Note: Sign in to access the data in this sample. username:
password:
Getting Started
A knowledge graph allows you work with a graph network.
This network connects people, places, and things
(represented by entities) with each other through
relationships that define how they are associated.
Both entities and relationships can have associated properties.
An entity with a spatial location can be connected with other entities that do not have a spatial location.
There are three different types of edits that can be applied to a knowledge graph:
- Add new entities and relationships to the graph.
- Update the properties of entities and relationships that already exist in the graph.
- Delete entities and relationships from the graph.
This example shows each edit to the knowledge graph independently, however, multiple edits can be executed in a single execute
call.
The sample dataset contains observations of bumble bees made at locations around the United States. Each observation was made and verified by users and is of a specific species of bumble bee.
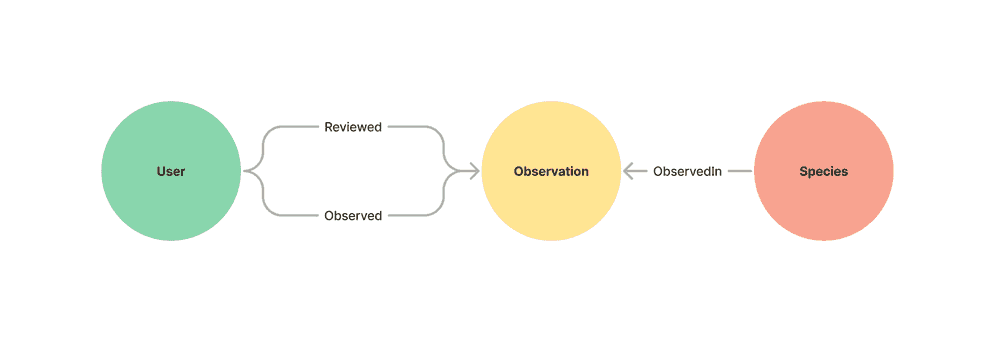
Good search terms include states abbreviations (e.g. CA, WA), countries, parks, and bumble bee descriptors (e.g. fuzzy, yellow, spotted).
For additional information on working with knowledge graph services see:
- Introduction to Knowledge Graph in the JavaScript Maps SDK.
- Search a knowledge graph
- Query a knowledge graph
- Working with knowledge graph layers
- Get started with ArcGIS Knowledge Server for overview of ArcGIS Knowledge for ArcGIS Enterprise.
- Hosted Knowledge Graph Service for information on managing knowledge graph services via ArcGIS Enterprise and the REST API.
- Get started with ArcGIS Knowledge (ArcGIS Pro) for information on ArcGIS Knowledge in ArcGIS Pro.
How to use this sample
1. Sign in
The data in this example is secured, as most knowledge graph data will be since the ArcGIS Knowledge Graph Server license is required. Therefore, the first step is to sign in to load the data.
2. Add records
Start by adding a new User
to the graph by providing the name (e.g. "Jane Doe").
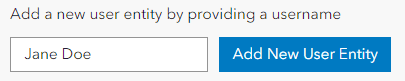
Then add a new Project
entity to the graph by providing a project name (e.g. "Backyard bees").

Finally, add a new Member
relationship between a user and a project.

