This sample demonstrates how to adjust marker placement properties of a CIMSymbol and draw those symbols on a map using the SketchViewModel.
To modify the symbol properties in the app, either select the graphic already drawn in the view, or use the sketch tools in the top right to draw a new polygon. The panel options allow you to select a polygon style, update basic symbol properties such as color and size, and change the marker placement of the symbol.
Select a graphic in the view and change some of these properties to see them update in real time. Use the code icon in the top right of the UI to view the JSON of the CIMSymbol. You can copy this JSON and paste it into another app build with the ArcGIS Maps SDK for JavaScript, as shown in the code snippet below.
const symbol = new CIMSymbol({
data: // paste symbol JSON here
});
How this sample works
Each of the symbols in the gallery under Choose a polygon style
are created from WebStyleSymbols.
WebStyleSymbols are predefined vector symbols that can be published from ArcGIS Pro and conveniently referenced in a web app with a simple reference to a symbol name and a style name.
const webStyleSymbol = new WebStyleSymbol({
name: "Airport",
styleName: "EsriIconsStyle"
});
Each WebStyleSymbol is simply a wrapper to a full-fledged CIMSymbol. You can convert any WebStyleSymbol to its CIMSymbol representation using the fetchCIMSymbol method. Fetching the CIMSymbol allows you to access properties not available on the WebStyleSymbol, such as marker placement.
Using cimSymbolUtils
Under the Basic symbol properties
accordion, you can adjust the size and color of the selected CIMSymbol.
The utility methods in cimSymbolUtils help make updating symbol layer properties easier, especially since CIMSymbols can contain multiple symbol layers that each have a different color and size.
The "get" methods, such as cimSymbolUtils.getCIMSymbolSize() and
cimSymbolUtils.getCIMSymbolColor(), help us make sure our inputs are up-to-date whenever a new symbol is selected.
When one of these inputs is changed, you can use one of several "set" methods to update the symbol to the new value. For example, when the color input is adjusted, then the cimSymbolUtils.applyCIMSymbolColor() should be used to adjust the color of the current symbol to the new input value.
// updates all the non color locked symbol layers of the cim symbol
colorInput.addEventListener("calciteInputInput", () => {
const clonedSym = currentSymbol.clone();
