GeoAnalytics Track Expressions are only available when using GeoAnalytics within specific track-enabled tools.
The following functions allow you to create and evaluate expressions with track inputs in GeoAnalytics tools. Tracks are sequentially ordered features with a field specified as a track identifier.
TrackAccelerationAt - TrackAccelerationWindow - TrackCurrentAcceleration - TrackCurrentDistance - TrackCurrentSpeed - TrackCurrentTime - TrackDistanceAt - TrackDistanceWindow - TrackDuration - TrackFieldWindow - TrackGeometryWindow - TrackIndex - TrackSpeedAt - TrackSpeedWindow - TrackStartTime - TrackWindow
TrackAccelerationAt
TrackAccelerationAt(value) -> Number
Function bundle: Track
Profiles: GeoAnalytics | Velocity
The acceleration at the observation relative to the current observation.
Parameter
- value: Number - The number of features before or after the current observation.
The current feature is index 0. Positive values represent features that occur in the future, after the current value. For example, position 1 is the next value in the array. Negative numbers represent features that occurred in the past, before the current feature. For example, -1 is the previous value in the array.
Return value: Number
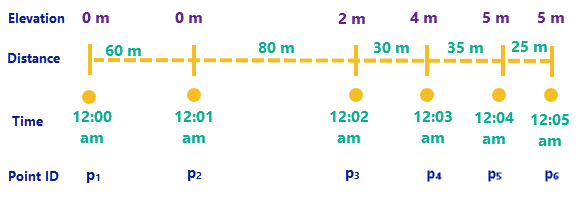
Examples
Your track has six features, as seen above. The expression returns a number for each feature representing the acceleration value in meters per second squared. In this example, we examine results of feature 1 (p1) with a value
of 1. The result is equal to the acceleration of feature 2 (p2).
var accelerationAt = TrackAccelerationAt(1)
accelerationAt;
// returns 0.0167
Your track has six features, as seen above. The expression returns a number for each feature representing the acceleration value in meters per second squared. In this example, we examine results of feature 1 (p1) with a value
of 3. The result is equal to the acceleration of feature 4 (p4).
var accelerationAt = TrackAccelerationAt(3)
accelerationAt;
// returns -0.0014
TrackAccelerationWindow
TrackAccelerationWindow(startIndex, endIndex) -> Array<Number>
Function bundle: Track
Profiles: GeoAnalytics | Velocity
The acceleration values between the first value (inclusive) to the last value (exclusive) in a window around the current observation (0).
Parameters
- startIndex: Number - The index of the beginning feature. The current feature is index 0. Positive values represent features that occur in the future, after the current value. For example, position 1 is the next value in the array. Negative numbers represent features that occurred in the past, before the current feature. For example, -1 is the previous value in the array.
- endIndex: Number - The index of the feature at the end of the window. The current feature is index 0. Positive values represent features that occur in the future, after the current value. For example, position 1 is the next value in the array. Negative numbers represent features that occurred in the past, before the current feature. For example, -1 is the previous value in the array.
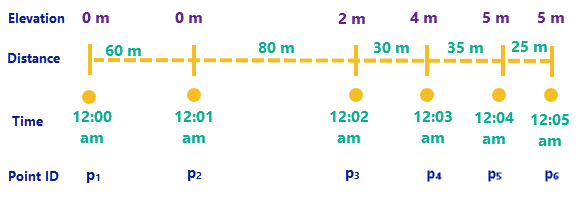
Examples
Your track has six features, as seen above. The expression returns an array containing the acceleration value for each feature in the specified window. Accelerations are calculated in meters per second squared. In this example, we examine results of feature 3 (p3) when evaluated with a start
of -1
and an end
of 2
.
var accelerationWindow = TrackAccelerationWindow(-1, 2)
accelerationWindow;
// returns [0.0167, 0.0056, -0.0014]
Your track has six features, as seen above. The expression returns an array containing the acceleration value for each feature in the specified window. Accelerations are calculated in meters per second squared. In this example, we examine results of feature 3 (p3) when evaluated with a start
of 1
and an end
of 3
.
var accelerationWindow = TrackAccelerationWindow(1, 3)
accelerationWindow;
// returns [-0.0014, 0.0014, -0.0028]
TrackCurrentAcceleration
TrackCurrentAcceleration() -> Number
Function bundle: Track
Profiles: GeoAnalytics | Velocity
The acceleration of the current observation measured between the previous observation and the current observation.
