Learn how to find demographic information for places around the world with GeoEnrichment service.
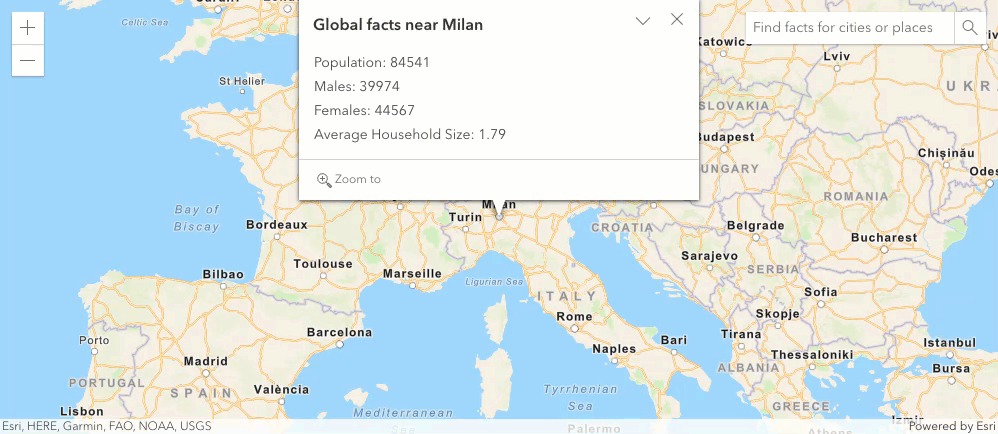
Prerequisites
Steps
Create a new pen
- To get started, either complete the Display a map tutorial or .
Get an access token
You need an access token with the correct privileges to access the location services used in this tutorial.
- Go to the Create an API key tutorial and create an API key with the following privilege(s):
- Privileges
- Location services > Basemaps
- Location services > Geocoding
- Location services > Data enrichment
- Privileges
- In CodePen, set
esri
to your access token.Config.api Key Use dark colors for code blocks var esriConfig = { apiKey: "YOUR_ACCESS_TOKEN" };
To learn about other ways to get an access token, go to Types of authentication.
Import ArcGIS REST JS
Import the ArcGIS REST JS modules to get access to some ArcGIS location services, such as access to global demographic data from the GeoEnrichment service.
<!-- Load ArcGIS REST JS libraries from https://unpkg.com -->
<script src="https://unpkg.com/@esri/arcgis-rest-request@4/dist/bundled/request.umd.js"></script>
<script src="https://unpkg.com/@esri/arcgis-rest-demographics@4/dist/bundled/demographics.umd.js"></script>
<!-- Load Calcite components from CDN -->
<link rel="stylesheet" type="text/css" href="https://js.arcgis.com/calcite-components/2.13.2/calcite.css" />
<script type="module" src="https://js.arcgis.com/calcite-components/2.13.2/calcite.esm.js"></script>
<!-- Load the ArcGIS Maps SDK for JavaScript from CDN -->
<link rel="stylesheet" href="https://js.arcgis.com/4.31/esri/themes/light/main.css" />
<script src="https://js.arcgis.com/4.31/"></script>
<!-- Load Map components from CDN -->
<script
type="module"
src="https://js.arcgis.com/map-components/4.31/arcgis-map-components.esm.js"
></script>
Update the map
A streets basemap layer is typically used in geocoding applications. Update the map's basemap
attribute to use the arcgis/navigation
basemap layer and change the position of the map to center on Milan, Italy.
-
Update the
basemap
property fromarcgis/topographic
toarcgis/navigation
, set thecenter
attribute to9.1900, 45.4642
and thezoom
attribute to8
.Use dark colors for code blocks <arcgis-map basemap="arcgis/navigation" center="9.1900, 45.4642" zoom="8"> <arcgis-zoom position="top-left"></arcgis-zoom> </arcgis-map>
Add the Search component
Next, add the Search component so the user can search for locations where they want to see the demographics.
