require(["esri/rest/support/FixedIntervalBinParameters"], (FixedIntervalBinParameters) => { /* code goes here */ });
import FixedIntervalBinParameters from "@arcgis/core/rest/support/FixedIntervalBinParameters.js";
esri/rest/support/FixedIntervalBinParameters
FixedIntervalBinParameters specifies binParameters on AttributeBinsQuery object.
For the FixedIntervalBinParameters, the number of bins is not important but the interval
size must match with the input data. This means that each bin will cover a range that exactly fits the specified interval. The fixed interval binning works with numeric
fields and fields of the date
type only. It does not support timestamp-offset
, date-only
, or time-only
field types.
For example, if you want to visualize the distribution temperature data in a specific area using fixed intervals of 5 degrees, you can use fixed interval bins to categorize the data effectively:
// Query bins with fixed interval bin parameters based on field "temp" with 5 degrees interval.
const binQuery = new AttributeBinsQuery({
binParameters: new FixedIntervalBinParameters({
interval: 5, // the interval size for each bin. In this case, 5 degrees celsius
field: "temp", // the field to bin, containing the temperature data
start: 0, // the lower boundary of the first bin. 0 degrees celsius
end: 30 // the upper boundary of the last bin. 30 degrees celsius
})
});
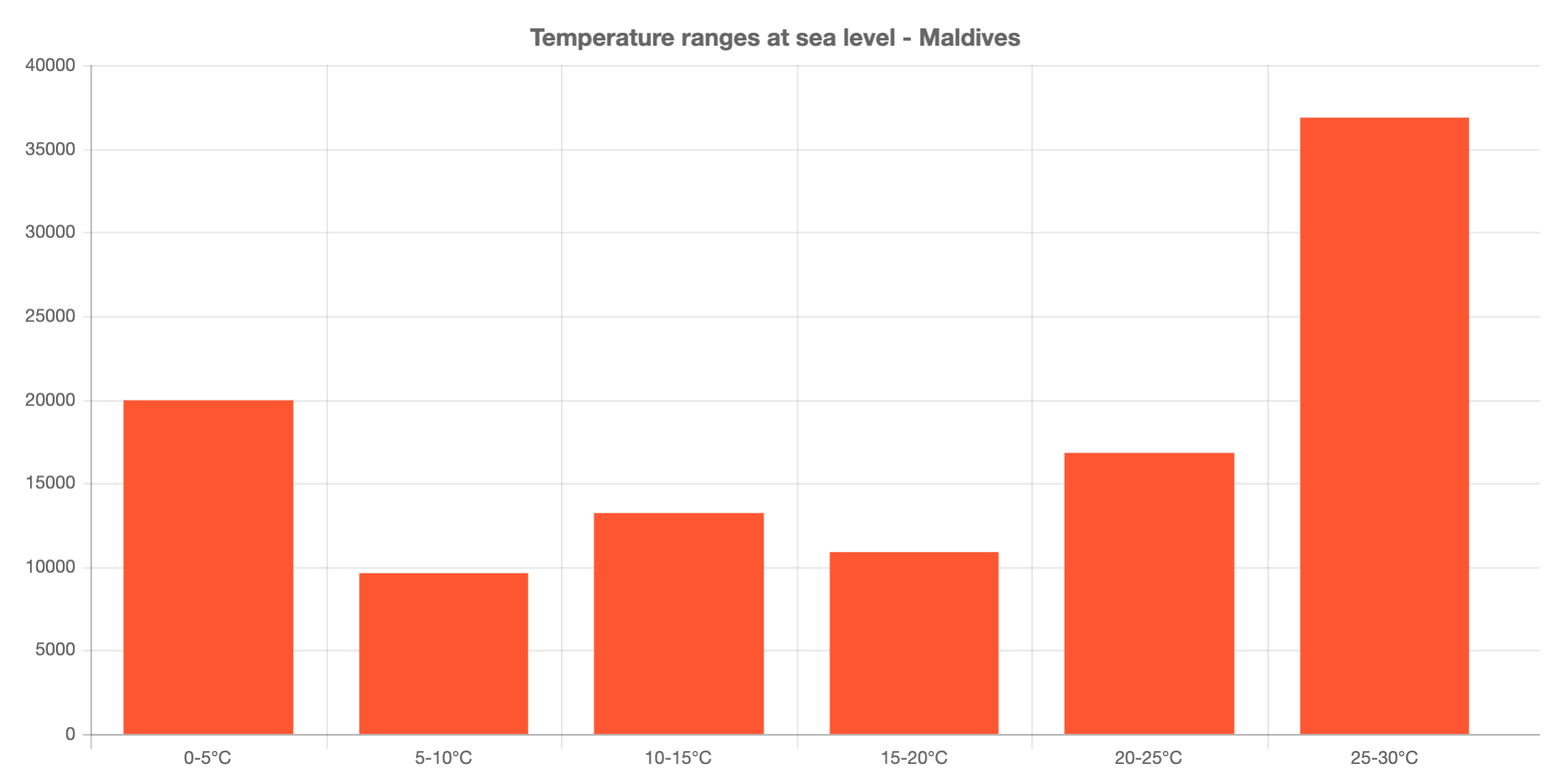
- See also
Constructors
-
Parameterproperties Objectoptional
See the properties for a list of all the properties that may be passed into the constructor.