3. Update records
Update the name
property of an existing User
.

4. Delete records
Delete a User
from the graph. This also deletes any associated relationships.
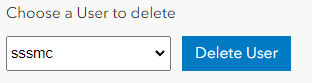
View edit 'results'
The results returned from the execute
detail the type of the records modified and the ids of the records that were
added, updated or deleted. It also includes any errors encountered in executing the edits.
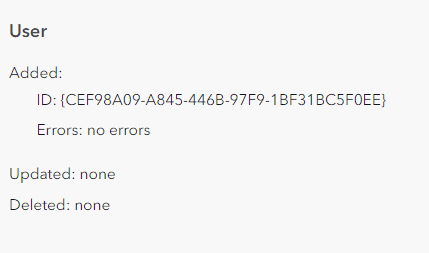
How it works
Add
To specify new records to add to the graph, first define any new entities
and relationships and
their properties.
These properties must match the properties of the entity type
or relationship type that the record is being added to.
This example adds to the User
and Project
entity types, both of which require the name
property.
Entities
/*
* Add an entity by providing the named type and the properties. If there are required properties for
* the entity type, the add operation will fail if they are not provided.
*/
const createEntity = (type, name) => {
KnowledgeGraph.fetchKnowledgeGraph(url).then((kg) => {
//create new entity
const entityAdd = new Entity({
typeName: type,
properties: {
name: name
}
});
//add entity to the graph
KnowledgeGraph.executeApplyEdits(kg, {
entityAdds: [entityAdd]
}).then((editResult) => {
formatResults(editResult)
updateLists();
getEntityOptions();
});
});
}
Relationships
When adding a relationship, the origin and destination entities must already exist in the graph. This example adds
a Member
relationship between a User
and Project
. This relationship has no additional properties.
/*
* Add a relationship. In order to add a relationship, both the origin and destination entity must
* already exist in the graph. To add a relationship, provide the named type, origin entity unique identifier,
* destination entity unique identifier and any properties.
*/
document.getElementById("add-memberof").addEventListener("click", () => {
KnowledgeGraph.fetchKnowledgeGraph(url).then((kg) => {
//getting the unique identifiers of the origin and destination entities based on the `name` property
const queryString = `MATCH (u:User {name: '${document.getElementById("user-select").value}'}),
(p:Project {name: '${document.getElementById("project-select").value}'}) RETURN u.globalid, p.globalid`
KnowledgeGraph.executeQuery(kg, { openCypherQuery: queryString }).then((result) => {
//creating the new relationship
const relationshipAdd = new Relationship({
typeName: "MemberOf",
originId: result['resultRows'][0][0],
destinationId: result['resultRows'][0][1],
properties: {}
});
//adding the relationship to the graph
KnowledgeGraph.executeApplyEdits(kg, {
relationshipAdds: [relationshipAdd]
}).then((editResult) => {
formatResults(editResult)
updateLists();
getEntityOptions();
});
})
});
});
Update record
To define updates to existing records, specify entities or relationships that are already in the graph. Property values can be
changed or added provided that the property exists for the named type. In this example, the name
property can be changed for any User
entity.
Properties themselves cannot be added or deleted since they are associated
with the entity type or relationship type. For information on adding or removing properties from a named type see ArcGIS REST API: Knowledge Graph Service - Edit (Data Model)
/*
* Update an entity. The properties of any entity or relationship record can be updated. In this example the
* `name` property on a `User` entity is updated.
*/
KnowledgeGraph.fetchKnowledgeGraph(url).then((kg) => {
//querying the graph to get the unique identifier of the user based on the `name` property
const queryString = `MATCH (u:User {name: '${document.getElementById("all-select").value}'}) RETURN u.globalid`;
KnowledgeGraph.executeQuery(kg, { openCypherQuery: queryString }).then((result) => {
//create entity to update. The id must be the unique identifier of an existing entity.
const entityUpdate = new Entity({
typeName: "User",
id: result['resultRows'][0][0],
properties: { "name": `${document.getElementById("update-input").value}` }
});
//apply the update
KnowledgeGraph.executeApplyEdits(kg, {
entityUpdates: [entityUpdate]
}).then((editResult) => {
formatResults(editResult)
updateLists();
getEntityOptions();
});
})
});
Delete record
Specify records to delete by providing the entity type or relationship type and a list of unique identifiers for records of that type to be deleted.
Note that by default, when deleting an entity you must also specify all connected relationships for deletion as well.
If you want these relationships to be deleted automatically, set cascade
to true
. If cascade
is false and the entity has connected relationships that are not in the edit, execute
will fail.
/*
* Delete an entity. Entity or relationship records can be deleted.
* When deleting entities, if the entity is the origin or destination of any relationships,
* the delete operation will fail unless `cascadeDelete` is set to true. When `cascadeDelete` is true
* the entity and all connected relationships will be deleted.
*/
KnowledgeGraph.fetchKnowledgeGraph(url).then((kg) => {
//querying the graph to get the unique identifier of the project based on the `name` property
const queryString = `MATCH (u:User {name: '${document.getElementById("all-select-delete").value}'}) RETURN u.globalid`
KnowledgeGraph.executeQuery(kg, { openCypherQuery: queryString }).then((result) => {
//delete the entity from the graph
KnowledgeGraph.executeApplyEdits(kg, {
entityDeletes: [{
typeName: "User",
ids: [result['resultRows'][0][0]]
}],
options: {
cascadeDelete: true
}
}).then((editResult) => {
formatResults(editResult)
updateLists();
getEntityOptions();
});
});
});