require(["esri/geometry/Mesh"], (Mesh) => { /* code goes here */ });
import Mesh from "@arcgis/core/geometry/Mesh.js";
esri/geometry/Mesh
A mesh is a general, client-side 3D geometry type composed of vertices with attributes. The vertices include geographic position, normals that affect lighting/shading and uv coordinates that can be used to map images to the mesh. Vertices are combined into 3D primitives to render the mesh in the scene (only triangle primitives are currently supported).
Mesh geometries can have an intrinsic material that determines how it is being displayed. Similar to 3D objects in scene layers, mesh geometries are symbolized with a MeshSymbol3D symbol containing a FillSymbol3DLayer.
To support multiple materials (as is often the case for complex 3D models), meshes may define components that define a material for a specific region in the mesh. In addition to supporting multiple materials, components can also reuse vertices that would otherwise be duplicated to form triangles.
Meshes are loadable since 4.27. In particular when meshes come from remote services like through SceneLayer.queryFeatures, then the contents of the mesh needs to be loaded asynchronously through the load method before vertexAttributes, extent, components, etc. are available.
Meshes represent fully georeferenced 3D geometry and care needs to be taken when importing mesh data.
It is typical for modern 3D GIS workflows that 3D geometry data be represented by 3D models, which can be stored in different file formats like GLTF, Obj, Fbx, IFC, etc. These models can come from different sources, such as 3D modeling software like SketchUp or Blender, CAD software like Revit, or even custom mesh generation code.
While some of the software may work in a georeferenced space and some of the file formats, such as IFC, may be georeferenced, much of the software and most of the model file formats are not georeferenced. This presents the challenge of expressing different georeferencing scenarios while maintaining a fast and efficient experience when displaying and editing 3D model content. In the Maps SDK we strive to strike a good balance between allowing our users to work with different scenarios while preventing situations in which good display and editing experience cannot be provided.
Mesh API
The entry point for representing 3D models in the Maps SDK is the Mesh. We refer to the API reference for details on mesh components, material and how to create meshes. This guide is concerned with georeferencing meshes.
A mesh represents a georeferenced 3D model in the Maps SDK. In order to fully georeference meshes three things are needed:
- Spatial reference
- Location of the mesh in that spatial reference. We call this the origin.
- The coordinate system in which mesh vertices are provided. We call it the vertex space.
Given these three things, meshes can be fully georeferenced. However, for certain configurations of spatial reference, mesh vertex space and viewing mode, costly projection may be required. Because that would greatly affect display and editing performance, we decided to not display or allow editing in those cases. Please see the limitations section for an overview of these restrictions.
The spatial reference of the mesh is provided with its construction. When using one of the create functions, such as createFromGLTF, the spatial reference will be taken from the provided location.
Mesh vertex spaces
The origin and spatial reference of a mesh provide georeferencing, but only for the origin. A mesh is composed of vertices, and they may exist in different coordinate systems depending on the data source. Hence, to fully georeference the mesh, it's crucial to define the coordinate system associated with the mesh vertices. The mesh vertex space property was introduced to address this need. This property specifies the meaning of the mesh vertex coordinates, ensuring that the entire mesh can be correctly georeferenced.
Mesh local vertex space
Most popular 3D model formats, such as GLTF, are not georeferenced and represent the mesh vertex coordinates in a plain local cartesian space with some length unit (e.g. meters
for GLTF). The coordinate (0,0,0) is the origin. We call this coordinate system "local" and represent it using the MeshLocalVertexSpace. The units in this vertex space are always "meters".
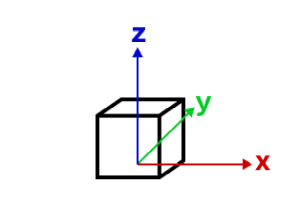
local vertex space
Georeferencing local coordinates is done by "putting them on the map" using a georeferenced cartesian reference frame. This frame is found by using the local tangent plane at the georeferenced origin of the mesh. When the spatial reference uses a geographic coordinate system (GCS) or WebMercator, the tangent plane is aligned such that the local Y tangent vector is always pointing to the north pole resulting in the east, north, up (ENU) frame.
Currently we use the tangent plane of the sphere even for ellipsoidal geographic coordinates systems. This is a simplification we use as we render on a perfect sphere in global viewing mode.
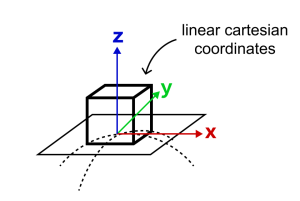
local vertex space on GCS or Web Mercator (ENU)
A local vertex space with a spatial reference which is a GCS or WebMercator is efficient to work with as the tangent plane is easy to find and we can simply move the vertices to its georeferenced location using efficient linear transformations. This comes at the cost of an approximation error between the tangent plane and the actual surface. Consequently for larger models, this error increases while it decreases as the models get smaller.
