Learn how to execute a spatial query to access features from a feature layer.
A feature layer can contain a large number of features. To access a subset of these features, you can execute an SQL or spatial query, either together or individually. The results can contain the attributes, geometry, or both for each record. SQL and spatial queries are useful when a feature layer is very large and you want to access only a subset of its data.
In this tutorial, you access a hosted feature layer and query the service with a geometry and spatial operator to only return intersecting records.
Prerequisites
The ArcGIS API for Python tutorials use Jupyter Notebooks to execute Python code. If you are new to this environment, please see the guide to install the API and use notebooks locally.
Steps
Import modules and log in
-
Import the
arcgis.gis
module.Use dark colors for code blocks from arcgis.gis import GIS
-
Log in anonymously to ArcGIS Online to access publicly shared data. Since this dataset is public you do not need credentials to access it. If it were a private dataset, you would be required to log in
Use dark colors for code blocks from arcgis.gis import GIS portal = GIS()
Access the feature layer
-
Use the
Content
class to access the dataset by Item ID.Manager Use dark colors for code blocks portal = GIS() parcel_layer_item = portal.content.get("a6fdf2ee0e454393a53ba32b9838b303") parcel_layer = parcel_layer_item.layers[0]
Run the query
- Create an
Extent
object as a geometry filter. Thecontains
method will return only the features that are wholly contained within this extent. Thea
parameter is set tos_ df False
so that the results will be returned asFeature
. Set theSet out_
as an array of field names to be included in the results.fields Use dark colors for code blocks parcel_layer_item = portal.content.get("a6fdf2ee0e454393a53ba32b9838b303") parcel_layer = parcel_layer_item.layers[0] from arcgis.geometry.filters import contains query_extent = { "xmin": -13198303.18, "ymin": 4059062.83, "xmax": -13197797.98, "ymax": 4059421.13, "spatialReference": {"wkid": 102100}, } query_filter = contains(query_extent, sr=102100) results = parcel_layer.query( geometry_filter=query_filter, out_fields="APN, UseType", as_df=False ) results
Display the results
-
Use the
map
method to create a map widget. Use theadd_
method to add the resultslayer() Feature
to the map and theSet zoom_
method to set the maps extent so the query results are visible.t o_ layer() Use dark colors for code blocks from arcgis.geometry.filters import contains query_extent = { "xmin": -13198303.18, "ymin": 4059062.83, "xmax": -13197797.98, "ymax": 4059421.13, "spatialReference": {"wkid": 102100}, } query_filter = contains(query_extent, sr=102100) results = parcel_layer.query( geometry_filter=query_filter, out_fields="APN, UseType", as_df=False ) results map = portal.map() map map.add_layer(results) map.zoom_to_layer(results)
-
Optional: Use the
export_
method to export the current state of the map widget to a static HTML file which can be viewed in any web browser.t o_ html Use dark colors for code blocks map = portal.map() map map.add_layer(results) map.zoom_to_layer(results) import os file_path = os.path.join(os.getcwd(), "home", "query-a-feature-layer-sql.html") map.export_to_html(file_path, title="Query a feature layer (SQL)")
When the map displays, the features returned from the query are displayed in the center of the map. Click on a parcel to show a pop-up with the features attributes.
What's next?