-
Create the Search component inside the
arcgis-map
element. Set attributes to modify the placeholder text, disable the default popup, and place the search widget in the top-right corner of the map.Use dark colors for code blocks <arcgis-map basemap="arcgis/navigation" center="9.1900, 45.4642" zoom="8"> <arcgis-zoom position="top-left"></arcgis-zoom> <arcgis-search all-placeholder="Get demographic data" popup-disabled position="top-right"></arcgis-search> </arcgis-map>
Add script and modules
-
In a new
<script
at the bottom of the> <body
, use a> require
statement to add the geodesicBufferOperator, Graphic, locator, and SimpleFillSymbol modules.The ArcGIS Maps SDK for JavaScript is available as AMD modules and ES modules, but this tutorial is based on AMD. The AMD
require
function uses references to determine which modules will be loaded – for example, you can specify"esri/layers/
for loading the FeatureLayer module. After the modules are loaded, they are passed as parameters (e.g.Feature Layer" Feature
) to the callback function where they can be used in your application. It is important to keep the module references and callback parameters in the same order. To learn more about the API's different modules visit the Overview Guide page.Layer Use dark colors for code blocks <body> <arcgis-map basemap="arcgis/navigation" center="9.1900, 45.4642" zoom="8"> <arcgis-zoom position="top-left"></arcgis-zoom> <arcgis-search all-placeholder="Get demographic data" popup-disabled position="top-right"></arcgis-search> </arcgis-map> <script> require([ "esri/geometry/operators/geodesicBufferOperator", "esri/Graphic", "esri/rest/locator", "esri/symbols/SimpleFillSymbol" ], (geodesicBufferOperator, Graphic, locator, SimpleFillSymbol) => { }); </script> </body>
-
Use your API key to create a new authentication constant for ArcGIS REST JS in the require statement.
Use dark colors for code blocks <script> require([ "esri/geometry/operators/geodesicBufferOperator", "esri/Graphic", "esri/rest/locator", "esri/symbols/SimpleFillSymbol" ], (geodesicBufferOperator, Graphic, locator, SimpleFillSymbol) => { const authentication = arcgisRest.ApiKeyManager.fromKey(esriConfig.apiKey); }); </script>
-
When the user selects a search result, query for the demographics of that location. To do this, listen for the
arcgis
event on the Search component, then call aSelect Result get
function, which we will further define in the Get demographic data section.Demographic Data() Use dark colors for code blocks <script> require([ "esri/geometry/operators/geodesicBufferOperator", "esri/Graphic", "esri/rest/locator", "esri/symbols/SimpleFillSymbol" ], (geodesicBufferOperator, Graphic, locator, SimpleFillSymbol) => { const authentication = arcgisRest.ApiKeyManager.fromKey(esriConfig.apiKey); const arcgisSearch = document.querySelector("arcgis-search"); arcgisSearch.addEventListener("arcgisSelectResult", async (event) => { if (!event.detail.result) { return; } getDemographicData(event.detail.result.name, event.detail.result.feature.geometry); }); async function getDemographicData(city, point) { } }); </script>
Add click event on the map
We also want users to search for demographics by clicking anywhere on the map. To do this, add an event listener for the arcgis
event on the Map.
-
Add an event listener for the
arcgis
event to watch for when users click on the map.View Click Use dark colors for code blocks const arcgisMap = document.querySelector("arcgis-map"); arcgisMap.addEventListener("arcgisViewClick", async (event) => { });
-
When the event is returned, use the resulting
map
to set your parameters, then execute thePoint location
method on the locator with the specified parameters. This will return the address for which we want to find the demographics.To Address() Use dark colors for code blocks arcgisMap.addEventListener("arcgisViewClick", async (event) => { const params = { location: event.detail.mapPoint, outFields: "*" }; const locatorUrl = "https://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer"; const address = await locator.locationToAddress(locatorUrl, params); });
-
Once the
location
method has resolved, use the results to get the city's name closest to the click point. Use the city and the point clicked on the map to call theTo Address() get
method defined in the next step. If an error is encountered, remove all graphics from the map and log the error.Demographic Data() Use dark colors for code blocks arcgisMap.addEventListener("arcgisViewClick", async (event) => { const params = { location: event.detail.mapPoint, outFields: "*" }; const locatorUrl = "https://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer"; const address = await locator.locationToAddress(locatorUrl, params); try { const city = address.attributes.City || address.attributes.Region; getDemographicData(city, params.location); } catch (error) { arcgisMap.graphics.removeAll(); console.log(error); } });
Get demographic data
To get the demographic data for an area on the map, we will use the ArcGIS REST JS query
method.