There is an important element to consider when a mesh is created with a local vertex space (e.g. by creating it from a GLTF) and displayed in local viewing mode where a projected coordinate system (PCS) is used:
The projection introduces a warping and distortion of the space in order to allow mapping of surface coordinates to a plane. This produces a mismatch between the linear coordinates of the local vertex space and the warped, non-linear coordinates of the underlying spatial reference. While steps along a line in the local vertex space are of same length, steps along a line in the underlying spatial reference are not. This means that measuring the same line in local space and the underlying spatial reference will produce different measurements. This difference further depends on the origin location of the mesh. It is important to realize that this scenario cannot be used when correct georeferencing is required.
Mesh georeferenced vertex spaces
The georeferenced vertex space indicates that mesh vertices are already projected and that their coordinates are in the spatial reference of the mesh. If an origin is provided, those vertex coordinates are interpreted as offsets relative to the origin. Otherwise, the coordinates are interpreted as absolute.
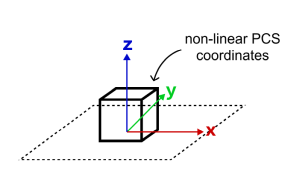
georeferenced vertex space on PCS
Display and editing are efficient for meshes with a georeferenced vertex space and when the spatial reference is a projected coordinate system and matches the spatial reference of the view. This is because in those cases, manipulations like moving a mesh can be expressed as linear transformations.
The effect of viewing modes
We will not display or allow edits for configurations where efficient display or editing is not possible due to costly reprojections being required. It is important to realize that the viewingMode defines the spatial reference in which the data is displayed and hence has a direct effect on which vertex space and spatial reference combination of meshes can be done efficiently. This means that, depending on the viewing mode, display and editing of meshes with certain combinations of vertex space and spatial reference are not supported. See limitations for an overview.
We established that for mesh spatial references which use a geographic coordinate system (or Web Mercator) and a local vertex space, display and editing can be done efficiently. That is true for "global"
viewing mode, where the view uses a geographic coordinate system (or Web Mercator) for display.
Meshes with a georeferenced vertex space and a spatial reference using a projected coordinate system (PCS) can be displayed and edited efficiently in a view using the "local"
viewing mode and when the view has the same spatial reference as the mesh.
Web Mercator
Web Mercator is a spatial reference with a projected coordinate system and the defacto standard for web mapping applications. This is due to its suitability for efficient map tiling. Even though it uses a projected coordinate system, we still support using Web Mercator in global viewing mode. This is because of its importance for web mapping and also because projecting Web Mercator on the globe cancels out the strong distortions near the poles. It is the only PCS based spatial reference we support in global viewing mode.
Web Mercator support for global viewing mode naturally extends to supporting efficient display and editing for meshes with a Web Mercator spatial reference and a local vertex space, even though it is based on a projected coordinate system. Since Web Mercator is a spatial reference based on a PCS, we also support efficient display and editing for Web Mercator with meshes using a georeferenced vertex space. Web Mercator is the only exception to the rule of only meshes with a spatial references with a geographic coordinate system can be supported in global viewing mode.
Limitations
- See Scene Layers in regards to limitations on display and editing for mesh features.
Troubleshooting mesh georeferencing
If meshes are not being displayed, then chances are that this is due a configuration which the API does not support for display and editing.
- First check the viewingMode, spatial reference and vertex space of the problematic mesh and consult the console of the javascript debugger. If the API rejects display of meshes, a warning message is printed.
- If it is determined that an unsupported configuration is used, consider switching the viewingMode in your application.
- If there is no other option, consider changing the data by switching to a supported spatial reference for the required viewingMode or by converting the mesh vertex data between local and georeferenced vertex space.
- If meshes are displayed wrongly, then this could also be due to the vertex space not being correct. If applications export geometry with georeferenced coordinates as Obj file for example, then one would need use the
"georeferenced"
vertex space.
Create simple mesh geometry primitives
The mesh geometry class has a number of convenience functions to create simple primitive shapes. These shapes can help you get started with understanding mesh geometries.
// Create a box mesh geometry
let mesh = Mesh.createBox(location, {
size: {
width: 100,
height: 50,
depth: 50
},
material: {
color: "red"
}
});
// Create a graphic and add it to the view
let graphic = new Graphic({
geometry: mesh,
symbol: {
type: "mesh-3d",
symbolLayers: [ { type: "fill" } ]
}
});
view.graphics.add(graphic);
Create mesh geometries manually
Mesh geometries can be manually created by specifying vertexAttributes and components like in the following example:
// Create a mesh geometry representing a pyramid
let pyramidMesh = new Mesh({
vertexAttributes: {
// vertex positions for the Louvre pyramid, Paris
position: [
// vertex 0 - base of the pyramid, south
2.336006, 48.860818, 0,
// vertex 1 - base of the pyramid, east
2.336172, 48.861114, 0,
// vertex 2 - base of the pyramid, north
2.335724, 48.861229, 0,
// vertex 3 - base of the pyramid, west
2.335563, 48.860922, 0,
// vertex 4 - top of the pyramid
2.335896, 48.861024, 21
]
},
// Add a single component with faces that index the vertices
// so we only need to define them once
components: [
{
faces: [
0, 4, 3,
0, 1, 4,
1, 2, 4,
2, 3, 4
]
}
],
// specify a spatial reference if the position of the vertices is not in WGS84
});
// add the mesh geometry to a graphic
let graphic = new Graphic({
geometry: pyramidMesh,
symbol: {
type: "mesh-3d",
symbolLayers: [ { type: "fill" } ]
}
});
view.graphics.add(graphic);
Note: Starting with version 4.11 autocasting is no longer supported for Mesh geometry.