Return value: Number
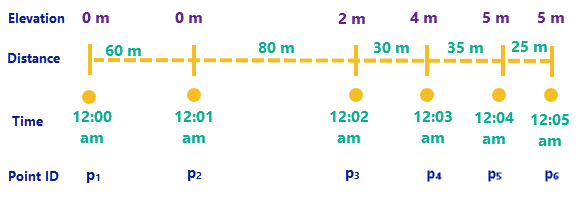
Examples
Your track has six features, as seen above. The expression returns a number for each feature representing the acceleration value in meters per second squared. In the first example, we examine results of feature 2 (p2).
var currentAcceleration = TrackCurrentAcceleration()
currentAcceleration;
// returns 0.0167
Your track has six features, as seen above. The expression returns a number for each feature representing the acceleration value in meters per second squared. In the following example, we examine results of feature 4 (p4).
var currentAcceleration = TrackCurrentAcceleration()
currentAcceleration;
// returns -0.0014
TrackCurrentDistance
TrackCurrentDistance() -> Number
Function bundle: Track
Profiles: GeoAnalytics | Velocity
The sum of the distances travelled between observations from the first to current observation.
Return value: Number
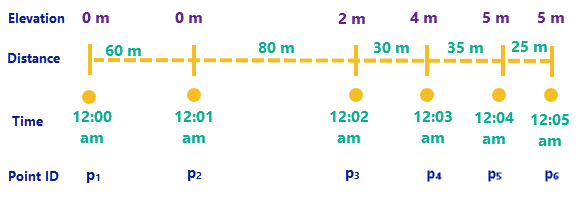
Examples
Your track has six features, as seen above. The expression returns a value for the current feature in the track. In the first example, we examine results for feature 3 (p3). The calculation is 80 + 60 = 140
.
var currentDistance = TrackCurrentDistance()
currentDistance;
// returns 140
Your track has six features, as seen above. The expression returns a value for the current feature in the track. Your track has six features, as seen above. The expression returns a value for each feature in the track. In the following example, we examine results for feature 6 (p6). The calculation is 25 + 35 + 30 + 80 + 60 = 230
.
var currentDistance = TrackCurrentDistance()
currentDistance;
// returns 230
TrackCurrentSpeed
TrackCurrentSpeed() -> Number
Function bundle: Track
Profiles: GeoAnalytics | Velocity
The speed between the previous observation and the current observation.
Return value: Number
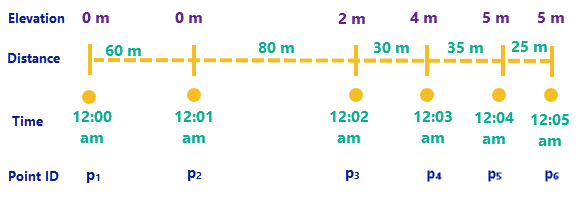
Examples
Your track has six features, as seen above. The expression returns a number for each feature representing the speed calculated in meters per second. In the first example, we examine results of feature 2 (p2). The calculation is 60/60
.
var currentSpeed = TrackCurrentSpeed()
currentSpeed;
// returns 1
Your track has six features, as seen above. The expression returns a number for each feature representing the speed calculated in meters per second. In the following example, we examine results of feature 6 (p6). The calculation is 25/60
.
var currentSpeed = TrackCurrentSpeed()
currentSpeed;
// returns 0.4167
TrackCurrentTime
TrackCurrentTime() -> Date
Function bundle: Track
Profiles: GeoAnalytics | Velocity
Calculates the time on the current feature in a track.
Return value: Date
Example
Returns the time of the current feature being evaluated. For example, given a track with three features at January 1, 2012, December 9, 2012, and May 3, 2013 the current time will be evaluated for each feature. In this example, it's evaluated at the middle feature, December 9, 2012.
