This sample demonstrates how to execute an Arcade expression outside the rendering context of a web map layer to create a scatter plot chart. Arcade expressions are executed in the context of a profile, which defines where an expression executes, which variables it can use, and what data types it can return. For example, the visualization Arcade profile is defined by the following rules:
- Execution context: Expressions may only execute in the
value
property of a renderer, visual variable, or CIMSymbol primitive override.Expression - Profile variables:
$feature
- The feature for which to calculate a value. Expressions have access to its geometry and attributes.$view.scale
- The view scale, for scale-dependent visualizations.
- Valid return types:
number
ortext
, depending on the context.
This example loads a web map that defines a renderer based on an Arcade expression that calculates the diversity of languages spoken in the home. The layer provides the total population that speak a language in each of five different language groups in the United States. The following is an expression referenced by a color visual variable, which colors each symbol along a continuous color ramp based on each feature's result of the expression.
This expression isn't present in the sample app, but it is stored in the web map.
var asian = $feature["B16007_calc_numAPIE"];
var english = $feature["B16007_calc_numEngOnlyE"];
var european = $feature["B16007_calc_numIEE"];
var other = $feature["B16007_calc_numOtherE"];
var spanish = $feature["B16007_calc_numSpanE"];
var groups = [asian,english,european,other,spanish];
// Returns a value 0-100.
// Indicates the % chance two people
// who speak different languages are
// randomly selected from the area.
function simpsonsDiversityIndex(vals){
var k = Array(Count(vals));
var t = sum(vals);
for(var i in vals){
var n = vals[i];
k[i] = n * (n-1);
}
var s = Sum(k);
var di = 1 - ( s / ( t * (t-1) ) );
return Round(di*100);
}
simpsonsDiversityIndex(groups)
This expression executes for each feature within the rendering engine, and the values are not available to the developer or end user for any other purpose. However, you can use the createArcadeExecutor method in the esri/arcade module to execute expressions stored in web maps (or used in any other part of your application) outside its original context for some other purpose. This sample shows how to do this for creating a chart based on the results of the expression above.
First, get the Arcade expression from the color variable in the layer's renderer.
// Arcade expression used by color visual variable
const colorVariableExpressionInfo = arcadeUtils
.getExpressionsFromLayer(spanishSpeakersLayer)
.filter(expressionInfo => expressionInfo.profileInfo.context === "color-variable")[0];
const diversityArcadeScript = colorVariableExpressionInfo.expression;
Then, define the profile used to execute the expression. This specifies the variables used and their associated Arcade types. You can either create the profile object yourself from the Arcade profile specs...
// Define the visualization profile variables
// Spec documented here:
// https://developers.arcgis.com/arcade/profiles/visualization/
const visualizationProfile = {
variables: [{
name: "$feature",
type: "feature"
}, {
name: "$view",
type: "dictionary",
properties: [{
name: "scale",
type: "number"
}]
}]
};
Or use the convenient createArcadeProfile method.
const visualizationProfile = arcade.createArcadeProfile("visualization");
Then create the executor, which compiles the expression for the given profile.
// Compile the Arcade expression and create an executor
const diversityArcadeExecutor =
await arcade.createArcadeExecutor(diversityArcadeScript, visualizationProfile);
// Ensure the input to $feature has all the
// data required by the expression.
// The additional field listed below is required by the y axis of
// the chart, but not used in the Arcade expression.
spanishSpeakersLayer.outFields = [
...diversityArcadeExecutor.fieldsUsed,
"B16007_calc_pctSpanE"
];
Once successfully compiled, execute the expression for each feature in the layer view. Once you have the results, you can build a chart or display the values in a table or some other component.