- See also
Constructors
-
Parameterproperties Objectoptional
See the properties for a list of all the properties that may be passed into the constructor.
Property Overview
Name | Type | Summary | Class |
---|---|---|---|
Object | The cache is used to store values computed from geometries that need to be cleared or recomputed upon mutation. | Geometry | |
MeshComponent[] | An array of mesh components that can be used to apply materials to different regions of the same mesh. | Mesh | |
String | The name of the class. | Accessor | |
Extent | The 3D extent of the mesh geometry. | Mesh | |
Boolean | Indicates if the geometry has M values. | Geometry | |
Boolean | Indicates if the geometry has z-values (elevation). | Geometry | |
Error | The Error object returned if an error occurred while loading. | Mesh | |
String | Represents the status of a load operation. | Mesh | |
Object[] | A list of warnings which occurred while loading. | Mesh | |
SpatialReference | The spatial reference of the geometry. | Geometry | |
MeshTransform | Additional local transformation of the mesh vertices. | Mesh | |
String | The string value representing the type of geometry. | Mesh | |
Accessor | Object describing the attributes of each vertex of the mesh. | Mesh | |
MeshGeoreferencedVertexSpace|MeshLocalVertexSpace | The vertex space of the mesh. | Mesh |
Property Details
-
components
components MeshComponent[]autocast
-
An array of mesh components that can be used to apply materials to different regions of the same mesh. There are three common usage patterns for components.
- Specify a material for the whole mesh. In this case, use a single
component with only a material (leaving faces as
null
). - Reuse vertex attributes. When modeling continuous surfaces, it can be convenient to only specify vertices once and then simply refer to them. In this case, use a single component with faces set to index the vertex attributes that form triangles.
- Specify multiple materials for the same mesh. In this case, use multiple components with faces that determine to which region of the mesh the material of the component applies.
- Specify a material for the whole mesh. In this case, use a single
component with only a material (leaving faces as
-
extent
extent Extentreadonly
-
The 3D extent of the mesh geometry. The extent is computed from the vertex positions stored in the vertexAttributes. The 3D extent is computed on-demand and cached. If you modify the vertexAttributes manually, then you must call vertexAttributesChanged() to make sure the extent will be recomputed.
-
hasZ
InheritedPropertyhasZ Boolean
Inherited from Geometry -
Indicates if the geometry has z-values (elevation).
Z-values defined in a geographic or metric coordinate system are expressed in meters. However, in local scenes that use a projected coordinate system, vertical units are assumed to be the same as the horizontal units specified by the service.
-
loadError
loadError Errorreadonly
-
The Error object returned if an error occurred while loading.
- Default Value:null
-
loadStatus
loadStatus Stringreadonly
-
Represents the status of a load operation.
Value Description not-loaded The object's resources have not loaded. loading The object's resources are currently loading. loaded The object's resources have loaded without errors. failed The object's resources failed to load. See loadError for more details. Possible Values:"not-loaded"|"loading"|"failed"|"loaded"
- Default Value:not-loaded
-
A list of warnings which occurred while loading.
-
spatialReference
InheritedPropertyspatialReference SpatialReferenceautocast
Inherited from Geometry -
The spatial reference of the geometry.
- Default Value:WGS84 (wkid: 4326)
-
transform
transform MeshTransformautocast
-
Additional local transformation of the mesh vertices.
-
Object describing the attributes of each vertex of the mesh. Vertex attributes are flat numerical arrays that describe the position (mandatory), normal (used for lighting calculations and shading) and uv (used for mapping material images to the mesh surface) for each vertex.
Vertex attributes can be addressed by indices specified in the components faces property. If the mesh does not contain any components, or if a component does not specify any faces, then the vertex attributes are interpreted as if each consecutive vertex triple makes up a triangle.
- Properties
-
position Float64ArrayAutocasts from Number[]|Float32Array
A flat array of vertex positions. Vertex positions have x, y and z coordinates and they should be in the spatial reference system of the geometry.
uv Float32ArrayAutocasts from Number[]|Float64ArrayA flat array of vertex uv coordinates (2 elements per vertex).
normal Float32ArrayAutocasts from Number[]|Float64ArrayA flat array of the vertex normals (3 elements per vertex ranging from -1 to 1).
color Uint8ArrayAutocasts from Number[]|Uint8ClampedArraySince: 4.9
A flat array of the vertex colors (4 elements per vertex ranging from 0 to 255). Vertex colors are multiplied by the component material color (if any is defined).
tangent Float32ArrayAutocasts from Number[]|Float64ArraySince: 4.11
A flat array of the vertex tangents (4 elements per vertex ranging from -1 to 1. The 4th element is a sign value (-1 or +1) indicating handedness of the tangent basis). Vertex tangents are used for normal mapping, see MeshMaterial.normalTexture.
