This sample shows the different properties that can be set on a PathSymbol3DLayer to create 3D visualization styles for Polyline features.
With a combination of profile, width and height, several styles can be created:
Wall style
This style extrudes the line vertically, which can be used to visualize trajectories or spatial separators like a boundaries or barriers.
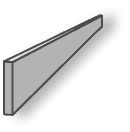
To generate this path style you need to set the profile to quad
and have a height larger than the width. This style works best when anchor is set to bottom
and profileRotation is set to heading
:
const wallSymbol = {
type: "line-3d",
symbolLayers: [{
type: "path",
profile: "quad",
material: {
color: [100, 100, 100]
},
width: 5, // the width in m
height: 30, // the height in m
anchor: "bottom", // the vertical anchor is set to the lowest point of the wall
profileRotation: "heading"
}]
}
Strip style
The strip style can be used for line features like roads, networks, flight lines etc.
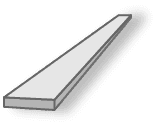
To create a path with a strip style the profile should be set to quad
and the height should be smaller than the width. The anchor is not set, so it defaults to center
.
Example:
const stripSymbol = {
type: "line-3d",
symbolLayers: [{
type: "path",
profile: "quad",
material: {
color: [100, 100, 100]
},
width: 30, // the width in m
height: 5, // the height in m
profileRotation: "heading"
}]
}
Round tube style
This style could be used to display utility pipelines or subway lines.
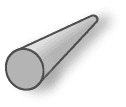
The profile for this style is "circle" and the width should have the same value as the height. The anchor is not set, so it defaults to center
.
Example:
const roundTubeSymbol = {
type: "line-3d",
symbolLayers: [{
type: "path",
profile: "circle",
material: {
color: [100, 100, 100]
},
width: 30, // the width in m
height: 30 // the height in m
}]
}
Square tube style
This style is suitable for displaying a tunnel, an air corridor or any other feature with a rectangular tube-like shape.
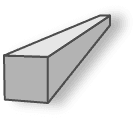
Create this by setting the profile to quad
and having the same width and height. The anchor is not set, so it defaults to center
.
Example:
const squareTubeSymbol = {
type: "line-3d",
symbolLayers: [{
type: "path",
profile: "quad",
material: {
color: [100, 100, 100]
},
width: 30, // the width in m
height: 30 // the height in m
}]
}
Another important visual aspect is how the start and end point of a path or the segment junctions look like. The documentation for cap and join properties on the PathSymbol3DLayer explains how the different values influence the path style.
The height/width and color of a path can also be driven by attributes using visual variables, which might be useful for visualizing data along trajectories. This use case is not covered by this sample, but to see how to do this, read the SizeVariable or the ColorVariable documentation.