Property Overview
Name | Type | Summary | Class |
---|---|---|---|
The name of the class. | Accessor | ||
The end value of bins to generate. | FixedIntervalBinParameters | ||
A standardized SQL expression used to calculate the bins, rather than referencing a field. | FixedIntervalBinParameters | ||
Specifies the expected data type of the output from the expression, based on the type of value the expression generates. | FixedIntervalBinParameters | ||
The field name used to calculate the bins. | FixedIntervalBinParameters | ||
The first day of the week. | FixedIntervalBinParameters | ||
If | FixedIntervalBinParameters | ||
Represents the interval of values to be included in each bin. | FixedIntervalBinParameters | ||
Name of the numeric field used to normalize values during binning. | FixedIntervalBinParameters | ||
The maximum value used to normalize the data during binning. | FixedIntervalBinParameters | ||
The minimum value used to normalize the data during binning. | FixedIntervalBinParameters | ||
The total value used when the normalizationType is | FixedIntervalBinParameters | ||
Normalization type used to normalize data during binning. | FixedIntervalBinParameters | ||
The | FixedIntervalBinParameters | ||
The | FixedIntervalBinParameters | ||
The start value of bins to generate. | FixedIntervalBinParameters | ||
The type of bin parameters. | FixedIntervalBinParameters |
Property Details
-
The end value of bins to generate.
- Default Value:null
-
A standardized SQL expression used to calculate the bins, rather than referencing a field. The specified SQL expression must evaluate to a numeric or date type. Both field and the
expression
cannot be specified for the same bin. The expressionValueType property must be set to the expected data type of the output from the expression.Known Limitations
The
expression
is only supported with server-side FeatureLayers.- See also
Exampleconst binQuery = new AttributeBinsQuery({ binParameters: new AutoIntervalBinParameters({ numBins: 5, // the interval size for each bin // sql expression to calculate the bins based on the product of Quantity and SalesAmount expression: "Quantity * SalesAmount" expressionValueType: "double" }) });
-
Specifies the expected data type of the output from the expression, based on the type of value the expression generates. The expression property must be set when this property is set.
Known Limitations
The
expressionValueType
is only supported with server-side FeatureLayers.Possible Values:"small-integer" |"integer" |"single" |"double" |"long" |"date-only" |"time-only" |"timestamp-offset" |"date"
- See also
Exampleconst binQuery = new AttributeBinsQuery({ binParameters: new AutoIntervalBinParameters({ numBins: 5, // the interval size for each bin // sql expression to calculate the bins based on the product of Quantity and SalesAmount expression: "Quantity * SalesAmount" expressionValueType: "double" }) });
-
The field name used to calculate the bins. The expression property cannot be set when this property is set.
- See also
-
The first day of the week. This property is used to determine the start of the week for date-based bins. The default value is 7, representing Sunday, if no value is specified.
Known Limitations
The
firstDayOfWeek
is only supported with server-side FeatureLayers.
-
If
true
, theupperBoundary
andbin
fields will not be included in the attributes.Known Limitations
The
hideUpperBound
is only supported with server-side FeatureLayers.
-
interval
interval Number
-
Represents the interval of values to be included in each bin. For example, if you want to visualize the distribution temperature data in a specific area using fixed intervals of 5 degrees, you can set the interval to 5.
-
Name of the numeric field used to normalize values during binning. The normalization field must be specified when the normalizationType is set to
field
. Only non-zero values from thenormalizationField
are used during binning.
-
The maximum value used to normalize the data during binning. It ensures that all data points are scaled relative to this maximum value.
Known Limitations
The
normalizationMaxValue
is only supported with server-side FeatureLayers.
-
The minimum value used to normalize the data during binning. It ensures that all data points are scaled relative to this minimum value.
Known Limitations
The
normalizationMinValue
is only supported with server-side FeatureLayers.
-
The total value used when the normalizationType is
percent-of-total
. Percent of total takes each data value, divides it by the normalizationTotal of all values, and then multiplies by 100 to turn it into a percentage.
-
Normalization type used to normalize data during binning. Some analytical methods require that data be normally distributed. When the data is skewed (the distribution is lopsided) you can transform the data to make it normal.
