Learn how to create and display a map with a basemap layer.
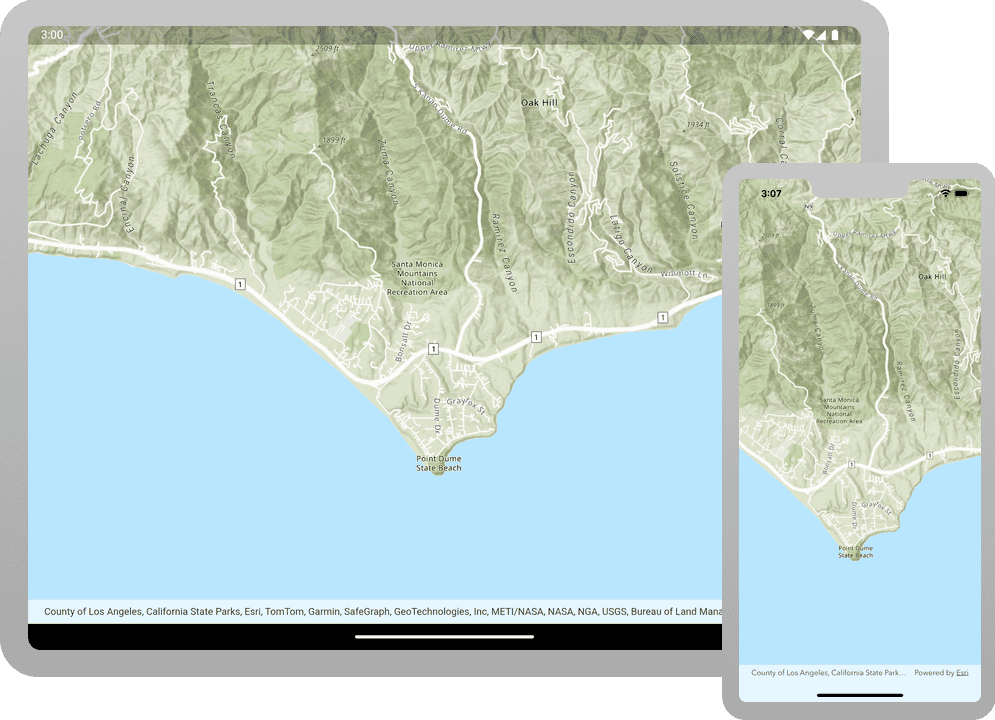
A map contains layers of geographic data. A map contains a basemap layer and, optionally, one or more data layers. You can display a specific area of a map by using a map view and setting the location and zoom level.
In this tutorial, you create and display a map of the Santa Monica Mountains in California using the topographic basemap layer.
The map and code will be used as the starting point for other 2D tutorials.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Your system meets the system requirements and you have set up your environment for Flutter development.
-
You need an IDE for Flutter - we recommend VS Code.
Steps
Create a new Flutter app
- Open VS Code, then select Open Folder... in the welcome tab. Choose your preferred project location.
- Go to View > Terminal.
- In the terminal window, use the following command to create a new Flutter app called display_a_map. Set your required target platform(s) and an org name, com.example.app.
Use dark colors for code blocks Copy flutter create -e display_a_map --platforms ios,android --org com.example.app
Add ArcGIS Maps SDK for Flutter
Add a dependency to the arcgis
package.