Examplelet mesh = new Mesh({ spatialReference: SpatialReference.WebMercator }); // Specify vertices for two triangles that make up a square // around a provided point. Uv coordinates are setup to cover the square // from (0, 0) to (1, 1) from corner to corner. mesh.vertexAttributes = { position: [ pt.x - 10, pt.y - 10, 100, pt.x + 10, pt.y - 10, 100, pt.x + 10, pt.y + 10, 100, pt.x - 10, pt.y - 10, 100, pt.x + 10, pt.y + 10, 100, pt.x - 10, pt.y + 10, 100 ], uv: [ 0, 0, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1 ] };
-
vertexSpace
vertexSpace MeshGeoreferencedVertexSpace|MeshLocalVertexSpaceautocast
-
The vertex space of the mesh. See mesh API for details.
Method Overview
Name | Return Type | Summary | Class |
---|---|---|---|
Adds a component to the mesh. | Mesh | ||
Adds one or more handles which are to be tied to the lifecycle of the object. | Accessor | ||
Cancels a load() operation if it is already in progress. | Mesh | ||
Mesh | Centers the mesh at the specified location without changing its scale. | Mesh | |
Mesh | Creates a deep clone of the Mesh object. | Mesh | |
Mesh | Creates a mesh representing a box. | Mesh | |
Mesh | Creates a mesh representing a cylinder. | Mesh | |
Promise<Mesh> | Creates a mesh geometry from a file or list of files and which is ready to be displayed in the view. | Mesh | |
Promise<Mesh> | Creates a new mesh geometry from a glTF model referenced by the | Mesh | |
Mesh | Creates a new mesh geometry from a polygon geometry. | Mesh | |
Mesh | Creates a mesh representing a plane. | Mesh | |
Mesh | Creates a mesh representing a sphere. | Mesh | |
* | Creates a new instance of this class and initializes it with values from a JSON object generated from an ArcGIS product. | Geometry | |
Boolean | Returns true if a named group of handles exist. | Accessor | |
Boolean |
| Mesh | |
Boolean |
| Mesh | |
Boolean |
| Mesh | |
Promise | Loads the resources referenced by this class. | Mesh | |
Mesh | Offsets the mesh geometry by the specified distance in x, y, and z. | Mesh | |
Removes a component from the mesh. | Mesh | ||
Removes a group of handles owned by the object. | Accessor | ||
Mesh | Rotates the mesh geometry around its x, y and z axis (in that order). | Mesh | |
Mesh | Scales the mesh geometry by the specified factor. | Mesh | |
Object | Converts an instance of this class to its ArcGIS portal JSON representation. | Geometry | |
Notifies that any cached values that depend on vertex attributes need to be recalculated. | Mesh | ||
Promise |
| Mesh |
Method Details
-
Adds a component to the mesh.
ParameterAutocasts from ObjectThe component to add.
-
Inherited from Accessor
Since: ArcGIS Maps SDK for JavaScript 4.25Accessor since 4.0, addHandles added at 4.25. -
Adds one or more handles which are to be tied to the lifecycle of the object. The handles will be removed when the object is destroyed.
// Manually manage handles const handle = reactiveUtils.when( () => !view.updating, () => { wkidSelect.disabled = false; }, { once: true } ); this.addHandles(handle); // Destroy the object this.destroy();
ParametershandleOrHandles WatchHandle|WatchHandle[]Handles marked for removal once the object is destroyed.
groupKey *optionalKey identifying the group to which the handles should be added. All the handles in the group can later be removed with Accessor.removeHandles(). If no key is provided the handles are added to a default group.
-
Cancels a load() operation if it is already in progress.
-
centerAt
centerAt(location, params){Mesh}
-
Centers the mesh at the specified location without changing its scale. This effectively adjust the mesh origin and mesh vertices such that vertices are given relative to the new location and the new location is the new origin. The effective georeferenced vertex positions will not change.
The mesh will be modified in place. To modify a copy of the mesh instead, use clone() before calling centerAt().
ParametersSpecificationlocation PointThe location at which to center the mesh.
params ObjectoptionalAdditional parameters.
Specificationgeographic BooleanoptionalWhether to georeference relative to the globe or the projected coordinate system (PCS). This parameter is only relevant for spatial references that can be used in both local and global viewing modes (currently only WebMercator), and otherwise ignored. The default value is true. When true, the translation applied to center the mesh is done in a Cartesian system with respect to the local coordinate system on the globe and is specified in meters.
origin PointoptionalThe origin at which to center. If not specified, the mesh will be centered at the mesh extent center.
ReturnsType Description Mesh The modified mesh.
-
createBox
createBox(location, params){Mesh}static
-
Creates a mesh representing a box. The spatial reference of the resulting mesh is the same as the location where it is placed.
Box UV coordinate space
The box geometry will have UV coordinates generated according to the following scheme:
Parameterslocation PointThe location bottom center of the box.
params CreateBoxParametersoptionalParameters to configure the box creation.
