ST_PointOnSurface takes a geometry column and returns a point column. The function returns a point that lies on the surface of linestring or polygon geometries.
Function | Syntax |
---|---|
Python | point |
SQL | ST |
Scala | point |
For more details, go to the GeoAnalytics for Microsoft Fabric API reference for point_on_surface.
This function implements the OpenGIS Simple Features Implementation Specification for SQL 1.2.1.
Python and SQL Examples
from geoanalytics_fabric.sql import functions as ST
data = [
("POINT (10 30)",),
("MULTIPOINT (0 0, 5 5, 0 5)", ),
("LINESTRING (15 15, 10 15, 12 2)", ),
("POLYGON ((20 30, 18 28, 22 35, 40 20))", )
]
df = spark.createDataFrame(data, ["wkt"])\
.select(ST.geom_from_text("wkt").alias("geometry"))
df = df.withColumn("point_on_surface", ST.point_on_surface("geometry"))
ax = df.st.plot("geometry", facecolor="none", edgecolor="red")
df.st.plot("point_on_surface", ax=ax, facecolor="none", edgecolor="blue")
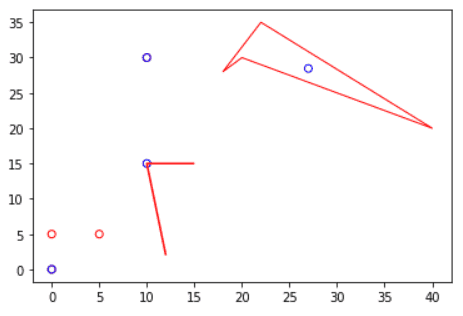
Scala Example
Scala
import com.esri.geoanalytics.sql.{functions => ST}
case class GeometryRow(wkt: String)
val data = Seq(GeometryRow("POINT (10 30)"),
GeometryRow("MULTIPOINT (0 0, 5 5, 0 5)"),
GeometryRow("LINESTRING (15 15, 10 15, 12 2)"),
GeometryRow("POLYGON ((20 30, 18 28, 22 35, 40 20))"))
val df = spark.createDataFrame(data)
.select(ST.geomFromText($"wkt").alias("geometry"))
.withColumn("point_on_surface", ST.pointOnSurface($"geometry"))
df.select("point_on_surface").show(truncate = false)
Result
+-----------------------------------------------+
|point_on_surface |
+-----------------------------------------------+
|{"x":10,"y":30} |
|{"x":0,"y":0} |
|{"x":10,"y":15} |
|{"x":26.984126984126984,"y":28.460317460317462}|
+-----------------------------------------------+
Version table
Release | Notes |
---|---|
1.0.0-beta | Python, SQL, and Scala functions introduced |