-
In VS Code's terminal, change the directory to your new project:
Use dark colors for code blocks Copy cd display_a_map
-
Run:
Use dark colors for code blocks Copy dart pub add arcgis_maps
-
Run:
Use dark colors for code blocks Copy flutter pub upgrade
-
Lastly, run:
Use dark colors for code blocks Copy dart run arcgis_maps install
Platform specific configuration
-
Update the minimum requirements by editing
android/app/build.gradle
file in your project:build.gradleUse dark colors for code blocks 8 9 10 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 28 29 30 31Change line Change line android { namespace = "com.example.app.display_a_map" compileSdk = flutter.compileSdkVersion ndkVersion = "25.2.9519653" compileOptions { sourceCompatibility = JavaVersion.VERSION_1_8 targetCompatibility = JavaVersion.VERSION_1_8 } kotlinOptions { jvmTarget = JavaVersion.VERSION_1_8 } defaultConfig { // TODO: Specify your own unique Application ID (https://developer.android.com/studio/build/application-id.html). applicationId = "com.example.app.display_a_map" // You can update the following values to match your application needs. // For more information, see: https://flutter.dev/to/review-gradle-config. minSdk = 26 targetSdk = flutter.targetSdkVersion versionCode = flutter.versionCode versionName = flutter.versionName }
-
Update the Kotlin version by editing
android/settings.gradle
file in your project:settings.gradleUse dark colors for code blocks 19 20 21 23Change line plugins { id "dev.flutter.flutter-plugin-loader" version "1.0.0" id "com.android.application" version "8.1.0" apply false id "org.jetbrains.kotlin.android" version "1.9.0" apply false }
-
Add a permission to
android/app/src/main/
to access online resources:Android Manifest.xml AndroidManifest.xmlUse dark colors for code blocks 2 3 5 6 7 8Add line. <manifest xmlns:android="http://schemas.android.com/apk/res/android"> <uses-permission android:name="android.permission.INTERNET" /> <application android:label="display_a_map" android:name="${applicationName}" android:icon="@mipmap/ic_launcher">
Get an access token
You need an access token to use the location services used in this tutorial.
-
Go to the Create an API key tutorial to obtain an access token using your ArcGIS Location Platform or ArcGIS Online account.
-
Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service.
-
Copy the access token as it will be used in the next step.
To learn more about other ways to get an access token, go to Types of authentication.
Set your API key
-
In VS Code, open
lib/main.dart
. -
Import the
arcgis
package._maps main.dartUse dark colors for code blocks 2 3 4Add line. import 'package:flutter/material.dart'; import 'package:arcgis_maps/arcgis_maps.dart';
-
In the
main()
function, define a constapi
and set its value with your access token.Key main.dartUse dark colors for code blocks 6 7 9 10 11Add line. void main() { const apiKey = ''; // Your access token here. runApp(const MainApp()); }
-
Set the
ArcGISEnvironment.apiKey
to theapi
constant.Key main.dartUse dark colors for code blocks 6 7 8 9 11 12 13Add line. void main() { const apiKey = ''; // Your access token here. ArcGISEnvironment.apiKey = apiKey; runApp(const MainApp()); }
-
Instantiate
Material
insideApp run
and set the named argument,App() home
, to an instance ofMain
.App main.dartUse dark colors for code blocks 17 18 19 20 21 22 23 24 28 29 30Change line Change line Change line void main() { const apiKey = ''; // Your access token here. ArcGISEnvironment.apiKey = apiKey; runApp( const MaterialApp( home: MainApp(), ), ); }
Add a map
Create a map that contains a topographic basemap layer. The map will be centered on the Santa Monica Mountains in California.
-
Refactor the template
Main
class definition to extendApp Stateful
. Hover your mouse over theWidget Stateless
keyword, right-click, select Refactor..., and chose Convert to StatefulWidget to refactor your code.Widget When working with the ArcGIS Maps SDK for Flutter, it is very common to require updates to application state, such as making updates to the UI in response to data changes. By implementing a stateful widget, your app is ready for this. It is also possible to simply display a map using a stateless widget implementation, see the Display map sample.
