You can geocode features within a feature layer using the Esri Leaflet Geocoder plugin. You can control how general or how strict you want the search to be by specifying the search mode.
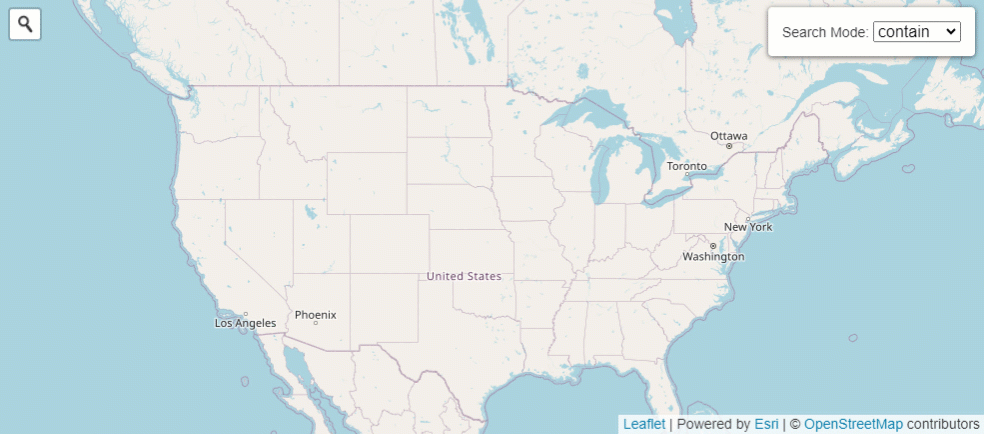
<html>
<head>
<meta charset="utf-8" />
<title>Set different search modes</title>
<meta name="viewport" content="initial-scale=1, maximum-scale=1, user-scalable=no" />
<link rel="stylesheet" href="https://unpkg.com/leaflet@1.9.4/dist/leaflet.css" crossorigin="" />
<script src="https://unpkg.com/leaflet@1.9.4/dist/leaflet.js" crossorigin=""></script>
<!-- Load Esri Leaflet from CDN -->
<script src="https://unpkg.com/esri-leaflet@3.0.12/dist/esri-leaflet.js"></script>
<!-- Load Esri Leaflet Geocoder from CDN -->
<link rel="stylesheet" href="https://unpkg.com/esri-leaflet-geocoder@3.1.4/dist/esri-leaflet-geocoder.css" crossorigin="" />
<script src="https://unpkg.com/esri-leaflet-geocoder@3.1.4/dist/esri-leaflet-geocoder.js" crossorigin=""></script>
<style>
html,
body,
#map {
padding: 0;
margin: 0;
height: 100%;
width: 100%;
font-family: Arial, Helvetica, sans-serif;
font-size: 14px;
color: #323232;
}
</style>
</head>
<body>
<style>
#selectContainer {
position: absolute;
top: 10px;
right: 10px;
z-index: 1000;
background: white;
padding: 1em;
}
#selectContainer select {
font-size: 16px;
}
</style>
<div id="map"></div>
<div id="selectContainer" class="leaflet-bar">
<label>
Search Mode:
<select id="searchMode">
<option value="contain">contain</option>
<option value="startWith">startWith</option>
<option value="endWith">endWith</option>
<option value="strict">strict</option>
</select>
</label>
</div>
<script>
const accessToken = "YOUR_ACCESS_TOKEN";
const map = L.map("map").setView([40.91, -96.63], 4);
L.tileLayer("https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png", {
attribution: '© <a href="https://osm.org/copyright">OpenStreetMap</a> contributors'
}).addTo(map);
// searchMode can be 'contain', 'startWith', 'endWith', or 'strict'
const gisDayProvider = L.esri.Geocoding.featureLayerProvider({
url:
"https://services.arcgis.com/BG6nSlhZSAWtExvp/ArcGIS/rest/services/GIS_Day_Registration_Form_2019_Hosted_View_Layer/FeatureServer/0",
searchFields: ["event_name"],
label: "GIS Day Events 2019",
formatSuggestion: function (feature) {
return feature.properties.event_name;
},
searchMode: "contain"
});
L.esri.Geocoding.geosearch({
providers: [gisDayProvider]
}).addTo(map);
const searchMode = document.getElementById("searchMode");
searchMode.addEventListener("change", function () {
gisDayProvider.options.searchMode = searchMode.value;
});
</script>
</body>
</html>