ReturnsType Description Mesh The resulting mesh. Exampleslet mesh = Mesh.createBox(point, { size: { width: 10, height: 100, depth: 20 }, material: { color: "green" } });
let mesh = Mesh.createBox(point, { imageFace: "top", material: { colorTexture: new MeshTexture({ url: "./url-to-image.png" }) } });
-
createCylinder
createCylinder(location, params){Mesh}static
-
Creates a mesh representing a cylinder. The spatial reference of the resulting mesh is the same as the location where it is placed.
Cylinder UV coordinate space
The cylinder geometry will have UV coordinates generated according to the following scheme (example is shown for 8 vertices cylinder):
Parameterslocation PointThe location of the bottom center of the cylinder.
params CreateCylinderParametersoptionalParameters to configure the cylinder creation.
ReturnsType Description Mesh The resulting mesh.
-
Since: ArcGIS Maps SDK for JavaScript 4.27Mesh since 4.7, createFromFiles added at 4.27. Deprecated Use convertMesh instead. -
Creates a mesh geometry from a file or list of files and which is ready to be displayed in the view. This utility may use a scene layer to perform the necessary file conversions to obtain the resulting Mesh.
ParametersSpecificationlocation PointThe location of the origin of the model. If the location doesn't contain a z-value, z is assumed to be
0
.The files from which to create the mesh.
params ObjectoptionalAdditional parameters.
Specificationlayer SceneLayeroptionalThe layer to which the files are to be uploaded and which will perform any necessary conversions.
signal AbortSignaloptionalAn AbortSignal to abort the loading process. If canceled, the promise will be rejected with an error named
AbortError
. See also AbortController.ReturnsType Description Promise<Mesh> A promise that resolves to a mesh geometry representing the specified files.
-
Since: ArcGIS Maps SDK for JavaScript 4.11Mesh since 4.7, createFromGLTF added at 4.11. -
Creates a new mesh geometry from a glTF model referenced by the
url
parameter. The spatial reference of the resulting mesh is the same as thelocation
parameter. For more information on the supported glTF features you can read the Visualizing points with 3D symbols guide topic. Animations are currently not supported.Parameterslocation PointThe location of the origin of the model. If the location doesn't contain a z-value, z is assumed to be
0
.url StringThe URL of the glTF model. The URL should point to a glTF file (.gltf or .glb) which can reference additional binary (.bin) and image files (.jpg, .png).
params CreateFromGLTFParametersoptionalParameters to configure the mesh from gltf creation.
ReturnsType Description Promise<Mesh> A promise that resolves to a mesh geometry representing the loaded glTF model.
-
createFromPolygon
createFromPolygon(polygon, params){Mesh}static
-
Creates a new mesh geometry from a polygon geometry. The resulting mesh contains only a position vertex attribute and a single component with faces. The default shading will be set to
flat
. The spatial reference of the resulting mesh is the same as the input polygon. The resulting mesh will not contain any uv nor normal vertex attributes.Parameterspolygon PolygonThe input polygon.
params ObjectoptionalOptional parameters.
Specificationoptional Autocasts from ObjectThe material to be used for the mesh.
ReturnsType Description Mesh A new mesh representing the triangulated polygon.
-
createPlane
createPlane(location, params){Mesh}static
-
Creates a mesh representing a plane. The spatial reference of the resulting mesh is the same as the location where it is placed. A plane consists of two triangles and may be conveniently oriented at creation time.
Plane UV coordinate space
The plane geometry will have UV coordinates generated according to the following scheme:
Parameterslocation PointThe location of the bottom center of the plane.
params CreatePlaneParametersoptionalParameters to configure the plane creation.
ReturnsType Description Mesh The resulting mesh.
-
createSphere
createSphere(location, params){Mesh}static
-
Creates a mesh representing a sphere. The spatial reference of the resulting mesh is the same as the location where it is placed.
Sphere UV coordinate space
The sphere geometry will have UV coordinates generated according to the following scheme (example is shown for 8x8 vertices sphere):
Parameterslocation PointThe location of the bottom center of the sphere.
params CreateSphereParametersoptionalParameters to configure the sphere creation.
ReturnsType Description Mesh The resulting mesh.
-
Creates a new instance of this class and initializes it with values from a JSON object generated from an ArcGIS product. The object passed into the input
json
parameter often comes from a response to a query operation in the REST API or a toJSON() method from another ArcGIS product. See the Using fromJSON() topic in the Guide for details and examples of when and how to use this function.Parameterjson ObjectA JSON representation of the instance in the ArcGIS format. See the ArcGIS REST API documentation for examples of the structure of various input JSON objects.
ReturnsType Description * Returns a new instance of this class.
-
hasHandles
InheritedMethodhasHandles(groupKey){Boolean}
Inherited from AccessorSince: ArcGIS Maps SDK for JavaScript 4.25Accessor since 4.0, hasHandles added at 4.25. -
Returns true if a named group of handles exist.
ParametergroupKey *optionalA group key.
