage
// Each unique tree type (combination of type and status) will have a count, average height, and maximum value of percent coverage
Intersects
Intersects(features, inputGeometry) -> FeatureSet
Function bundle: Data Access
Returns features from a FeatureSet that intersect another geometry. In the graphic below, the red highlight illustrates the spatial relationships where the function will return features.
Feature geometries in the visualization and labeling profiles are generalized according to the view's scale resolution to improve drawing performance. Therefore, using a feature's geometry (i.e. $feature
) as input to any geometry function in these contexts will return different results at each scale level. Other profiles, such as popup, provide the full resolution geometry.
Parameters
- features: FeatureSet - The FeatureSet that is tested for the intersects relationship to
geometry
. - inputGeometry: Geometry | Feature - The geometry being intersected.
Return value: FeatureSet
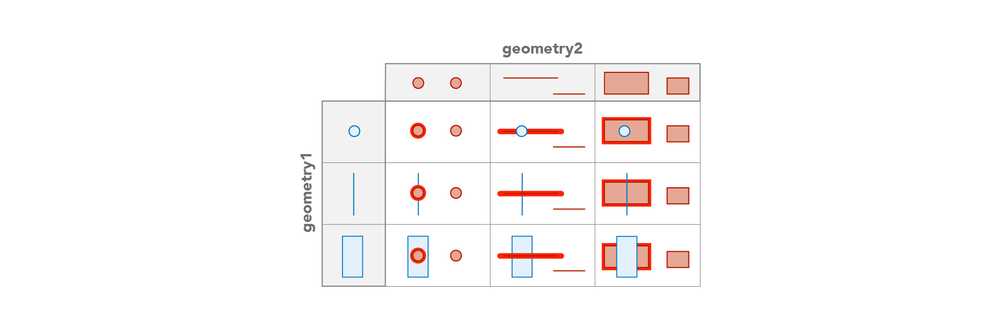
Example
Returns the number of features that intersect the polygon
var geom2 = Polygon({ ... });
Count( Intersects($layer, geom2) );
Length
Length(features, unit?) -> Number
Function bundle: Data Access
Returns the length of the input FeatureSet in the given units. This is a planar measurement using Cartesian mathematics.
Feature geometries in the visualization and labeling profiles are generalized according to the view's scale resolution to improve drawing performance. Therefore, using a feature's geometry (i.e. $feature
) as input to any geometry function in these contexts will return different results at each scale level. Other profiles, such as popup, provide the full resolution geometry.
Parameters
- features: FeatureSet - The FeatureSet for which to calculate the planar length.
- unit (Optional): Text | Number - Measurement unit of the return value. For the visualization, labeling, and popup profiles, the default unit is the map's spatial reference. In other profiles, like field calculation, the default is based on the spatial reference of the data.
Possible values:feet
|kilometers
|miles
|nautical-miles
|meters
|yards
Return value: Number
Example
Returns the planar length of the layer in meters
Length($layer, 'meters')
Length3D
Length3D(features, unit?) -> Number
Function bundle: Data Access
Profiles: Attribute Rules | Popups | Field Calculation | Form Calculation | Tasks
Returns the planar (i.e. Cartesian) length of the input FeatureSet taking height or Z information into account. The geometry provided to this function must be assigned a projected coordinate system. If the spatial reference does not provide a value for Z units, then the result will be returned in meters. Keep in mind that not all clients (such as the 3.x series of the ArcGIS API for JavaScript) support requesting Z values even when the data contains Z information.
Feature geometries in the visualization and labeling profiles are generalized according to the view's scale resolution to improve drawing performance. Therefore, using a feature's geometry (i.e. $feature
) as input to any geometry function in these contexts will return different results at each scale level. Other profiles, such as popup, provide the full resolution geometry.
Parameters
- features: FeatureSet - The FeatureSet for which to calculate the planar length in 3D space.
- unit (Optional): Text | Number - Measurement unit of the return value. For the visualization, labeling, and popup profiles, the default unit is the map's spatial reference. In other profiles, like field calculation, the default is based on the spatial reference of the data.
Possible values:feet
|kilometers
|miles
|nautical-miles
|meters
|yards
Return value: Number
Example
Returns the 3D length of the layer's features in meters
Length3D($layer, 'meters')
LengthGeodetic
LengthGeodetic(features, unit?) -> Number
Function bundle: Data Access
Returns the geodetic length of the input FeatureSet in the given units. This is more reliable measurement of length than Length()
because it takes into account the Earth's curvature. Support is limited to geometries with a Web Mercator (wkid 3857) or a WGS 84 (wkid 4326) spatial reference.
