This tutorial will walk you through working with popup templates.
Popups provide an easy way for users to access data attributes from layers and graphics. They enhance the interactivity of custom applications by allowing users to click on features in the map to view content specific to the selected feature.
Each view has a popup associated with it. In most cases, the content of the popup is set with a template specific to your data. The template is defined using PopupTemplate. The PopupTemplate may be set on individual graphics or on layers. When a feature inside a layer is selected by the user, the view's popup sets its content based on the PopupTemplate assigned to the layer.
In the most basic scenarios, you won't need to modify the properties of the view's popup. Rather, the majority of popup customization may be handled with the PopupTemplate.
This sample will walk you through how to use the PopupTemplate in a FeatureLayer. The principles mentioned here may similarly be applied to other layer types, such as ImageryLayer.
Prior to completing the following steps, you should be familiar with views, Map, and FeatureLayer. If necessary, complete the following tutorials first:
In addition to the steps provided below, you can also set the Popup
via a text
type element directly within the content. For more information and an example, please see its API reference and a sample showing how to display multiple popupTemplate elements.
1. Create a FeatureLayer and add it to a map
Create a FeatureLayer using this service that depicts various statistics for zip codes in New York. Next, add it to a basic Map and then set the Map instance to a View. Your JavaScript should look similar to what is shown below:
require(["esri/Map", "esri/views/MapView", "esri/layers/FeatureLayer"], (
Map,
MapView,
FeatureLayer
) => {
// Create a FeatureLayer
const featureLayer = new FeatureLayer({
url: "https://services.arcgis.com/P3ePLMYs2RVChkJx/arcgis/rest/services/ACS_Marital_Status_Boundaries/FeatureServer/2"
});
// Create the Map and add the featureLayer defined above
const map = new Map({
basemap: "gray-vector",
layers: [featureLayer]
});
// Create the MapView
const view = new MapView({
container: "viewDiv",
map: map,
center: [-73.95, 40.702],
zoom: 10
});
});
2. Create a PopupTemplate object and add a title
The PopupTemplate allows you to show feature attributes, or data specific to the selected feature (such as region name, population, state code, etc.), inside both the title and content properties of the popup. Attributes are referenced in the following syntax: {Attribute
.
The PopupTemplate has a title property that will display at the top of the popup. Since this layer's renderer depicts marriage rates by zip code, we will add a title related to marriage with the zip code number of the selected geography.
const template = {
// NAME and COUNTY are fields in the service containing the Census Tract (NAME) and county of the feature
title: "{NAME} in {COUNTY}",
};
Since a PopupTemplate may be autocast you don't need to require the esri/Popup
module. Simply create a simple object containing the same properties as those specified in the PopupTemplate documentation and set it on the popup
property of the layer.
3. Add content to the PopupTemplate
The PopupTemplate's content property provides a template defining the content of the popup for each selected feature. In this application, we want to add a simple table that lists the marriage statistics related to the selected feature. The content is populated from the field
, which is an array of objects.
Add the following to the content of the template:
const template = {
// autocasts as new PopupTemplate()
title: "{NAME} in {COUNTY}",
content: [
{
type: "fields",
fieldInfos: [
{
fieldName: "B12001_calc_pctMarriedE",
label: "Married %"
},
{
fieldName: "B12001_calc_numMarriedE",
label: "People Married"
},
{
fieldName: "B12001_calc_numNeverE",
label: "People that Never Married"
},
{
fieldName: "B12001_calc_numDivorcedE",
label: "People Divorced"
}
]
}
]
};
Other content types may be added other than straight text. You can also add images, charts, tables, attachments, and any combination of the above to a popup. See the Multiple popup elements sample for an example of this.
The popup should look like the following:
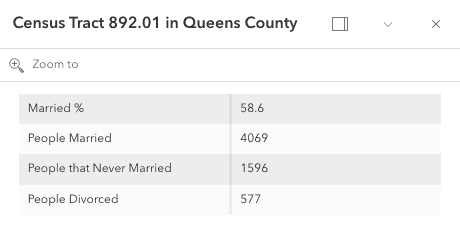
That's pretty good, but formatting the values will help the content look cleaner.
4. Use fieldInfos to format field values
The fieldInfos property of PopupTemplate allows you to format number and date fields. In this case, we want to use a comma separator for values larger than 1,000 and round to the nearest whole number in case any decimal values are provided.
See the code below on how this is accomplished:
const template = {
// autocasts as new PopupTemplate()
title: "{NAME} in {COUNTY}",
content: [
{
type: "fields",
fieldInfos: [
{
fieldName: "B12001_calc_pctMarriedE", // The field whose values you want to format
label: "Married %"
},
{
fieldName: "B12001_calc_numMarriedE", // The field whose values you want to format
label: "People Married",
format: {
digitSeparator: true, // Uses a comma separator in numbers >999
places: 0 // Sets the number of decimal places to 0 and rounds up
}
},
{
fieldName: "B12001_calc_numNeverE", // The field whose values you want to format
label: "People that Never Married",
format: {
digitSeparator: true, // Uses a comma separator in numbers >999
places: 0 // Sets the number of decimal places to 0 and rounds up
}
},
{
fieldName: "B12001_calc_numDivorcedE", // The field whose values you want to format
label: "People Divorced",
format: {
digitSeparator: true, // Uses a comma separator in numbers >999
places: 0 // Sets the number of decimal places to 0 and rounds up
}
}
]
}
]
};
5. Set the PopupTemplate on the layer
Set the template on the layer's popupTemplate property. This can be handled in the FeatureLayer's constructor or directly on the layer instance prior to adding it to the map.
featureLayer.popupTemplate = template;
The snippet above indicates that the popup should show on the view. In addition, a defined template is set on this popup instance.
The remaining steps will walk you through setting the content of the template inside the PopupTemplate's constructor. This is normally handled prior to setting the template on the layer.
Now the popup content should look formatted in the following manner:
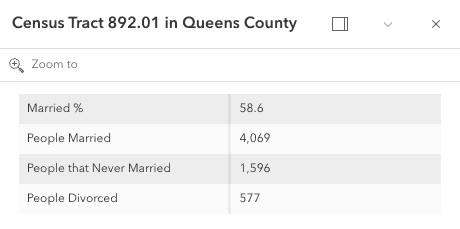
Click the Explore in the sandbox button above to view this full sample alongside its code.