ST_Buffer takes a geometry column and a numeric distance value and returns a polygon column. The resulting buffer polygons represent the area that is less than or equal to the specified planar distance from each input geometry. The distance can be specified as a single value or a numeric column. The distance value should be in the same units as the input geometry. For example, if your input geometry is in a spatial reference that uses meters, you should specify the distance in meters. To create a buffer polygon using geodesic distance calculations use ST_GeodesicBuffer.
Function | Syntax |
---|---|
Python | buffer(geometry, distance) |
SQL | ST |
Scala | buffer(geometry, distance) |
For more details, go to the GeoAnalytics for Microsoft Fabric API reference for buffer.
This function implements the OpenGIS Simple Features Implementation Specification for SQL 1.2.1.
Python and SQL Examples
from geoanalytics_fabric.sql import functions as ST
data = [
("POINT (10 30)",),
("MULTIPOINT (0 0, 5 5, 0 5)", ),
("LINESTRING (15 15, 10 15, 12 2)", ),
("POLYGON ((20 30, 18 28, 22 35, 40 20))", )
]
df = spark.createDataFrame(data, ["wkt"])\
.select(ST.geom_from_text("wkt").alias("geometry"))
df = df.withColumn("buffer", ST.buffer("geometry", 2))
ax = df.st.plot("geometry", facecolor="none", edgecolor="red")
df.st.plot("buffer", ax=ax, facecolor="none", edgecolor="blue")
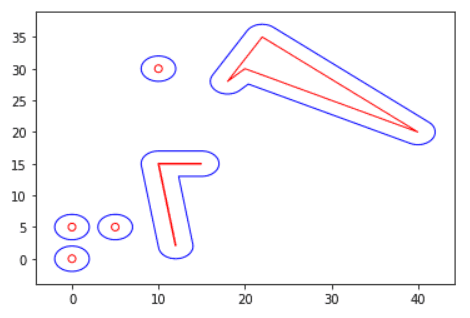
Scala Example
import com.esri.geoanalytics.sql.{functions => ST}
import org.apache.spark.sql.{functions => F}
case class GeometryRow(wkt: String)
val data = Seq(GeometryRow("POINT (10 30)"),
GeometryRow("MULTIPOINT (0 0, 5 5, 0 5)"),
GeometryRow("LINESTRING (15 15, 10 15, 12 2)"),
GeometryRow("POLYGON ((20 30, 18 28, 22 35, 40 20))"))
val df = spark.createDataFrame(data)
.select(ST.geomFromText($"wkt").alias("geometry"))
.withColumn("buffer", ST.buffer($"geometry", 2))
.withColumn("buffer_area", F.round(ST.area($"buffer"), 3))
df.select("buffer", "buffer_area").show()
+--------------------+-----------+
| buffer|buffer_area|
+--------------------+-----------+
|{"rings":[[[12,30...| 12.557|
|{"rings":[[[2,0],...| 37.672|
|{"rings":[[[12,0]...| 83.951|
|{"rings":[[[40,18...| 188.535|
+--------------------+-----------+
Version table
Release | Notes |
---|---|
1.0.0-beta | Python, SQL, and Scala functions introduced |