ST_Envelope takes a geometry column and returns a polygon column. The function returns an envelope for each geometry in the input column, where an envelope is the smallest rectangle that encompasses the input geometry and aligns to the x-axis and y-axis. To find the smallest rectangle that encompasses a geometry but is not axis-aligned, use ST_MinBoundingBox. If the input geometry is a single point, the function will create a degenerate polygon at the location of the point.
Function | Syntax |
---|---|
Python | envelope(geometry) |
SQL | ST |
Scala | envelope(geometry) |
For more details, go to the GeoAnalytics for Microsoft Fabric API reference for envelope.
This function implements the OpenGIS Simple Features Implementation Specification for SQL 1.2.1.
Python and SQL Examples
from geoanalytics_fabric.sql import functions as ST
data = [
("POINT (10 30)",),
("MULTIPOINT (0 0, 5 5, 0 5)", ),
("LINESTRING (15 15, 10 15, 12 2)", ),
("POLYGON ((20 30, 18 28, 22 35, 40 20))", )
]
df = spark.createDataFrame(data, ["wkt"])\
.select(ST.geom_from_text("wkt").alias("geometry"))
df = df.withColumn("envelope", ST.envelope("geometry"))
ax = df.st.plot("geometry", facecolor="none", edgecolor="red")
df.st.plot("envelope", ax=ax, facecolor="none", edgecolor="blue")
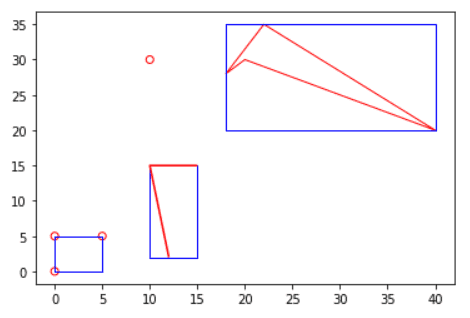
Scala Example
import com.esri.geoanalytics.sql.{functions => ST}
case class GeometryRow(wkt: String)
val data = Seq(GeometryRow("POINT (10 30)"),
GeometryRow("MULTIPOINT (0 0, 5 5, 0 5)"),
GeometryRow("LINESTRING (15 15, 10 15, 12 2)"),
GeometryRow("POLYGON ((20 30, 18 28, 22 35, 40 20))"))
val df = spark.createDataFrame(data)
.select(ST.geomFromText($"wkt").alias("geometry"))
.withColumn("envelope", ST.envelope($"geometry"))
df.select("envelope").show(truncate = false)
+-----------------------------------------------------+
|envelope |
+-----------------------------------------------------+
|{"rings":[[[10,30],[10,30],[10,30],[10,30]]]} |
|{"rings":[[[0,0],[0,5],[5,5],[5,0],[0,0]]]} |
|{"rings":[[[10,2],[10,15],[15,15],[15,2],[10,2]]]} |
|{"rings":[[[18,20],[18,35],[40,35],[40,20],[18,20]]]}|
+-----------------------------------------------------+
Version table
Release | Notes |
---|---|
1.0.0-beta | Python, SQL, and Scala functions introduced |