main.dartUse dark colors for code blocks 26 27 28 31 32 33Change line Change line Change line Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. class MainApp extends StatefulWidget { const MainApp({super.key}); @override State<MainApp> createState() => _MainAppState(); } class _MainAppState extends State<MainApp> { @override Widget build(BuildContext context) { return const MaterialApp( home: Scaffold( body: Center( child: Text('Hello World!'), ), ), ); } }
-
Remove the
Material
widget that is returned within the build method. This code was generated by the Flutter create template and will be replaced with code to return a widget that contains a map next.App main.dartUse dark colors for code blocks 25 26 27 28 29 30 31 32 33 34 35 36 37 45 46 47Remove line Remove line Remove line Remove line Remove line Remove line Remove line class MainApp extends StatefulWidget { const MainApp({super.key}); @override State<MainApp> createState() => _MainAppState(); } class _MainAppState extends State<MainApp> { @override Widget build(BuildContext context) { return const MaterialApp( home: Scaffold( body: Center( child: Text('Hello World!'), ), ), ); } }
-
Inside
_Main
class define a final class member variable,App State _map
, and initialize it withView Controller ArcGISMapViewController
by calling thecreateController()
on theArcGISMapView
class.The ArcGIS map view controller is a user interface control that displays two-dimensional (2D) geographic content defined by an
ArcGISMap
.main.dartUse dark colors for code blocks 49 50 52 53 54 55 56 57 58Add line. class _MainAppState extends State<MainApp> { final _mapViewController = ArcGISMapView.createController(); @override Widget build(BuildContext context) { } }
-
Within the build method, add an
ArcGISMapView
widget to the widget tree consisting ofScaffold
,Column
, andExpanded
. Set the named argument,controllerProvider
, to the class member variable_map
and theView Controller onMapViewReady
argument to a new method with the same name as the argument that you will define next.The
Scaffold
widget provides a basic material design visual layout structure, while theColumn
widget displays its children in a vertical array. TheArcGISMapView
widget can only be used within a widget that has a bounded size. Using it with unbounded size will cause the application to throw an exception. For example, to use anArcGIS
within aMap View Column
widget, which would give it unbounded size, would produce such an exception. Instead, you could wrap theArcGIS
in anMap View Expanded
widget to provide it proper bounds as illustrated in this step of the tutorial.main.dartUse dark colors for code blocks 49 50 51 52 53 54 55 68 69 70 71Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. class _MainAppState extends State<MainApp> { final _mapViewController = ArcGISMapView.createController(); @override Widget build(BuildContext context) { return Scaffold( body: Column( children: [ Expanded( child: ArcGISMapView( controllerProvider: () => _mapViewController, onMapViewReady: onMapViewReady, ), ), ], ), ); } }
-
Within
_Main
, define a new method:App State on
that does not return anything.Map View Ready() main.dartUse dark colors for code blocks 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 74 75Add line. Add line. Add line. class _MainAppState extends State<MainApp> { final _mapViewController = ArcGISMapView.createController(); @override Widget build(BuildContext context) { return Scaffold( body: Column( children: [ Expanded( child: ArcGISMapView( controllerProvider: () => _mapViewController, onMapViewReady: onMapViewReady, ), ), ], ), ); } void onMapViewReady() { } }
-
Within the new method define a final variable,
map
, and initialize it to anArcGISMap
with the ArcGIS Topographic basemap style.main.dartUse dark colors for code blocks 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 74 75 76 77Add line. class _MainAppState extends State<MainApp> { final _mapViewController = ArcGISMapView.createController(); @override Widget build(BuildContext context) { return Scaffold( body: Column( children: [ Expanded( child: ArcGISMapView( controllerProvider: () => _mapViewController, onMapViewReady: onMapViewReady, ), ), ], ), ); } void onMapViewReady() { final map = ArcGISMap.withBasemapStyle(BasemapStyle.arcGISTopographic); } }
-
Set the ArcGIS map view controller's
arcGISMap
property to themap
. In addition, callsetViewpoint()
to zoom to the Santa Monica Mountains in California.main.dartUse dark colors for code blocks 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 83 84 85 86Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. class _MainAppState extends State<MainApp> { final _mapViewController = ArcGISMapView.createController(); @override Widget build(BuildContext context) { return Scaffold( body: Column( children: [ Expanded( child: ArcGISMapView( controllerProvider: () => _mapViewController, onMapViewReady: onMapViewReady, ), ), ], ), ); } void onMapViewReady() { final map = ArcGISMap.withBasemapStyle(BasemapStyle.arcGISTopographic); _mapViewController.arcGISMap = map; _mapViewController.setViewpoint( Viewpoint.withLatLongScale( latitude: 34.02700, longitude: -118.80500, scale: 72000, ), ); } }
Run the app
-
Make sure you have an Android emulator, iOS simulator or physical device configured and running.
-
In VS Code, select Run > Run Without Debugging.
Run download solution
If you have downloaded the project solution, follow these steps to run the application.
-
Open the extracted project in VS code.
-
In VS Code's terminal, run:
Use dark colors for code blocks Copy flutter pub upgrade
-
Run:
Use dark colors for code blocks Copy dart run arcgis_maps install
-
Make sure to get an access token and set your API key in the
lib/main.dart
source code file. -
Make sure you have an Android emulator, iOS simulator or physical device configured and running.
-
In VS Code, select Run > Run Without Debugging.