The following normalization types are supported:
Value Description natural-log Natural logarithmic transformation can be used when the data has a positively skewed distribution and there are a few large values. If these large values are located in the dataset, the log transformation will help make the variances more constant and normalize the data. log The logarithmic transformation (base 10) is similar to the natural logarithmic transformation. It is used to reduce skewness, particularly in datasets with large values. square-root Square root transformation is similar to a logarithmic transformation in that it reduces right skewness of a dataset. Unlike logarithmic transformations, square root transformations can be applied to zero. percent-of-total Percent of total takes each data value, divides it by the normalizationTotal of all values, and then multiplies by 100 to turn it into a percentage. If you don’t provide a normalizationTotal
, it will be calculated automatically.field Divides the data value by the value from the specified field. You must provide the normalizationField, and values of 0 are filtered out. Possible Values:"natural-log" |"square-root" |"percent-of-total" |"log" |"field"
-
splitBy
splitBy AttributeBinsGrouping |null |undefinedautocast
-
The
splitBy
parameter divides data into separate bins for each unique value in the categorical field. Each category will have different bin boundaries based on the data distribution within that category. For example, when analyzing sales by region (e.g., Central, Northeast, etc.),splitBy
will create separate bin ranges for each region, allowing the boundaries to adjust to the specific data distribution of each region. ThesplitBy
parameter is useful when the distribution of values within each category (e.g., branch or region) differs significantly, and you want each category’s binning to reflect its unique data range.- Field with many unique values are not appropriate for splitting bins into multiple series.
Example// create bins based on the SalesTotal field, split by the Branch field. const binQuery = new AttributeBinsQuery({ binParameters: new AutoIntervalBinParameters({ numBins: 5, // the interval size for each bin field: "SalesTotal", splitBy: { // autocasts to AttributeBinsGrouping type: "field", value: "Branch" } }) }); const result = await layer.queryAttributeBins(binQuery);
-
stackBy
stackBy AttributeBinsGrouping |null |undefinedautocast
-
The
stackBy
parameter divides each bin into segments based on unique values from a categorical field, allowing you to compare multiple categories within the same bin while keeping the bin boundaries consistent across all categories. This enables you to visualize or analyze the distribution of categories stacked together within the same range of values. For example, with 3 bins based on total sales with ranges like $0 to $5000, $5001 to $10,000, and $10,001 to $15,000, settingstackBy = SalesRep
will stack each sales rep's contribution within the same bin range. The bin boundaries remain the same, and each segment within the bin shows how individual categories contribute to the total frequency or value.- Field with many unique values are not appropriate for splitting bins into multiple series.
Example// create bins based on the SalesTotal field, stacked by the Month field. const binQuery = new AttributeBinsQuery({ binParameters: new AutoIntervalBinParameters({ numBins: 5, // the interval size for each bin field: "SalesTotal", stackBy: { value: "EXTRACT(MONTH from invoiceDate)", type: "expression", valueType: "double", alias: "Month" } }) }); const result = await layer.queryAttributeBins(binQuery);
-
The start value of bins to generate.
- Default Value:null
-
type
type Stringreadonly
-
The type of bin parameters.
For FixedIntervalBinParameters the type is always "fixed-interval".
Method Overview
Name | Return Type | Summary | Class |
---|---|---|---|
Adds one or more handles which are to be tied to the lifecycle of the object. | Accessor | ||
Creates a new instance of this class and initializes it with values from a JSON object generated from an ArcGIS product. | FixedIntervalBinParameters | ||
Returns true if a named group of handles exist. | Accessor | ||
Removes a group of handles owned by the object. | Accessor | ||
Converts an instance of this class to its ArcGIS portal JSON representation. | FixedIntervalBinParameters |
Method Details
-
Inherited from Accessor
-
Adds one or more handles which are to be tied to the lifecycle of the object. The handles will be removed when the object is destroyed.
// Manually manage handles const handle = reactiveUtils.when( () => !view.updating, () => { wkidSelect.disabled = false; }, { once: true } ); this.addHandles(handle); // Destroy the object this.destroy();
ParametershandleOrHandles WatchHandle|WatchHandle[]Handles marked for removal once the object is destroyed.
groupKey *optionalKey identifying the group to which the handles should be added. All the handles in the group can later be removed with Accessor.removeHandles(). If no key is provided the handles are added to a default group.
-
Creates a new instance of this class and initializes it with values from a JSON object generated from an ArcGIS product. The object passed into the input
json
parameter often comes from a response to a query operation in the REST API or a toJSON() method from another ArcGIS product. See the Using fromJSON() topic in the Guide for details and examples of when and how to use this function.Parameterjson ObjectA JSON representation of the instance in the ArcGIS format. See the ArcGIS REST API documentation for examples of the structure of various input JSON objects.
Returns
-
hasHandles
InheritedMethodhasHandles(groupKey){Boolean}
Inherited from Accessor -
Returns true if a named group of handles exist.
ParametergroupKey *optionalA group key.
ReturnsType Description Boolean Returns true
if a named group of handles exist.Example// Remove a named group of handles if they exist. if (obj.hasHandles("watch-view-updates")) { obj.removeHandles("watch-view-updates"); }
-
Inherited from Accessor
-
Removes a group of handles owned by the object.
ParametergroupKey *optionalA group key or an array or collection of group keys to remove.
Exampleobj.removeHandles(); // removes handles from default group obj.removeHandles("handle-group"); obj.removeHandles("other-handle-group");
-
toJSON
toJSON(){Object}
-
Converts an instance of this class to its ArcGIS portal JSON representation. See the Using fromJSON() guide topic for more information.
ReturnsType Description Object The ArcGIS portal JSON representation of an instance of this class.