-
Query for the demographic data using the
query
method from ArcGIS REST JS. Set theDemographic Data() study
to the longitude and latitude of our point parameter. Use theAreas authentication
variable set from our API key earlier to authenticate this request. Thequery
method will return a promise with the response, soDemographic Data() await
the result in an asynchronous function.Use dark colors for code blocks async function getDemographicData(city, point) { const demographicData = await arcgisRest.queryDemographicData({ studyAreas: [ { geometry: { x: point.longitude, y: point.latitude } } ], authentication: authentication }); }
-
Check that results were returned and add them to an attributes variable. Then, call the
show
function to show the results in a popup.Data() Use dark colors for code blocks async function getDemographicData(city, point) { const demographicData = await arcgisRest.queryDemographicData({ studyAreas: [ { geometry: { x: point.longitude, y: point.latitude } } ], authentication: authentication }); if (!demographicData.results || demographicData.results.length === 0) { return; } if ( demographicData.results[0].value.FeatureSet.length > 0 && demographicData.results[0].value.FeatureSet[0].features.length > 0 ) { const attributes = demographicData.results[0].value.FeatureSet[0].features[0].attributes; showData(city, attributes, point); } }
Display data in a Popup
Once we have the results from the demographic query, show those results in a popup to display to the user.
-
Create the
show
function mentioned in the last step. If the function parameters are not valid, return. Otherwise, display a new popup and buffer graphic.Data Use dark colors for code blocks async function showData(city, attributes, point) { if (!city || !attributes || !point) { return; } }
-
Set the
dock
andOptions visible
on the map's default Popup to hide the collapse and dock buttons.Elements Use dark colors for code blocks async function showData(city, attributes, point) { if (!city || !attributes || !point) { return; } arcgisMap.popup.dockOptions = { buttonEnabled: false }; arcgisMap.popup.visibleElements = { collapseButton: false }; }
-
Define the
title
andcontent
of the Popup. In the title, enter the name of the city. In the content, display the demographics for that city.Use dark colors for code blocks async function showData(city, attributes, point) { if (!city || !attributes || !point) { return; } arcgisMap.popup.dockOptions = { buttonEnabled: false }; arcgisMap.popup.visibleElements = { collapseButton: false }; const title = `Global facts near ${city}`; const content = `Population: ${attributes.TOTPOP}<br>Males: ${attributes.TOTMALES} <br>Females: ${attributes.TOTFEMALES}<br>Average Household Size: ${attributes.AVGHHSZ}`; }
-
Open the popup with the title and content displayed at the given point.
Use dark colors for code blocks async function showData(city, attributes, point) { if (!city || !attributes || !point) { return; } arcgisMap.popup.dockOptions = { buttonEnabled: false }; arcgisMap.popup.visibleElements = { collapseButton: false }; const title = `Global facts near ${city}`; const content = `Population: ${attributes.TOTPOP}<br>Males: ${attributes.TOTMALES} <br>Females: ${attributes.TOTFEMALES}<br>Average Household Size: ${attributes.AVGHHSZ}`; arcgisMap.openPopup({ location: point, title: title, content: content }); }
-
Next, display a buffer around the map point where we get the demographics so the user knows what is included in the query. Create a one-mile buffer graphic using the geodesicBufferOperator and add it to the map.
Use dark colors for code blocks await geodesicBufferOperator.load(); const buffer = geodesicBufferOperator.execute(point, 1, { unit: "miles" }); const bufferGraphic = new Graphic({ geometry: buffer, symbol: new SimpleFillSymbol({ color: [50, 50, 50, 0.1], outline: { color: [0, 0, 0, 0.25], width: 0.5 } }) }); arcgisMap.graphics.removeAll(); arcgisMap.graphics.add(bufferGraphic);
Show the Popup on app load
To give users an idea of how the app works, show the popup on the app's initial load. Once the map component is ready, call get
for the center of the view.
-
Outside of the
show
function and beneath theData() arcgis
event listener, check if the map component isView Click ready
and call theget
function. If the map is notDemographic Data() ready
, add an event listener for thearcgis
event to call theView Ready Change get
function after the map is ready.Demographic Data() Use dark colors for code blocks if (!arcgisMap.ready) { arcgisMap.addEventListener( "arcgisViewReadyChange", () => { getDemographicData("Milan", arcgisMap.center); }, { once: true } ); } else { getDemographicData("Milan", arcgisMap.center); }
Run the App
In CodePen, run your code to display the map.
Click on the map or perform a search to get the demographics for a given city.
What's next?
Learn how to use additional API features and ArcGIS services in these tutorials: