This sample demonstrates how to display values returned from an Arcade expression in a PopupTemplate defined on a FeatureLayer. Arcade is useful for creating visualizations in a FeatureLayer based on a value calculated from an expression executed on the client. PopupTemplates can reference the same expressions used in renderers to effectively communicate the data-driven visualization.
In this sample, a layer representing U.S. counties is added to the map. Each feature contains attributes related to labor statistics in the county. The fields include the unemployment rate, population, and total employment. Arcade is used to calculate the labor force participation of each county.
Four arcade expressions are used in the app:
- One for categorizing counties by whether the majority of the working-age population is participating in the labor force. This is an expression determining the predominant value among a set of values.
<script type="text/plain" id="predominance-arcade">
// The fields from which to calculate predominance
// The expression will return the alias of the predominant field
var fields = [
{ value: $feature.NOT_LABORFORCE_16, alias: "NOT participating in the labor force" },
{ value: $feature.CIVLBFR_CY, alias: "participating in labor the force" }
];
// Returns the predominant category as the alias
// defined in the fields array. If there is a tie,
// then both names are concatenated and used to
// indicate the tie
function getPredominantCategory(fieldsArray){
var maxValue = -Infinity;
var maxCategory = "";
for(var k in fieldsArray){
if(fieldsArray[k].value > maxValue){
maxValue = fieldsArray[k].value;
maxCategory = fieldsArray[k].alias;
} else if (fieldsArray[k].value == maxValue){
maxCategory = maxCategory + "/" + fieldsArray[k].alias;
}
}
return maxCategory;
}
getPredominantCategory(fields);
</script>
- Another for returning the majority category's value.
<script type="text/plain" id="strength-arcade">
// Returns the share of the dominant demographic as a percentage
var fieldValues = [ $feature.NOT_LABORFORCE_16, $feature.CIVLBFR_CY ];
var winner = Max(fieldValues);
var total = Sum(fieldValues);
return (winner/total) * 100;
</script>
- One that returns the total population not employed or in the labor force.
$feature.POP_16UP - $feature.EMP_CY;
- Lastly, another one for the percentage of the working-age population that is not employed.
Round((($feature.POP_16UP - $feature.EMP_CY) / $feature.POP_16UP) * 100) + "%";
Each Arcade expression must be referenced in the expressionInfos property of the PopupTemplate. The expression
is an array of objects that assigns a name and a title to each expression.
const arcadeExpressionInfos = [
{
name: "predominance-arcade",
title: "Is the majority (>50%) of the population 16+ participating in the labor force?",
// defined in separate script element
expression: document.getElementById("predominance-arcade").text
},
{
name: "strength-arcade",
title: "% of population belonging to majority category",
// defined in separate script element
expression: document.getElementById("strength-arcade").text
},
{
name: "not-working-arcade",
title: "Total population 16+ not employed or in labor force",
expression: "$feature.POP_16UP - $feature.EMP_CY"
},
{
name: "%-not-working-arcade",
title: "% of population 16+ not employed or in labor force",
expression: "Round((($feature.POP_16UP - $feature.EMP_CY)/$feature.POP_16UP)*100,2) + '%'"
}
];
popupTemplate.expressionInfos = arcadeExpressionInfos;
The name
is used to reference the expression value in the popupTemplate's content. The name must be prefaced by expression/
.
popupTemplate.content = [
{
type: "text",
text:
"In this county, {UNEMPRT_CY}% of the labor force is unemployed. " +
" {expression/strength-arcade}% of the {POP_16UP} people ages 16+" +
" living here are {expression/predominance-arcade}."
},
{
type: "fields",
fieldInfos: [
{
fieldName: "expression/not-working-arcade",
format: {
digitSeparator: true,
places: 0
}
},
{
fieldName: "expression/%-not-working-arcade",
format: {
digitSeparator: true,
places: 0
}
}
]
}
];
The values circled in red are those returned from the Arcade expressions referenced above.
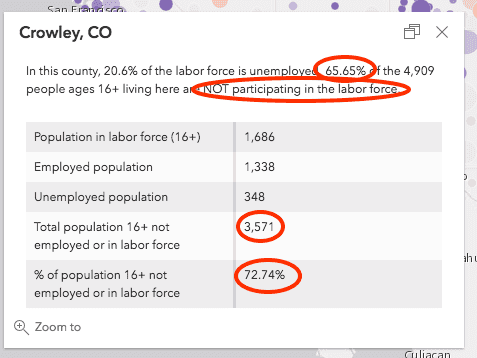
See the Popup profile of the Arcade expressions guide for more information.