ST_GeohashBin takes a geometry column and a numeric precision and returns a bin column. The output column contains a single Geohash bin for each record in the input column. Geohash encodes a geographic location into a short string of letters and digits. It is a hierarchical spatial data structure which subdivides space into buckets of grid shape. The specified precision determines the size of each Geohash bin.
When the input is a multipoint, linestring, or polygon geometry, ST_GeohashBin may choose a different bin than the bin chosen by other Geohash implementations.
ST_GeohashBin requires the spatial reference to be set to World Geodetic System 1984 (EPSG:4326). If the input geometry is in a different spatial reference, the function automatically transforms the geometry into World Geodetic System 1984. To learn more about spatial references, see Coordinate systems and transformations.
ST_BinGeometry can be used to obtain the geometry of the result bin. You can also use the function ST_BinId to get the id of the result bin.
This function can also be called with a string column representing the ID of the bin (see ST_BinId). The bin ID will be cast to a bin column.
Function | Syntax |
---|---|
Python | geohash |
SQL | ST |
Python | geohash |
For more details, go to the GeoAnalytics for Microsoft Fabric API reference for geohash_bin.
Python and SQL Examples
from geoanalytics_fabric.sql import functions as ST
data = [
('POINT (1 1)',),
('LINESTRING (0 0, 0 0.4, 0 0.8, 0 1)',),
("POLYGON ((0.5 0.5, 0.5 0.8, 0.8 0.8, 0.8 0.5, 0.5 0.5))", )
]
df = spark.createDataFrame(data, ["wkt"])\
.withColumn("geometry", ST.geom_from_text("wkt", srid=4326))\
.withColumn("geohash_bin", ST.geohash_bin("geometry", 5)) \
.withColumn("bin_geometry", ST.bin_geometry("geohash_bin")) \
.withColumn("bin_id", ST.bin_id("geohash_bin"))
df.select("bin_id").show()
ax = df.st.plot("geometry", facecolor="none", edgecolor="red")
df.st.plot("bin_geometry", ax=ax, facecolor="none", edgecolor="blue")
+------+
|bin_id|
+------+
| s00tw|
| s004b|
| s007w|
+------+
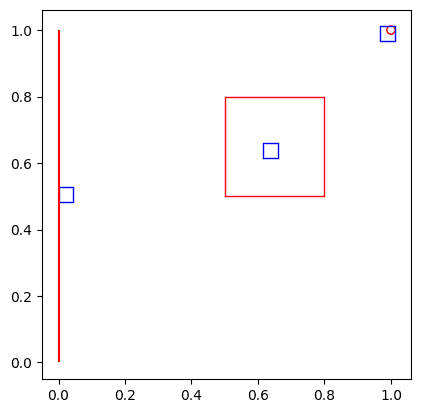
from geoanalytics_fabric.sql import functions as ST
data = [('s00wq',), ('s004b',), ('s007w',)]
df = spark.createDataFrame(data, ["bin_id"]) \
.withColumn("geohash_bin", ST.geohash_bin("bin_id", 5)) \
.withColumn("bin_geometry", ST.bin_geometry("geohash_bin"))
ax = df.st.plot("bin_geometry", facecolor="none", edgecolor="blue")
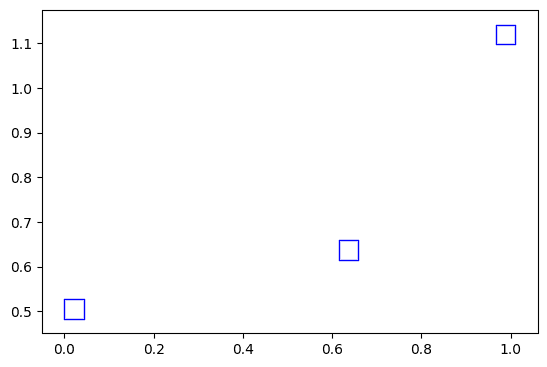
Scala Examples
import com.esri.geoanalytics.sql.{functions => ST}
import org.apache.spark.sql.{functions => F}
case class GeometryRow(wkt: String)
val data = Seq(GeometryRow("POINT (1 1)"),
GeometryRow("LINESTRING (0 0, 0 0.4, 0 0.8, 0 1)"),
GeometryRow("POLYGON ((0.5 0.5, 0.5 0.8, 0.8 0.8, 0.8 0.5, 0.5 0.5))"))
val df = spark.createDataFrame(data)
.withColumn("geometry", ST.geomFromText($"wkt", F.lit(4326)))
.withColumn("geohash_bin", ST.geohashBin($"geometry", F.lit(5)))
.withColumn("bin_id", ST.binId($"geohash_bin"))
df.select("geohash_bin", "bin_id").show()
+------------------+------+
| geohash_bin|bin_id|
+------------------+------+
|bin#70746701332837| s00tw|
|bin#70368744210613| s004b|
|bin#70609262379237| s007w|
+------------------+------+
import com.esri.geoanalytics.sql.{functions => ST}
case class BinID(binID: String)
val data = Seq(BinID("s00wq"),
BinID("s004b"),
BinID("s007w"))
val df = spark.createDataFrame(data)
.withColumn("geohash_bin", ST.geohashBin($"binID", F.lit(5)))
df.select("geohash_bin").show()
+------------------+
| geohash_bin|
+------------------+
|bin#70746701332885|
|bin#70368744210613|
|bin#70609262379237|
+------------------+
Version table
Release | Notes |
---|---|
1.0.0-beta | Python, SQL, and Scala functions introduced |