require(["esri/tasks/AlgorithmicColorRamp"], function(AlgorithmicColorRamp) { /* code goes here */ });
Description
(Added at v2.6)
Create an algorithmic color ramp to define the range of colors used in the renderer generated by the
GenerateRendererTask. The algorithmic color ramp is defined by specifying two colors and the algorithm used to traverse the intervening color spaces. Specify the from and to colors using
esri/Color.
There are three algorithms that can be used to define the color values between the from and to colors: 'cie-lab', 'hsv', and 'lab-lch'. There is very little difference between these algorithms when the from and the to colors are of the same or very similar hues. But when the hues for the from and to colors are different (Hue is different by 40 or more on a 0-360 scale), the algorithms produce different results. The 'hsv' algorithim traverses the hue difference in a purely linear fashion, resulting in a bright ramp where all intermediate colors are represented. For instance, a ramp from red to green would include orange, yellow, and yellow-green. The 'cie-lab' and 'lab-lch' produce a more blended result. Thus, a ramp from dark green to orange would not contain a bright yellow, but instead a more brown and green-gold or green-brown intermediate color. The advantage of the 'cie-lab' algorithm is that the colors of the ramp are visually equidistant, which can produce a better ramp.
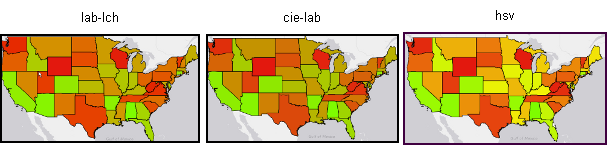
Samples
Search for
samples that use this class.
Class hierarchy
esri/tasks/ColorRamp
|_esri/tasks/AlgorithmicColorRamp
Constructors
Properties
Methods
toJson() | Object | Returns an easily serializable object representation of an algorithmic color ramp. |
Constructor Details
Creates a new AlgorithmicColorRamp object.
Sample:
require([
"esri/tasks/AlgorithmicColorRamp", ...
], function(AlgorithmicColorRamp, ... ) {
var colorRamp = new AlgorithmicColorRamp();
...
});
Property Details
The algorithm used to generate the colors between the
fromColor
and
toColor
. Each algorithm uses different methods for generating the intervening colors.
cie-lab |
Blends the from and to colors without traversing the intervening hue space. |
lab-lch |
The hue, saturation, value (hsv) algorithm is a linear traverse of colors between pairs: Color 1 H to Color 2 H, Color 1 S to Color 2 S, and Color 1 V to Color 2 V. |
hsv |
The lab-lch algorithm is very similar to the cie-lab but does not seek the shortest path between colors. |
Known values: cie-lab | hsv | lab-lch
Sample:
colorRamp.algorithm = "hsv";
The first color in the color ramp.
Sample:
require([
"esri/Color", ...
], function(Color, ... ) {
colorRamp.fromColor = new Color.fromHex('#7CFC00');
...
});
The last color in the color ramp.
Sample:
require([
"esri/Color", ...
], function(Color, ... ) {
colorRamp.toColor = new Color.fromHex('#E3170D');
...
});
A string value representing the color ramp type.
Method Details
Returns an easily serializable object representation of an algorithmic color ramp.