TrackCurrentTime();
// returns December 9, 2012
TrackDistanceAt
TrackDistanceAt(index) -> Number
Function bundle: Track
Profiles: GeoAnalytics | Velocity
The sum of the distances travelled between observations from the first to the current observation plus the given value.
Parameter
- index: Number - The index of the track feature to calculate distance for. For example, a value of
2
would calculate the distance from the first feature (index0
) in the track to the third feature (index2
) in the track.
Return value: Number
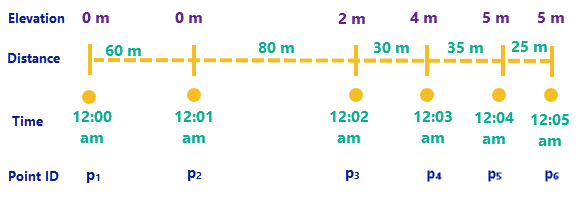
Examples
Your track has six features, as seen above. The expression returns a value for each feature in the track. In the first example, we examine results when evaluated at feature 2 (p2) with an index value of 2. The calculation is 30 + 80 + 60 = 170
.
TrackDistanceAt(2)
// returns 170
Your track has six features, as seen above. The expression returns a value for each feature in the track. In the following example, we examine results when evaluated at feature 4 (p4) with an index value of 4. The calculation is 25 + 35 + 30 + 80 + 60 = 230
.
TrackDistanceAt(4)
// returns 230
TrackDistanceWindow
TrackDistanceWindow(startIndex, endIndex) -> Array<Number>
Function bundle: Track
Profiles: GeoAnalytics | Velocity
The distances between the first value (inclusive) to the last value (exclusive) in a window about the current observation (0).
Parameters
- startIndex: Number - The index of the beginning feature. The current feature is index 0. Positive values represent features that occur in the future, after the current value. For example, position 1 is the next value in the array. Negative numbers represent features that occurred in the past, before the current feature. For example, -1 is the previous value in the array.
- endIndex: Number - The index of the feature at the end of the window. The current feature is index 0. Positive values represent features that occur in the future, after the current value. For example, position 1 is the next value in the array. Negative numbers represent features that occurred in the past, before the current feature. For example, -1 is the previous value in the array.
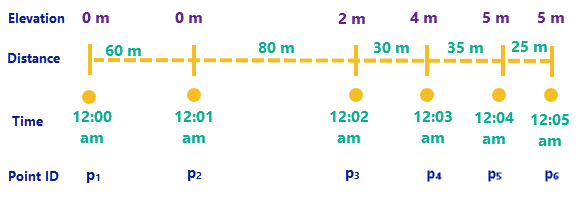
Examples
Your track has six features, as seen above. The expression returns an array containing the distance value for each feature in the window. In the first example, we examine results of feature 3 (p3) when evaluated with a start
of -1
and an end
of 2
.
var distanceWindow = TrackDistanceWindow(-1, 2)
distanceWindow;
// returns [60, 140, 170]
Your track has six features, as seen above. The expression returns an array containing the distance value for each feature in the window. In the following example, we examine results of feature 5 (p5) when evaluated with a start
of -1
and an end
of 2
.
var distanceWindow = TrackDistanceWindow(-1, 2)
distanceWindow;
// returns [170, 205, 230]
TrackDuration
TrackDuration() -> Number
Function bundle: Track
Profiles: GeoAnalytics | Velocity
Calculates the duration of the track from the start feature to the current feature in milliseconds from epoch.
Return value: Number
Example
Returns the duration of a track that starts on January 1, 2012 until the current feature at May 3, 2013.
TrackDuration();
// returns 42163200000
TrackFieldWindow
TrackFieldWindow(fieldName, startIndex, endIndex) -> Array<Number>
Function bundle: Track
Profiles: GeoAnalytics | Velocity
Returns an array of attribute values from the specified field
for the specified time span. The window function allows you to go forward and backward in time.
Parameters
- fieldName: Text - The field name from which to return values.
- startIndex: Number - The index of the beginning feature. The current feature is index
0
. Positive values represent features that occur in the future, after the current value. For example, position1
is the next value in the array. Negative numbers represent features that occurred in the past, before the current feature. For example,-1
is the previous value in the array. - endIndex: Number - The index of the feature at the end of the window. The current feature is index
0
. Positive values represent features that occur in the future, after the current value. For example, position1
is the next value in the array. Negative numbers represent features that occurred in the past, before the current feature. For example,-1
is the previous value in the array.