ReturnsType Description Boolean Returns true
if a named group of handles exist.Example// Remove a named group of handles if they exist. if (obj.hasHandles("watch-view-updates")) { obj.removeHandles("watch-view-updates"); }
-
isFulfilled
isFulfilled(){Boolean}
-
isFulfilled()
may be used to verify if creating an instance of the class is fulfilled (either resolved or rejected). If it is fulfilled,true
will be returned.ReturnsType Description Boolean Indicates whether creating an instance of the class has been fulfilled (either resolved or rejected).
-
load
load(signal){Promise}
-
Loads the resources referenced by this class. This method automatically executes for a View and all of the resources it references in Map if the view is constructed with a map instance.
This method must be called by the developer when accessing a resource that will not be loaded in a View.
The
load()
method only triggers the loading of the resource the first time it is called. The subsequent calls return the same promise.It's possible to provide a
signal
to stop being interested into aLoadable
instance load status. When the signal is aborted, the instance does not stop its loading process, only cancelLoad can abort it.Parametersignal AbortSignaloptionalSignal object that can be used to abort the asynchronous task. The returned promise will be rejected with an Error named
AbortError
when an abort is signaled. See also AbortController for more information on how to construct a controller that can be used to deliver abort signals.Returns
-
offset
offset(dx, dy, dz){Mesh}
-
Offsets the mesh geometry by the specified distance in x, y, and z. The units of x, y, and z are the units of the spatial reference. When the vertex space is local or georeferenced with an origin, the offset is applied to the origin of the vertex space. When the vertex space is georeferenced without origin, the offset is applied to the vertex positions directly.
The mesh will be modified in place. To modify a copy of the mesh instead, use clone() before calling offset().
Parametersdx NumberThe amount to offset the geometry in the x direction.
dy NumberThe amount to offset the geometry in the y direction.
dz NumberThe amount to offset the geometry in the z direction.
ReturnsType Description Mesh The modified mesh (this instance).
-
Removes a component from the mesh.
Parametercomponent MeshComponentThe component to remove.
-
Inherited from Accessor
Since: ArcGIS Maps SDK for JavaScript 4.25Accessor since 4.0, removeHandles added at 4.25. -
Removes a group of handles owned by the object.
ParametergroupKey *optionalA group key or an array or collection of group keys to remove.
Exampleobj.removeHandles(); // removes handles from default group obj.removeHandles("handle-group"); obj.removeHandles("other-handle-group");
-
rotate
rotate(angleX, angleY, angleZ, params){Mesh}
-
Rotates the mesh geometry around its x, y and z axis (in that order). For each rotation angle, the rotation direction is clockwise when looking in the direction of the respective axis. The mesh will be modified in place. To modify a copy of the mesh instead, use clone() before calling rotate().
ParametersSpecificationangleX NumberThe angle by which to rotate around the x-axis (in degrees).
angleY NumberThe angle by which to rotate around the y-axis (in degrees).
angleZ NumberThe angle by which to rotate around the z-axis (in degrees).
params ObjectoptionalAdditional parameters.
Specificationgeographic BooleanoptionalWhether to georeference relative to the globe or the projected coordinate system (PCS). This parameter is only relevant for spatial references that can be used in both local and global viewing modes (currently only WebMercator), and otherwise ignored. The default value is true. When true, the rotation is applied in a Cartesian system with respect to the local coordinate system on the globe and is specified in meters.
origin PointoptionalThe origin around which to rotate. If not specified, the mesh will be rotated around the mesh extent center.
ReturnsType Description Mesh The modified mesh (this instance). Example// rotate the mesh in the horizontal plane (around the z axis) by 90 degrees and tilt it in the lateral // vertical plane (around the y axis) by 20 degrees. mesh.rotate(0, 20, 90);
-
scale
scale(factor, params){Mesh}
-
Scales the mesh geometry by the specified factor. The mesh will be modified in place. To modify a copy of the mesh instead, use clone() before calling scale().
ParametersSpecificationfactor NumberThe amount to scale the geometry.
params ObjectoptionalAdditional parameters.
Specificationgeographic BooleanoptionalWhether to georeference relative to the globe or the projected coordinate system (PCS). This parameter is only relevant for spatial references that can be used in both local and global viewing modes (currently only WebMercator), and otherwise ignored. The default value is true. When true, the scale is applied in a Cartesian system with respect to the local coordinate system on the globe.
origin PointoptionalThe origin point for scaling. If not specified, the mesh will be scaled around the mesh extent center.
ReturnsType Description Mesh The modified mesh (this instance).
-
toJSON
InheritedMethodtoJSON(){Object}
Inherited from Geometry -
Converts an instance of this class to its ArcGIS portal JSON representation. See the Using fromJSON() guide topic for more information.
ReturnsType Description Object The ArcGIS portal JSON representation of an instance of this class.
-
Notifies that any cached values that depend on vertex attributes need to be recalculated. Use this method after modifying the vertex attributes in place so that values that depend on them (such as the calculation of the extent) are recalculated accordingly.
-
when
when(callback, errback){Promise}
-
when()
may be leveraged once an instance of the class is created. This method takes two input parameters: acallback
function and anerrback
function. Thecallback
executes when the instance of the class loads. Theerrback
executes if the instance of the class fails to load.Parameterscallback FunctionoptionalThe function to call when the promise resolves.
errback FunctionoptionalThe function to execute when the promise fails.
