Learn how to use an item to access and display a feature layer in a map.
You can host a variety of geographic data and other resources using ArcGIS Online. These items can also define how the data is presented. A web map or web scene, for example, not only defines the layers for a map or scene, but also how layers are symbolized, the minimum and/or maximum scales at which they display, and several other properties. Likewise, a hosted feature layer contains the data for the layer and also defines the symbols and other display properties for how it is presented. When you add a map, scene, or layer from a portal item to your app, everything that has been saved with the item is applied in your application. Adding items to your application rather than creating them programmatically saves you from writing a lot of code, and can provide consistency across applications that use the same data.
In this tutorial, you will add a hosted feature layer to display trailheads in the Santa Monica Mountains of Southern California. The hosted layer defines the trailhead locations (points) as well as the symbols used to display them.
Prerequisites
The ArcGIS API for Python tutorials use Jupyter Notebooks to execute Python code. If you are new to this environment, please see the guide to install the API and use notebooks locally.
Steps
Import modules and log in
-
Import the
arcgis.gis
module. This module is the entry point into the GIS and provides the functionality to manage GIS content, users, and groups.Use dark colors for code blocks from arcgis.gis import GIS
-
Log in anonymously to access publicly shared content. The data used in this tutorial is public so you do not need credentials to access it. If it were private data, you would be required to provide authentication.
Use dark colors for code blocks from arcgis.gis import GIS gis = GIS()
Access the item by ID
-