Feature geometries in the visualization and labeling profiles are generalized according to the view's scale resolution to improve drawing performance. Therefore, using a feature's geometry (i.e. $feature
) as input to any geometry function in these contexts will return different results at each scale level. Other profiles, such as popup, provide the full resolution geometry.
Parameters
- features: FeatureSet - The FeatureSet for which to calculate the geodetic length.
- unit (Optional): Text | Number - Measurement unit of the return value. For the visualization, labeling, and popup profiles, the default unit is the map's spatial reference. In other profiles, like field calculation, the default is based on the spatial reference of the data.
Possible values:feet
|kilometers
|miles
|nautical-miles
|meters
|yards
Return value: Number
Additional resources
Example
Returns the geodetic length of the layer in meters
LengthGeodetic($layer, 'meters')
Max
Max(features, fieldNameOrSQLExpression) -> Number
Function bundle: Data Access
Returns the highest value for a given numeric field from a FeatureSet.
Parameters
- features: FeatureSet - A FeatureSet on which to perform the operation.
- fieldNameOrSQLExpression: Text - Specifies the name of a numeric field or SQL92 expression for which the statistic will be calculated from the input FeatureSet.
Return value: Number
Examples
prints the max value of the population field for all features in the layer
Max($layer, 'population')
calculates the max population per square mile of all features in the layer
Max($layer, 'population / area')
Mean
Mean(features, fieldNameOrSQLExpression) -> Number
Function bundle: Data Access
Returns the mean value of a given numeric field in a FeatureSet.
Parameters
- features: FeatureSet - A FeatureSet on which to calculate the mean.
- fieldNameOrSQLExpression: Text - Specifies the name of a numeric field or SQL92 expression for which the statistic will be calculated from the input FeatureSet.
Return value: Number
Examples
calculates the difference between the feature's population and the mean population of all features in the layer
$feature.population - Mean($layer, 'population')
calculates the mean population per square mile of all features in the layer
Mean($layer, 'population / area')
Min
Min(features, fieldNameOrSQLExpression) -> Number
Function bundle: Data Access
Returns the lowest value for a given numeric field from a FeatureSet.
Parameters
- features: FeatureSet - A FeatureSet on which to perform the operation.
- fieldNameOrSQLExpression: Text - Specifies the name of a numeric field or SQL92 expression for which the statistic will be calculated from the input FeatureSet.
Return value: Number
Examples
prints the min value of the population field for all features in the layer
Min($layer, 'population')
returns the minimum population per square mile of all features in the layer
Min($layer, 'population / area')
OrderBy
OrderBy(features, sqlExpression) -> FeatureSet
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Popups | Field Calculation | Form Calculation | Tasks
Orders a FeatureSet by using a SQL92 OrderBy clause.
Parameters
- features: FeatureSet - The FeatureSet, or layer, to order.
- sqlExpression: Text - The SQL92 expression used to order features in the layer.
Return value: FeatureSet
Examples
Order features by population where features with the highest population are listed first
OrderBy($layer, 'POPULATION DESC')
Order features by rank in ascending order
OrderBy($layer, 'Rank ASC')
Overlaps
Overlaps(overlappingFeatures, inputGeometry) -> FeatureSet
Function bundle: Data Access
Returns features from a FeatureSet that overlap another geometry. In the graphic below, the red highlight illustrates the spatial relationships where the function will return features.
Feature geometries in the visualization and labeling profiles are generalized according to the view's scale resolution to improve drawing performance. Therefore, using a feature's geometry (i.e. $feature
) as input to any geometry function in these contexts will return different results at each scale level. Other profiles, such as popup, provide the full resolution geometry.