Learn how to use additional functionality in these tutorials:
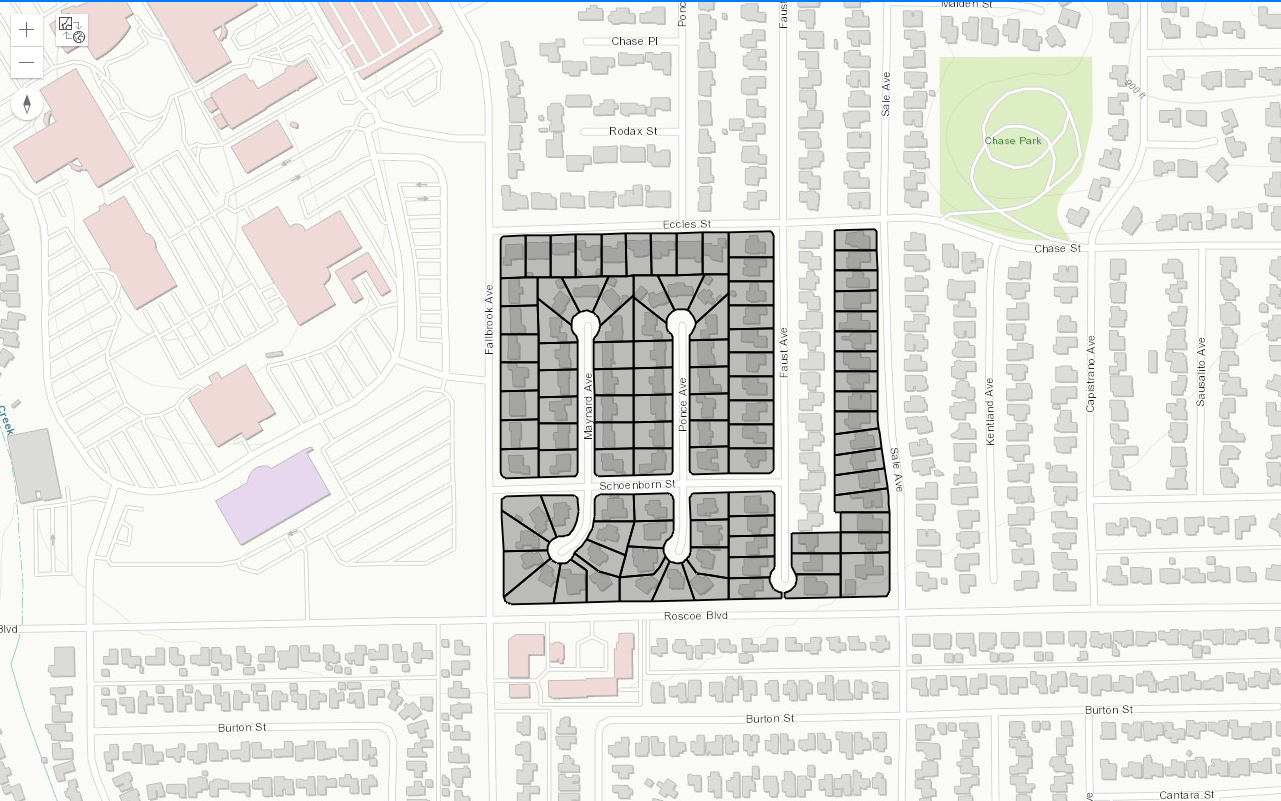
Query a feature layer (SQL)
Learn how to execute a SQL query to access polygon features from feature services.
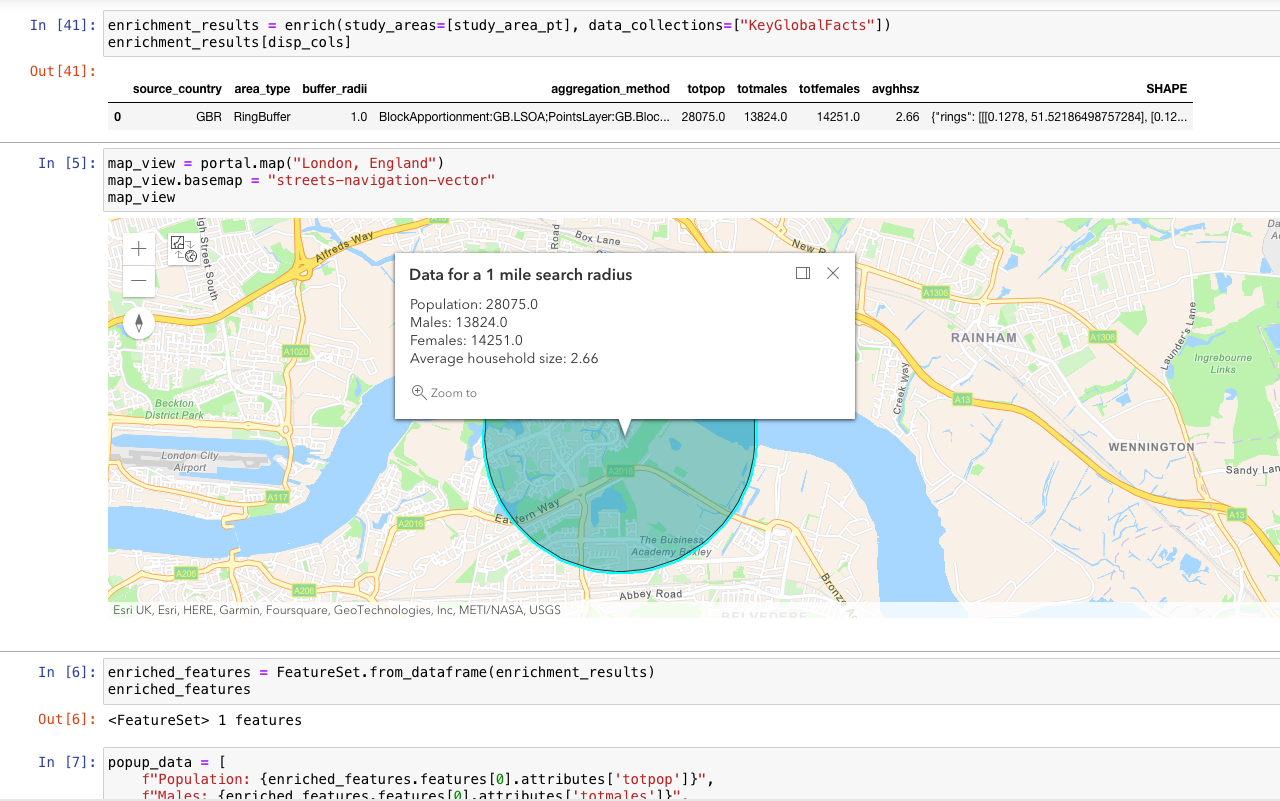
