require(["esri/analysis/LengthDimension"], (LengthDimension) => { /* code goes here */ });
import LengthDimension from "@arcgis/core/analysis/LengthDimension.js";
esri/analysis/LengthDimension
LengthDimension enables the measurement of linear distances between the specified start and end points. Depending on the measure type, either the direct, horizontal, or vertical distance between these points is measured.
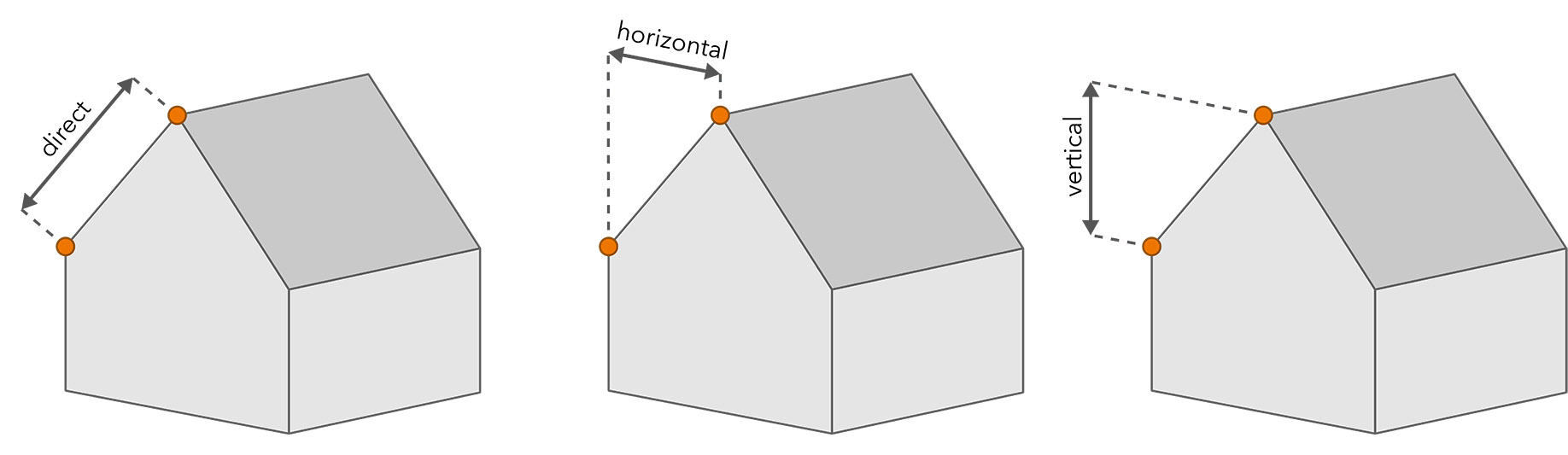
Length dimensions can be displayed by adding them to a DimensionAnalysis.
// create the dimension object
const lengthDimension = new LengthDimension({
measureType: "vertical",
startPoint: new Point({
spatialReference: {
wkid: 32610
},
x: 265,
y: 24,
z: 26
}),
endPoint: new Point({
spatialReference: {
wkid: 32610
},
x: 265,
y: 24,
z: 38
}),
orientation: 90,
offset: 2
});
// create analysis and add the dimension object to it.
const dimensionAnalysis = new DimensionAnalysis({
dimensions: [lengthDimension]
});
// add the analysis to the view
view.analyses.push(dimensionAnalysis);
Known Limitations
- Dimensioning is only supported in a 3D SceneView.
- Direct and vertical distances are always computed in a Euclidean manner.
- How horizontal distances are computed depends on the scene’s spatial reference:
- In geographic coordinate systems (GCS) and in Web Mercator, they are computed geodetically.
- In projected coordinate systems (PCS), apart from Web Mercator, they are computed in a Euclidean manner.
- Vertical and horizontal dimensions can be used to measure distances of up to 100 kilometers. To measure longer distances use the
"direct"
measureType
instead.
Constructors
-
Parameterproperties Objectoptional
See the properties for a list of all the properties that may be passed into the constructor.
Property Overview
Name | Type | Summary | Class |
---|---|---|---|
The name of the class. | Accessor | ||
Ending point for the dimension. | LengthDimension | ||
The type of length that should be measured between the startPoint and endPoint. | LengthDimension | ||
Styling option that controls the shortest distance from the startPoint or endPoint to the dimension line in meters. | LengthDimension | ||
The orientation determines the relative direction the dimension line is extended to. | LengthDimension | ||
Starting point for the dimension. | LengthDimension |
Property Details
-
measureType
measureType String
-
The type of length that should be measured between the startPoint and endPoint. The
measureType
allows the user to measure either the horizontal distance (delta in xy space), vertical distance (elevation difference), or direct distance between the start and end points. If either vertical or horizontal mode is used, the orientation is not applied and the offset direction is relative to the input points (on a plane derived from them).Possible Values:"direct" |"horizontal" |"vertical"
- Default Value:"direct"
-
offset
offset Number
-
Styling option that controls the shortest distance from the startPoint or endPoint to the dimension line in meters.
- Default Value:0
-
orientation
orientation Number
-
The orientation determines the relative direction the dimension line is extended to. It applies only to direct dimensions and when an offset is specified.
An orientation of 0 extends the offset upwards, whereas an orientation of 90 extends the offset sideways, to the right side of the dimension when viewing from its start point. When the start and end points are vertically aligned, increasing the orientation rotates the dimension clockwise relative to compass north.
- Default Value:0
Method Overview
Name | Return Type | Summary | Class |
---|---|---|---|
Adds one or more handles which are to be tied to the lifecycle of the object. | Accessor | ||
this | Creates a deep clone of this object. | LengthDimension | |
Returns true if a named group of handles exist. | Accessor | ||
Removes a group of handles owned by the object. | Accessor |
Method Details
-
Inherited from Accessor
-
Adds one or more handles which are to be tied to the lifecycle of the object. The handles will be removed when the object is destroyed.
// Manually manage handles const handle = reactiveUtils.when( () => !view.updating, () => { wkidSelect.disabled = false; }, { once: true } ); this.addHandles(handle); // Destroy the object this.destroy();
ParametershandleOrHandles WatchHandle|WatchHandle[]Handles marked for removal once the object is destroyed.
groupKey *optionalKey identifying the group to which the handles should be added. All the handles in the group can later be removed with Accessor.removeHandles(). If no key is provided the handles are added to a default group.
-
Creates a deep clone of this object. Any properties that store values by reference will be assigned copies of the referenced values on the cloned instance.
ReturnsType Description this A deep clone of the class instance that invoked this method.
-
hasHandles
InheritedMethodhasHandles(groupKey){Boolean}
Inherited from Accessor -
Returns true if a named group of handles exist.
ParametergroupKey *optionalA group key.
ReturnsType Description Boolean Returns true
if a named group of handles exist.Example// Remove a named group of handles if they exist. if (obj.hasHandles("watch-view-updates")) { obj.removeHandles("watch-view-updates"); }
-
Inherited from Accessor
-
Removes a group of handles owned by the object.
ParametergroupKey *optionalA group key or an array or collection of group keys to remove.
Exampleobj.removeHandles(); // removes handles from default group obj.removeHandles("handle-group"); obj.removeHandles("other-handle-group");