Examples
Your track has a field with sequentially ordered values of [10, 20, 30, 40, 50]
. The geometries of the features are [{x:
. The expression is evaluated at each feature in the track. Results are returned inclusive of the start feature, and exclusive of the end feature. This example is evaluated at the second feature (20) and returns an array of the previous value (-1, inclusive).
var window = TrackFieldWindow('MyField', -1,0)
window;
// returns [10]
Your track has a field named Speed
with sequentially ordered values of [10, 20, 30, 40, 50]
. The geometries of the features are [{x:
. The expression is evaluated at each feature in the track. For this example, we examine results when evaluated at the third feature (30). Results are returned inclusive of the start feature, and exclusive of the end feature.
var window = TrackFieldWindow('Speed', -2,2)
window;
// returns [10,20,30,40]
TrackGeometryWindow
TrackGeometryWindow(startIndex, endIndex) -> Array<Geometry>
Function bundle: Track
Profiles: GeoAnalytics | Velocity
Returns an array of geometries for the specified time indices. The window function allows you to go forward and backward in time.
Parameters
- startIndex: Number - The index of the beginning feature. The current feature is index
0
. Positive values represent features that occur in the future, after the current value. For example, position1
is the next value in the array. Negative numbers represent features that occurred in the past, before the current feature. For example,-1
is the previous value in the array. - endIndex: Number - The index of the feature at the end of the window. The current feature is index
0
. Positive values represent features that occur in the future, after the current value. For example, position1
is the next value in the array. Negative numbers represent features that occurred in the past, before the current feature. For example,-1
is the previous value in the array.
Example
Your track has a field with sequentially ordered values of [10, 20, 30, 40, 50]
. The geometries of the features are [{x:
. The expression is evaluated at each feature in the track. For this example, we examine results when evaluated at the third feature (30). Results are returned inclusive of the start feature, and exclusive of the end feature
var window = TrackGeometryWindow(-2,2)
window;
// returns [{x: 1, y: 1},{x: 2, y: 2} ,{x: null, y: null},{x: 4, y: 4}]
TrackIndex
TrackIndex() -> Number
Function bundle: Track
Profiles: GeoAnalytics | Velocity
Returns the index of the feature being calculated. Features are indexed in order of time within a track.
Return value: Number
Example
Returns the index of the first feature in a track.
TrackIndex() // returns 0
TrackSpeedAt
TrackSpeedAt(value) -> Number
Function bundle: Track
Profiles: GeoAnalytics | Velocity
The speed at the observation relative to the current observation. For example, at value 2, it's the speed at the observation two observations after the current.
Parameter
- value: Number - The number of features before or after the current observation. The current feature is index 0. Positive values represent features that occur in the future, after the current value. For example, position 1 is the next value in the array. Negative numbers represent features that occurred in the past, before the current feature. For example, -1 is the previous value in the array.
Return value: Number
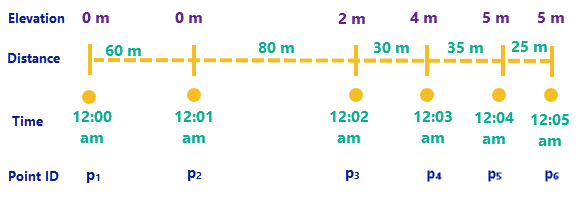
Examples
Your track has six features, as seen above. The expression returns a number for each feature representing the speed calculated in meters per second. In the first example, we examine results of feature 1 (p1) with a value
of 2. The calculation is 80/60
.
var speedAt = TrackSpeedAt(2)
speedAt;
// returns 1.33
Your track has six features, as seen above. The expression returns a number for each feature representing the speed calculated in meters per second. In the following example, we examine results of feature 3 (p3) with a value
of -1. The calculation is 60/60
.
var speedAt = TrackSpeedAt(2)
speedAt;
// returns 1
TrackSpeedWindow
TrackSpeedWindow(startIndex, endIndex) -> Array<Number>
Function bundle: Track
Profiles: GeoAnalytics | Velocity
The speed values between the first value (inclusive) to the last value (exclusive) in a window around the current observation (0).