Get global data
Query demographic information for locations around the world with the GeoEnrichment service.
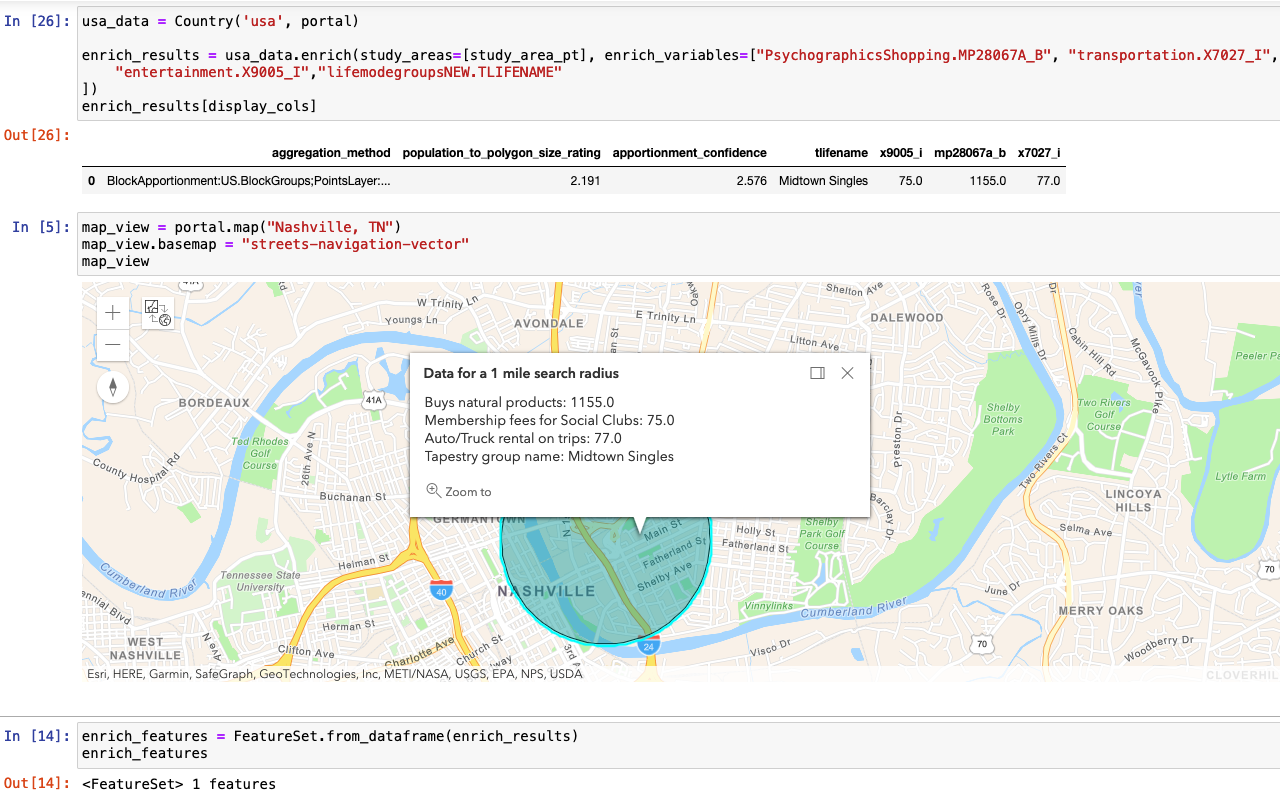
Get local data
Query local analysis variables in select countries around the world with the GeoEnrichment service.