cimSymbolUtils.applyCIMSymbolColor(clonedSym, colorInput.value);
handleSymbolUpdate(clonedSym);
});
CIMSymbol.markerPlacement
When a marker symbol (CIMVectorMarker or CIMPictureMarker)
is used inside a CIMPolygonSymbol, it needs to define a markerPlacement (either CIMMarkerPlacementInsidePolygon or CIMMarkerPlacementPolygonCenter).
All the web styles in this example use CIMMarker
, which places the markers inside the polygon at a fixed or random position.
Use the toggle under Marker placement properties
to toggle between Fixed
and Random
, and watch how this affects the symbol.
When one of the inputs under Marker placement properties
is adjusted, the app updates the marker
properties on the symbol by iterating through each symbol layer, looking for the symbol layer that contains the marker
property, and adjusting the values based on the input values in our app, as shown in the code snippet below.
// updates the marker placement on the symbol based on the UI components
function updateMarkerPlacement() {
let clonedSym = currentSymbol.clone();
// disable randomness slider if gridType is not Random
randomnessSlider.disabled = gridType.value !== "Random";
// disable shift odd rows checkbox if gridType is not Fixed
shiftOddRows.disabled = gridType.value == "Fixed" ? false : true;
// find the symbol layer that has marker placement set,
// and update based on the current UI values
clonedSym.data.symbol.symbolLayers.forEach((symLayer, index) => {
if (symLayer.markerPlacement) {
let mp = symLayer.markerPlacement;
mp.gridType = gridType.value;
mp.randomness = randomnessSlider.value;
mp.stepX = stepXSlider.value;
mp.stepY = stepYSlider.value;
mp.shiftOddRows = shiftOddRows.checked;
clonedSym.data.symbol.symbolLayers[index].markerPlacement = mp;
}
});
handleSymbolUpdate(clonedSym);
}
Related samples and resources
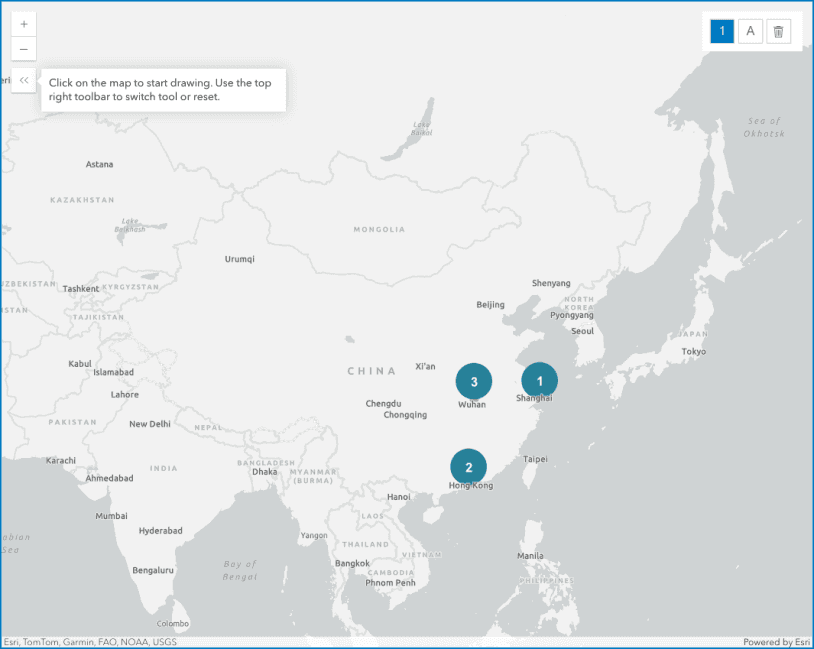
Intro to CIMSymbol
Learn the basics about CIMSymbol and how to use a primitiveOverride on the text
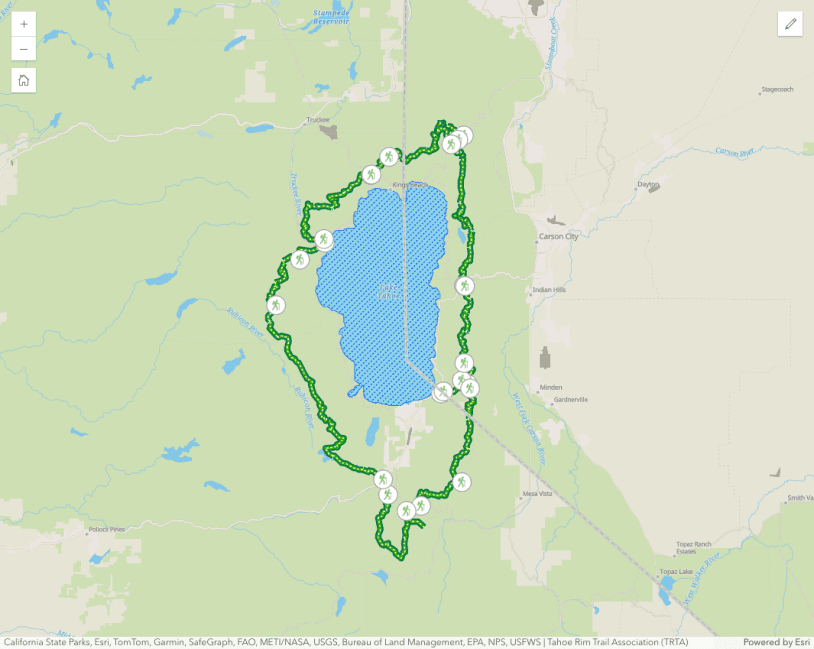
CIMSymbol lines and polygons
Learn how to create CIM line and polygon symbols.
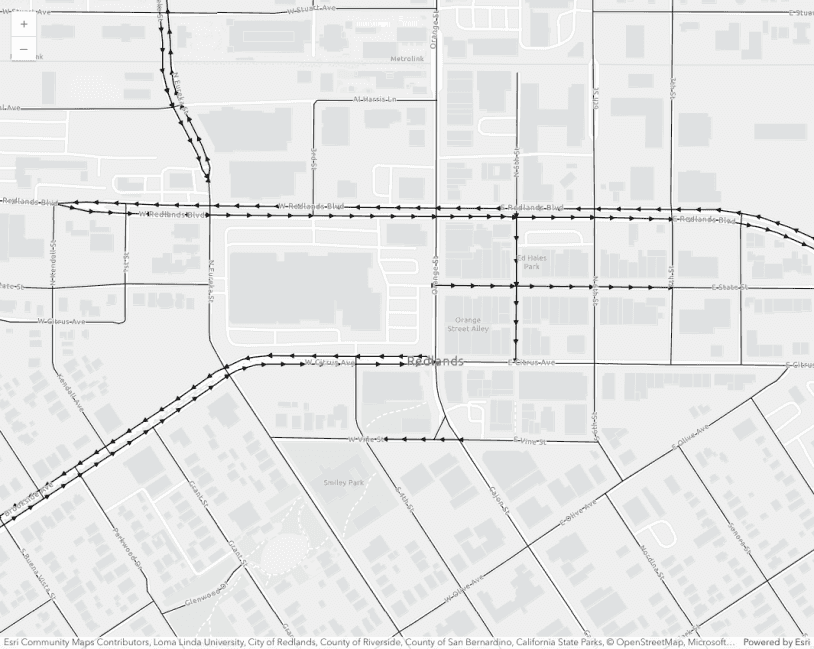
Arrows along a line
Use a CIMSymbol to draw a line with arrow markers at a fixed distance
CIMSymbol
Read the API Reference for more information.
Esri Web Style Symbols (2D)
Browse dozens of 2D web styles and copy/paste the required code into your app
Symbol Builder
Symbol Builder application provides a UI for creating any symbol