Create a variable to hold the item ID of the public dataset. An item hosted in ArcGIS has a unique item ID attribute that can be referenced. For example, the item ID for this feature layer is:
Use dark colors for code blocks gis = GIS() trailheads_id = "2e4b3df6ba4b44969a3bc9827de746b3"
-
Use the
content
property on theGIS
class, passing in thetrailheads_
variable to return anid item
object.Use dark colors for code blocks trailheads_id = "2e4b3df6ba4b44969a3bc9827de746b3" trailheads_item = gis.content.get(trailheads_id) trailheads_item
Display the item on a map
-
Create a map widget instance by calling the
map
method. Pass the item object to theadd_
method to display the layer.layer Use dark colors for code blocks trailheads_item = gis.content.get(trailheads_id) trailheads_item m = gis.map() m.add_layer(trailheads_item) m
-
Use the
center
andzoom
methods to set the display extent of the map.Use dark colors for code blocks m = gis.map() m.add_layer(trailheads_item) m m.center = [34.09042, -118.71511] # [latitude, longitude] m.zoom = 11
Your map should display the locations of trailheads in the Santa Monica Mountains.
Optional: Export the map widget
- Create a standalone HTML page that can be shared and rendered in a web browser.
Use dark colors for code blocks from os import path, getcwd export_dir = path.join(getcwd(), "home") if not path.isdir(export_dir): os.mkdir(export_dir) export_path = path.join(export_dir, "add-a-layer-from-an-item.html") m.export_to_html(export_path, title="Add a layer from an item")
What's next?
Learn how to use additional functionality in these tutorials:
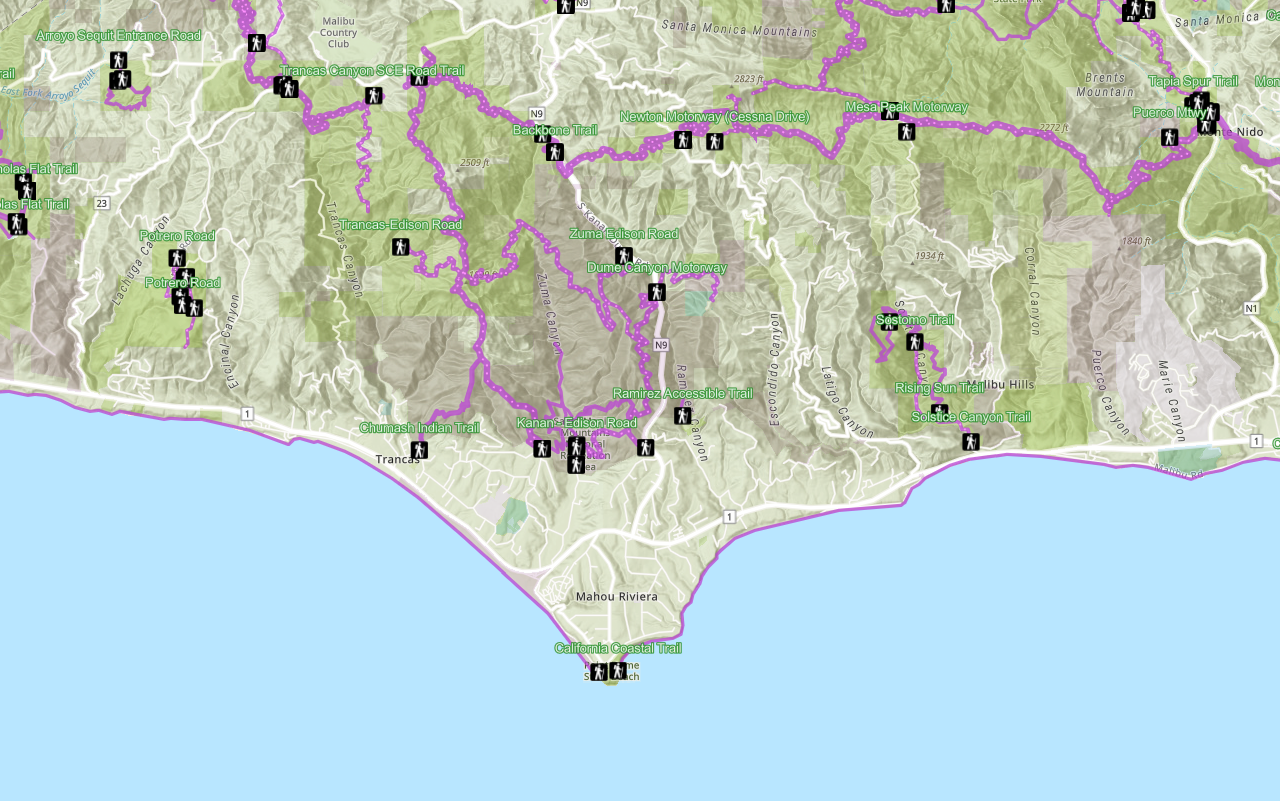
Display a web map
Search ArcGIS Online for an existing web map and display it.
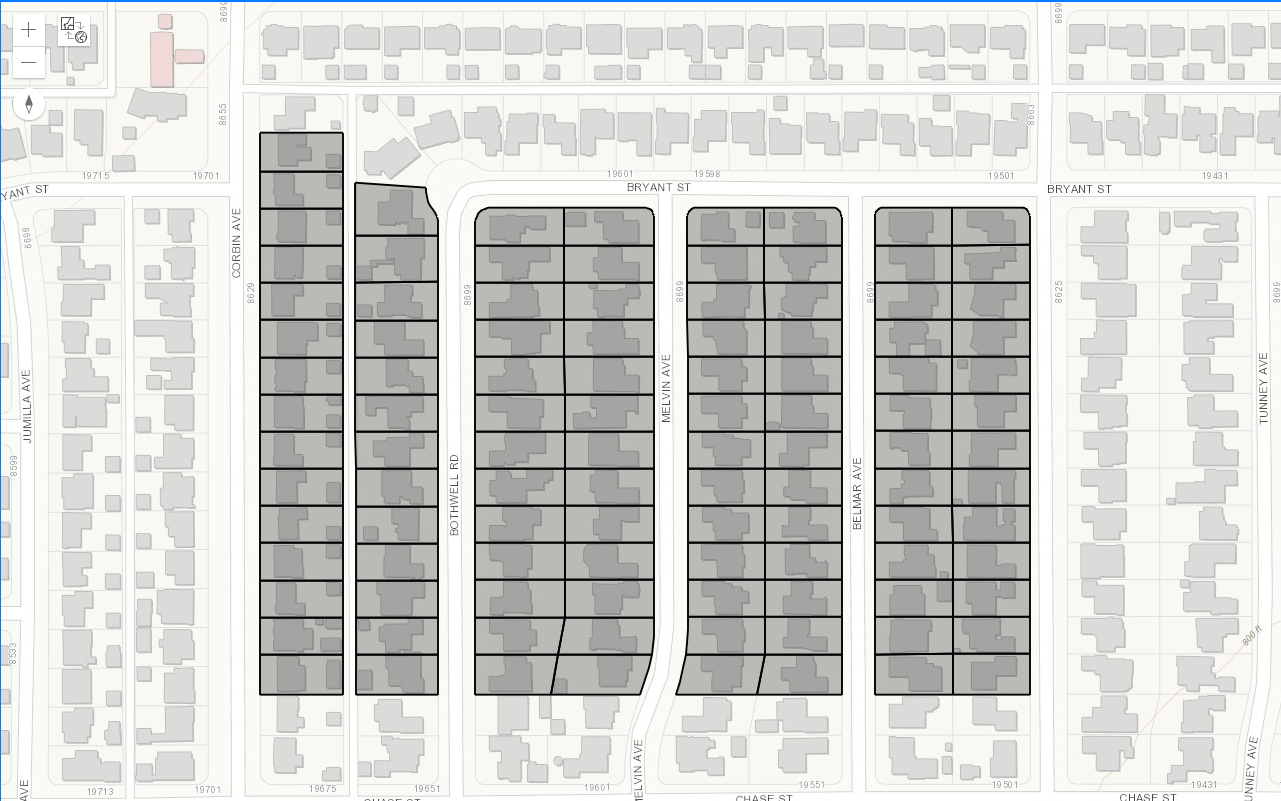
Query a feature layer (spatial)
Learn how to execute a spatial query to access polygon features from feature services.
