Learn how to use Charts Components and Map Components using Vite developer build tools.
In this tutorial, you will:
- Render a webmap with Map Components and a chart (scatterplot) with Charts Components.
- Add the action bar component to the chart, and explore different functionality such as filter by extent and sync up the selection between the chart and the map.
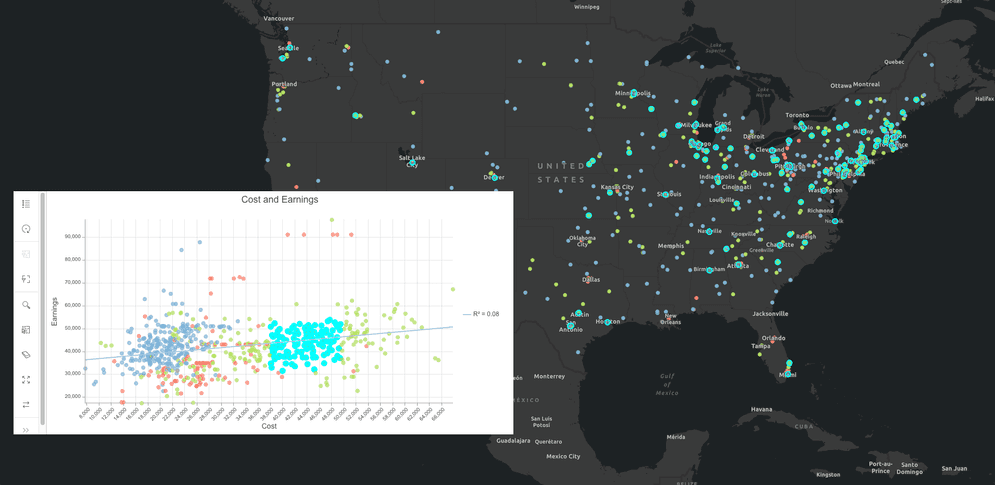
Prerequisites
- The most recent LTS Node.js runtime environment.
- A text editor to edit files.
- A terminal to enter commands.
Steps
Create a new project using Vite
- Download the initial Vite vanilla Javascript project to your local machine.
- Unzip the downloaded file.
- Open the unzipped folder's files in your text editor.
- Navigate to the unzipped folder in your terminal.
Install dependencies
-
Install Charts Components and Map Components in the terminal window as a dependency.
Use dark colors for code blocks Copy npm install @arcgis/charts-components @arcgis/map-components
-
Later in this tutorial we will need to know which version of
@esri/calcite-components
was installed as a dependency of@arcgis/map-components
, run this command in the terminal window to find the correct version.Use dark colors for code blocks Copy npm list @esri/calcite-components
-
Start the Vite development server.
Use dark colors for code blocks Copy npm run dev
-
Open a web browser and navigate to
http:
. Initially, this web page will be empty, but it will update as we proceed through the remaining steps.//localhost: 5173 -
Import functions to define the custom HTML elements from the Charts Components, Map Components and Calcite Components libraries in
main.js
. You will need to use an alias, because the imported function from each library has the same name,define
.Custom Elements Use dark colors for code blocks Copy import { defineCustomElements as defineCalciteElements } from '@esri/calcite-components/dist/loader'; import { defineCustomElements as defineChartsElements } from '@arcgis/charts-components/dist/loader'; import { defineCustomElements as defineMapElements } from '@arcgis/map-components/dist/loader';
-
Define the custom elements on the window using the Calcite Components distribution build. You will use CDN-hosted assets. When using the CDN-hosted assets, you need to keep the version number in the path the same as the version of
@esri/calcite-components
found in step two.Use dark colors for code blocks Copy defineCalciteElements(window, { resourcesUrl: 'https://js.arcgis.com/calcite-components/2.8.5/assets' });
-
Next, use the Charts Components and Map Components distribution build to define and lazy load the custom charts elements.
