This sample demonstrates how to create graphics with 3D symbols and how to update these graphics using the Sketch widget and SketchViewModel in a SceneView.
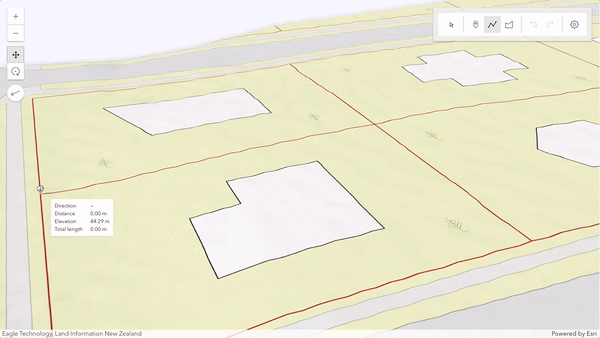
For example, a symbol with ExtrudeSymbol3DLayer is used for drawing extruded building footprints:
const sketchViewModel = new SketchViewModel({
layer: graphicsLayer,
view: view,
polygonSymbol: new PolygonSymbol3D({
symbolLayers: [
new ExtrudeSymbol3DLayer({
size: 3.5, // extrude by 3.5m meters
material: {
color: [255, 255, 255, 0.8]
},
edges: new SolidEdges3D({
size: 1,
color: [82, 82, 122, 1]
})
})
]
})
});
Reshape options
With reshapeOptions it is possible to modify the behavior for the edge and move operation within the reshape tool for polygon and polyline.
In this sample, the logic to toggle the reshape options is handled via a UI component that appears whenever Sketch
calls the update() operation.
The following code snippet demonstrates how to configure the reshape
manually.
defaultUpdateOptions: {
reshapeOptions: {
edgeOperation: "offset",
shapeOperation: "move"
}
}
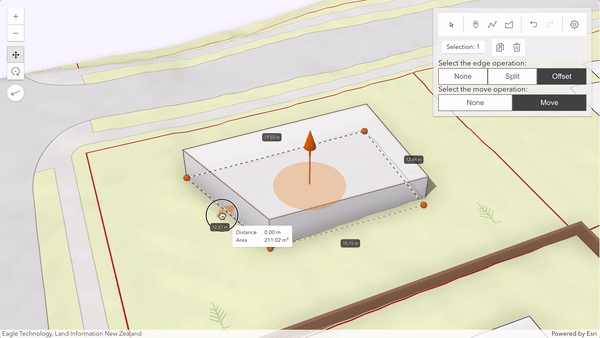
Snapping
It is also possible to configure snapping within the Sketch
widget. This is handled via the snappingOptions property.
The Sketch widget provides a UI to toggle snapping
.
...
sketchViewModel.snappingOptions.enabled = true;
sketchViewModel.snappingOptions.featureSources = [{ layer: graphicsLayer }];
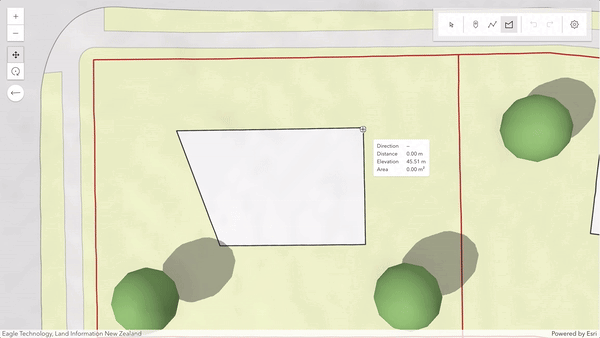
Tooltips, labels and sketching with constraints
The tooltips and segment labels
provide useful information when creating or updating existing features. They can be enabled programmatically or toggled via the Sketch widget's UI.
Pressing Tab
while drawing a new feature activates the tooltips' input mode. Then, one can set editing constraints to achieve greater control over segment lengths, angles, and elevation values.
...
sketchViewModel.tooltipOptions.enabled = true;
sketchViewModel.labelOptions.enabled = true;
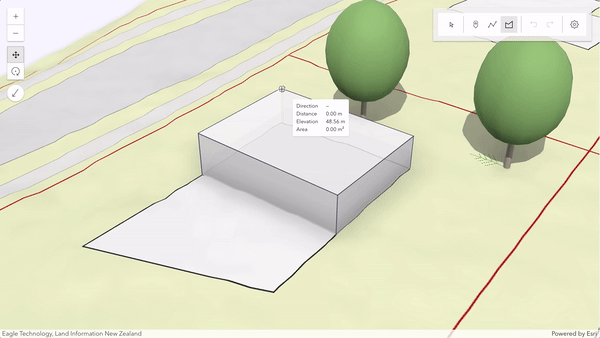
In this sample, when starting to draw a polygon or polyline, the tooltip shows the absolute direction mode (relative to the map's main axis, usually the north). But after the second vertex is drawn, the UI will switch to show relative direction (deflection) instead. This is set via the valueOptions.
...
if (event.state === "start") {
sketchViewModel.valueOptions.directionMode = "absolute";
}
if (
event.state === "active" &&
event.toolEventInfo.type === "vertex-add" &&
event.toolEventInfo.vertices[0].vertexIndex === 1
) {
sketchViewModel.valueOptions.directionMode = "relative";
}