ReturnsType Description Promise Returns a new promise for the result of callback
that may be used to chain additional functions.Example// Although this example uses MapView, any class instance that is a promise may use when() in the same way let view = new MapView(); view.when(function(){ // This function will execute once the promise is resolved }, function(error){ // This function will execute if the promise is rejected due to an error });
Type Definitions
-
CreateBoxParameters
CreateBoxParameters Object
-
Options used to configure box mesh creation.
- Properties
-
optional A uniform size value or an object containing individual values width, height and depth. The unit of the size values is derived from the spatial reference of the provided location, unless a unit is specified.
geographic BooleanDEPRECATED: Use vertexSpace option instead - Whether to georeference relative to the globe or the projected coordinate system (PCS). This parameter is only relevant for spatial references that can be used in both local and global viewing modes (currently only WebMercator), and otherwise ignored. The default value is true. When true, the mesh is created in a Cartesian system with respect to the local coordinate system on the globe and sizes are specified in meters.
unit StringThe unit of the size (defaults to the unit of the location's spatial reference).
Possible Values:"millimeters"|"centimeters"|"decimeters"|"meters"|"kilometers"|"inches"|"feet"|"yards"|"miles"|"nautical-miles"|"us-feet"
vertexSpace StringSpecifies the vertex space of the created mesh. If not specified, a vertex space is chosen according to the spatial reference where a MeshLocalVertexSpace is used for geographic or WebMercator spatial references and MeshGeoreferencedVertexSpace is used otherwise. If vertexSpace is "georeferenced", then the vertex space will be also MeshGeoreferencedVertexSpace where mesh vertices are georeferenced offsets to the location provided with the create function. In case of vertex space being "local", mesh vertices will be defined in a local cartesian coordinate frame placed at the location provided with the create function. This will be represented by MeshLocalVertexSpace
Possible Values:"local"|"georeferenced"
material MeshMaterialThe material to be used for the mesh.
imageFace StringThe face for generating image uv coordinates. By default, a single set of unwrapped UV coordinates are generated for all the faces. By setting the
imageFace
parameter to one ofeast
,west
,north
,south
,up
ordown
, the specified face will have full sized UV coordinates while the other faces will pertain their regular unwrapped UV coordinates. This is useful for applying an image only to a single face of the box. The providedmaterial
parameter will be applied to the specifiedimageFace
. The resulting mesh will have two components, the first contains the selected image face and the second contains the other faces of the box.
-
CreateCylinderParameters
CreateCylinderParameters Object
-
Options used to configure cylinder mesh creation.
- Properties
-
optional A uniform size value or an object containing individual values width, height and depth. The unit of the size values is derived from the spatial reference of the provided location, unless a specific unit is specified.
width NumberThe width of the created mesh.
depth NumberThe depth of the created mesh.
height NumberThe height of the created mesh.
geographic BooleanDEPRECATED: Use vertexSpace option instead - Whether to georeference relative to the globe or the projected coordinate system (PCS). This parameter is only relevant for spatial references that can be used in both local and global viewing modes (currently only WebMercator), and otherwise ignored. The default value is true. When true, the mesh is created in a Cartesian system with respect to the local coordinate system on the globe and sizes are specified in meters.
params.unit StringThe unit of the size (defaults to the unit of the location's spatial reference).
Possible Values:"millimeters"|"centimeters"|"decimeters"|"meters"|"kilometers"|"inches"|"feet"|"yards"|"miles"|"nautical-miles"|"us-feet"
densificationFactor NumberThe additional number of subdivisions for generating the mesh representing a sphere. A densificationFactor parameter of 0 will generate a default of 16-by-16 vertices to approximate the sphere. A densificationFactor of 1 will generate 32-by-32 vertices, etc. The larger the densificationFactor, the better the mesh will approximate a perfect sphere (at the cost of processing and rendering performance).
vertexSpace StringSpecifies the vertex space of the created mesh. If not specified, a vertex space is chosen according to the spatial reference where a MeshLocalVertexSpace is used for geographic or WebMercator spatial references and MeshGeoreferencedVertexSpace is used otherwise. If vertexSpace is "georeferenced", then the vertex space will be also MeshGeoreferencedVertexSpace where mesh vertices are georeferenced offsets to the location provided with the create function. In case of vertex space being "local", mesh vertices will be defined in a local cartesian coordinate frame placed at the location provided with the create function. This will be represented by MeshLocalVertexSpace
Possible Values:"local"|"georeferenced"
material MeshMaterialThe material to be used for the mesh.
-
CreateFromGLTFParameters
CreateFromGLTFParameters Object
-
Options used to configure gltf mesh creation.