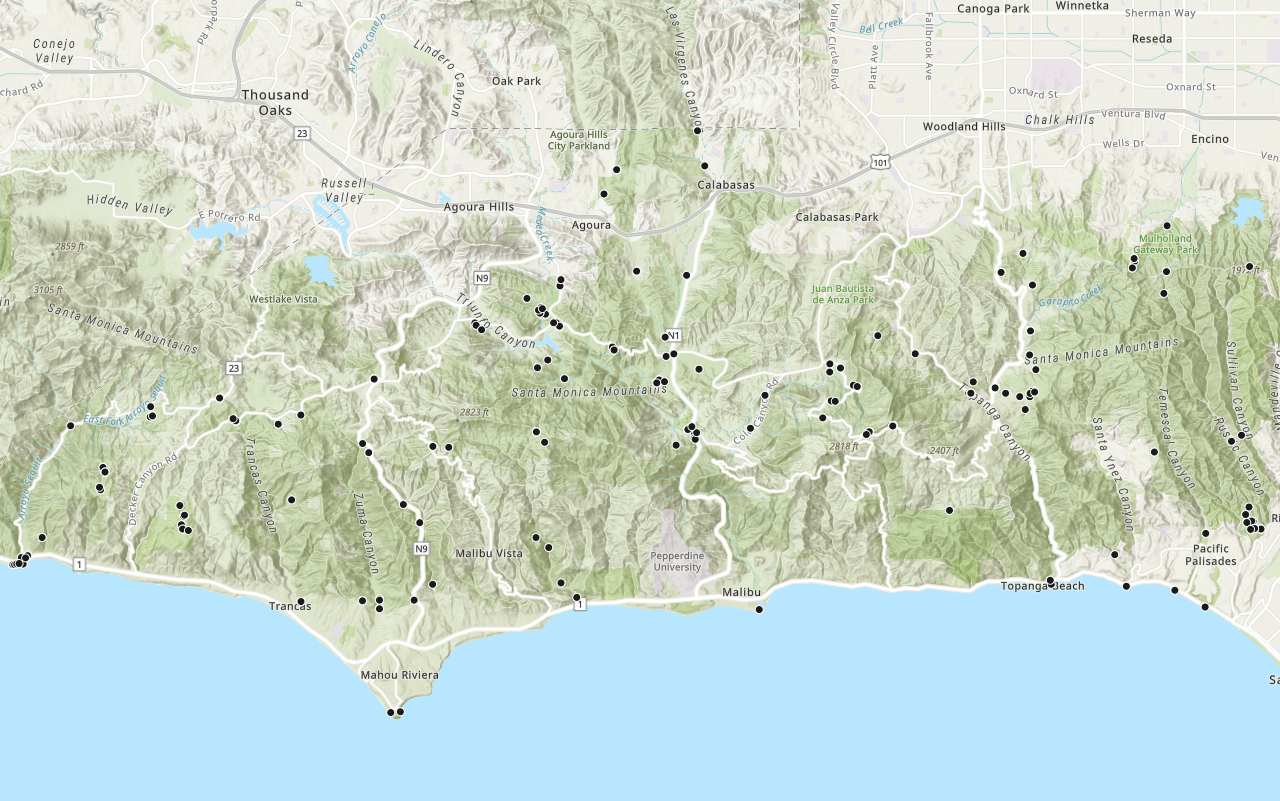
Add a layer from a portal item
Learn how to use a portal item to access and display point features from a feature service.