ST_Scale takes a geometry column and two numbers and returns a geometry column. The result is the input geometry scaled to a new size in the x and y directions by the specified factors.
Function | Syntax |
---|---|
Python | scale(geometry, x |
SQL | ST |
Scala | scale(geometry, x |
For more details, go to the GeoAnalytics for Microsoft Fabric API reference for scale.
Python and SQL Examples
from geoanalytics_fabric.sql import functions as ST
data = [
("POINT (0.5 0.5)",),
("MULTIPOINT (4.0 4.5, 5.0 4.5, 5.5 5.0)",),
("LINESTRING (2.0 0.0, 3.0 1.0, 4.0 0.5)", ),
("POLYGON ((5.0 2.0, 6.0 1.0, 5.5 1.0, 4.0 2.0, 5.0 2.0))", )
]
df = spark.createDataFrame(data, ["wkt"]) \
.withColumn("geometry", ST.geom_from_text("wkt"))
df_scale = df.withColumn("scale", ST.scale(geometry="geometry",
x_scale_factor=2,
y_scale_factor=2))
ax = df_scale.st.plot("geometry", facecolor="none", edgecolor="red")
df_scale.st.plot("scale", ax=ax, facecolor="none", edgecolor="blue")
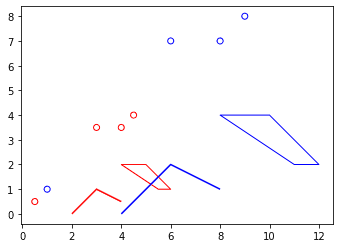
Scala Example
Scala
import com.esri.geoanalytics.sql.{functions => ST}
case class GeometryRow(wkt: String)
val data = Seq(GeometryRow("POINT (0.5 0.5)"),
GeometryRow("MULTIPOINT (4.0 4.5, 5.0 4.5, 5.5 5.0)"),
GeometryRow("LINESTRING (2.0 0.0, 3.0 1.0, 4.0 0.5)"),
GeometryRow("POLYGON ((5.0 2.0, 6.0 1.0, 5.5 1.0, 4.0 2.0, 5.0 2.0))"))
val df = spark.createDataFrame(data)
.withColumn("geometry", ST.geomFromText($"wkt"))
.withColumn("scale", ST.scale($"geometry", 2, 2))
df.select("scale").show(truncate = false)
Result
+-----------------------------------------------+
|scale |
+-----------------------------------------------+
|{"x":1,"y":1} |
|{"points":[[8,9],[10,9],[11,10]]} |
|{"paths":[[[4,0],[6,2],[8,1]]]} |
|{"rings":[[[10,4],[12,2],[11,2],[8,4],[10,4]]]}|
+-----------------------------------------------+
Version table
Release | Notes |
---|---|
1.0.0-beta | Python, SQL, and Scala functions introduced |