- Properties
-
geographic Boolean
DEPRECATED: Use vertexSpace option instead - Whether to georeference relative to the globe or the projected coordinate system (PCS). This parameter is only relevant for spatial references that can be used in both local and global viewing modes (currently only WebMercator), and otherwise ignored. The default value is true. When true, the mesh is created in a Cartesian system with respect to the local coordinate system on the globe and sizes are specified in meters.
signal AbortSignalAn AbortSignal to abort the loading process. If canceled, the promise will be rejected with an error named
AbortError
. See also AbortController.vertexSpace StringSpecifies the vertex space of the created mesh. If not specified, a vertex space is chosen according to the spatial reference where a MeshLocalVertexSpace is used for geographic or WebMercator spatial references and MeshGeoreferencedVertexSpace is used otherwise. If vertexSpace is "georeferenced", then the vertex space will be also MeshGeoreferencedVertexSpace where mesh vertices are georeferenced offsets to the location provided with the create function. In case of vertex space being "local", mesh vertices will be defined in a local cartesian coordinate frame placed at the location provided with the create function. This will be represented by MeshLocalVertexSpace
Possible Values:"local"|"georeferenced"
material MeshMaterialThe material to be used for the mesh.
-
CreatePlaneParameters
CreatePlaneParameters Object
-
Options used to configure plane mesh creation.
- Properties
-
optional A uniform size value or an object containing individual values width, height and depth. The unit of the size values is derived from the spatial reference of the provided location, unless a specific unit is specified.
width NumberThe width of the created mesh.
depth NumberThe depth of the created mesh.
height NumberThe height of the created mesh.
params.facing StringDefault Value:"up"Direction the plane is facing.
Possible Values:"east"|"west"|"north"|"south"|"up"|"down"
geographic BooleanDEPRECATED: Use vertexSpace option instead - Whether to georeference relative to the globe or the projected coordinate system (PCS). This parameter is only relevant for spatial references that can be used in both local and global viewing modes (currently only WebMercator), and otherwise ignored. The default value is true. When true, the mesh is created in a Cartesian system with respect to the local coordinate system on the globe and sizes are specified in meters.
params.unit StringThe unit of the size (defaults to the unit of the location's spatial reference).
Possible Values:"millimeters"|"centimeters"|"decimeters"|"meters"|"kilometers"|"inches"|"feet"|"yards"|"miles"|"nautical-miles"|"us-feet"
densificationFactor NumberThe additional number of subdivisions for generating the mesh representing a sphere. A densificationFactor parameter of 0 will generate a default of 16-by-16 vertices to approximate the sphere. A densificationFactor of 1 will generate 32-by-32 vertices, etc. The larger the densificationFactor, the better the mesh will approximate a perfect sphere (at the cost of processing and rendering performance).
vertexSpace StringSpecifies the vertex space of the created mesh. If not specified, a vertex space is chosen according to the spatial reference where a MeshLocalVertexSpace is used for geographic or WebMercator spatial references and MeshGeoreferencedVertexSpace is used otherwise. If vertexSpace is "georeferenced", then the vertex space will be also MeshGeoreferencedVertexSpace where mesh vertices are georeferenced offsets to the location provided with the create function. In case of vertex space being "local", mesh vertices will be defined in a local cartesian coordinate frame placed at the location provided with the create function. This will be represented by MeshLocalVertexSpace
Possible Values:"local"|"georeferenced"
material MeshMaterialThe material to be used for the mesh.
-
CreateSphereParameters
CreateSphereParameters Object
-
Options used to configure sphere mesh creation.
- Properties
-
optional A uniform size value or an object containing individual values width, height and depth. The unit of the size values is derived from the spatial reference of the provided location, unless a unit is specified.
width NumberThe width of the created mesh.
depth NumberThe depth of the created mesh.
height NumberThe height of the created mesh.
geographic BooleanDEPRECATED: Use vertexSpace option instead - Whether to georeference relative to the globe or the projected coordinate system (PCS). This parameter is only relevant for spatial references that can be used in both local and global viewing modes (currently only WebMercator), and otherwise ignored. The default value is true. When true, the mesh is created in a Cartesian system with respect to the local coordinate system on the globe and sizes are specified in meters.
params.unit StringThe unit of the size (defaults to the unit of the location's spatial reference).
Possible Values:"millimeters"|"centimeters"|"decimeters"|"meters"|"kilometers"|"inches"|"feet"|"yards"|"miles"|"nautical-miles"|"us-feet"
densificationFactor NumberThe additional number of subdivisions for generating the mesh representing a sphere. A densificationFactor parameter of 0 will generate a default of 16-by-16 vertices to approximate the sphere. A densificationFactor of 1 will generate 32-by-32 vertices, etc. The larger the densificationFactor, the better the mesh will approximate a perfect sphere (at the cost of processing and rendering performance).
vertexSpace StringSpecifies the vertex space of the created mesh. If not specified, a vertex space is chosen according to the spatial reference where a MeshLocalVertexSpace is used for geographic or WebMercator spatial references and MeshGeoreferencedVertexSpace is used otherwise. If vertexSpace is "georeferenced", then the vertex space will be also MeshGeoreferencedVertexSpace where mesh vertices are georeferenced offsets to the location provided with the create function. In case of vertex space being "local", mesh vertices will be defined in a local cartesian coordinate frame placed at the location provided with the create function. This will be represented by MeshLocalVertexSpace
Possible Values:"local"|"georeferenced"
material MeshMaterialThe material to be used for the mesh.