Note: Sign in to access the data in this sample. username:
password:
Getting Started
A knowledge graph allows you work with a graph network.
This network connects people, places, and things
(represented by entities) with each other through
relationships that define how they are associated.
Both entities and relationships can have associated properties.
An entity with a spatial location can be connected with other entities that do not have a spatial location.
A KnowledgeGraphLayer is a composite layer that represents a knowledge graph service on a map. The layer contains sublayers for each entity type and relationship type contained in the KnowledgeGraphLayer.
The sample dataset contains observations of bumble bees made at locations around the United States. Each observation was made and verified by users and is of a specific species of bumble bee.
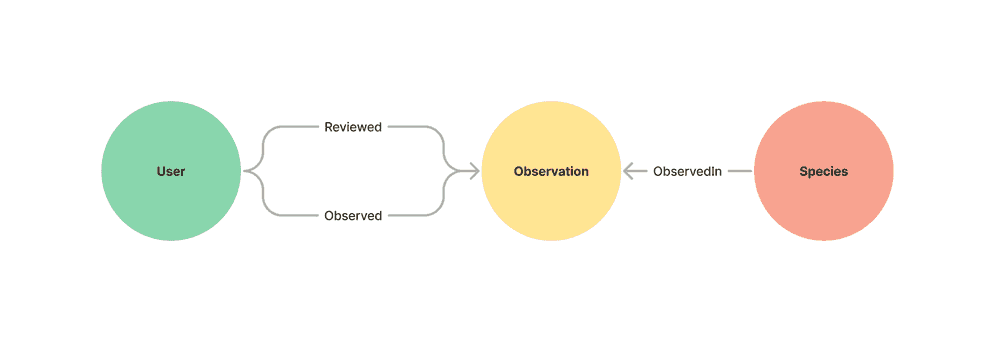
Known Limitations
A KnowledgeGraphLayer can be added to an instance of a map but it cannot be saved as an ArcGIS Enterprise portal item or added to an a web map in ArcGIS Map Viewer. KnowledgeGraphLayer can only be used with KnowledgeGraphServices on ArcGIS Enterprise 11.1 or later.
For additional information on working with knowledge graph services see:
- Introduction to Knowledge Graph in the Javascript SDK.
- Search a knowledge graph
- Query a knowledge graph
- Edit knowledge graph data
- Get started with ArcGIS Knowledge Server for overview of ArcGIS Knowledge for ArcGIS Enterprise.
- Hosted Knowledge Graph Service for information on managing knowledge graph services via ArcGIS Enterprise and the REST API.
- Get started with ArcGIS Knowledge (ArcGIS Pro) for information on ArcGIS Knowledge in ArcGIS Pro.
How it works
To create a KnowledgeGraphLayer from a knowledge graph service, set the url property to the REST endpoint of the service.
A KnowledgeGraphLayer also optionally takes an inclusion definition.
The inclusion definition specifies a set of named types and/or specific entities and relationships to be included in the layer.
This sample illustrates how to use an inclusion definition to only include the research grade Observations
in the layer.
These specific observations are determined by first querying the knowledge graph to get just the observations who's property quality_
is "research".
See KnowledgeGraphLayer.inclusionModeDefinition for more on inclusion lists.
The inclusionModeDefinition is optional. If excluded, all named types in the array will be included as sublayers. All spatial entity types will be drawn on the map.
Any non spatial entity types and all relationship types will be included as table sublayers and will not be drawn on the map.
//create a map of the ids of those research grade observations to include in the layer.
//Since we only returned one variable we know that the first item in the array will be the observation entity
let members = new Map();
for (observation of observationList.resultRows) {
members.set(observation[0].id, { id: observation[0].id })
}
//Define an inclusion list that limits the layer to only one sublayer for
//only the research grade observation returned from the query.
let namedTypes = new Map();
//only one named type, and only the subset of instance of that type.
namedTypes.set("Observation", { useAllData: false, members: members });
//only create a sublayer for the specified named types.
const inclusionDef = {
generateAllSublayers: false,
namedTypeDefinitions: namedTypes
}
//Create the layer
//The inclusionModeDefinition is optional. If excluded, all named types in the array will be included as sublayers.
//Any non spatial entity types and all relationship types will be included as table sublayers and will not be drawn on the map.
const compositeLayer = new KnowledgeGraphLayer({
url: url,
inclusionModeDefinition: inclusionDef
});
Define the renderer and labels for each named type. In this example the inclusion list contains only one named type so only one renderer and label definition is need.
//define renderer for the geometry (all of the Observations are point geometry)
const observationRenderer = {
type: "simple", // autocasts as new SimpleFillSymbol()
symbol: {
type: "simple-marker",
size: 6,
color: "yellow",
outline: { // autocasts as new SimpleLineSymbol()
width: 0.5,
color: "black"
}
}
};
//define labels
const observationLabels = new LabelClass({
labelExpressionInfo: {
expression: `$feature.species_guess`
},
labelPlacement: "above-center",
symbol: {
type: "text", // autocasts as new TextSymbol()
color: "black"
}
})
Enable popups and apply a renderer to the sublayers once the composite layer is loaded. The labels and renderers are applied to the KnowledgeGraphSublayer that represents each named type included in the KnowledgeGraphLayer (i.e. each entity type or relationship type).
//once the layer has loaded, enable popups and apply the renderer to each sublayer
//since an inclusion list is set to only return Observations, there will only be one sublayer
await compositeLayer.load()
compositeLayer.layers.items.forEach((sublayer) => {
sublayer.popupTemplate = sublayer.createPopupTemplate();
sublayer.renderer = observationRenderer;
sublayer.labelingInfo = [observationLabels];
sublayer.labelsVisible = true;
map.add(compositeLayer);
});