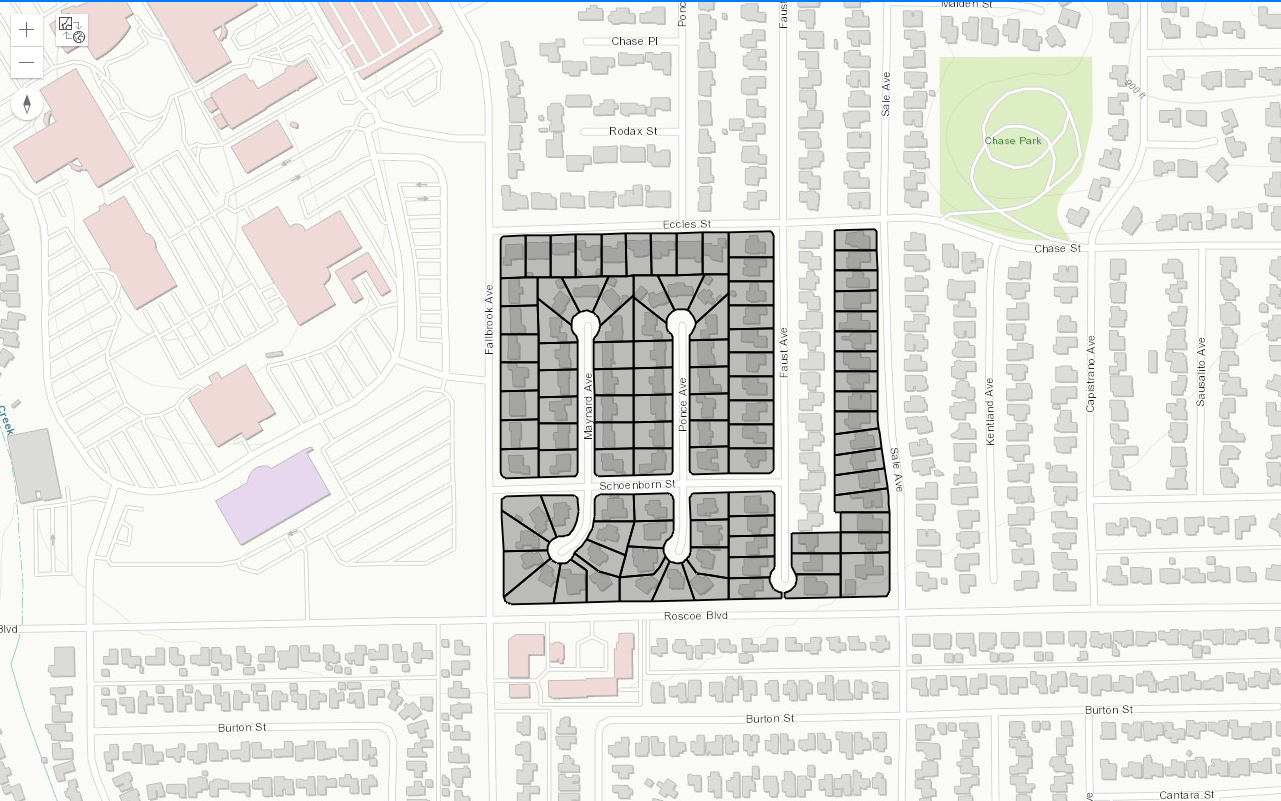
Query a feature layer (SQL)
Learn how to execute a SQL query to access polygon features from feature services.