const spanishSpeakersLayerView = await view.whenLayerView(spanishSpeakersLayer);
const { features } = await spanishSpeakersLayerView.queryFeatures();
const chartPoints = features.map( async (feature) => {
// Execute the Arcade expression for each feature in the layer view
const diversityIndex = await diversityArcadeExecutor.executeAsync({
"$feature": feature,
"$view": view
});
const backgroundColor = await symbolUtils.getDisplayedColor(feature);
// Return the chart data along with the color used to represent
// the diversity index value in the color variable
return {
data: {
x: diversityIndex,
y: feature.attributes.B16007_calc_pctSpanE
},
backgroundColor
}
});
// Use a Chart API to build a chart from the Arcade results
const chartData = await Promise.all(chartPoints);
Related samples and resources
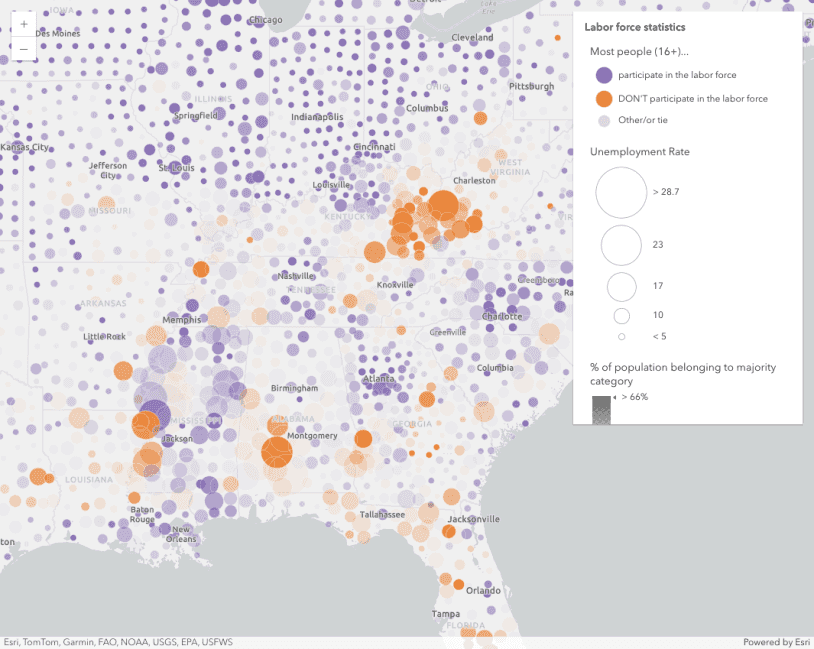
Reference Arcade expressions in PopupTemplate
Reference Arcade expressions in PopupTemplate
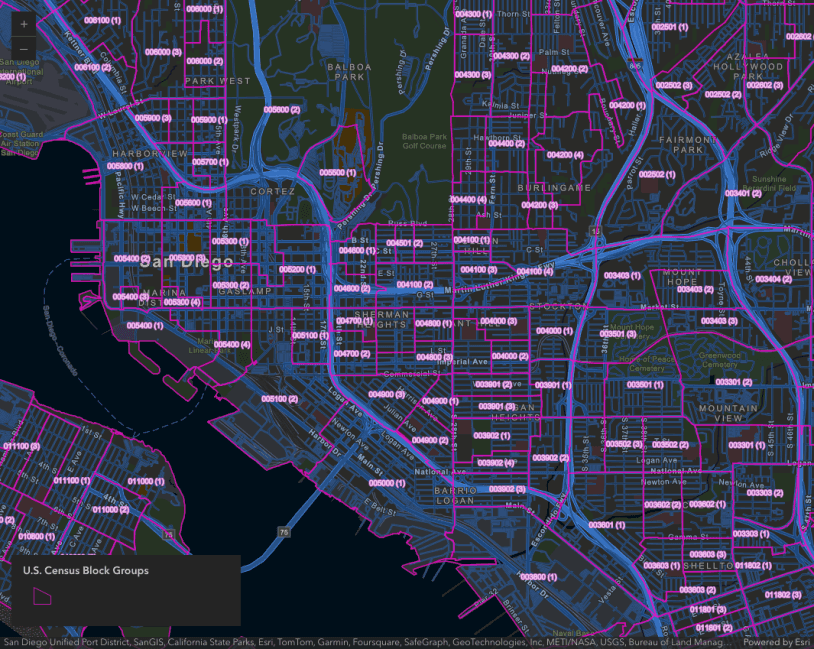
Summarize intersecting points in a popup
Summarize intersecting points in a popup
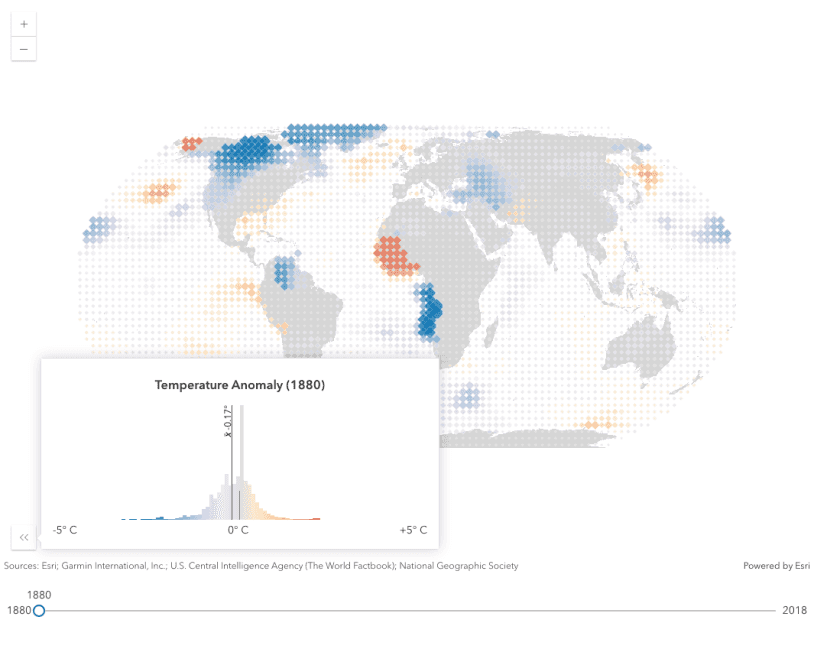
Update a renderer's attribute
Update a renderer's attribute's attribute
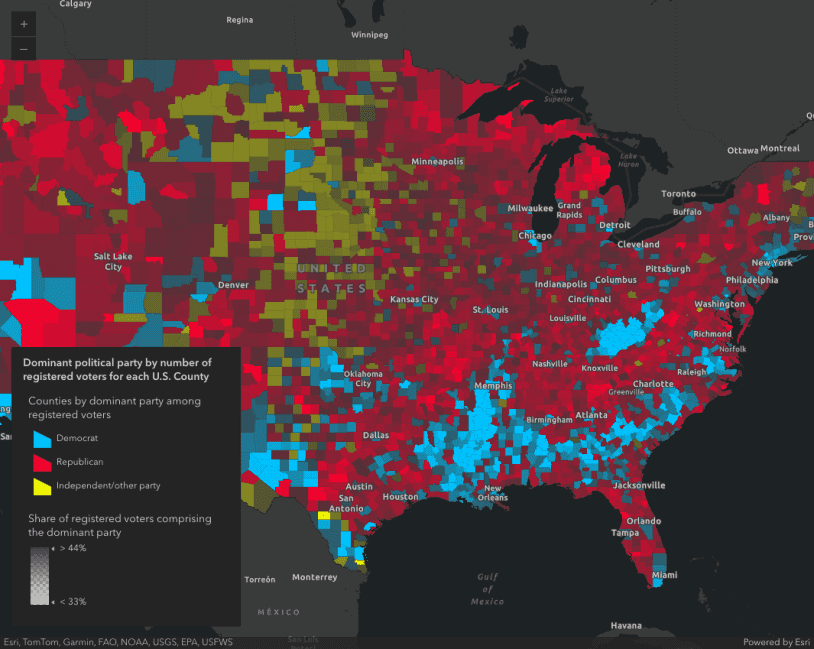
Create a custom visualization using Arcade
Create a custom visualization using Arcade
API Reference
Read the API Reference for more information.