Parameters
- overlappingFeatures: FeatureSet - The features that are tested for the 'overlaps' relationship with
geometry
. - inputGeometry: Geometry | Feature - The comparison geometry that is tested for the 'overlaps' relationship with
overlapping
.Features
Return value: FeatureSet
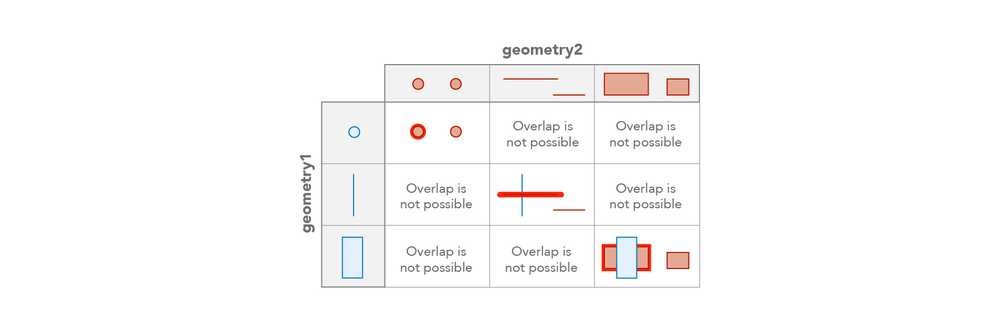
Example
Returns the number of features that overlap the polygon
var geom2 = Polygon({ ... });
Count( Overlaps($layer, geom2) );
Schema
Schema(features) -> Dictionary
Function bundle: Data Access
Profiles: Attribute Rules | Dashboard Data | Popups | Field Calculation | Form Calculation | Velocity | Tasks
Returns the schema description of the provided FeatureSet.
Parameter
- features: FeatureSet - The FeatureSet whose schema to return.
Return value: Dictionary
Returns a dictionary described by the properties below.
- objectIdField: Text - The objectId field of the FeatureSet.
- globalIdField: Text - The global ID field of the FeatureSet. Returns
""
if not globalId-enabled. - geometryType: Text - The geometry type of features in the FeatureSet. Returns
esri
for tables with no geometry.Geometry Null
Possible values:esri
,Geometry Point esri
,Geometry Line esri
,Geometry Polygon esri
Geometry Null - fields: Array<Dictionary> - Returns an array of dictionaries describing the fields in the FeatureSet. Each dictionary describes the field
name
,alias
,type
,subtype
,domain
,length
, and whether it iseditable
andnullable
.
Stdev
Stdev(features, fieldNameOrSQLExpression) -> Number
Function bundle: Data Access
Returns the standard deviation for the values from a given numeric field in a FeatureSet.
Parameters
- features: FeatureSet - A FeatureSet on which to perform the operation.
- fieldNameOrSQLExpression: Text - Specifies the name of a numeric field or SQL92 expression for which the statistic will be calculated from the input FeatureSet.
Return value: Number
Examples
prints the standard deviation of values from the 'population' field
Stdev($layer, 'population')
calculates the standard deviation of the population per square mile of all features in the layer
Stdev($layer, 'population / area')
Subtypes
Subtypes(features) -> Dictionary
Function bundle: Data Access
Returns the subtype coded value Dictionary. Returns null
when subtypes are not enabled on the layer.
Parameter
- features: FeatureSet - The FeatureSet from which to get subtypes.
Return value: Dictionary
Returns a dictionary described by the properties below.
- subtypeField: Text - The field containing a subtype.
- subtypes: Array<Dictionary> - An array of dictionaries describing the subtypes. Each dictionary has a
code
property, which contains the actual field value, and aname
property containing a user-friendly description of the value (e.g.{ code:
)1, name: "pavement" }
Example
Returns subtypes with coded values from a FeatureSet
var fsTransformer = FeatureSetByName($layer, "Transformer")
Subtypes(fsTransformer)
// returns the following dictionary
// {
// subtypeField: 'assetGroup',
// subtypes: [
// { name: "Unknown", code: 0 },
// { name: "Single Phase", code: 1 },
// { name: "Two Phase", code: 2 }
// ]
// }
Sum
Sum(features, fieldNameOrSQLExpression) -> Number
Function bundle: Data Access
Returns the sum of values returned from a given numeric field in a FeatureSet.
Parameters
- features: FeatureSet - A FeatureSet on which to perform the operation.
- fieldNameOrSQLExpression: Text - Specifies the name of a numeric field or SQL92 expression for which the statistic will be calculated from the input FeatureSet.
Return value: Number
Examples
calculates the population of the current feature as a % of the total population of all features in the layer
( $feature.population / Sum($layer, 'population') ) * 100
calculates the total number of votes cast in an election for the entire dataset
Sum($layer, 'democrat + republican + other')
Top
Top(features, numItems) -> FeatureSet
Function bundle: Data Access
Truncates the FeatureSet and returns the first given number of features.
Parameters
- features: FeatureSet - The FeatureSet to truncate.
- numItems: Number - The number of features to return from the beginning of the FeatureSet.
Return value: FeatureSet
Example
Returns the top 5 features with the highest population
Top( OrderBy($layer, 'POPULATION DESC'), 5 )
Touches
Touches(touchingFeatures, inputGeometry) -> FeatureSet
Function bundle: Data Access
Returns features from a FeatureSet that touch another geometry. In the graphic below, the red highlight illustrates the spatial relationships where the function will return features.