Parameters
- startIndex: Number - The index of the beginning feature. The current feature is index 0. Positive values represent features that occur in the future, after the current value. For example, position 1 is the next value in the array. Negative numbers represent features that occurred in the past, before the current feature. For example, -1 is the previous value in the array.
- endIndex: Number - The index of the feature at the end of the window. The current feature is index 0. Positive values represent features that occur in the future, after the current value. For example, position 1 is the next value in the array. Negative numbers represent features that occurred in the past, before the current feature. For example, -1 is the previous value in the array.
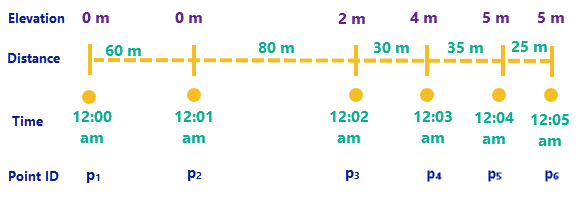
Examples
Your track has six features, as seen above. The expression returns an array containing the speed value for each feature in the specified window. Speeds are calculated in meters per second. In this example, we examine results of feature 3 (p3) when evaluated with a start
of -1
and an end
of 2
.
var speedWindow = TrackSpeedWindow(-1, 2)
speedWindow // returns [1, 1.3, 0.5]
Your track has six features, as seen above. The expression returns an array containing the speed value for each feature in the specified window. Speeds are calculated in meters per second. In this example, we examine results of feature 3 (p3) when evaluated with a start
of 1
and an end
of 3
.
var speedWindow = TrackSpeedWindow(1,3)
speedWindow // returns [0.5, 0.583, 0.4167]
TrackStartTime
TrackStartTime() -> Date
Function bundle: Track
Profiles: GeoAnalytics | Velocity
Calculates the start time of a track.
Return value: Date
Example
Returns the start time of a track that spans from January 1, 2012 to May 3, 2013.
TrackStartTime() // returns January 1, 2012
TrackWindow
TrackWindow(startIndex, endIndex) -> Array<Feature>
Function bundle: Track
Profiles: GeoAnalytics | Velocity
Returns an array of features for the specified time index. This function allows you to go forward and backward in time.
Parameters
- startIndex: Number - The index of the beginning feature. The current feature is index
0
. Positive values represent features that occur in the future, after the current value. For example, position1
is the next value in the array. Negative numbers represent features that occurred in the past, before the current feature. For example,-1
is the previous value in the array. - endIndex: Number - The index of the feature at the end of the window. The current feature is index
0
. Positive values represent features that occur in the future, after the current value. For example, position1
is the next value in the array. Negative numbers represent features that occurred in the past, before the current feature. For example,-1
is the previous value in the array.
Examples
Your track has a field with sequentially ordered values of [10, 20, 30, 40, 50]
. The geometries of the features are [{x:
. The expression is evaluated at each feature in the track. Results are returned inclusive of the start feature, and exclusive of the end feature. This example is evaluated at the second feature (20) and returns an array of a single value -- the previous feature.
var window = TrackWindow(-1,0)
window;
// returns [{'geometry': {x: 1, y: 1}}, {'attributes': {'MyField' : 10, 'trackName':'ExampleTrack1'}}]
Your track has a field with sequentially ordered values of [10, 20, 30, 40, 50]
. The geometries of the features are [{x:
. The expression is evaluated at each feature in the track. For this example, we examine results when evaluated at the third feature (30). Results are returned inclusive of the start feature, and exclusive of the end feature.
var window = TrackWindow(-2,2)
window;
/* returns
[{
geometry: [{
x: 1,
y: 1
}, {
x: 2,
y: 2
}, {
x: null,
y: null
}, {
x: 4,
y: 4
}]
}, {
attributes: [{
MyField: 10,
trackName: 'ExampleTrack1'
}, {
MyField: 20,
trackName: 'ExampleTrack1'
}, {
MyField: 30,
trackName: 'ExampleTrack1'
}, {
MyField: 40,
trackName: 'ExampleTrack1'
}]
}]