This sample demonstrates how to construct a HeatmapRenderer in a SceneView for visualizing large, dense point datasets. The HeatmapRenderer visualizes points as a raster surface that is draped on the terrain and where strong colors indicate areas with a high density of points. Areas with weak or no colors indicate places that don't have many points.
Knowledge of how the heatmap is implemented is crucial for understanding how to construct the renderer. Each pixel in the view has a density
value depending on its proximity to one or more points. If a field is specified, then the density value is multiplied by the field value.
The heatmap is constructed by passing a set of colorStops to the renderer. These map a specific color to a ratio value. The ratio represents the ratio of the pixel's density value to the maxDensity of the renderer. Therefore, the lower the max
, the hotter the map will look.
Additionally, you can add a reference
to fix the radius
to a certain scale, so that it remains constant in real world size.
const heatmapRenderer = {
type: "heatmap", // autocasts as new HeatmapRenderer()
colorStops: [
{ ratio: 0 / 6, color: "rgba(25, 43, 51, 0.6)" },
{ ratio: 1 / 6, color: "rgba(30, 140, 160, 1)" },
{ ratio: 2 / 6, color: "rgba(64, 184, 156, 1)" },
{ ratio: 3 / 6, color: "rgba(73, 214, 181, 1)" },
{ ratio: 4 / 6, color: "rgba(83, 245, 207, 1)" },
{ ratio: 5 / 6, color: "rgba(102, 255, 219, 1)" },
{ ratio: 6 / 6, color: "rgba(158, 255, 233, 1)" }
],
maxDensity: 0.0045,
minDensity: 0,
radius: 35,
referenceScale: 13000
};
const layer = new FeatureLayer({
url: "https://services2.arcgis.com/jUpNdisbWqRpMo35/arcgis/rest/services/Verkehrsunf%C3%A4lle_2019/FeatureServer",
opacity: 0.75,
renderer: heatmapRenderer
});
See the HeatmapRenderer documentation for more specifics of this implementation.
Related samples and resources
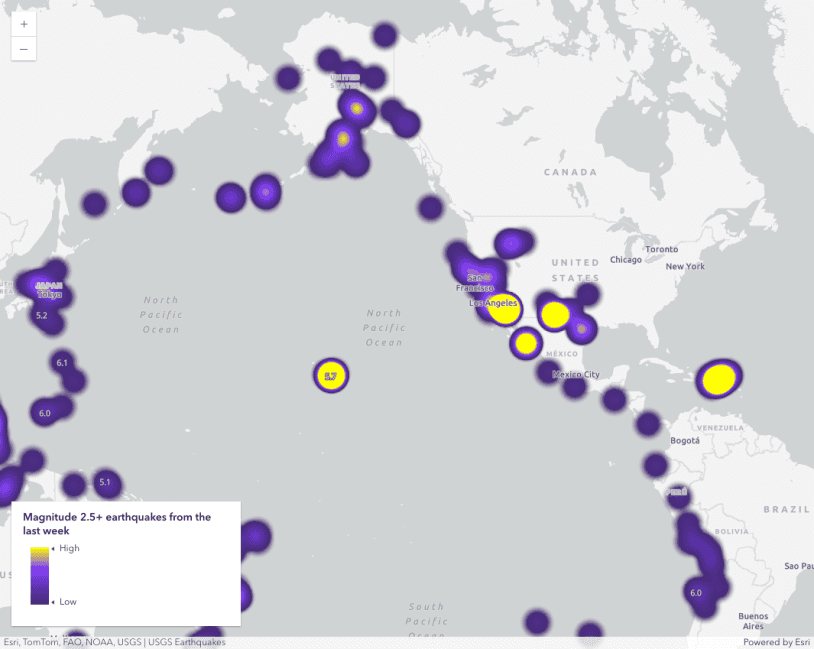
Intro to heatmap
Visualize points with a heatmap
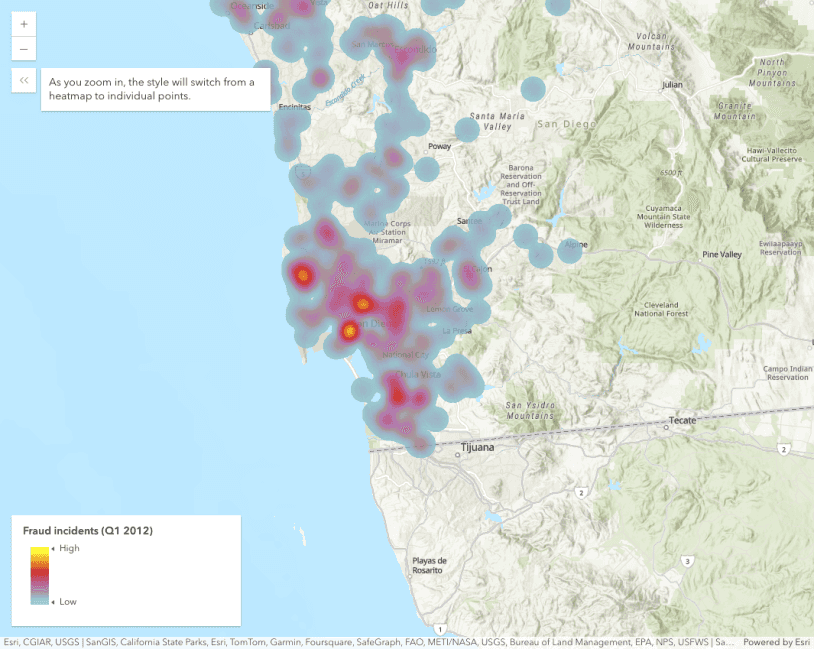
Create a scale-dependent visualization
Create a scale-dependent visualization
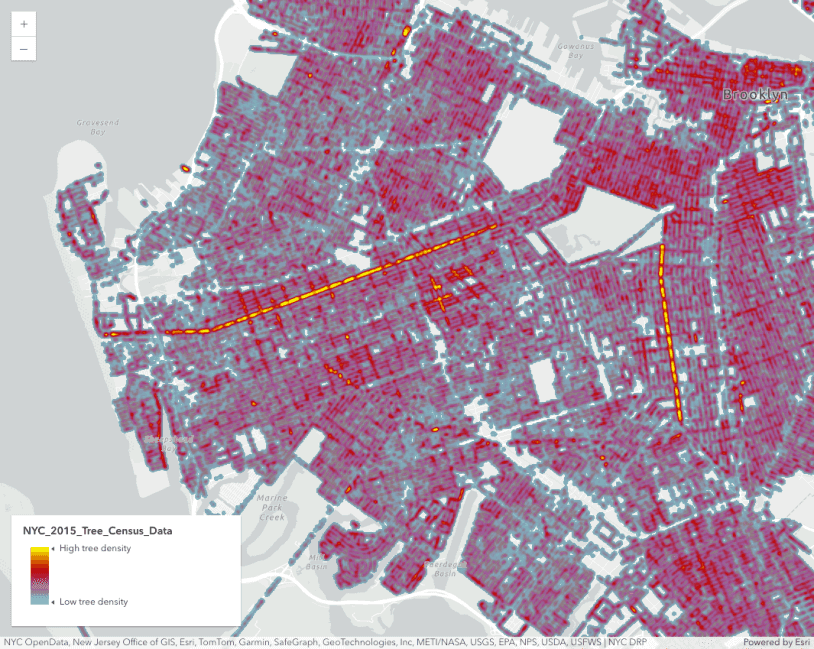
Create a static heatmap
Create a static heatmap that doesn't adjust based on scale
Heatmap
Learn how to represent point features as a continuous heatmap surface.
HeatmapRenderer
Read the API Reference for more information.