Feature geometries in the visualization and labeling profiles are generalized according to the view's scale resolution to improve drawing performance. Therefore, using a feature's geometry (i.e. $feature
) as input to any geometry function in these contexts will return different results at each scale level. Other profiles, such as popup, provide the full resolution geometry.
Parameters
- touchingFeatures: FeatureSet - The features to test the 'touches' relationship with
geometry
. - inputGeometry: Geometry | Feature - The geometry to test the 'touches' relationship with
touching
.Features
Return value: FeatureSet
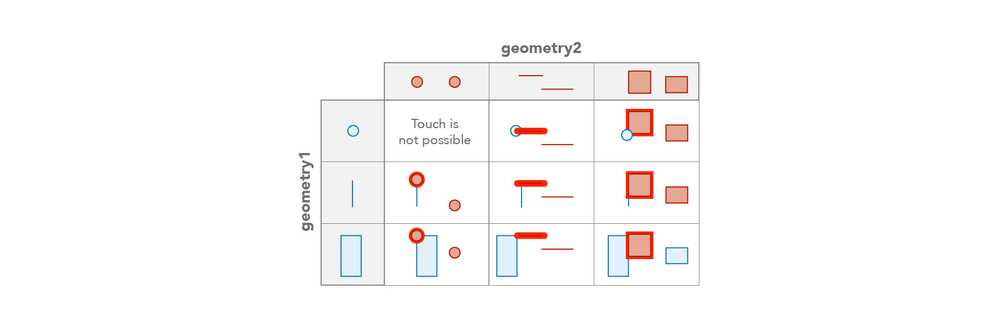
Example
Returns the number of features in the layer that touch the geometry.
var geom = Polygon({ ... });
Count( Touches($layer, geom) );
Variance
Variance(features, fieldNameOrSQLExpression) -> Number
Function bundle: Data Access
Returns the variance of the values from a given numeric field in a FeatureSet.
Parameters
- features: FeatureSet - A FeatureSet on which to perform the operation.
- fieldNameOrSQLExpression: Text - Specifies the name of a numeric field or SQL92 expression for which the statistic will be calculated from the input FeatureSet.
Return value: Number
Examples
prints the variance for the population field in the given layer
Variance($layer, 'population')
calculates the variance of the population per square mile of all features in the layer
Variance($layer, 'population / area')
Within
Within(innerGeometry, outerFeatures) -> FeatureSet
Function bundle: Data Access
Returns features from a FeatureSet that contain the inner
. In the graphic below, the red highlight illustrates the spatial relationships where the function will return features.
Feature geometries in the visualization and labeling profiles are generalized according to the view's scale resolution to improve drawing performance. Therefore, using a feature's geometry (i.e. $feature
) as input to any geometry function in these contexts will return different results at each scale level. Other profiles, such as popup, provide the full resolution geometry.
Parameters
- innerGeometry: Geometry | Feature - The base geometry that is tested for the 'within' relationship to
outer
.Features - outerFeatures: FeatureSet - The comparison features that are tested for the 'contains' relationship to
inner
.Geometry
Return value: FeatureSet
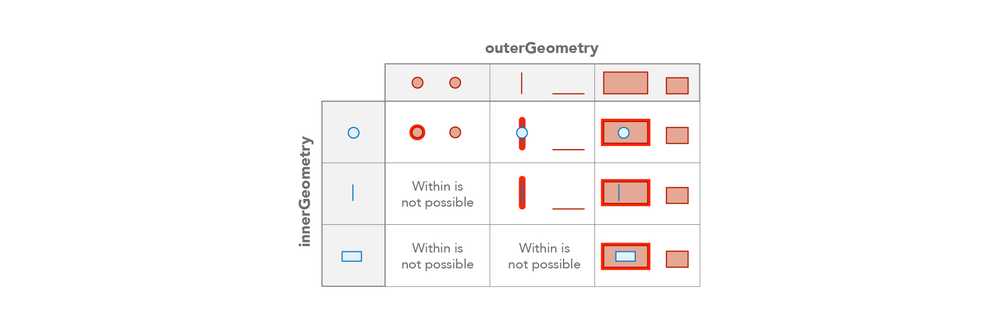
Example
Returns the number of features in the layer within the polygon
var outerGeom = Polygon({ ... });
Count( Within(outerGeom, $layer) );