ST_BinId takes a bin column and returns a long column. The long column represents the unique identifier of each bin.
ST_BinId can be used to save a bin column to a data source for storage or for interoperability with other systems that use bins. Bin functions like ST_SquareBin, ST_HexBin, and ST_H3Bin can be called with a bin ID to recreate a bin column when reading from data sources. For example, you could create H3 bins from geometries in GeoAnalytics for Microsoft Fabric and then use ST_BinId to convert the bins to IDs for use in the H3 Python API.
Function | Syntax |
---|---|
Python | bin |
SQL | ST |
Scala | bin |
For more details, go to the GeoAnalytics for Microsoft Fabric API reference for bin_id.
Python and SQL Examples
from geoanalytics_fabric.sql import functions as ST, Polygon
data = [(Polygon([[[0,0],[0,10],[10,10],[10,0],[0,0]]]),),
(Polygon([[[20,0],[30,20],[40,0],[20,0]]]),),
(Polygon([[[20,30],[25,35],[30,30],[20,30]]]),)]
df = spark.createDataFrame(data,["polygon"]) \
.withColumn("bin_ids", ST.bin_id(ST.square_bin("polygon", 4)))
df.select('bin_ids').show()
# Save the bin id
df.select('bin_ids').write.parquet(r"C:\data\bin_ids")
# Load the bin id
bin_ids = spark.read.format("parquet").load(r"C:\data\bin_ids") \
.select(ST.bin_geometry(ST.square_bin("bin_ids", 4)).alias("bin_geometry"))
ax = df.st.plot("polygon", facecolor="none", edgecolor="red")
bin_ids.st.plot("bin_geometry", ax=ax, facecolor="none", edgecolor="blue")
+-----------+
| bin_ids|
+-----------+
| 4294967297|
|30064771073|
|25769803783|
+-----------+
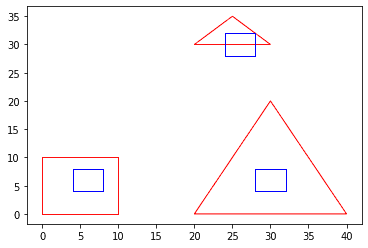
Scala Example
import com.esri.geoanalytics.geometry._
import com.esri.geoanalytics.sql.{functions => ST}
case class PolygonRow(polygon: Polygon)
val data = Seq(PolygonRow(Polygon(Point(0, 0), Point(0, 10), Point(10, 10), Point(10, 0), Point(0, 0))),
PolygonRow(Polygon(Point(20, 0), Point(30, 20), Point(40, 0), Point(20, 0))),
PolygonRow(Polygon(Point(20, 30), Point(25, 35), Point(30, 30), Point(20, 30))))
val df = spark.createDataFrame(data)
.withColumn("bin_ids", ST.binId(ST.squareBin($"polygon", 4)))
df.select("bin_ids").show()
+-----------+
| bin_ids|
+-----------+
| 4294967297|
|30064771073|
|25769803783|
+-----------+
Version table
Release | Notes |
---|---|
1.0.0-beta | Python, SQL, and Scala functions introduced |