Use dark colors for code blocks Copy defineMapElements(window, { resourcesUrl: 'https://js.arcgis.com/map-components/4.30/assets' }); defineChartsElements(window, { resourcesUrl: 'https://js.arcgis.com/charts-components/4.30/t9n' });
Add styles
-
In
style.css
, import the @arcgis/core light theme.Use dark colors for code blocks Copy @import 'https://js.arcgis.com/4.30/@arcgis/core/assets/esri/themes/light/main.css';
-
Add CSS styles to the scatterplot in
style.css
. This will position the chart component directly on top of the map component.Use dark colors for code blocks Copy html, body { padding: 0; margin: 0; height: 100%; width: 100%; } #scatterplot { position: absolute; bottom: 100px; left: 30px; height: 40%; width: 50%; }
-
Import the CSS file in
main.js
.Use dark colors for code blocks Copy import './style.css';
Add components
-
Add the
arcgis-map
component to index.html under<body>
.Use dark colors for code blocks Copy <arcgis-map item-id="2c7d98b24ed64da0985666d937c85196"></arcgis-map>
-
Add the
arcgis-charts-scatter-plot
component to index.html under<body>
.Use dark colors for code blocks Copy <arcgis-charts-scatter-plot id="scatterplot"></arcgis-charts-scatter-plot>
-
Add the
arcgis-charts-action-bar
component to be slotted inside the scatterplot component.Use dark colors for code blocks Copy <arcgis-charts-scatter-plot id="scatterplot"> <arcgis-charts-action-bar slot="action-bar"></arcgis-charts-action-bar> </arcgis-charts-scatter-plot>
Create a reference to components
- In
main.js
, create a reference to thearcgis-map
,arcgis-charts-scatter-plot
andarcgis-charts-action-bar
components.Use dark colors for code blocks Copy const mapElement = document.querySelector('arcgis-map'); const scatterplotElement = document.querySelector('arcgis-charts-scatter-plot'); const actionBarElement = document.querySelector('arcgis-charts-action-bar');
Load the scatterplot
-
In
main.js
, add an event listenerarcgis
to theView Ready Change map
.Element Use dark colors for code blocks Copy mapElement.addEventListener('arcgisViewReadyChange', (event) => { // to be implemented });
-
Inside the event listener, get the map and view from the event target.
Use dark colors for code blocks Copy const { map, view } = event.target;
-
Get the
College
layer from the map and the first chart'sScorecard_ Charts config
from the layer.Use dark colors for code blocks Copy const featureLayer = map.layers.find((layer) => layer.title === 'CollegeScorecard_Charts'); const scatterplotConfig = featureLayer.charts[0];
-
Assign the layer and set
config
to the scatterplot element.Use dark colors for code blocks Copy scatterplotElement.config = scatterplotConfig; scatterplotElement.layer = featureLayer;
Show selected features from the chart on the map
-
In the
arcgis
event listener, get the layer views from the view.View Ready Change Use dark colors for code blocks Copy const featureLayerViews = view.layerViews;
-
Add an event listener to the scatterplot element. When the
arcgis
event is fired, remove the previous selection and highlight the currently selected features on the map.Charts Selection Complete Use dark colors for code blocks Copy scatterplotElement.addEventListener('arcgisChartsSelectionComplete', (event) => { map.highlightSelect?.remove(); map.highlightSelect = featureLayerViews.items[0].highlight(event.detail.selectionOIDs); });
Add functionality to filter by extent
-
In the
arcgis
event listener's callback function, add theView Ready Change arcgis
event listener to theCharts Default Action Select action
.B a r Element Use dark colors for code blocks Copy actionBarElement.addEventListener('arcgisChartsDefaultActionSelect', (event) => { // to be implemented });
-
In the
arcgis
event listener's callback function, get theCharts Default Action Select action
andI d action
properties from the event detail.Active Use dark colors for code blocks Copy const { actionId, actionActive } = event.detail;
-
Set the view of the scatterplot element to the map view if the
Filter By Extent
action is toggled on.Use dark colors for code blocks Copy if (actionId === 'filterByExtent') { if (mapElement.view !== undefined) { scatterplotElement.view = actionActive ? mapElement.view : undefined; } }
Show selected features from the map on the chart
-
Outside of the
arcgis
event listener, add a second event listener to theView Ready Change map
:Element arcgis
.View Click Use dark colors for code blocks Copy mapElement.addEventListener('arcgisViewClick', (event) => { // to be implemented });
-
In the
arcgis
event listener's callback, get the view from the event target.View Click Use dark colors for code blocks Copy const { view } = event.target;
-
Get the screen points from the event detail.
Use dark colors for code blocks Copy let screenPoints = event.detail.screenPoint; view.hitTest(screenPoints).then(getFeatures);
-
- Lastly, get the features from the hit test. With in the function, get the selected feature's OID (in this case, the attribute is
Object
) and assign it to the scatterplot element's selection data.I d
Use dark colors for code blocks Copy function getFeatures(response) { const selectedFeatureOID = response.results[0].graphic.attributes['ObjectId']; scatterplotElement.selectionData = { selectionOIDs: [selectedFeatureOID], }; }
- Lastly, get the features from the hit test. With in the function, get the selected feature's OID (in this case, the attribute is
Run the application
The app should display the scatterplot on top of the map. When you select some features on the chart, the corresponding features should also be selected on the map.